mirror of
https://github.com/m-lamonaca/dev-notes.git
synced 2025-07-05 19:52:12 +00:00
Upload of pre-existing files
This commit is contained in:
commit
4c21152830
150 changed files with 730703 additions and 0 deletions
414
.NET/ASP.NET/MVC.md
Normal file
414
.NET/ASP.NET/MVC.md
Normal file
|
@ -0,0 +1,414 @@
|
|||
# ASP.NET (Core) MVC Web App
|
||||
|
||||
## Project Structure
|
||||
|
||||
```txt
|
||||
Project
|
||||
|-Properties
|
||||
| |- launchSettings.json
|
||||
|
|
||||
|-wwwroot --> location of static files
|
||||
| |-css
|
||||
| | |- site.css
|
||||
| |
|
||||
| |-js
|
||||
| | |- site.js
|
||||
| |
|
||||
| |-lib
|
||||
| | |- bootstrap
|
||||
| | |- jquery
|
||||
| | |- ...
|
||||
| |
|
||||
| |- favicon.ico
|
||||
|
|
||||
|-Model
|
||||
| |-ErrorViewModel.cs
|
||||
| |- Index.cs
|
||||
| |-...
|
||||
|
|
||||
|-Views
|
||||
| |-Home
|
||||
| | |- Index.cshtml
|
||||
| |
|
||||
| |-Shared
|
||||
| | |- _Layout.cshtml --> reusable default page layout
|
||||
| | |- _ValidationScriptsPartial --> jquery validation script imports
|
||||
| |
|
||||
| |- _ViewImports.cshtml --> shared imports and tag helpers for all views
|
||||
| |- _ViewStart.cshtml --> shared values for all views
|
||||
| |- ...
|
||||
|
|
||||
|-Controllers
|
||||
| |-HomeController.cs
|
||||
|
|
||||
|- appsettings.json
|
||||
|- Program.cs --> App entrypoiny
|
||||
|- Startup.cs --> App config
|
||||
```
|
||||
|
||||
**Note**: `_` prefix indicates page to be imported.
|
||||
|
||||
## Important Files
|
||||
|
||||
### `Program.cs`
|
||||
|
||||
```cs
|
||||
using Microsoft.AspNetCore.Hosting;
|
||||
using Microsoft.Extensions.Hosting;
|
||||
|
||||
namespace App
|
||||
{
|
||||
public class Program
|
||||
{
|
||||
public static void Main(string[] args)
|
||||
{
|
||||
CreateHostBuilder(args).Build().Run(); // start and config ASP.NET App
|
||||
}
|
||||
|
||||
public static IHostBuilder CreateHostBuilder(string[] args) =>
|
||||
Host.CreateDefaultBuilder(args)
|
||||
.ConfigureWebHostDefaults(webBuilder =>
|
||||
{
|
||||
webBuilder.UseStartup<Startup>(); // config handled in Startup.cs
|
||||
});
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### `Startup.cs`
|
||||
|
||||
```cs
|
||||
using Microsoft.AspNetCore.Builder;
|
||||
using Microsoft.AspNetCore.Hosting;
|
||||
using Microsoft.AspNetCore.HttpsPolicy;
|
||||
using Microsoft.Extensions.Configuration;
|
||||
using Microsoft.Extensions.DependencyInjection;
|
||||
using Microsoft.Extensions.Hosting;
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.Linq;
|
||||
using System.Threading.Tasks;
|
||||
|
||||
namespace App
|
||||
{
|
||||
public class Startup
|
||||
{
|
||||
public Startup(IConfiguration configuration)
|
||||
{
|
||||
Configuration = configuration;
|
||||
}
|
||||
|
||||
public IConfiguration Configuration { get; }
|
||||
|
||||
// This method gets called by the runtime. Use this method to add services to the container.
|
||||
public void ConfigureServices(IServiceCollection services)
|
||||
{
|
||||
// set db context for the app using the connection string
|
||||
services.AddDbContext<AppDbContext>(options => options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
|
||||
|
||||
// Captures synchronous and asynchronous Exception instances from the pipeline and generates HTML error responses.
|
||||
services.AddDatabaseDeveloperPageExceptionFilter();
|
||||
|
||||
services.AddControllersWithViews(); // MVC Services
|
||||
}
|
||||
|
||||
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
|
||||
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
|
||||
{
|
||||
if (env.IsDevelopment())
|
||||
{
|
||||
app.UseDeveloperExceptionPage();
|
||||
}
|
||||
else
|
||||
{
|
||||
app.UseExceptionHandler("/Home/Error");
|
||||
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
|
||||
app.UseHsts();
|
||||
}
|
||||
app.UseHttpsRedirection();
|
||||
app.UseStaticFiles();
|
||||
|
||||
app.UseRouting();
|
||||
|
||||
app.UseAuthorization();
|
||||
|
||||
app.UseEndpoints(endpoints =>
|
||||
{
|
||||
endpoints.MapControllerRoute(
|
||||
name: "default",
|
||||
pattern: "{controller=Home}/{action=Index}/{id?}");
|
||||
// URL => www.site.com/Controller/Action/id
|
||||
// id optional, action is a method of the controller returnig an ActionResult
|
||||
});
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### `appsettings.json`
|
||||
|
||||
Connection Strings & Secrets.
|
||||
|
||||
```json
|
||||
{
|
||||
// WARNING: not for production (use appsecrets.json)
|
||||
"ConnectionStrings": {
|
||||
"DefaultConnection": "Server=<server>;Database=<database>;Trusted_Connection=true;MultipleActiveResultSets=true;"
|
||||
}
|
||||
"Logging": {
|
||||
"LogLevel": {
|
||||
"Default": "Information",
|
||||
"Microsoft": "Warning",
|
||||
"Microsoft.Hosting.Lifetime": "Information"
|
||||
}
|
||||
},
|
||||
"AllowedHosts": "*"
|
||||
}
|
||||
```
|
||||
|
||||
### `launchsettings.json`
|
||||
|
||||
Launch Settings & Deployement Settings.
|
||||
|
||||
```json
|
||||
{
|
||||
"iisSettings": {
|
||||
"windowsAuthentication": false,
|
||||
"anonymousAuthentication": true,
|
||||
"iisExpress": {
|
||||
"applicationUrl": "http://localhost:55045",
|
||||
"sslPort": 44301
|
||||
}
|
||||
},
|
||||
"profiles": {
|
||||
"IIS Express": {
|
||||
"commandName": "IISExpress",
|
||||
"launchBrowser": true,
|
||||
"environmentVariables": {
|
||||
"ASPNETCORE_ENVIRONMENT": "Development",
|
||||
"ASPNETCORE_HOSTINGSTARTUPASSEMBLIES": "Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation"
|
||||
}
|
||||
},
|
||||
"App": {
|
||||
"commandName": "Project",
|
||||
"dotnetRunMessages": "true",
|
||||
"launchBrowser": true,
|
||||
"applicationUrl": "https://localhost:5001;http://localhost:5000",
|
||||
"environmentVariables": {
|
||||
"ASPNETCORE_ENVIRONMENT": "Development",
|
||||
"ASPNETCORE_HOSTINGSTARTUPASSEMBLIES": "Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation"
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Controllers
|
||||
|
||||
```cs
|
||||
using Microsoft.AspNetCore.Mvc;
|
||||
using App.Models;
|
||||
using System.Collections.Generic;
|
||||
|
||||
namespace App.Controllers
|
||||
{
|
||||
public class CategoryController : Controller
|
||||
{
|
||||
private readonly AppDbContext _db;
|
||||
|
||||
// get db context through dependency injection
|
||||
public CategoryController(AppDbContext db)
|
||||
{
|
||||
_db = db;
|
||||
}
|
||||
|
||||
// GET /Controller/Index
|
||||
public IActionResult Index()
|
||||
{
|
||||
IEnumerable<Entity> enities = _db.Entities;
|
||||
return View(Entities); // pass data to the @model
|
||||
}
|
||||
|
||||
// GET /Controller/Create
|
||||
public IActionResult Create()
|
||||
{
|
||||
return View();
|
||||
}
|
||||
|
||||
// POST /Controller/Create
|
||||
[HttpPost]
|
||||
[ValidateAntiForgeryToken]
|
||||
public IActionResult Create(Entity entity) // recieve data from the @model
|
||||
{
|
||||
_db.Entities.Add(entity);
|
||||
_db.SaveChanges();
|
||||
return RedirectToAction("Index"); // redirection
|
||||
}
|
||||
|
||||
// GET - /Controller/Edit
|
||||
public IActionResult Edit(int? id)
|
||||
{
|
||||
if(id == null || id == 0)
|
||||
{
|
||||
return NotFound();
|
||||
}
|
||||
|
||||
Entity entity = _db.Entities.Find(id);
|
||||
if (entity == null)
|
||||
{
|
||||
return NotFound();
|
||||
}
|
||||
|
||||
return View(entity); // return pupulated form for updating
|
||||
}
|
||||
|
||||
// POST /Controller/Edit
|
||||
[HttpPost]
|
||||
[ValidateAntiForgeryToken]
|
||||
public IActionResult Edit(Entity entity)
|
||||
{
|
||||
if (ModelState.IsValid) // all rules in model have been met
|
||||
{
|
||||
_db.Entities.Update(entity);
|
||||
_db.SaveChanges();
|
||||
return RedirectToAction("Index");
|
||||
}
|
||||
|
||||
return View(entity);
|
||||
}
|
||||
|
||||
// GET /controller/Delete
|
||||
public IActionResult Delete(int? id)
|
||||
{
|
||||
if (id == null || id == 0)
|
||||
{
|
||||
return NotFound();
|
||||
}
|
||||
|
||||
Entity entity = _db.Entities.Find(id);
|
||||
if (entity == null)
|
||||
{
|
||||
return NotFound();
|
||||
}
|
||||
|
||||
return View(entity); // return pupulated form for confirmation
|
||||
}
|
||||
|
||||
// POST /Controller/Delete
|
||||
[HttpPost]
|
||||
[ValidateAntiForgeryToken]
|
||||
public IActionResult Delete(Entity entity)
|
||||
{
|
||||
if (ModelState.IsValid) // all rules in model have been met
|
||||
{
|
||||
_db.Entities.Remove(entity);
|
||||
_db.SaveChanges();
|
||||
return RedirectToAction("Index");
|
||||
}
|
||||
|
||||
return View(entity);
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Data Validation
|
||||
|
||||
### Model Annotations
|
||||
|
||||
In `Entity.cs`:
|
||||
|
||||
```cs
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.ComponentModel;
|
||||
using System.ComponentModel.DataAnnotations;
|
||||
using System.Linq;
|
||||
|
||||
namespace App.Models
|
||||
{
|
||||
public class Entity
|
||||
{
|
||||
[DisplayName("Integer Number")]
|
||||
[Required]
|
||||
[Range(1, int.MaxValue, ErrorMessage = "Error Message")]
|
||||
public int IntProp { get; set; }
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Tag Helpers & Client Side Validation
|
||||
|
||||
In `View.cshtml`;
|
||||
|
||||
```cs
|
||||
<form method="post" asp-action="Create">
|
||||
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
|
||||
|
||||
<div class="form-group row">
|
||||
<div class="col-4">
|
||||
<label asp-for="IntProp"></label>
|
||||
</div>
|
||||
|
||||
<div class="col-8">
|
||||
<input asp-for="IntProp" class="form-control"/>
|
||||
<span asp-validation-for="IntProp" class="text-danger"></span> // error message displyed here
|
||||
</div>
|
||||
</div>
|
||||
</form>
|
||||
|
||||
// client side validation
|
||||
@section Scripts{
|
||||
@{ <partial name="_ValidationScriptsPartial" /> }
|
||||
}
|
||||
```
|
||||
|
||||
### Server Side Validation
|
||||
|
||||
```cs
|
||||
using Microsoft.AspNetCore.Mvc;
|
||||
using App.Models;
|
||||
using System.Collections.Generic;
|
||||
|
||||
namespace App.Controllers
|
||||
{
|
||||
public class CategoryController : Controller
|
||||
{
|
||||
private readonly AppDbContext _db;
|
||||
|
||||
// get db context through dependency injection
|
||||
public CategoryController(AppDbContext db)
|
||||
{
|
||||
_db = db;
|
||||
}
|
||||
|
||||
// GET /Controller/Index
|
||||
public IActionResult Index()
|
||||
{
|
||||
IEnumerable<Entity> enities = _db.Entities;
|
||||
return View(Entities); // pass data to the @model
|
||||
}
|
||||
|
||||
// GET /Controller/Create
|
||||
public IActionResult Create()
|
||||
{
|
||||
return View();
|
||||
}
|
||||
|
||||
// POST /Controller/Create
|
||||
[HttpPost]
|
||||
[ValidateAntiForgeryToken]
|
||||
public IActionResult Create(Entity entity) // recieve data from the @model
|
||||
{
|
||||
if (ModelState.IsValid) // all rules in model have been met
|
||||
{
|
||||
_db.Entities.Add(entity);
|
||||
_db.SaveChanges();
|
||||
return RedirectToAction("Index");
|
||||
}
|
||||
|
||||
return View(entity); // return model and display error messages
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
336
.NET/ASP.NET/REST API.md
Normal file
336
.NET/ASP.NET/REST API.md
Normal file
|
@ -0,0 +1,336 @@
|
|||
# ASP .NET REST API
|
||||
|
||||
## Startup class
|
||||
|
||||
- Called by `Program.cs`
|
||||
|
||||
```cs
|
||||
using Microsoft.AspNetCore.Builder;
|
||||
using Microsoft.AspNetCore.Hosting;
|
||||
using Microsoft.Extensions.Configuration;
|
||||
using Microsoft.Extensions.DependencyInjection;
|
||||
using Microsoft.Extensions.Hosting;
|
||||
|
||||
namespace <Namespace>
|
||||
{
|
||||
public class Startup
|
||||
{
|
||||
public Startup(IConfiguration configuration)
|
||||
{
|
||||
Configuration = configuration;
|
||||
}
|
||||
|
||||
public IConfiguration Configuration { get; }
|
||||
|
||||
// This method gets called by the runtime. Use this method to add services to the container.
