mirror of
https://github.com/m-lamonaca/dev-notes.git
synced 2025-06-07 18:27:13 +00:00
Add ASP.NET Core Filters & Middlewares notes
This commit is contained in:
parent
f72223374e
commit
a2385fbff5
2 changed files with 341 additions and 0 deletions
134
.NET/ASP.NET/Filters.md
Normal file
134
.NET/ASP.NET/Filters.md
Normal file
|
@ -0,0 +1,134 @@
|
|||
# [Filters](https://docs.microsoft.com/en-us/aspnet/core/mvc/controllers/filters)
|
||||
|
||||
**Filters** in ASP.NET Core allow code to be run *before* or *after* specific stages in the request processing pipeline.
|
||||
|
||||
Built-in filters handle tasks such as:
|
||||
|
||||
- Authorization (preventing access to resources a user isn't authorized for).
|
||||
- Response caching (short-circuiting the request pipeline to return a cached response).
|
||||
|
||||
Custom filters can be created to handle cross-cutting concerns. Examples of cross-cutting concerns include error handling, caching, configuration, authorization, and logging. Filters avoid duplicating code.
|
||||
|
||||
## **How filters work**
|
||||
|
||||
Filters run within the *ASP.NET Core action invocation pipeline*, sometimes referred to as the *filter pipeline*. The filter pipeline runs after ASP.NET Core selects the action to execute.
|
||||
|
||||
![1][filter-pipeline-1] ![2][filter-pipeline-2]
|
||||
|
||||
[filter-pipeline-1]: https://docs.microsoft.com/it-it/aspnet/core/mvc/controllers/filters/_static/filter-pipeline-1.png
|
||||
[filter-pipeline-2]: https://docs.microsoft.com/en-gb/aspnet/core/mvc/controllers/filters/_static/filter-pipeline-2.png
|
||||
|
||||
## **Filter types**
|
||||
|
||||
Each filter type is executed at a different stage in the filter pipeline:
|
||||
|
||||
- **Authorization filters** run first and are used to determine whether the user is authorized for the request. Authorization filters short-circuit the pipeline if the request is not authorized.
|
||||
- **Resource filters**:
|
||||
- Run after authorization.
|
||||
- `OnResourceExecuting` runs code before the rest of the filter pipeline. For example, `OnResourceExecuting` runs code before model binding.
|
||||
- `OnResourceExecuted` runs code after the rest of the pipeline has completed.
|
||||
- **Action filters**:
|
||||
- Run code immediately before and after an action method is called.
|
||||
- Can change the arguments passed into an action.
|
||||
- Can change the result returned from the action.
|
||||
- Are **not** supported in Razor Pages.
|
||||
- **Exception filters** apply global policies to unhandled exceptions that occur before the response body has been written to.
|
||||
- **Result filters** run code immediately before and after the execution of action results. They run only when the action method has executed successfully. They are useful for logic that must surround view or formatter execution.
|
||||
|
||||
## **Implementation**
|
||||
|
||||
Filters support both synchronous and asynchronous implementations through different interface definitions.
|
||||
|
||||
For example, `OnActionExecuting` is called before the action method is called. `OnActionExecuted` is called after the action method returns.
|
||||
Asynchronous filters define an `On-Stage-ExecutionAsync` method, for example `OnActionExecutionAsync`.
|
||||
|
||||
Interfaces for multiple filter stages can be implemented in a single class.
|
||||
|
||||
## **Built-in filter attributes**
|
||||
|
||||
ASP.NET Core includes built-in *attribute-based* filters that can be subclassed and customized.
|
||||
Several of the filter interfaces have corresponding attributes that can be used as base classes for custom implementations.
