mirror of
https://github.com/m-lamonaca/dev-notes.git
synced 2025-05-14 23:24:46 +00:00
Rename .NET folder to DotNet
This commit is contained in:
parent
68723844c3
commit
d2148a38ee
32 changed files with 0 additions and 0 deletions
35
DotNet/Xamarin/App.xaml.cs.md
Normal file
35
DotNet/Xamarin/App.xaml.cs.md
Normal file
|
@ -0,0 +1,35 @@
|
|||
# App.xaml.cs
|
||||
|
||||
This `App` class is defined as `public` and derives from the Xamarin.Forms `Application` class.
|
||||
The constructor has just one responsibility: to set the `MainPage` property of the `Application` class to an object of type `Page`.
|
||||
|
||||
```cs
|
||||
using System;
|
||||
using Xamarin.Forms;
|
||||
using Xamarin.Forms.Xaml;
|
||||
|
||||
namespace AppName
|
||||
{
|
||||
public partial class App : Application
|
||||
{
|
||||
public App()
|
||||
{
|
||||
InitializeComponent();
|
||||
|
||||
MainPage = new MainPage();
|
||||
}
|
||||
|
||||
protected override void OnStart()
|
||||
{
|
||||
}
|
||||
|
||||
protected override void OnSleep()
|
||||
{
|
||||
}
|
||||
|
||||
protected override void OnResume()
|
||||
{
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
51
DotNet/Xamarin/App.xaml.md
Normal file
51
DotNet/Xamarin/App.xaml.md
Normal file
|
@ -0,0 +1,51 @@
|
|||
# App.xaml
|
||||
|
||||
## Root Tag
|
||||
|
||||
The `<Application>` tag begins with two XML namespace declarations, both of which are URIs.
|
||||
|
||||
The default namespace belongs to **Xamarin**. This is the XML namespace (`xmlns`) for elements in the file with no prefix, such as the `ContentPage` tag.
|
||||
The URI includes the year that this namespace came into being and the word *forms* as an abbreviation for Xamarin.Forms.
|
||||
|
||||
The second namespace is associated with a prefix of `x` by convention, and it belongs to **Microsoft**. This namespace refers to elements and attributes that are intrinsic to XAML and are found in every XAML implementation.
|
||||
The word *winfx* refers to a name once used for the .NET Framework 3.0, which introduced WPF and XAML.
|
||||
|
||||
The `x:Class` attribute can appear only on the root element of a XAML file. It specifies the .NET namespace and name of a derived class. The base class of this derived class is the root element.
|
||||
|
||||
In other words, this `x:Class` specification indicates that the `App` class in the `AppName` namespace derives from `Application`.
|
||||
That’s exactly the same information as the `App` class definition in the `App.xaml.cs` file.
|
||||
|
||||
```xml
|
||||
<?xml version="1.0" encoding="utf-8" ?>
|
||||
<Application xmlns="http://xamarin.com/schemas/2014/forms"
|
||||
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
|
||||
x:Class="AppName.App">
|
||||
</Application>
|
||||
```
|
||||
|
||||
## Shared Resources
|
||||
|
||||
```xml
|
||||
<?xml version="1.0" encoding="utf-8" ?>
|
||||
<Application xmlns="http://xamarin.com/schemas/2014/forms"
|
||||
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
|
||||
x:Class="AppName.App">
|
||||
|
||||
<!-- collection of shared resources definiions -->
|
||||
<Application.Resources>
|
||||
|
||||
<!-- Application resource dictionary -->
|
||||
<ResourceDictionary>
|
||||
<!-- Key-Value Pair -->
|
||||
<Type x:Key="DictKey">value<Type>
|
||||
</ResourceDictionary>
|
||||
|
||||
<!-- define a reusable style -->
|
||||
<Style x:Key="Style Name" TargetType="Element Type">
|
||||
<!-- set poperties of the style -->
|
||||
<Setter Property="PropertyName" Value="PropertyValue">
|
||||
</Style>
|
||||
|
||||
</Application.Resources>
|
||||
</Application>
|
||||
```
|
22
DotNet/Xamarin/Page.xaml.cs.md
Normal file
22
DotNet/Xamarin/Page.xaml.cs.md
Normal file
|
@ -0,0 +1,22 @@
|
|||
# Page.xaml.cs
|
||||
|
||||
```cs
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.ComponentModel;
|
||||
using System.Linq;
|
||||
using System.Text;
|
||||
using System.Threading.Tasks;
|
||||
using Xamarin.Forms;
|
||||
|
||||
namespace AppName
|
||||
{
|
||||
public partial class PageName : ContentPage
|
||||
{
|
||||
public PageName()
|
||||
{
|
||||
InitializeComponent();
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
86
DotNet/Xamarin/Page.xaml.md
Normal file
86
DotNet/Xamarin/Page.xaml.md
Normal file
|
@ -0,0 +1,86 @@
|
|||
# Page.xaml
|
||||
|
||||
## Anatomy of an app
|
||||
|
||||
The modern user interface is constructed from visual objects of various sorts. Depending on the operating system, these visual objects might go by different names—controls, elements, views, widgets—but they are all devoted to the jobs of presentation or interaction or both.