|
||||
public void ConfigureServices(IServiceCollection services)
|
||||
{
|
||||
services.AddControllers(); // controllers w/o views
|
||||
//or
|
||||
sevices.AddControllersWithViews(); // MVC Controllers
|
||||
}
|
||||
|
||||
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
|
||||
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
|
||||
{
|
||||
if (env.IsDevelopment())
|
||||
{
|
||||
app.UseDeveloperExceptionPage();
|
||||
}
|
||||
|
||||
app.UseHttpsRedirection();
|
||||
|
||||
app.UseRouting();
|
||||
|
||||
app.UseAuthorization();
|
||||
|
||||
app.UseEndpoints(endpoints =>
|
||||
{
|
||||
endpoints.MapControllers();
|
||||
});
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## DB Context (EF to access DB)
|
||||
|
||||
NuGet Packages to install:
|
||||
|
||||
- `Microsoft.EntityFrameworkCore`
|
||||
- `Microsoft.EntityFrameworkCore.Tools`
|
||||
- `Microsoft.EntityFrameworkCore.Design` *or* `Microsoft.EntityFrameworkCore.<db_provider>.Design`
|
||||
- `Microsoft.EntityFrameworkCore.<db_provider>`
|
||||
|
||||
In `AppDbContext.cs`:
|
||||
|
||||
```cs
|
||||
using <Namespace>.Model;
|
||||
using Microsoft.EntityFrameworkCore;
|
||||
|
||||
namespace <Namespace>.Repo
|
||||
{
|
||||
public class AppDbContext : DbContext
|
||||
{
|
||||
public AppDbContext(DbContextOptions<ProjectContext> options) : base(options)
|
||||
{
|
||||
|
||||
}
|
||||
//DBSet<TEntity> represents the collection of all entities in the context, or that can be queried from the database, of a given type
|
||||
public DbSet<Entity> entities { get; set; }
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
In `appsettings.json`:
|
||||
|
||||
```json
|
||||
{
|
||||
"Logging": {
|
||||
"LogLevel": {
|
||||
"Default": "Information",
|
||||
"Microsoft": "Warning",
|
||||
"Microsoft.Hosting.Lifetime": "Information"
|
||||
}
|
||||
},
|
||||
"AllowedHosts": "*",
|
||||
"ConnectionStrings": {
|
||||
"CommanderConnection" : "Server=<server>;Database=<database>;UID=<user>;Pwd=<password>"
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
In `Startup.cs`:
|
||||
|
||||
```cs
|
||||
// This method gets called by the runtime. Use this method to add services to the container.
|
||||
public void ConfigureServices(IServiceCollection services)
|
||||
{
|
||||
// SqlServer is the db used in this example
|
||||
services.AddDbContext<CommanderContext>(option => option.UseSqlServer(Configuration.GetConnectionString("CommanderConnection")));
|
||||
services.AddControllers();
|
||||
}
|
||||
```
|
||||
|
||||
### Migrations
|
||||
|
||||
- Mirroring of model in the DB.
|
||||
- Will create & update DB Schema if necessary
|
||||
|
||||
In Packge Manager Shell:
|
||||
|
||||
```ps1
|
||||
add-migrations <migration_name>
|
||||
update-database # use the migrations to modify the db
|
||||
```
|
||||
|
||||
## Repository
|
||||
|
||||
In `IEntityRepo`:
|
||||
|
||||
```cs
|
||||
using <Namespace>.Model;
|
||||
using System.Collections.Generic;
|
||||
|
||||
namespace <Namespace>.Repository
|
||||
{
|
||||
public interface IEntityRepo
|
||||
{
|
||||
IEnumerable<Entity> SelectAll();
|
||||
Entity SelectOneById(int id);
|
||||
|
||||
...
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
In `EntityRepo`:
|
||||
|
||||
```cs
|
||||
using <Namespace>.Model;
|
||||
using System.Collections.Generic;
|
||||
|
||||
namespace <Namespace>.Repo
|
||||
{
|
||||
public class EntityRepo : IEntityRepo
|
||||
{
|
||||
private readonly AppDbContext _context;
|
||||
|
||||
public EntityRepo(AppDbContext context)
|
||||
{
|
||||
_context = context;
|
||||
}
|
||||
|
||||
public IEnumerable<Entity> SelectAll()
|
||||
{
|
||||
return _context.Entities.ToList(); // linq query (ToList()) becomes sql query
|
||||
}
|
||||
|
||||
public Entity SelectOneById(int id)
|
||||
{
|
||||
return _context.Entities.FirstOrDefault(p => p.Id == id);
|
||||
}
|
||||
|
||||
...
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Data Transfer Objects (DTOs)
|
||||
|
||||
A **DTO** is an object that defines how the data will be sent and recieved over the network (usually as JSON).
|
||||
Without a DTO the JSON response (or reqest) could contain irrilevant, wrong or unformatted data.
|
||||
Moreover, by decoupling the JSON response from the actual data model, it's possible to change the latter without breaking the API.
|
||||
DTOs must be mapped to the internal methods.
|
||||
|
||||
Required NuGet Packages:
|
||||
|
||||
- AutoMapper.Extensions.Microsoft.DependencyInjection
|
||||
|
||||
In `StartUp.cs`:
|
||||
|
||||
```cs
|
||||
using AutoMapper;
|
||||
|
||||
// ...
|
||||
|
||||
public void ConfigureServices(IServiceCollection services)
|
||||
{
|
||||
// other services
|
||||
|
||||
services.AddAutoMapper(AppDomain.CurrentDomain.GetAssemblies()); // set automapper service
|
||||
}
|
||||
```
|
||||
|
||||
In `Entity<CrudOperation>DTO.cs`:
|
||||
|
||||
```cs
|
||||
namespace <Namespace>.DTOs
|
||||
{
|
||||
// define the data to be serialized in JSON (can differ from model)
|
||||
public class EntityCrudOpDTO // e.g: EntityReadDTO, ...
|
||||
{
|
||||
// only properties to be serialized
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
In `EntitiesProfile.cs`:
|
||||
|
||||
```cs
|
||||
using AutoMapper;
|
||||
using <Namespace>.DTOs;
|
||||
using <Namespace>.Model;
|
||||
|
||||
namespace <Namespace>.Profiles
|
||||
{
|
||||
public class EntitiesProfile : Profile
|
||||
{
|
||||
public EntitiesProfile()
|
||||
{
|
||||
CreateMap<Entity, EntityCrudOpDTO>(); // map entity to it's DTO
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Controller (No View)
|
||||
|
||||
Uses [Dependency Injection](https://en.wikipedia.org/wiki/Dependency_injection) to recieve a suitable implementation of `IEntityRepo`,
|
||||
|
||||
### Service Lifetimes
|
||||
|
||||
- `AddSignleton`: same for every request
|
||||
- `AddScoped`: created once per client
|
||||
- `Transient`: new instance created every time
|
||||
|
||||
In `Startup.cs`:
|
||||
|
||||
```cs
|
||||
// This method gets called by the runtime. Use this method to add services to the container.
|
||||
public void ConfigureServices(IServiceCollection services)
|
||||
{
|
||||
services.AddControllers();
|
||||
services.AddScoped<IEntityRepo, EntityRepo>(); // map the interface to its implementation, needed for dependency injection
|
||||
}
|
||||
```
|
||||
|
||||
### Request Mappings
|
||||
|
||||
```cs
|
||||
using <App>.Model;
|
||||
using <App>.Repo;
|
||||
using Microsoft.AspNetCore.Mvc;
|
||||
using System.Collections.Generic;
|
||||
|
||||
namespace <App>.Controllers
|
||||
{
|
||||
[Route("api/endpoint")]
|
||||
[ApiController]
|
||||
public class EntitiesController : ControllerBase // MVC controller w/o view
|
||||
{
|
||||
private readonly ICommandRepo _repo;
|
||||
private readonly IMapper _mapper; // AutoMapper class
|
||||
|
||||
public EntitiesController(IEntityRepo repository, IMapper mapper) // injection og the dependency
|
||||
{
|
||||
_repo = repository;
|
||||
_mapper = mapper
|
||||
}
|
||||
|
||||
[HttpGet] // GET api/endpoint
|
||||
public ActionResult<IEnumerable<EntityCrudOpDTO>> SelectAllEntities()
|
||||
{
|
||||
var results = _repo.SelectAll();
|
||||
|
||||
return Ok(_mapper.Map<EntityCrudOpDTO>(results)); // return an action result OK (200) with the results
|
||||
}
|
||||
|
||||
// default binding source: [FromRoute]
|
||||
[HttpGet("{id}")] // GET api/endpoint/{id}
|
||||
public ActionResult<EntityCrudOpDTO> SelectOneEntityById(int id)
|
||||
{
|
||||
var result = _repo.SelectOneById(id);
|
||||
|
||||
if(result != null)
|
||||
{
|
||||
return Ok(_mapper.Map<EntityCrudOp>(result)); // transfrom entity to it's DTO
|
||||
}
|
||||
|
||||
return NotFound(); // ActionResult NOT FOUND (404)
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Controller (With View)
|
||||
|
||||
```cs
|
||||
using <App>.Model;
|
||||
using Microsoft.AspNetCore.Mvc;
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.Linq;
|
||||
using System.Threading.Tasks;
|
||||
|
||||
namespace <App>.Controllers
|
||||
{
|
||||
[Route("api/endpoint")]
|
||||
[ApiController]
|
||||
public class EntitiesController : Controller
|
||||
{
|
||||
private readonly AppDbContext _db;
|
||||
|
||||
public EntitiesController(AppDbContext db)
|
||||
{
|
||||
_db = db;
|
||||
}
|
||||
|
||||
[HttpGet]
|
||||
public IActionResult SelectAll()
|
||||
{
|
||||
return Json(new { data = _db.Entities.ToList() }); // json view
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
384
.NET/ASP.NET/Razor Pages.md
Normal file
384
.NET/ASP.NET/Razor Pages.md
Normal file
|
@ -0,0 +1,384 @@
|
|||
# Razor Pages
|
||||
|
||||
## Project Structure
|
||||
|
||||
```txt
|
||||
Project
|
||||
|-Properties
|
||||
| |- launchSettings.json
|
||||
|
|
||||
|-wwwroot --> static files
|
||||
| |-css
|
||||
| | |- site.css
|
||||
| |
|
||||
| |-js
|
||||
| | |- site.js
|
||||
| |
|
||||
| |-lib
|
||||
| | |- jquery
|
||||
| | |- bootstrap
|
||||
| | |- ...