|
||||
|
||||
Filter attributes:
|
||||
|
||||
- [ActionFilterAttribute](https://docs.microsoft.com/en-us/dotnet/api/microsoft.aspnetcore.mvc.filters.actionfilterattribute)
|
||||
- [ExceptionFilterAttribute](https://docs.microsoft.com/en-us/dotnet/api/microsoft.aspnetcore.mvc.filters.exceptionfilterattribute)
|
||||
- [ResultFilterAttribute](https://docs.microsoft.com/en-us/dotnet/api/microsoft.aspnetcore.mvc.filters.resultfilterattribute)
|
||||
- [FormatFilterAttribute](https://docs.microsoft.com/en-us/dotnet/api/microsoft.aspnetcore.mvc.formatfilterattribute)
|
||||
- [ServiceFilterAttribute](https://docs.microsoft.com/en-us/dotnet/api/microsoft.aspnetcore.mvc.servicefilterattribute)
|
||||
- [TypeFilterAttribute](https://docs.microsoft.com/en-us/dotnet/api/microsoft.aspnetcore.mvc.typefilterattribute)
|
||||
|
||||
## **Filter scopes**
|
||||
|
||||
A filter can be added to the pipeline at one of three *scopes*:
|
||||
|
||||
- Using an attribute on a controller action. Filter attributes cannot be applied to Razor Pages handler methods.
|
||||
|
||||
```cs
|
||||
// services.AddScoped<CustomActionFilterAttibute>();
|
||||
[ServiceFilter(typeof(CustomActionFilterAttribute))]
|
||||
public IActionResult Index()
|
||||
{
|
||||
return Content("Header values by configuration.");
|
||||
}
|
||||
```
|
||||
|
||||
- Using an attribute on a controller or Razor Page.
|
||||
|
||||
```cs
|
||||
// services.AddControllersWithViews(options => { options.Filters.Add(new CustomResponseFilterAttribute(args)); });
|
||||
[CustomResponseFilterAttribute(args)]
|
||||
public class SampleController : Controller
|
||||
|
||||
// or
|
||||
|
||||
[CustomResponseFilterAttribute(args)]
|
||||
[ServiceFilter(typeof(CustomActionFilterAttribute))]
|
||||
public class IndexModel : PageModel
|
||||
```
|
||||
|
||||
- Globally for all controllers, actions, and Razor Pages.
|
||||
|
||||
```cs
|
||||
public void ConfigureServices(IServiceCollection services)
|
||||
{
|
||||
services.AddControllersWithViews(options =>
|
||||
{
|
||||
options.Filters.Add(typeof(CustomActionFilter));
|
||||
});
|
||||
}
|
||||
```
|
||||
|
||||
## Filter Order of Execution
|
||||
|
||||
When there are multiple filters for a particular stage of the pipeline, scope determines the default order of filter execution. Global filters surround class filters, which in turn surround method filters.
|
||||
|
||||
As a result of filter nesting, the *after* code of filters runs in the reverse order of the *before* code. The filter sequence:
|
||||
|
||||
- The *before* code of global filters.
|
||||
- The *before* code of controller and Razor Page filters.
|
||||
- The *before* code of action method filters.
|
||||
- The *after* code of action method filters.
|
||||
- The *after* code of controller and Razor Page filters.
|
||||
- The *after* code of global filters.
|
||||
|
||||
### Cancellation and Short-Circuiting
|
||||
|
||||
The filter pipeline can be short-circuited by setting the `Result` property on the `ResourceExecutingContext` parameter provided to the filter method.
|
||||
|
||||
```cs
|
||||
public class ShortCircuitingResourceFilterAttribute : Attribute, IResourceFilter
|
||||
{
|
||||
public void OnResourceExecuting(ResourceExecutingContext context)
|
||||
{
|
||||
context.Result = new ContentResult()
|
||||
{
|
||||
Content = "Resource unavailable - header not set."
|
||||
};
|
||||
}
|
||||
|
||||
public void OnResourceExecuted(ResourceExecutedContext context)
|
||||
{
|
||||
}
|
||||
}
|
||||
```
|
207
.NET/ASP.NET/Middleware.md
Normal file
207
.NET/ASP.NET/Middleware.md
Normal file
|
@ -0,0 +1,207 @@
|
|||
# [Middleware](https://docs.microsoft.com/en-us/aspnet/core/fundamentals/middleware)
|
||||
|
||||
Middleware is software that's assembled into an app pipeline to handle requests and responses. Each component:
|
||||
|
||||
- Chooses whether to pass the request to the next component in the pipeline.
|
||||
- Can perform work before and after the next component in the pipeline.
|
||||
|
||||
Request delegates are used to build the request pipeline. The request delegates handle each HTTP request.
|
||||
|
||||
Request delegates are configured using [Run][Run_docs], [Map][Map_docs], and [Use][Use_docs] extension methods.
|
||||
|
||||
An individual request delegate can be specified in-line as an anonymous method (called in-line middleware), or it can be defined in a reusable class.