|
||||
|
||||
In Xamarin.Forms, the objects that appear on the screen are collectively called *visual elements* (`VisualElement` class).
|
||||
|
||||
They come in three main categories:
|
||||
|
||||
- page (`Page` class)
|
||||
- layout (`Layout` class)
|
||||
- view (`View` class)
|
||||
|
||||
A Xamarin.Forms application consists of one or more pages. A page usually occupies all (or at least a large area) of the screen.
|
||||
Some applications consist of only a single page, while others allow navigating between multiple pages.
|
||||
|
||||
On each page, the visual elements are organized in a parent-child hierarchy.
|
||||
Some layouts have a single child, but many layouts have multiple children that the layout arranges within itself. These children can be other layouts or views.
|
||||
|
||||
The term *view* in Xamarin.Forms denotes familiar types of presentation and interactive objects.
|
||||
|
||||
## Pages
|
||||
|
||||
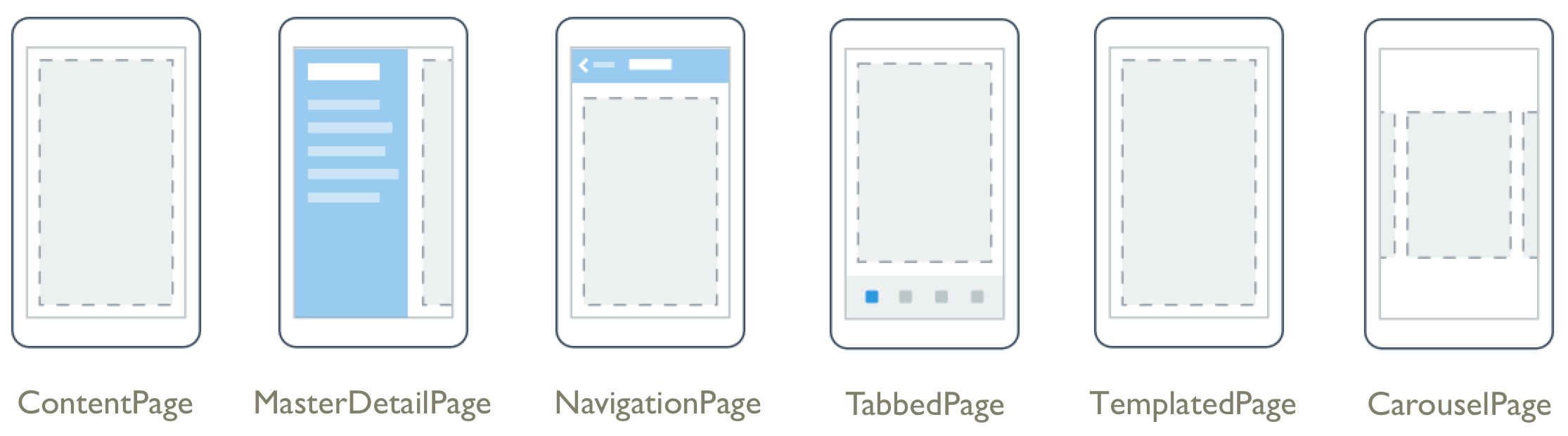
|
||||
|
||||
```xml
|
||||
<?xml version="1.0" encoding="utf-8" ?>
|
||||
|
||||
<!-- Sets the Content property to a single View -->
|
||||
<ContentPage></ContentPage>
|
||||
|
||||