|
||||
| |
|
||||
| |- favicon.ico
|
||||
|
|
||||
|-Pages
|
||||
| |-Shared
|
||||
| | |- _Layout.cshtml --> reusable default page layout
|
||||
| | |- _ValidationScriptsPartial --> jquery validation script imports
|
||||
| |
|
||||
| |- _ViewImports.cshtml --> shared imports and tag helpers for all views
|
||||
| |- _ViewStart.cshtml --> shared values for all views
|
||||
| |- Index.cshtml
|
||||
| |- Index.cshtml.cs
|
||||
| |- ...
|
||||
|
|
||||
|- appsettings.json --> application settings
|
||||
|- Program.cs --> App entrypoint
|
||||
|- Startup.cs
|
||||
```
|
||||
|
||||
**Note**: `_` prefix indicates page to be imported
|
||||
|
||||
Razor Pages components:
|
||||
|
||||
- Razor Page (UI/View - `.cshtml`)
|
||||
- Page Model (Handlers - `.cshtml.cs`)
|
||||
|
||||
in `Index.cshtml`:
|
||||
|
||||
```cs
|
||||
@page // mark as Razor Page
|
||||
@model IndexModel // Link Page Model
|
||||
|
||||
@{
|
||||
ViewData["Title"] = "Page Title" // same as <title>Page Title</title>
|
||||
}
|
||||
|
||||
// body contents
|
||||
```
|
||||
|
||||
in `Page.cshtml.cs`:
|
||||
|
||||
```cs
|
||||
using Microsoft.AspNetCore.Mvc.RazorPages;
|
||||
using Microsoft.Extensions.Logging;
|
||||
|
||||
namespace App.Pages
|
||||
{
|
||||
public class IndexModel : PageModel
|
||||
{
|
||||
// HTTP Method
|
||||
public void OnGet() { }
|
||||
|
||||
// HTTP Method
|
||||
public void OnPost() { }
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Important Files
|
||||
|
||||
### `Program.cs`
|
||||
|
||||
```cs
|
||||
using Microsoft.AspNetCore.Hosting;
|
||||
using Microsoft.Extensions.Hosting;
|
||||
|
||||
namespace App
|
||||
{
|
||||
public class Program
|
||||
{
|
||||
public static void Main(string[] args)
|
||||
{
|
||||
CreateHostBuilder(args).Build().Run(); // start and config ASP.NET App
|
||||
}
|
||||
|
||||
public static IHostBuilder CreateHostBuilder(string[] args) =>
|
||||
Host.CreateDefaultBuilder(args)
|
||||
.ConfigureWebHostDefaults(webBuilder =>
|
||||
{
|
||||
webBuilder.UseStartup<Startup>(); // config handled in Startup.cs
|
||||
});
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### `Startup.cs`
|
||||
|
||||
```cs
|
||||
using Microsoft.AspNetCore.Builder;
|
||||
using Microsoft.AspNetCore.Hosting;
|
||||
using Microsoft.AspNetCore.HttpsPolicy;
|
||||
using Microsoft.Extensions.Configuration;
|
||||
using Microsoft.Extensions.DependencyInjection;
|
||||
using Microsoft.Extensions.Hosting;
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.Linq;
|
||||
using System.Threading.Tasks;
|
||||
|
||||
namespace App
|
||||
{
|
||||
public class Startup
|
||||
{
|
||||
public Startup(IConfiguration configuration)
|
||||
{
|
||||
Configuration = configuration;
|
||||
}
|
||||
|
||||
public IConfiguration Configuration { get; }
|
||||
|
||||
// This method gets called by the runtime. Use this method to add services to the container.
|
||||
public void ConfigureServices(IServiceCollection services)
|
||||
{
|
||||
// use Razor Pages
|
||||
// runtime compilation needs Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation pkg
|
||||
services.AddRazorPages().AddRazorRuntimeCompilation();
|
||||
|
||||
// set db context for the app using the connection string
|
||||
services.AddDbContext<ApplicationDbContext>(options => options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
|
||||
|
||||
// Captures synchronous and asynchronous Exception instances from the pipeline and generates HTML error responses.
|
||||
services.AddDatabaseDeveloperPageExceptionFilter();
|
||||
|
||||
}
|
||||
|
||||
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
|
||||
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
|
||||
{
|
||||
if (env.IsDevelopment())
|
||||
{
|
||||
app.UseDeveloperExceptionPage();
|
||||
}
|
||||
else
|
||||
{
|
||||
app.UseExceptionHandler("/Home/Error");
|
||||
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
|
||||
app.UseHsts();
|
||||
}
|
||||
app.UseHttpsRedirection();
|
||||
app.UseStaticFiles();
|
||||
|
||||
app.UseRouting();
|
||||
|
||||
app.UseAuthorization();
|
||||
|
||||
app.UseEndpoints(endpoints =>
|
||||
{
|
||||
endpoints.MapRazorPages();
|
||||
});
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### `appsettings.json`
|
||||
|
||||
Connection Strings & Secrets.
|
||||
|
||||
```json
|
||||
{
|
||||
"Logging": {
|
||||
"LogLevel": {
|
||||
"Default": "Information",
|
||||
"Microsoft": "Warning",
|
||||
"Microsoft.Hosting.Lifetime": "Information"
|
||||
}
|
||||
},
|
||||
"AllowedHosts": "*"
|
||||
}
|
||||
```
|
||||
|
||||
### `launchsettings.json`
|
||||
|
||||
Launch Settings & Deployement Settings.
|
||||
|
||||
```json
|
||||
{
|
||||
"iisSettings": {
|
||||
"windowsAuthentication": false,
|
||||
"anonymousAuthentication": true,
|
||||
"iisExpress": {
|
||||
"applicationUrl": "http://localhost:51144",
|
||||
"sslPort": 44335
|
||||
}
|
||||
},
|
||||
"profiles": {
|
||||
"IIS Express": {
|
||||
"commandName": "IISExpress",
|
||||
"launchBrowser": true,
|
||||
"environmentVariables": {
|
||||
"ASPNETCORE_ENVIRONMENT": "Development"
|
||||
}
|
||||
},
|
||||
"BookListRazor": {
|
||||
"commandName": "Project",
|
||||
"dotnetRunMessages": "true",
|
||||
"launchBrowser": true,
|
||||
"applicationUrl": "https://localhost:5001;http://localhost:5000",
|
||||
"environmentVariables": {
|
||||
"ASPNETCORE_ENVIRONMENT": "Development"
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Razor Page
|
||||
|
||||
```cs
|
||||
using Microsoft.AspNetCore.Mvc.RazorPages;
|
||||
using Microsoft.Extensions.Logging;
|
||||
|
||||
namespace App.Pages
|
||||
{
|
||||
public class IndexModel : PageModel
|
||||
{
|
||||
private readonly ApplicationDbContext _db; // EF DB Context
|
||||
|
||||
// Get DBContex through DI
|
||||
public IndexModel(ApplicationDbContext db)
|
||||
{
|
||||
_db = db;
|
||||
}
|
||||
|
||||
[BindProperty] // assumed to be recieved on POST
|
||||
public IEnumerable<Entity> Entities { get; set; }
|
||||
|
||||
// HTTP Method Handler
|
||||
public aysnc Task OnGet()
|
||||
{
|
||||
// get data from DB (example operation)
|
||||
Entities = await _db.Entities.ToListAsync();
|
||||
}
|
||||
|
||||
// HTTP Method Handler
|
||||
public async Task<IActionResult> OnPost()
|
||||
{
|
||||
if (ModelState.IsValid)
|
||||
{
|
||||
// save to DB (example operation)
|
||||
await _db.Entities.AddAsync(Entity);
|
||||
await _db.SaveChangesAsync();
|
||||
|
||||
return RedirectToPage("Index");
|
||||
}
|
||||
else
|
||||
{
|
||||
return Page();
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Routing
|
||||
|
||||
Rules:
|
||||
|
||||
- URL maps to a physical file on disk
|
||||
- Razor paged needs a root folder (Default "Pages")
|
||||
- file extension not included in URL
|
||||
- `Index.cshtml` is enrtypoint and default document (missing file in URL redirects to index)
|
||||
|
||||
| URL | Maps TO |
|
||||
|------------------------|----------------------------------------------------|
|
||||
| www.domain.com | /Pages/Index.cshtml |
|
||||
| www.doamin.com/Index | /Pages/Index.html |
|
||||
| www.domain.com/Account | /Pages/Account.cshtml, /Pages/Account/Index.cshtml |
|
||||
|
||||
## Data Validation
|
||||
|
||||
### Model Annotations
|
||||
|
||||
In `Entity.cs`:
|
||||
|
||||
```cs
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.ComponentModel;
|
||||
using System.ComponentModel.DataAnnotations;
|
||||
using System.Linq;
|
||||
|
||||
namespace App.Models
|
||||
{
|
||||
public class Entity
|
||||
{
|
||||
[DisplayName("Integer Number")]
|
||||
[Required]
|
||||
[Range(1, int.MaxValue, ErrorMessage = "Error Message")]
|
||||
public int IntProp { get; set; }
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Tag Helpers & Client Side Validation
|
||||
|
||||
In `View.cshtml`;
|
||||
|
||||
```cs
|
||||
<form method="post" asp-action="Create">
|
||||
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
|
||||
|
||||
<div class="form-group row">
|
||||
<div class="col-4">
|
||||
<label asp-for="IntProp"></label>
|
||||
</div>
|
||||
|
||||
<div class="col-8">
|
||||
<input asp-for="IntProp" class="form-control"/>
|
||||
<span asp-validation-for="IntProp" class="text-danger"></span> // error message displyed here
|
||||
</div>
|
||||
</div>
|
||||
</form>
|
||||
|
||||
// client side validation
|
||||
@section Scripts{
|
||||
@{ <partial name="_ValidationScriptsPartial" /> }
|
||||
}
|
||||
```
|
||||
|
||||
### Server Side Validation
|
||||
|
||||
```cs
|
||||
using Microsoft.AspNetCore.Mvc;
|
||||
using App.Models;
|
||||
using System.Collections.Generic;
|
||||
|
||||
namespace App.Controllers
|
||||
{
|
||||
public class IndexModel : PageModel
|
||||
{
|
||||
private readonly ApplicationDbContext _db;
|
||||
|
||||
// get db context through dependency injection
|
||||
public IndexModel(AppDbContext db)
|
||||
{
|
||||
_db = db;
|
||||
}
|
||||
|
||||
[BindProperty]
|
||||
public Entity Entity { get; set; }
|
||||
|
||||
public async Task OnGet(int id)
|
||||
{
|
||||
Entity = await _db.Entities.FindAsync(id);
|
||||
}
|
||||
|
||||
public async Task<IActionResult> OnPost()
|
||||
{
|
||||
if (ModelState.IsValid)
|
||||
{
|
||||
|
||||
await _db.SaveChangesAsync();
|
||||
|
||||
return RedirectToPage("Index");
|
||||
}
|
||||
else
|
||||
{
|
||||
return Page();
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
164
.NET/ASP.NET/Razor Syntax.md
Normal file
164
.NET/ASP.NET/Razor Syntax.md
Normal file
|
@ -0,0 +1,164 @@
|
|||
# [Razor Syntax](https://docs.microsoft.com/en-us/aspnet/core/mvc/views/razor)
|
||||
|
||||
## Markup
|
||||
|
||||
```cs
|
||||
@page // set this as razor page
|
||||
|
||||
@model <App>.Models.Entity // if MVC set type of elements passed to the view
|
||||
@model <Page>Model // if Razor page set underlying class
|
||||
|
||||
@* razor comment *@
|
||||
|
||||
// substituite @variable with it's value
|
||||
<tag>@variable</tag>
|
||||
|
||||
@{
|
||||
// razor code block
|
||||
// can contain C# or HTML
|
||||
|
||||
Model // acces to passed @model (MVC)
|
||||
}
|
||||
|
||||
@if (condition) { }
|
||||
|
||||
@for (init, condition, iteration) { }
|
||||
|
||||
@Model.Property // display Property value (MVC)
|
||||
```
|
||||
|
||||
---
|
||||
|
||||
## Tag Helpers (ASP.NET Core)
|
||||
|
||||
**Tag helpers** are reusable components for automating the generation of HTML in Razor Pages. Tag helpers target specific HTML tags.