|
||||
These reusable classes and in-line anonymous methods are *middleware*, also called *middleware components*.
|
||||
|
||||
Each middleware component in the request pipeline is responsible for invoking the next component in the pipeline or short-circuiting the pipeline.
|
||||
When a middleware short-circuits, it's called a *terminal middleware* because it prevents further middleware from processing the request.
|
||||
|
||||
[Use_docs]: https://docs.microsoft.com/en-us/dotnet/api/microsoft.aspnetcore.builder.useextensions.use
|
||||
[Run_docs]: https://docs.microsoft.com/en-us/dotnet/api/microsoft.aspnetcore.builder.runextensions.run
|
||||
[Map_docs]: https://docs.microsoft.com/en-us/dotnet/api/microsoft.aspnetcore.builder.mapextensions.map
|
||||
|
||||
## Middleware Pipeline
|
||||
|
||||
The ASP.NET Core request pipeline consists of a sequence of request delegates, called one after the other.
|
||||
|
||||
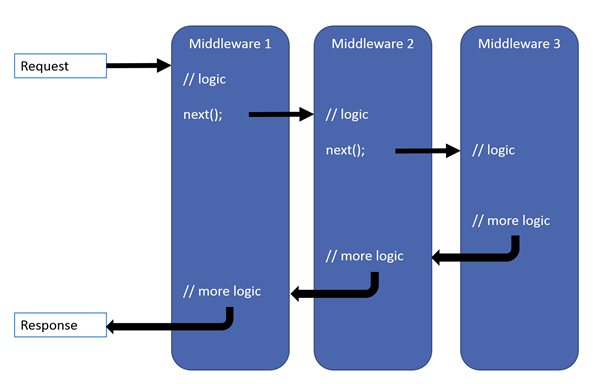
|
||||
|
||||
Each delegate can perform operations before and after the next delegate. Exception-handling delegates should be called early in the pipeline, so they can catch exceptions that occur in later stages of the pipeline. It's possible to chain multiple request delegates together with `Use`.
|
||||
|
||||
The *next* parameter represents the next delegate in the pipeline. It's possible to short-circuit the pipeline by *not calling* the next parameter.
|
||||
When a delegate doesn't pass a request to the next delegate, it's called *short-circuiting the request pipeline*.
|
||||
Short-circuiting is often desirable because it avoids unnecessary work.
|
||||
|
||||
It's possible to perform actions both *before* and *after* the next delegate:
|
||||
|
||||
```cs
|
||||
public class Startup
|
||||
{
|
||||
public void Configure(IApplicationBuilder app)
|
||||
{
|
||||
// "inline" middleware, best if in own class
|
||||
app.Use(async (context, next) =>
|
||||
{
|
||||
// Do work that doesn't write to the Response.
|
||||
await next.Invoke();
|
||||
// Do logging or other work that doesn't write to the Response.
|
||||
});
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
`Run` delegates don't receive a next parameter. The first `Run` delegate is always terminal and terminates the pipeline.
|
||||
|
||||
```cs
|
||||
public class Startup
|
||||
{
|
||||
public void Configure(IApplicationBuilder app)
|
||||
{
|
||||
// "inline" middleware, best if in own class
|
||||
app.Use(async (context, next) =>
|
||||
{
|
||||
// Do work that doesn't write to the Response.
|
||||
await next.Invoke();
|
||||
// Do logging or other work that doesn't write to the Response.
|
||||
});
|
||||
|
||||
app.Run(async context =>
|
||||
{
|
||||
// no invocation of next
|
||||
});
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Middleware Order
|
||||
|
||||

|
||||

|
||||
|
||||
The Endpoint middleware executes the filter pipeline for the corresponding app type.
|
||||
|
||||
The order that middleware components are added in the `Startup.Configure` method defines the order in which the middleware components are invoked on requests and the reverse order for the response. The order is **critical** for security, performance, and functionality.