<!-- Manages two panes of information (Master and Detail property) -->
|
||||
<MasterDetailPage></MasterDetailPage>
|
||||
|
||||
<!-- Manages navigation among other pages using a stack-based architecture -->
|
||||
<NavigationPage></NavigationPage>
|
||||
|
||||
<!-- Allows navigation among child pages using tabs -->
|
||||
<TabbedPage></TabbedPage>
|
||||
|
||||
<!-- Displays full-screen content with a control template -->
|
||||
<TemplatePage></TemplatePage>
|
||||
|
||||
<!-- Allows navigation among child pages through finger swiping -->
|
||||
<CarouselPage></CarouselPage>
|
||||
```
|
||||
|
||||
## Layouts
|
||||
|
||||
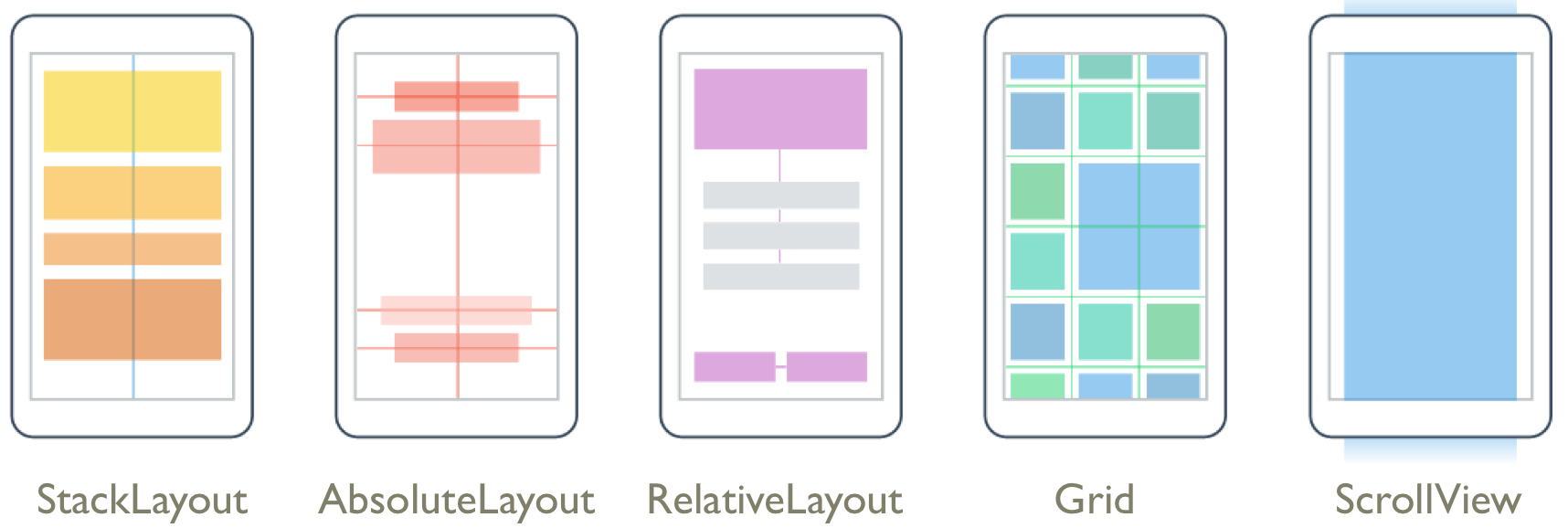
|
||||
|
||||
- StackLayout: Organizes views linearly, either horizontally or vertically.
|
||||
- AbsoluteLayout: Organizes views by setting coordinates & size in terms of absolute values or ratios.
|
||||
- RelativeLayout: Organizes views by setting constraints relative to their parent’s dimensions & position.
|
||||
- Grid: Organizes views in a grid of Rows and Columns
|
||||
- FlexLayout: Organizes views horizontally or vertically with wrapping.
|
||||
- ScrollView: Layout that's capable of scrolling its content.