|
||||
|
||||
Example:
|
||||
|
||||
```html
|
||||
<!-- tag helpers for a lin in ASP.NET MVC -->
|
||||
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Index">Home</a>
|
||||
```
|
||||
|
||||
### Managing Tag Helpers
|
||||
|
||||
The `@addTagHelper` directive makes Tag Helpers available to the view. Generally, the view file is `Pages/_ViewImports.cshtml`, which by default is inherited by all files in the `Pages` folder and subfolders, making Tag Helpers available.
|
||||
|
||||
```cs
|
||||
@using <App>
|
||||
@namespace <App>.Pages // or <Project>.Models
|
||||
@addTagHelper *, Microsoft.AspNetCore.Mvc.TagHelpers
|
||||
```
|
||||
|
||||
The first parameter after `@addTagHelper` specifies the Tag Helpers to load (`*` for all Tag Helpers), and the second parameter (e.g. `Microsoft.AspNetCore.Mvc.TagHelpers`) specifies the assembly containing the Tag Helpers.
|
||||
`Microsoft.AspNetCore.Mvc.TagHelpers` is the assembly for the built-in ASP.NET Core Tag Helpers.
|
||||
|
||||
#### Opting out of individual elements
|
||||
|
||||
It's possible to disable a Tag Helper at the element level with the Tag Helper opt-out character (`!`)
|
||||
|
||||
```cshtml
|
||||
<!-- disable email vaidation -->
|
||||
<!span asp-validation-for="Email" ></!span>
|
||||
```
|
||||
|
||||
### Explicit Tag Helpers
|
||||
|
||||
The `@tagHelperPrefix` directive allows to specify a tag prefix string to enable Tag Helper support and to make Tag Helper usage explicit.
|
||||
|
||||
```cshtml
|
||||
@tagHelpersPrefix th:
|
||||
```
|
||||
|
||||
### Important Tag Helpers (`asp-`) & HTML Helpers (`@Html`)
|
||||
|
||||
[Understanding Html Helpers](https://stephenwalther.com/archive/2009/03/03/chapter-6-understanding-html-helpers)
|
||||
|
||||
```cs
|
||||
@model <App>.Models.Entity
|
||||
|
||||
// Display the name of the property
|
||||
@Html.DisplayNameFor(model => model.EntityProp)
|
||||
@nameof(Model.EntityProp)
|
||||
|
||||
// Display the value of the property
|
||||
@Html.DisplayFor(model => model.EntityProp)
|
||||
@Model.EntityProp
|
||||
|
||||
<from>
|
||||
// use the property as the label, eventyally w/ [DysplayName("...")]
|
||||
<label asp-for="EntityProp"></label>
|
||||
@Html.LabelFor()
|
||||
|
||||
// automatically set the value at form compilation and submission
|
||||
<input asp-for="EntityProp"/>
|
||||
@Html.EditorFor()
|
||||
</from>
|
||||
|
||||
// route config is {Controller}/{Action}/{Id?}
|
||||
<a asp-controller="<Controller>" asp-action="<Action>">Link</a> // link to /Controller/Action
|
||||
<a asp-controller="<Controller>" asp-action="<Action>" asp-route-Id="@model.Id">Link</a> // link to /Controller/Action/Id
|
||||
@Html.ActionLink("<Link Text>", "<Action>", "<Controller>", new { @HTmlAttribute = value, Id = value }) // link to /Controller/Action/Id
|
||||
|
||||
// link to /Controller/Action?queryParameter=value
|
||||
@Html.ActionLink("<Link Text>", "<Action>", "<Controller>", new { @HTmlAttribute = value, queryParameter = value })
|
||||
<a asp-controller="<Controller>" asp-action="<Action>" asp-route-queryParameter="value">Link</a> // asp-route-* for query strings
|
||||
```
|
||||
|
||||
### [Select Tag Helper](https://docs.microsoft.com/en-us/aspnet/core/mvc/views/working-with-forms)
|
||||
|
||||
[StackOverflow](https://stackoverflow.com/a/34624217)
|
||||
[SelectList Docs](https://docs.microsoft.com/en-us/dotnet/api/system.web.mvc.selectlist)
|
||||
|
||||
In `ViewModel.cs`:
|
||||
|
||||
```cs
|
||||
class ViewModel
|
||||
{
|
||||
public int EntityId { get; set; } // value selected in form ends up here
|
||||
|
||||
// object has numeric id and other props
|
||||
public SelectList Entities = new()
|
||||
|
||||
public ViewModel(){ } // parameterless constructor (NEEDED)
|
||||
}
|
||||
```
|
||||
|
||||
In `View.cs`
|
||||
|
||||
```cs
|
||||
@model ViewModel
|
||||
|
||||
<form asp-controller="Controller" asp-action="PostAction">
|
||||
|
||||
<select asp-for"EntityId" asp-items="Model.Enities">
|
||||
</select>
|
||||
|
||||
<button type="submit">Send<button>
|
||||
</form>
|
||||
```
|
||||
|
||||
In `Controller.cs`:
|
||||
|
||||
```cs
|
||||
public IActionResult GetAction()
|
||||
{
|
||||
var vm = new ViewModel();
|
||||
|
||||
vm.Entities = new SelectList(_context.Enities, "Id", "Text"); // fill SelectList
|
||||
vm.EntityId = value; // set selected option (OPTIONAL)
|
||||
|
||||
return View(vm);
|
||||
}
|
||||
|
||||
|
||||
[HttpPost]
|
||||
public IActionResult PostAction(ViewModel)
|
||||
{
|
||||
if(ModelState.IsValid)
|
||||
{
|
||||
// extract info from view model
|
||||
// save to db
|
||||
}
|
||||
}
|
||||
```
|
26
.NET/ASP.NET/WebForms/Page.aspx.cs.md
Normal file
26
.NET/ASP.NET/WebForms/Page.aspx.cs.md
Normal file
|
@ -0,0 +1,26 @@
|
|||
# Page.aspx.cs
|
||||
|
||||
```cs
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.Linq;
|
||||
using System.Web;
|
||||
using System.Web.UI;
|
||||
using System.Web.UI.WebControls;
|
||||
|
||||
namespace Project
|
||||
{
|
||||
public partial class Default : System.Web.UI.Page
|
||||
{
|
||||
protected void Page_Load(object sender, EventArgs e)
|
||||
{
|
||||
|
||||
}
|
||||
|
||||
protected void Control_Event(object sender, EventAtgs e)
|
||||
{
|
||||
// actions on event trigger
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
58
.NET/ASP.NET/WebForms/Page.aspx.md
Normal file
58
.NET/ASP.NET/WebForms/Page.aspx.md
Normal file
|
@ -0,0 +1,58 @@
|
|||
# Page.aspx
|
||||
|
||||
The fist loaded page is `Default.aspx` and its undelying code.
|
||||
|
||||
```html
|
||||
<!-- directive -->
|
||||
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="Project.Default" %>
|
||||
|
||||
<!DOCTYPE html>
|
||||
|
||||
<html xmlns="http://www.w3.org/1999/xhtml"> <!-- XML Namespace -->
|
||||
<head runat="server"> <!-- runat: handle as ASP code -->
|
||||
<title></title>
|
||||
</head>
|
||||
<body>
|
||||
<!-- web forms require a form tag to be the whole body -->
|
||||
<form id="form1" runat="server"> <!-- runat: handle as ASP code -->
|
||||
<div>
|
||||
</div>
|
||||
</form>
|
||||
</body>
|
||||
</html>
|
||||
```
|
||||
|
||||
## ASP.NET Directives
|
||||
|
||||
### Page Directive
|
||||
|
||||
```cs
|
||||
<%@ Page Language="C#" // define language used (can be C# or VB)
|
||||
AutoEventWireup="true" // automatically create and setup event handlers
|
||||
CodeBehind="Default.aspx.cs" // define the underlying code file
|
||||
Inherits="EmptyWebForm.Default" %>
|
||||
```
|
||||
|
||||
## Web Controls
|
||||
|
||||
```xml
|
||||
<asp:Control ID="" runat="server" ...></asp:Control>
|
||||
|
||||
<!-- Label: empty text will diplay ID, use empty space as text for empty label -->
|
||||
<asp:Label ID="lbl_" runat="server" Text=" "></asp:Label>
|
||||
<!-- TextBox -->
|
||||
<asp:TextBox ID="txt_" runat="server"></asp:TextBox>
|
||||
<!-- Button -->
|
||||
<asp:Button ID="btn_" runat="server" Text="ButtonText" OnClick="btn_Click" />
|
||||
<!-- HyperLink -->
|
||||
<asp:HyperLink ID="lnk_" runat="server" NavigateUrl="~/Page.aspx">LINK TEXT</asp:HyperLink>
|
||||
<!-- LinkButton: POstBackEvent reloads the page -->
|
||||
<asp:LinkButton ID="lbtHome" runat="server" PostBackUrl="~/Page.aspx" OnClick="lbt_Click">BUTTON TEXT</asp:LinkButton>
|
||||
<!-- Image -->
|
||||
<asp:Image ID="img_" runat="server" ImageUrl="~/Images/image.png"/>
|
||||
<!-- ImageButton -->
|
||||
<asp:ImageButton ID="imb_" runat="server" ImageUrl="~/Images/image.png" PostBackUrl="~/Page.aspx"/>
|
||||
|
||||
<!-- SqlSataSource; connection string specified in Web.config -->
|
||||
<asp:SqlDataSource ID="sds_" runat="server" ConnectionString="<%$ ConnectionStrings:ConnectionString %>" SelectCommand="SQL Query"></asp:SqlDataSource>
|
||||
```
|
305
.NET/C#/C# Collections.md
Normal file
305
.NET/C#/C# Collections.md
Normal file
|
@ -0,0 +1,305 @@
|
|||
# C# Collections
|
||||
|
||||
## Arrays
|
||||
|
||||
An array is an object that contains multiple elements of a particular type. The number of elements is fixed for the lifetime of the array, so it must be specied when the array is created.
|
||||
|
||||
An array type is always a reference type, regardless of the element type. Nonetheless, the choice between reference type and value type elements makes a significant difference in an array’s behavior.