|
||||
|
||||
```cs
|
||||
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
|
||||
{
|
||||
if (env.IsDevelopment())
|
||||
{
|
||||
app.UseDeveloperExceptionPage();
|
||||
app.UseDatabaseErrorPage();
|
||||
}
|
||||
else
|
||||
{
|
||||
app.UseExceptionHandler("/Error");
|
||||
app.UseHsts();
|
||||
}
|
||||
|
||||
app.UseHttpsRedirection();
|
||||
app.UseStaticFiles();
|
||||
// app.UseCookiePolicy();
|
||||
|
||||
app.UseRouting();
|
||||
// app.UseRequestLocalization();
|
||||
// app.UseCors();
|
||||
|
||||
app.UseAuthentication();
|
||||
app.UseAuthorization();
|
||||
// app.UseSession();
|
||||
// app.UseResponseCompression();
|
||||
// app.UseResponseCaching();
|
||||
|
||||
app.UseEndpoints(endpoints =>
|
||||
{
|
||||
endpoints.MapRazorPages();
|
||||
endpoints.MapControllerRoute(
|
||||
name: "default",
|
||||
pattern: "{controller=Home}/{action=Index}/{id?}");
|
||||
});
|
||||
}
|
||||
```
|
||||
|
||||
[Built-in Middleware](https://docs.microsoft.com/en-us/aspnet/core/fundamentals/middleware/#built-in-middleware)
|
||||
|
||||
## Branching the Middleware Pipeline
|
||||
|
||||
`Map` extensions are used as a convention for branching the pipeline. `Map` branches the request pipeline based on matches of the given request path.
|
||||
If the request path starts with the given path, the branch is executed.
|
||||
|
||||
When `Map` is used, the matched path segments are removed from `HttpRequest.Path` and appended to `HttpRequest.PathBase` for each request.
|
||||
|
||||
`MapWhen` branches the request pipeline based on the result of the given predicate.
|
||||
Any *predicate* of type `Func<HttpContext, bool>` can be used to map requests to a new branch of the pipeline.
|
||||
|
||||
`UseWhen` also branches the request pipeline based on the result of the given predicate.
|
||||
Unlike with `MapWhen`, this branch is rejoined to the main pipeline if it doesn't short-circuit or contain a terminal middleware.
|
||||
|
||||
## Custom Middleware Classes
|
||||
|
||||
Middleware is generally encapsulated in a class and exposed with an extension method.
|
||||
|
||||
```cs
|
||||
using Microsoft.AspNetCore.Http;
|
||||
using System.Globalization;
|
||||
using System.Threading.Tasks;
|
||||
|
||||
namespace <App>
|
||||
{
|
||||
public class CustomMiddleware
|
||||
{
|
||||
private readonly RequestDelegate _next;
|
||||
|
||||
public RequestCultureMiddleware(RequestDelegate next)
|
||||
{
|
||||
_next = next;
|
||||
}
|
||||
|
||||
public async Task InvokeAsync(HttpContext context)
|
||||
{
|
||||
// Do work that doesn't write to the Response.
|
||||
await _next(context); // Call the next delegate/middleware in the pipeline
|
||||
// Do logging or other work that doesn't write to the Response.
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
The middleware class **must** include:
|
||||
|
||||
- A public constructor with a parameter of type [RequestDelegate][RequestDelegate_docs].
|
||||
- A public method named `Invoke` or `InvokeAsync`. This method must:
|
||||
- Return a `Task`.
|
||||
- Accept a first parameter of type [HttpContext][HttpConrext_Docs].
|
||||
|
||||
[RequestDelegate_docs]: https://docs.microsoft.com/en-us/dotnet/api/microsoft.aspnetcore.http.requestdelegate
|
||||
[HttpConrext_Docs]: https://docs.microsoft.com/en-us/dotnet/api/microsoft.aspnetcore.http.httpcontext
|
||||
|
||||
## Middleware Extension Methods
|
||||
|
||||
```cs
|
||||
using Microsoft.AspNetCore.Builder;
|
||||
|
||||
namespace <App>
|
||||
{
|
||||
public static class MiddlewareExtensions
|
||||
{
|
||||
public static IApplicationBuilder UseCustom(this IApplicationBuilder builder)
|
||||
{
|
||||
return builder.UseMiddleware<CustomMiddleware>();
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
```cs
|
||||
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
|
||||
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
|
||||
{
|
||||
// other middlewares
|
||||
|
||||
app.UseCustom(); // add custom middleware in the pipeline
|
||||
|
||||
app.UseEndpoints(endpoints =>
|
||||
{
|
||||
endpoints.MapControllers();
|
||||
});
|
||||
}
|
||||
```
|
Loading…
Add table
Add a link
Reference in a new issue