|
||||
|
||||
### Grid Layout
|
||||
|
||||
```xml
|
||||
<!-- "<num>*" makes the dimesions proportional -->
|
||||
<Gird.RowDefinitions>
|
||||
<!-- insert a row in the layout -->
|
||||
<RowDefinition Height="2*"/>
|
||||
</Gird.RowDefinitions>
|
||||
|
||||
<Grid.ColumnDefinitions>
|
||||
<!-- insert column in layout -->
|
||||
<ColumnDefinition Width=".5*"/>
|
||||
</Grid.ColumnDefinitions>
|
||||
```
|
||||
|
||||
## [Views](https://docs.microsoft.com/en-us/xamarin/xamarin-forms/xaml/xaml-controls "XAML Views")
|
||||
|
||||
```xml
|
||||
<Image Source="" BackgroundColor="" [LayoutPosition]/>
|
||||
<!-- source contains reference to imagefile in Xamarin.[OS]/Resources/drawable -->
|
||||
|
||||
<!-- box to insert text -->
|
||||
<Editor Placeholder="placeholder text" [LayoutPosition]/>
|
||||
|
||||
<!-- clickable button -->
|
||||
<Button Text="button text" BackgroundColor="" Clicked="function_to_call" [LayoytPosition]/>
|
||||
```
|
35
DotNet/Xamarin/ViewModel.cs.md
Normal file
35
DotNet/Xamarin/ViewModel.cs.md
Normal file
|
@ -0,0 +1,35 @@
|
|||
# ViewModel.cs
|
||||
|
||||
```cs
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.Collections.ObjectModel;
|
||||
using System.ComponentModel;
|
||||
using System.Text;
|
||||
using Xamarin.Forms;
|
||||
|
||||
namespace AppName.ViewModels
|
||||
{
|
||||
class PageNameViewModel : INotifyPropertyChanged
|
||||
{
|
||||
public MainPageViewModel()
|
||||
{
|
||||
}
|
||||
|
||||
public event PropertyChangedEventHandler PropertyChanged; // event handler to notify changes
|
||||
|
||||
public string field; // needed, if substituted by ExprBody will cause infinite loop of access with the binding
|
||||
public string Property
|
||||
{
|
||||
get => field;
|
||||
set
|
||||
{
|
||||
field = value;
|
||||
|
||||
var args = new PropertyChangedEventArgs(nameof(Property)); // EVENT: let view know that the Property has changed
|
||||
PropertyChanged?.Invoke(this, args); // Ivoke event to notify the view
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
41
DotNet/Xamarin/Xamarin.Essentials.md
Normal file
41
DotNet/Xamarin/Xamarin.Essentials.md
Normal file
|
@ -0,0 +1,41 @@
|
|||
# Xamarin.Essentials
|
||||
|
||||
Android, iOS, and UWP offer unique operating system and platform APIs that developers have access to all in C# leveraging Xamarin.
|
||||
**Xamarin.Essentials** provides a *single cross-platform API* that works with any Xamarin.Forms, Android, iOS, or UWP application that can be accessed from shared code no matter how the user interface is created.
|
||||
|
||||
- [Accelerometer](https://docs.microsoft.com/en-us/xamarin/essentials/accelerometer): Retrieve acceleration data of the device in three dimensional space.
|
||||
- [App Information](https://docs.microsoft.com/en-us/xamarin/essentials/app-information): Find out information about the application.
|
||||
- [App Theme](https://docs.microsoft.com/en-us/xamarin/essentials/app-theme): Detect the current theme requested for the application.
|
||||
- [Barometer](https://docs.microsoft.com/en-us/xamarin/essentials/barometer): Monitor the barometer for pressure changes.
|
||||
- [Battery](https://docs.microsoft.com/en-us/xamarin/essentials/battery): Easily detect battery level, source, and state.
|
||||
- [Clipboard](https://docs.microsoft.com/en-us/xamarin/essentials/clipboard): Quickly and easily set or read text on the clipboard.
|
||||
- [Color Converters](https://docs.microsoft.com/en-us/xamarin/essentials/color-converters): Helper methods for System.Drawing.Color.