|
||||
|
||||
```cs
|
||||
type[] array = new type[dimension];
|
||||
type array[] = new type[dimension]; //invalid
|
||||
|
||||
type[] array = {value1, value2, ..., valueN}; // initializer
|
||||
var array = new type[] {value1, value2, ..., valueN}; // initializer (var type needs new operator)
|
||||
var array = new[] {value1, value2, ..., valueN}; // initializer w/ element type inference (var type needs new operator), can be used as method arg
|
||||
|
||||
array[index]; // value acces
|
||||
array[index] = value; // value assignement
|
||||
array.Lenght; // dimension of the array
|
||||
|
||||
// from IEnumerable<T>
|
||||
array.OfType<Type>(); // filter array based on type, returns IEnumerable<Type>
|
||||
```
|
||||
|
||||
### [Array Methods](https://docs.microsoft.com/en-us/dotnet/api/system.array?view=netcore-3.1#methods)
|
||||
|
||||
```cs
|
||||
// overloaded search methods
|
||||
Array.IndexOf(array, item); // return index of searched item in passed array
|
||||
Array.LastIndexOf(array, item); // return index of searched item staring from the end of the arrary
|
||||
Array.FindIndex(array, Predicate<T>) // returns the index of the first item matching the predicate (can be lambda function)
|
||||
Array.FindLastIndex(array, Predicate<T>) // returns the index of the last item matching the predicate (can be lambda function)
|
||||
Array.Find(array, Predicate<T>) // returns the value of the first item matching the predicate (can be lambda function)
|
||||
Array.FindLast(array, Predicate<T>) // returns the value of the last item matvhing the predicate (can be lambda function)
|
||||
Array.FindAll(array, Predicate<T>) // returns array of all items matching the predicate (can be lambda function)
|
||||
Array.BinarySearch(array, value) // Searches a SORTED array for a value, using a binary search algorithm; retuns the index of the found item
|
||||
|
||||
Array.Sort(array);
|
||||
Array.Reverse(array); // reverses the order of array elements
|
||||
Array.Clear(start_index, x); //removes reference to x elements starting at start index. Dimension of array uncanged (cleared elements value is set tu null)
|
||||
Array.Resize(ref array, target_dimension); //expands or shrinks the array dimension. Shrinking drops trailing values. Array passed by reference.
|
||||
|
||||
// Copies elements from an Array starting at the specified index and pastes them to another Array starting at the specified destination index.
|
||||
Array.Copy(sourceArray, sourceStartIndex, destinationArray, destinationStartIndex, numItemsToCopy);
|
||||
// Copies elements from an Array starting at the first element and pastes them into another Array starting at the first element.
|
||||
Array.Copy(sourceArray, destinationArray, numItemsToCopy);
|
||||
Array.Clone(); // returns a shallow copy of the array
|
||||
```
|
||||
|
||||
### Multidimensional Arrays
|
||||
|
||||
C# supports two multidimensional array forms: [jagged][jagg_arrays] arrays and [rectangular][rect_arrays] arrays (*matrices*).
|
||||
|
||||
[jagg_arrays]: https://docs.microsoft.com/en-us/dotnet/csharp/programming-guide/arrays/jagged-arrays
|
||||
[rect_arrays]: https://docs.microsoft.com/en-us/dotnet/csharp/programming-guide/arrays/multidimensional-arrays
|
||||
|
||||
```cs
|
||||
//specify first dimension
|
||||
type[][] jagged = new type[][]
|
||||
{
|
||||
new[] {item1, item2, item3},
|
||||
new[] {item1},
|
||||
new[] {item1, item2},
|
||||
...
|
||||
}
|
||||
|
||||
// shorthand
|
||||
type[][] jagged =
|
||||
{
|
||||
new[] {item1, item2, item3},
|
||||
new[] {item1},
|
||||
new[] {item1, item2},
|
||||
...
|
||||
}
|
||||
|
||||
// matrices
|
||||
type[,] matrix = new type[n, m]; // n * m matrix
|
||||
type[,] matrix = {{}, {}, {}, ...}; // {} for each row to initialize
|
||||
type[, ,] tensor = new type[n, m, o] // n * m * o tensor
|
||||
|
||||
matrix.Length; // total numeber of elements (n * m)
|
||||
matrix.GetLenght(int dimension); // get the size of a particular direction
|
||||
// row = 0, column = 1, ...
|
||||
```
|
||||
|
||||
## [Lists](https://docs.microsoft.com/en-us/dotnet/api/system.collections.generic.list-1)
|
||||
|
||||
`List<T>` stores sequences of elements. It can grow or shrink, allowing to add or remove elements.
|
||||
|
||||
```cs
|
||||
using System.Collections.Generics;
|
||||
|
||||
List<T> list = new List<T>();
|
||||
List<T> list = new List<T> {item_1, ...}; // initialized usable since list implements IEnumerable<T> and has Add() method (even extension method)
|
||||
List<T> list = new List<T>(dimension); // set list starting dimension
|
||||
List<T> list = new List<T>(IEnumerable<T>); // create a list from an enumerable collection
|
||||
|
||||
|
||||
list.Add(item); //item insertion into the list
|
||||
list.AddRange(IEnumerable<T> collection); // insert multiple items
|
||||
list.Insert(index, item); // insert an item at the specified index
|
||||
list.InsertRange(index, item); // insert items at the specified index
|
||||
|
||||
list.IndexOf(item); // return index of searched item in passed list
|
||||
list.LastIndexOf(item); // return index of searched item staring from the end of the arrary
|
||||
list.FindIndex(Predicate<T>) // returns the index of the first item matching the predicate (can be lambda function)
|
||||
list.FindLastIndex(Predicate<T>) // returns the index of the last item matching the predicate (can be lambda function)
|
||||
list.Find(Predicate<T>) // returns the value of the first item matching the predicate (can be lambda function)
|
||||
list.FindLast(Predicate<T>) // returns the value of the last item matvhing the predicate (can be lambda function)
|
||||
list.FindAll(Predicate<T>) // returns list of all items matching the predicate (can be lambda function)
|
||||
list.BinarySearch(value) // Searches a SORTED list for a value, using a binary search algorithm; retuns the index of the found item
|
||||
|
||||
list.Remove(item); // remove item from list
|
||||
list.RemoveAt(index); // remove item at specified position
|
||||
list.RemoveRange(index, quantity); // remove quantity items at specified position
|
||||
|
||||
list.Contains(item); // check if item is in the list
|
||||
list.TrueForAll(Predicate<T>); // Determines whether every element matches the conditions defined by the specified predicate
|
||||
|
||||
list[index]; // access to items by index
|
||||
list[index] = value; // modify to items by index
|
||||
list.Count; // number of items in the list
|
||||
|
||||
list.Sort(); // sorts item in crescent order
|
||||
list.Reverse(); // Reverses the order of the elements in the list
|
||||
|
||||
// from IEnumerable<T>
|
||||
list.OfType<Type>(); // filter list based on type, returns IEnumerable<Type>
|
||||
list.OfType<Type>().ToList(); // filter list based on type, returns List<Type>
|
||||
```
|
||||
|
||||
## [Iterators](https://docs.microsoft.com/en-us/dotnet/csharp/programming-guide/concepts/iterators)
|
||||
|
||||
An iterator can be used to step through collections such as lists and arrays.
|
||||
|
||||
An iterator method or `get` accessor performs a custom iteration over a collection. An iterator method uses the `yield return` statement to return each element one at a time.
|
||||
When a `yield return` statement is reached, the current location in code is remembered. Execution is restarted from that location the next time the iterator function is called.
|
||||
|
||||
It's possible to use a `yield break` statement or exception to end the iteration.
|
||||
|
||||
**Note**: Since an iteartor returns an `IEnumerable<T>` is can be used to implement a `GetEnumerator()`.
|
||||
|
||||
```cs
|
||||
// simple iterator
|
||||
public static System.Collections.IEnumerable<int> IterateRange(int start = 0, int end)
|
||||
{
|
||||
for(int i = start; i < end; i++){
|
||||
yield return i;
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## List & Sequence Interfaces
|
||||
|
||||
### [`IEnumerable<T>`](https://docs.microsoft.com/en-us/dotnet/api/system.collections.generic.ienumerable-1)
|
||||
|
||||
Exposes the enumerator, which supports a simple iteration over a collection of a specified type.
|
||||
|
||||
```cs
|
||||
public interface IEnumerable<out T> : IEnumerable
|
||||
{
|
||||
IEnumerator<T> GetEnumerator(); // retrun an enumerator
|
||||
}
|
||||
|
||||
// iterate through a collection
|
||||
public interface IEnumerator<T>
|
||||
{
|
||||
// properties
|
||||
object Current { get; } // Get the element in the collection at the current position of the enumerator.
|
||||
|
||||
// methods
|
||||
void IDisposable.Dispose(); // Perform application-defined tasks associated with freeing, releasing, or resetting unmanaged resources
|
||||
bool MoveNext(); // Advance the enumerator to the next element of the collection.
|
||||
void Reset(); // Set the enumerator to its initial position, which is before the first element in the collection.
|
||||
}
|
||||
```
|
||||
|
||||
**Note**: must call `Dispose()` on enumerators once finished with them, because many of them rely on this. `Reset()` is legacy and can, in some situations, throw `NotSupportedException()`.
|
||||
|
||||
### [`ICollection<T>`](https://docs.microsoft.com/en-us/dotnet/api/system.collections.generic.icollection-1)
|
||||
|
||||
```cs
|
||||
public interface ICollection<T> : IEnumerable<T>
|
||||
{
|
||||
// properties
|
||||
int Count { get; } // Get the number of elements contained in the ICollection<T>
|
||||
bool IsReadOnly { get; } // Get a value indicating whether the ICollection<T> is read-only
|
||||
|
||||
// methods
|
||||
void Add (T item); // Add an item to the ICollection<T>
|
||||
void Clear (); // Removes all items from the ICollection<T>
|
||||
bool Contains (T item); // Determines whether the ICollection<T> contains a specific value
|
||||
IEnumerator GetEnumerator (); // Returns an enumerator that iterates through a collection
|
||||
bool Remove (T item); // Removes the first occurrence of a specific object from the ICollection<T>
|
||||
}
|
||||
```
|
||||
|
||||
### [`IList<T>`](https://docs.microsoft.com/en-us/dotnet/api/system.collections.generic.ilist-1)
|
||||
|
||||
```cs
|
||||
public interface IList<T> : ICollection<T>, IEnumerable<T>
|
||||
{
|
||||
// properties
|
||||
int Count { get; } // Get the number of elements contained in the ICollection<T>
|
||||
bool IsReadOnly { get; } // Get a value indicating whether the ICollection<T> is read-only
|
||||
T this[int index] { get; set; } // Get or set the element at the specified index
|
||||
|
||||
// methods
|
||||
void Add (T item); // Add an item to the ICollection<T>
|
||||
void Clear (); // Remove all items from the ICollection<T>
|
||||
bool Contains (T item); // Determine whether the ICollection<T> contains a specific value
|
||||
void CopyTo (T[] array, int arrayIndex); // Copy the elements of the ICollection<T> to an Array, starting at a particular Array index
|
||||
IEnumerator GetEnumerator (); // Return an enumerator that iterates through a collection
|
||||
int IndexOf (T item); // Determine the index of a specific item in the IList<T>
|
||||
void Insert (int index, T item); // Insert an item to the IList<T> at the specified index
|
||||
bool Remove (T item); // Remove the first occurrence of a specific object from the ICollection<T>
|
||||
oid RemoveAt (int index); // Remove the IList<T> item at the specified index
|
||||
}
|
||||
```
|
||||
|
||||
## `Span<T>`
|
||||
|
||||
```cs
|
||||
t[] array = {...}
|
||||
Span<T> wholeArraySpan = array;
|
||||
Span<T> slice = array[start..end];
|
||||
ReadOnlySpan<T> slice = array[start..end];
|
||||
```
|
||||
|
||||
## [Dictioanaries](https://docs.microsoft.com/en-us/dotnet/api/system.collections.generic.dictionary-2)
|
||||
|
||||
[ValueCollection](https://docs.microsoft.com/en-us/dotnet/api/system.collections.generic.dictionary-2.valuecollection)
|
||||
[KeyCollection](https://docs.microsoft.com/en-us/dotnet/api/system.collections.generic.dictionary-2.keycollection)
|
||||
|
||||
**Notes**:
|
||||
|
||||
- Enumerating a dictionary will return `KeyValuePair<TKey, TValue>`.
|
||||
- The `Dictionary<TKey, TValue>` collection class relies on hashes to offer fast lookup (`TKey` should have a good `GetHashCode()`).
|
||||
|
||||
```cs
|
||||
Dictionary<TKey, TValue> dict = new Dictionary<TKey, TValue>(); // init empty dict
|
||||
Dictionary<TKey, TValue> dict = new Dictionary<TKey, TValue>(IEqualityComparer<TKey>); // specify key comparer (TKey must implement Equals() and GetHashCode())
|
||||
|
||||
// initializer (implicitly uses Add method)
|
||||
Dictionary<TKey, TValue> dict =
|
||||
{
|
||||
{ key, value }
|
||||
{ key, value },
|
||||
...
|
||||
}
|
||||
|
||||
// object initializer
|
||||
Dictionary<TKey, TValue> dict =
|
||||
{
|
||||
[key] = value,
|
||||
[key] = value,
|
||||
...
|
||||
}
|
||||
|
||||
// indexer access
|
||||
dict[key]; // read value associted with key (throws KeyNotFoundException if key does not exist)
|
||||
dict[key] = value; // modify value associted with key (throws KeyNotFoundException if key does not exist)
|
||||
|
||||
dict.Count; // numer of key-value pair stored in the dict
|
||||
dict.Keys; // Dictionary<TKey,TValue>.KeyCollection containing the keys of the dict
|
||||
dict.Values; // Dictionary<TKey,TValue>.ValueCollection containing the values of the dict
|
||||
|
||||
dict.Add(key, value); // ArgumentException if the key already exists
|
||||
dict.Clear(); // empty the dictionary
|
||||
dict.ContainsKey(key); // check if a key is in the dictionary
|
||||
dict.ContainsValue(value); // check if a value is in the dictionary
|
||||
dict.Remove(key); // remove a key-value pair
|
||||
dict.Remove(key, out var); // remove key-value pair and copy TValue to var parameter
|
||||
dict.TryAdd(key, value); // adds a key-value pair; returns true if pair is added, false otherwise
|
||||
dict.TryGetValue(key, out var); // put the value associated with kay in the var parameter; true if the dict contains an element with the specified key, false otherwise.
|
||||
```
|
||||
|
||||
## [Sets](https://docs.microsoft.com/en-us/dotnet/api/system.collections.generic.hashset-1)
|
||||
|
||||
Collection of non duplicate items.
|
||||
|
||||
```cs
|
||||
HashSet<T> set = new HashSet<T>();
|
||||
|
||||
set.Add(T); // adds an item to the set; true if the element is added, false if the element is already present.
|
||||
set.Clear(); //Remove all elements from a HashSet<T> object.
|
||||
set.Contains(T); // Determine whether a HashSet<T> object contains the specified element.
|
||||
set.CopyTo(T[]); // Coy the elements of a HashSet<T> object to an array.
|
||||
set.CopyTo(T[], arrayIndex); // Copy the elements of a HashSet<T> object to an array, starting at the specified array index.
|
||||
set.CopyTo(T[], arrayIndex, count); // Copies the specified number of elements of a HashSet<T> object to an array, starting at the specified array index.
|
||||
set.CreateSetComparer(); // Return an IEqualityComparer object that can be used for equality testing of a HashSet<T> object.
|
||||
set.ExceptWith(IEnumerable<T>); // Remove all elements in the specified collection from the current HashSet<T> object.
|
||||
set.IntersectWith(IEnumerable<T>); // Modify the current HashSet<T> object to contain only elements that are present in that object and in the specified collection.
|
||||
set.IsProperSubsetOf(IEnumerable<T>); // Determine whether a HashSet<T> object is a proper subset of the specified collection.
|
||||
set.IsProperSupersetOf(IEnumerable<T>); // Determine whether a HashSet<T> object is a proper superset of the specified collection.
|
||||
set.IsSubsetOf(IEnumerable<T>); // Determine whether a HashSet<T> object is a subset of the specified collection.
|
||||
set.IsSupersetOf(IEnumerable<T>); // Determine whether a HashSet<T> object is a superset of the specified collection.
|
||||
set.Overlaps(IEnumerable<T>); // Determine whether the current HashSet<T> object and a specified collection share common elements.
|
||||
set.Remove(T); // Remove the specified element from a HashSet<T> object.
|
||||
set.RemoveWhere(Predicate<T>); // Remove all elements that match the conditions defined by the specified predicate from a HashSet<T> collection.
|
||||
set.SetEquals(IEnumerable<T>); // Determine whether a HashSet<T> object and the specified collection contain the same elements.
|
||||
set.SymmetricExceptWith(IEnumerable<T>); // Modify the current HashSet<T> object to contain only elements that are present either in that object or in the specified collection, but not both.
|
||||
set.UnionWith(IEnumerable<T>); // Modify the current HashSet<T> object to contain all elements that are present in itself, the specified collection, or both.
|
||||
set.TryGetValue(T, out T); // Search the set for a given value and returns the equal value it finds, if any.
|
||||
```
|
2883
.NET/C#/C#.md
Normal file
2883
.NET/C#/C#.md
Normal file
File diff suppressed because it is too large
Load diff
71
.NET/C#/LINQ .md
Normal file
71
.NET/C#/LINQ .md
Normal file
|
@ -0,0 +1,71 @@
|
|||
# LINQ
|
||||
|
||||
## LINQ to Objects
|
||||
|
||||
<!-- Page: 423/761 of "Ian Griffiths - Programming C# 8.0 - Build Cloud, Web, and Desktop Applications.pdf" -->
|
||||
|
||||
The term **LINQ to Objects** refers to the use of LINQ queries with any `IEnumerable` or `IEnumerable<T>` collection directly, without the use of an intermediate LINQ provider or API such as [LINQ to SQL](https://docs.microsoft.com/en-us/dotnet/framework/data/adonet/sql/linq/) or [LINQ to XML](https://docs.microsoft.com/en-us/dotnet/standard/linq/linq-xml-overview).
|
||||
|
||||
LINQ to Objects will be used when any `IEnumerable<T>` is specified as the source, unless a more specialized provider is available.
|
||||
|
||||
### Query Expressions
|
||||
|
||||
All query expressions are required to begin with a `from` clause, which specifies the source of the query.
|
||||
The final part of the query is a `select` (or `group`) clause. This determines the final output of the query and its sytem type.
|
||||
|
||||
```cs
|
||||
// query expression
|
||||
var result = from item in enumerable select item;
|
||||
|
||||
// where clause
|
||||
var result = from item in enumerable where condition select item;
|
||||
|
||||
// ordering
|
||||
var result = from item in enumerable orderby item.property select item; // ordered Ienumerble
|
||||
|
||||
// let clause
|
||||
var result = from item in enumerable let tmp = <sub-expr> ... // assign expression to variable to avoid re-evaluetion on each cycle
|
||||
// BEWARE: compiled code has a lot of overhead to satisfy let caluse
|
||||
|
||||
// grouping (difficult to re-implement to obtain better performance)
|
||||
var result = from item in enumerable group item by item.property; // returns IEnumerable<IGrouping<TKey,TElement>>
|
||||
```
|
||||
|
||||
### How Query Expressions Expand
|
||||
|
||||
The compiler converts all query expressions into one or more method calls. Once it has done that, the LINQ provider is selected through exactly the same mechanisms that C# uses for any other method call.
|
||||
The compiler does not have any built-in concept of what constitutes a LINQ provider.
|
||||
|
||||
```cs
|
||||
// expanded query expression
|
||||
var result = Enumerable.Where(item => condition).Select(item => item);
|
||||
```
|
||||
|
||||
The `Where` and `Select` methods are examples of LINQ operators. A LINQ operator is nothing more than a method that conforms to one of the standard patterns.
|
||||
|
||||
### Methods on `Enumerable` or `IEnumerable<T>`
|
||||
|
||||
```cs
|
||||
Enumerable.Range(int start, int end); // IEnumerable<int> of values between start & end
|
||||
|
||||
// max item in the IEnumerable
|
||||
IEnumerable<T>.Max(); // source must implement IComparable<T>
|
||||
|
||||
// check if condition is true for all IEnumerbale
|
||||
IEnumerable<T>.All(IEnumerable<T> source, Func<T, bool> predicate);
|
||||
IEnumerable<T>.All(IEnumerable<T> source.Predicate);
|
||||
```
|
||||
|
||||
## LINQ to JSON (JSON.NET)
|
||||
|
||||
Parses JSON data into objects of type `JObject`, `JArray`, `JProperty`, and `JValue`, all of which derivefrom a `JToken` base class.
|
||||
Using these types is similar to working with JSON from JavaScript: it's possible to access the content directly without having to define classes.
|
||||
|
||||
```cs
|
||||
var jo = (JObject) JToken.Parse(json); // parse json
|
||||
|
||||
var propery = jo["property"].Value<Type>(); // extract and convert data from json
|
||||
|
||||
// linq query
|
||||
IENumerable<JProperty> props = jo.Descendants().OfType<JProperty>().Where(p => condition);
|
||||
```
|
39
.NET/C#/Reactive Extensions.md
Normal file
39
.NET/C#/Reactive Extensions.md
Normal file
|
@ -0,0 +1,39 @@
|
|||
# Reactive Extensions (Rx)
|
||||
|
||||
The **Reactive Extensions** for .NET, or **Rx**, are designed for working with asynchro‐nous and event-based sources of information.
|
||||
Rx provides services that help orchestrate and synchronize the way code reacts to data from these kinds of sources.
|
||||
|
||||
Rx’s fundamental abstraction, `IObservable<T>`, represents a sequence of items, and its operators are defined as extension methods for this interface.
|
||||
|
||||
This might sound a lot like LINQ to Objects, and there are similarities, not only does `IObservable<T>` have a lot in common with `IEnumerable<T>`, but Rx also supports almost all of the standard LINQ operators.
|
||||
|
||||
The difference is that in Rx, sequences are less passive. Unlike `IEnumerable<T>`, Rx sources do not wait to be asked for their items, nor can the consumer
|
||||
of an Rx source demand to be given the next item. Instead, Rx uses a *push* model in which *the source notifies* its recipients when items are available.
|
||||
|
||||
Because Rx implements standard LINQ operators, it's possible to write queries against a live source. Rx goes beyond standard LINQ, adding its own operators that take into account the temporal nature of a live event source.
|
||||
|
||||
## Foundamental Interfaces
|
||||
|
||||
The two most important types in Rx are the `IObservable<T>` and `IObserver<T>` interfaces. They are important enough to be in the System namespace.
|
||||
The other parts of Rx are in the `System.Reactive` NuGet package.
|
||||
|
||||
```cs
|
||||
public interface IObservable<out T>
|
||||
{
|
||||
IDisposable Subscribe(IObserver<T> observer);
|
||||
}
|
||||
|
||||
public interface IObserver<in T>
|
||||
{
|
||||
void OnCompleted();
|
||||
void OnError(Exception error);
|
||||
void OnNext(T value);
|
||||
}
|
||||
```
|
||||
|
||||
The fundamental abstraction in Rx, `IObservable<T>`, is implemented by *event sources*. Instead of using the `event` keyword, it models events as a *sequence of items*. An `IObservable<T>` provides items to subscribers as and when it’s ready to.
|
||||
|
||||
It's possible to subscribe to a source by passing an implementation of `IObserver<T>` to the `Subscribe` method.
|
||||
The source will invoke `OnNext` when it wants to report events, and it can call `OnCompleted` to indicate that there will be no further activity.
|
||||
If the source wants to report an error, it can call `OnError`.
|
||||
Both `OnCompleted` and `OnError` indicate the end of the stream, an observable should not call any further methods on the observer after that.
|
113
.NET/Database/ADO.NET.md
Normal file
113
.NET/Database/ADO.NET.md
Normal file
|
@ -0,0 +1,113 @@
|
|||
# [ADO.NET](https://docs.microsoft.com/en-us/dotnet/framework/data/adonet/ "ADO.NET Docs")
|
||||
|
||||
`ADO.NET` is a set of classes that expose data access services for .NET.