|
||||
- [Compass](https://docs.microsoft.com/en-us/xamarin/essentials/compass): Monitor compass for changes.
|
||||
- [Connectivity](https://docs.microsoft.com/en-us/xamarin/essentials/connectivity): Check connectivity state and detect changes.
|
||||
- [Detect Shake](https://docs.microsoft.com/en-us/xamarin/essentials/detect-shake): Detect a shake movement of the device.
|
||||
- [Device Display Information](https://docs.microsoft.com/en-us/xamarin/essentials/device-display): Get the device's screen metrics and orientation.
|
||||
- [Device Information](https://docs.microsoft.com/en-us/xamarin/essentials/device-information): Find out about the device with ease.
|
||||
- [Email](https://docs.microsoft.com/en-us/xamarin/essentials/email): Easily send email messages.
|
||||
- [File System Helpers](https://docs.microsoft.com/en-us/xamarin/essentials/file-system-helpers): Easily save files to app data.
|
||||
- [Flashlight](https://docs.microsoft.com/en-us/xamarin/essentials/flashlight): A simple way to turn the flashlight on/off.
|
||||
- [Geocoding](https://docs.microsoft.com/en-us/xamarin/essentials/geocoding): Geocode and reverse geocode addresses and coordinates.
|
||||
- [Geolocation](https://docs.microsoft.com/en-us/xamarin/essentials/geolocation): Retrieve the device's GPS location.
|
||||
- [Gyroscope](https://docs.microsoft.com/en-us/xamarin/essentials/gyroscope): Track rotation around the device's three primary axes.
|
||||
- [Launcher](https://docs.microsoft.com/en-us/xamarin/essentials/launcher): Enables an application to open a URI by the system.
|
||||
- [Magnetometer](https://docs.microsoft.com/en-us/xamarin/essentials/magnetometer): Detect device's orientation relative to Earth's magnetic field.
|
||||
- [MainThread](https://docs.microsoft.com/en-us/xamarin/essentials/main-thread): Run code on the application's main thread.
|
||||
- [Maps](https://docs.microsoft.com/en-us/xamarin/essentials/maps): Open the maps application to a specific location.
|
||||
- [Open Browser](https://docs.microsoft.com/en-us/xamarin/essentials/open-browser): Quickly and easily open a browser to a specific website.
|
||||
- [Orientation Sensor](https://docs.microsoft.com/en-us/xamarin/essentials/orientation-sensor): Retrieve the orientation of the device in three dimensional space.
|
||||
- [Permissions](https://docs.microsoft.com/en-us/xamarin/essentials/permissions): Check and request permissions from users.
|
||||
- [Phone Dialer](https://docs.microsoft.com/en-us/xamarin/essentials/phone-dialer): Open the phone dialer.
|
||||
- [Platform Extensions](https://docs.microsoft.com/en-us/xamarin/essentials/platform-extensions): Helper methods for converting Rect, Size, and Point.
|
||||
- [Preferences](https://docs.microsoft.com/en-us/xamarin/essentials/preferences): Quickly and easily add persistent preferences.
|
||||
- [Secure Storage](https://docs.microsoft.com/en-us/xamarin/essentials/secure-storage): Securely store data.
|
||||
- [Share](https://docs.microsoft.com/en-us/xamarin/essentials/share): Send text and website uris to other apps.
|
||||
- [SMS](https://docs.microsoft.com/en-us/xamarin/essentials/sms): Create an SMS message for sending.
|
||||
- [Text-to-Speech](https://docs.microsoft.com/en-us/xamarin/essentials/text-to-speech): Vocalize text on the device.
|
||||
- [Unit Converters](https://docs.microsoft.com/en-us/xamarin/essentials/unit-converters): Helper methods to convert units.
|
||||
- [Version Tracking](https://docs.microsoft.com/en-us/xamarin/essentials/version-tracking): Track the applications version and build numbers.
|
||||
- [Vibrate](https://docs.microsoft.com/en-us/xamarin/essentials/vibrate): Make the device vibrate.
|
||||
- [Web Authenticator](https://docs.microsoft.com/en-us/xamarin/essentials/web-authenticator): Start web authentication flows and listen for a callback.
|
Loading…
Add table
Add a link
Reference in a new issue