|
||||
The `ADO.NET` classes are found in `System.Data.dll`, and are integrated with the XML classes found in `System.Xml.dll`.
|
||||
|
||||
[ADO.NET provider for SQLite](https://system.data.sqlite.org/index.html/doc/trunk/www/index.wiki "System.Data.SQLite")
|
||||
|
||||
## [Connection Strings](https://www.connectionstrings.com)
|
||||
|
||||
### [SQL Server 2019](https://www.connectionstrings.com/sql-server-2019/)
|
||||
|
||||
- Standard Security:
|
||||
- `Server=<server_name>; Database=<database>; UID=<user>; Pwd=<password>;`
|
||||
- `Server=<server_name>; Database=<database>; User ID=<user>; Password=<password>;`
|
||||
- `Data Source=<server_name>; Initial Catalog=<database>; UID=<user>; Pwd=<password>;`
|
||||
- Specific Instance: `Server=<server_name>\<instance_name>; Database=<database>; User ID=<user>; Password=<password>;`
|
||||
- Trusted Connection (WinAuth): `Server=<server_name>; Database=<database>; Trusted_Connection=True;`
|
||||
- MARS: `Server=<server_name>; Database=<database>; Trusted_Connection=True; MultipleActiveResultSets=True;`
|
||||
|
||||
**NOTE**: *Multiple Active Result Sets* (MARS) is a feature that works with SQL Server to allow the execution of multiple batches on a single connection.
|
||||
|
||||
### [SQLite](https://www.connectionstrings.com/sqlite/)
|
||||
|
||||
- Basic: `Data Source: path\to\db.sqlite3; Version=3;`
|
||||
- In-Memory Dataabse: `Data Source=:memory:; Version=3; New=True`
|
||||
- With Password: `Data Source: path\to\db.sqlite3; Version=3; Password=<password>`
|
||||
|
||||
## Connection to DB
|
||||
|
||||
```cs
|
||||
using System;
|
||||
using System.Data.SqlClient; // ADO.NET Provider, installed through NuGet
|
||||
|
||||
namespace <namespace>
|
||||
{
|
||||
class Program
|
||||
{
|
||||
static void Main(string[] args)
|
||||
{
|
||||
// Connection to SQL Server DBMS
|
||||
SqlConnectionStringBuilder connectionString = new SqlConnectionStringBuilder();
|
||||
connectionString.DataSource = "<server_name>";
|
||||
connectionString.UserID = "<user>";
|
||||
connectionString.Password = "<password>";
|
||||
connectionString.InitialCatalog = "<database>";
|
||||
|
||||
// more compact
|
||||
SqlConnectionStringBuilder connectionString = new SqlConnectionStringBuilder("Server=<server_name>;Database=<database>;UID=<user>;Pwd=<password>")
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## DB Interrogation
|
||||
|
||||
### `SqlConnection`
|
||||
|
||||
```cs
|
||||
using (SqlConnection connection = new SqlConnection())
|
||||
{
|
||||
connection.ConnectionString = connectionString.ConnectionString;
|
||||
connection.Open(); // start comunication w/ sql server
|
||||
}
|
||||
|
||||
// more compact
|
||||
using (SqlConnection connection = new SqlConnection(connectionString)) {
|
||||
connection.Open()
|
||||
}
|
||||
```
|
||||
|
||||
### [SqlCommand](https://docs.microsoft.com/en-us/dotnet/api/system.data.sqlclient.sqlcommand)
|
||||
|
||||
```cs
|
||||
string sql = "<sql_instruction>";
|
||||
|
||||
using (SqlCommand command = new SqlCommand())
|
||||
{
|
||||
command.Connection = connection; // SqlConnection
|
||||
command.CommandText = "... @Parameter"; // or name of StoredProcedure
|
||||
|
||||
// add parameters to the SqlParameterCollection, WARNING: table names or columns cannot be parameters
|
||||
command.Parameters.Add("@Parameter", SqlDbType.<DBType>, columnLength).Value = value;
|
||||
command.Parameters.AddWithValue("@Parameter", value);
|
||||
command.Parameters.AddWithValue("@Parameter", (object) value ?? DBNull.Value); // if Parameter is nullable
|
||||
|
||||
// Create an instance of a SqlParameter object.
|
||||
command.CreateParameter();
|
||||
|
||||
command.CommandType = CommandType.Text; // or StoredProcedure
|
||||
|
||||
int affectedRows = command.ExecuteNonQuery(); // execute the query and return the number of affected rows
|
||||
}
|
||||
```
|
||||
|
||||
### `SqlDataReader`
|
||||
|
||||
```cs
|
||||
using (SqlDataReader cursor = command.ExecuteReader()) // object to get data from db
|
||||
{
|
||||
while (cursor.Read()) // get data till possible
|
||||
{
|
||||
// preferred methodology
|
||||
cursor["<column_name>"].ToString(); // retrieve data form the column
|
||||
cursor[<column_index>].ToString(); // retrieve data form the column
|
||||
|
||||
// check for null before retrieving the value
|
||||
if(!cursor.IsDBNull(n))
|
||||
{
|
||||
sqlreader.Get<SystemType>(index); // retrieve data form the n-th column
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
203
.NET/Database/EntityFramework.md
Normal file
203
.NET/Database/EntityFramework.md
Normal file
|
@ -0,0 +1,203 @@
|
|||
# Entity Framework
|
||||
|
||||
## Model & Data Annotations
|
||||
|
||||
```cs
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.ComponentModel.DataAnnotations;
|
||||
using System.Linq;
|
||||
|
||||
namespace <Project>.Model
|
||||
{
|
||||
public class Entity
|
||||
{
|
||||
[Key] // set as PK (Id & EntityId are automatically detected to be PKs)
|
||||
public int Id { get; set; }
|
||||
|
||||
[Required]
|
||||
public Type ForeignObject { get; set; } // Not Null in DB
|
||||
public Type ForeignObject { get; set; } // Allow Null in DB
|
||||
|
||||
public int Prop { get; set; } // Not Null in DB (primitive are not nullable)
|
||||
public int? Prop { get; set; } // Allow Null in DB
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Context
|
||||
|
||||
NuGet Packages to install:
|
||||
|
||||
- `Microsoft.EntityFrameworkCore`
|
||||
- `Microsoft.EntityFrameworkCore.Tools` to use migrations
|
||||
- `Microsoft.EntityFrameworkCore.Design` *or* `Microsoft.EntityFrameworkCore.<db_provider>.Design` needed for tools to work (bundled w\ tools)
|
||||
- `Microsoft.EntityFrameworkCore.<db_provider>`
|
||||
|
||||
```cs
|
||||
using Microsoft.EntityFrameworkCore;
|
||||
|
||||
namespace <Project>.Model
|
||||
{
|
||||
class Context : DbContext
|
||||
{
|
||||
public Context(DbContextOptions options) : base(options)
|
||||
{
|
||||
}
|
||||
}
|
||||
|
||||
// or
|
||||
|
||||
class Context : DbContext
|
||||
{
|
||||
private const string _connectionString = "Server=<server_name>;Database=<database>;UID=<user>;Pwd=<password>";
|
||||
|
||||
// connect to db
|
||||
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
|
||||
{
|
||||
optionsBuilder.UseSqlServer(_connectionString); // specify connection
|
||||
}
|
||||
|
||||
//DBSet<TEntity> represents the collection of all entities in the context (or that can be queried from the database) of a given type
|
||||
public DbSet<Entity> Entities { get; set; }
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Migrations
|
||||
|
||||
Create & Update DB Schema if necessary.
|
||||
|
||||
In Packge Manager Shell:
|
||||
|
||||
```ps1
|
||||
PM> Add-Migration <migration_name>
|
||||
PM> update-database [-Verbose] # use the migrations to modify the db, -Verbose to show SQL queries
|
||||
```
|
||||
|
||||
In dotnet cli:
|
||||
|
||||
```ps1
|
||||
dotnet tool install --global dotnet-ef # if not already installed
|
||||
|
||||
dotnet ef migrations add <migration_name>
|
||||
dotnet ef database update
|
||||
```
|
||||
|
||||
## CRUD
|
||||
|
||||
### Create
|
||||
|
||||
```cs
|
||||
public static bool InsertOne(Entity entity)
|
||||
{
|
||||
int rows = 0;
|
||||
|
||||
using(var context = new Context())
|
||||
{
|
||||
context.Add(entity);
|
||||
context.SaveChanges();
|
||||
}
|
||||
|
||||
return rows == 1;
|
||||
}
|
||||
|
||||
public static bool InsertMany(IEnumerable<Entity> entities)
|
||||
{
|
||||
int rows = 0;
|
||||
|
||||
using(var context = new Context())
|
||||
{
|
||||
context.AddRange(entities);
|
||||
context.SaveChanges();
|
||||
}
|
||||
|
||||
return rows == entities.Count();
|
||||
}
|
||||
```
|
||||
|
||||
### Read
|
||||
|
||||
[Referenced Object Not Loading Fix](https://stackoverflow.com/a/5385288)
|
||||
|
||||
```cs
|
||||
public static List<Entity> SelectAll()
|
||||
{
|
||||
using(var context = new Context())
|
||||
{
|
||||
return context.Entities.ToList();
|
||||
}
|
||||
}
|
||||
|
||||
static Entity SelectOneById(int id)
|
||||
{
|
||||
using(var context = new Context())
|
||||
{
|
||||
return context.Entities.Find(id);
|
||||
|
||||
// force read of foreign key identifying referenced obj
|
||||
return context.Entities.Include(c => c.ForeignObject).Find(id);
|
||||
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Update
|
||||
|
||||
```cs
|
||||
public static bool UpdateOne(Entity entity)
|
||||
{
|
||||
int rows = 0;
|
||||
|
||||
using(var context = new Context())
|
||||
{
|
||||
context.Entities.Update(entity);
|
||||
context.SaveChanges();
|
||||
}
|
||||
|
||||
return rows == 1;
|
||||
}
|
||||
|
||||
public static bool UpdateMany(IEnumerable<Entity> entities)
|
||||
{
|
||||
int rows = 0;
|
||||
|
||||
using(var context = new Context())
|
||||
{
|
||||
context.UpdateRange(entities);
|
||||
context.SaveChanges();
|
||||
}
|
||||
|
||||
return rows == entities.Count();
|
||||
}
|
||||
```
|
||||
|
||||
### Delete
|
||||
|
||||
```cs
|
||||
public static bool DeleteOne(Entity entity)
|
||||
{
|
||||
int rows = 0;
|
||||
|
||||
using(var context = new Context())
|
||||
{
|
||||
context.Entities.Remove(entity);
|
||||
context.SaveChanges();
|
||||
}
|
||||
|
||||
return rows == 1;
|
||||
}
|
||||
|
||||
public static bool DeleteMany(IEnumerable<Entity> entities)
|
||||
{
|
||||
int rows = 0;
|
||||
|
||||
using(var context = new Context())
|
||||
{
|
||||
context.RemoveRange(entities);
|
||||
context.SaveChanges();
|
||||
}
|
||||
|
||||
return rows == entities.Count();
|
||||
}
|
||||
```
|
35
.NET/Lib/SkiaSharp.md
Normal file
35
.NET/Lib/SkiaSharp.md
Normal file
|
@ -0,0 +1,35 @@
|
|||
# SkiaSharp
|
||||
|
||||
```cs
|
||||
SKImageInfo info = new SKImageInfo(width, height);
|
||||
|
||||
using (SKSurface surface = SKSurface.Create(info))
|
||||
{
|
||||
SKCanvas canvas = surface.Canvas;
|
||||
canvas.DrawColor(SKColors.<color>);
|
||||
|
||||
using (SKPaint paint = new SKPaint())
|
||||
{
|
||||
paint.Color = SKColors.Blue;
|
||||
paint.IsAntialias = true;
|
||||
paint.StrokeWidth = 5;
|
||||
paint.Style = SKPaintStyle.Stroke;
|
||||
|
||||
// draw
|
||||
canvas.DrawLine(x0, y0, x1, y1, paint);
|
||||
|
||||
}
|
||||
|
||||
// save to file
|
||||
using (var image = surface.Snapshot())
|
||||
{
|
||||
using (var data = image.Encode(SKEncodedImageFormat.Png, 100))
|
||||
{
|
||||
using (var stream = File.OpenWrite(png_filename))
|
||||
{
|
||||
data.SaveTo(stream);
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
81
.NET/Unity/Collisions.md
Normal file
81
.NET/Unity/Collisions.md
Normal file
|
@ -0,0 +1,81 @@
|
|||
# Collisions (Physics)
|
||||
|
||||
## Rigidbody Component
|
||||
|
||||
Enables physycs on the game objects.
|
||||
|
||||
Rigidbodies collide with other objects instead of going through them.
|
||||
|
||||
Avoid obejct rotation on colisions:
|
||||
|
||||
1. Assign `Rigidbody` component to object
|
||||
2. Enable Freeze Rotaion in Rigidbody > Constraints
|
||||
|
||||
```cs
|
||||
using UnityEngine;
|
||||
using System.Collections;
|
||||
|
||||
public class GameObject : MonoBehaviour {
|
||||
|
||||
Rigidbody = rigidbody; // game object rigidbody reference container
|
||||
|
||||
void Start()
|
||||
{
|
||||
rigidbody = GetComponenet<Rigidbody>(); // get rigidbody reference
|
||||
}
|
||||
|
||||
void Update()
|
||||
{
|
||||
}
|
||||
|
||||
// FixedUpdate is calles every x seconds (not influenced by FPS instability)
|
||||
// used for physics calculations which sould be FPS independant
|
||||
void FixedUpdate()
|
||||
{
|
||||
Time.fixedDeltaTime; // fixed amount of time
|
||||
Time.timeDelta; // if called inside FIxedUpadate() behaves like fixedDeltaTime
|
||||
}
|
||||
|
||||
}
|
||||
```
|
||||
|
||||
## Box Collider Component
|
||||
|
||||
Enable `Is Trigger` to register the collision but avoid blocking the movement of the objects.
|
||||
The trigger can generate a event to signal the contact with the object.
|
||||
|
||||
One of the collidng GameObjects *must have* the `Rigidbody` component and the other `Is Trigger` enabled.
|
||||
To detect the collison but avoid computing the physycs `Is Kinematic` must be enabled in the `Rigidbody` component.
|
||||
|
||||
```cs
|
||||
using UnityEngine;
|
||||
using System.Collections;
|
||||
|
||||
public class GameObject : MonoBehaviour {
|
||||
|
||||
Rigidbody = rigidbody; // game object rigidbody reference container
|
||||
|
||||
void Start()
|
||||
{
|
||||
rigidbody = GetComponenet<Rigidbody>(); // get rigidbody reference
|
||||
}
|
||||
|
||||
// FixedUpdate is calles every x seconds (not influenced by FPS instability)
|
||||
// used for physics calculations which sould be FPS independant
|
||||
void FixedUpdate()
|
||||
{
|
||||
Time.fixedDeltaTime; // fixed amount of time
|
||||
Time.timeDelta; // if called inside FIxedUpadate() behaves like fixedDeltaTime
|
||||
}
|
||||
|
||||
// called on box collision.
|
||||
void OnTriggerEnter(Collider triggerCollider) {
|
||||
|
||||
// detect a collison with a perticular GameObject(must have a TAG)
|
||||
if (triggerCollider.tag = "tag") {
|
||||
Destroy(triggerCollider.gameObject); // destroy tagged item on collision
|
||||
//or
|
||||
Destroy(gameObject); // destroy itself
|
||||
}
|
||||
}
|
||||
```
|
30
.NET/Unity/Coroutines.md
Normal file
30
.NET/Unity/Coroutines.md
Normal file
|
@ -0,0 +1,30 @@
|
|||
# Coroutines
|
||||
|
||||
[Coroutines - Unity manual](https://docs.unity3d.com/Manual/Coroutines.html)
|
||||
|
||||
When you call a function, it runs to completion before returning. This effectively means that any action taking place in a function must happen *within a single frame update*; a function call can’t be used to contain a procedural animation or a sequence of events over time.
|
||||
|
||||
A coroutine is like a function that has the ability to pause execution and return control to Unity but then to continue where it left off on the following frame.
|
||||
|
||||
It is essentially a function declared with a return type of IEnumerator and with the yield return statement included somewhere in the body. The `yield return null` line is the point at which execution will pause and be resumed the following frame.
|
||||
|
||||
```cs
|
||||
//coroutine
|
||||
IEnumerator coroutine()
|
||||
{
|
||||
// action performed
|
||||
yield return null; // pause until next iteration
|
||||
|
||||
// or
|
||||
|
||||
// By default, a coroutine is resumed on the frame after it yields but it is also possible to introduce a time delay
|
||||
yield return new WaitForSeconds(seconds); // wait seconds before resuming
|
||||
|
||||
// or
|
||||
|
||||
yeld return StartCoroutine(coroutine()); // wait for anothe coroitine to finish before starting
|
||||
}
|
||||
|
||||
StartCoroutine(coroutine()); // start the coroutine
|
||||
StopCoroutine(coroutine()); // stop the coroutine
|
||||
```
|
81
.NET/Unity/Input Manager.md
Normal file
81
.NET/Unity/Input Manager.md
Normal file
|
@ -0,0 +1,81 @@
|
|||
# Input Manager
|
||||
|
||||
The Input Manager uses the following types of controls:
|
||||
|
||||
- **Key** refers to any key on a physical keyboard, such as `W`, `Shift`, or the `space bar`.
|
||||
- **Button** refers to any button on a physical controller (for example, gamepads), such as the `X` button on an Xbox One controller.
|
||||
- A **virtual axis** (plural: axes) is mapped to a **control**, such as a button or a key. When the user activates the control, the axis receives a value in the range of `[–1..1]`.
|
||||
|
||||
## Virtual axes
|
||||
|
||||
### Axis Properties
|
||||
|
||||
**Name**: Axis name. You can use this to access the axis from scripts.
|
||||
**Negative Button**, **Positive Button**: The controls to push the axis in the negative and positive direction respectively. These can be keys on a keyboard, or buttons on a joystick or mouse.
|
||||
**Alt Negative Button**, **Alt Positive Button**: Alternative controls to push the axis in the negative and positive direction respectively.
|
||||
**Gravity**: Speed in units per second that the axis falls toward neutral when no input is present.
|
||||
**Dead**: How far the user needs to move an analog stick before your application registers the movement. At runtime, input from all analog devices that falls within this range will be considered null.
|
||||
**Sensitivity**: Speed in units per second that the axis will move toward the target value. This is for digital devices only.
|
||||
**Snap**: If enabled, the axis value will reset to zero when pressing a button that corresponds to the opposite direction.
|
||||
**Type**: The type of input that controls the axis. Select from these values:
|
||||
|
||||
- Key or Mouse button
|
||||
- Mouse Movement
|
||||
- Joystick Axis
|
||||
|
||||
**Axis**: The axis of a connected device that controls this axis.
|
||||
**JoyNum**: The connected Joystick that controls this axis. You can select a specific joystick, or query input from all joysticks.
|
||||
|
||||
### Axis Values
|
||||
|
||||
Axis values can be:
|
||||
|
||||
- Between `–1` and `1` for joystick and keyboard input. The neutral position for these axes is `0`. Some types of controls, such as buttons on a keyboard, aren’t sensitive to input intensity, so they can’t produce values other than `–1`, `0`, or `1`.
|
||||
- Mouse delta (how much the mouse has moved during the last frame) for mouse input. The values for mouse input axes can be larger than `1` or smaller than `–1` when the user moves the mouse quickly.
|
||||
|
||||
```cs
|
||||
//Define the speed at which the object moves.
|
||||
float moveSpeed = 10;
|
||||
|
||||
//Get the value of the Horizontal input axis.
|
||||
float horizontalInput = Input.GetAxis("Horizontal");
|
||||
|
||||
//Get the value of the Vertical input axis.
|
||||
float verticalInput = Input.GetAxis("Vertical");
|
||||
|
||||
Vector3 direction = new Vector3(horizontalInput, 0, verticalInput).normalized;
|
||||
Vector3 velocity = direction * moveSpeed;
|
||||
|
||||
//Move the object to XYZ coordinates defined as horizontalInput, 0, and verticalInput respectively.
|
||||
transform.Translate(velocity * Time.deltaTime);
|
||||
```
|
||||
|
||||
[Time.deltaTime][dt] represents the time that passed since the last frame. Multiplying the moveSpeed variable by Time.deltaTime ensures that the GameObject moves at a constant speed every frame.
|
||||
|
||||
[dt]: https://docs.unity3d.com/ScriptReference/Time-deltaTime.html
|
||||
|
||||
`GetAxis`: returns the value of the virtual axis identified.
|
||||
`GetAxisRaw`: returns the value of the virtual axis identified with no smoothing filtering applied.
|
||||
|
||||
## Keys
|
||||
|
||||
**Letter keys**: `a`, `b`, `c`, ...
|
||||
**Number keys**: `1`, `2`, `3`, ...
|
||||
**Arrow keys**: `up`, `down`, `left`, `right`
|
||||
**Numpad keys**: `[1]`, `[2]`, `[3]`, `[+]`, `[equals]`, ...
|
||||
**Modifier keys**: `right shift`, `left shift`, `right ctrl`, `left ctrl`, `right alt`, `left alt`, `right cmd`, `left cmd`
|
||||
**Special keys**: `backspace`, `tab`, `return`, `escape`, `space`, `delete`, `enter`, `insert`, `home`, `end`, `page up`, `page down`
|
||||
**Function keys**: `f1`, `f2`, `f3`, ...
|
||||
|
||||
**Mouse buttons**: `mouse 0`, `mouse 1`, `mouse 2`, ...
|
||||
|
||||
**Specific button on *any* joystick**: `joystick button 0`, `joystick button 1`, `joystick button 2`, ...
|
||||
**specific button on a *specific* joystick**: `joystick 1 button 0`, `joystick 1 button 1`, `joystick 2 button 0`, ...
|
||||
|
||||
```cs
|
||||
Input.GetKey("a");
|
||||
Input.GetKey(KeyCode.A);
|
||||
```
|
||||
|
||||
[Input.GetKey](https://docs.unity3d.com/ScriptReference/Input.GetKey.html)
|
||||
[KeyCode](https://docs.unity3d.com/ScriptReference/KeyCode.html)
|
14
.NET/Unity/Prefabs Instantiation.md
Normal file
14
.NET/Unity/Prefabs Instantiation.md
Normal file
|
@ -0,0 +1,14 @@
|
|||
# Prefabs
|
||||
|
||||
Prefabs are a blueprint for GameObcets and any change made to the prefab is inherited by all it's instances.
|
||||
|
||||
## Script Instantation
|
||||
|
||||
```cs
|
||||
|
||||
public GameObject prefab; // reference to the prefab
|
||||
|
||||
// instantiate a game GameObject from the prefab with specified position and rotation (rotation must be a quaternion)
|
||||
GameObject newInstance = (GameObject) Instantiate(prefab, positionVector3, Quaternion.Euler(rotationVector3)); // instantiate prefab and get reference to instance
|
||||
// Instance returns a object and since a GameObject was passed to Instantiate() the returned object can be casted to a GameObject
|
||||
```
|
83
.NET/Unity/Raycasting.md
Normal file
83
.NET/Unity/Raycasting.md
Normal file
|
@ -0,0 +1,83 @@
|
|||
# Raycasting Notes
|
||||
|
||||
A raycast is conceptually like a laser beam that is fired from a point in space along a particular directuin. Any object making contact with the beam can be detected and reported.
|
||||
|
||||
## 3D Raycasting
|
||||
|
||||
```cs
|
||||
void Update()
|
||||
{
|
||||
// constructor takes in a position end direction
|
||||
Ray ray = new Ray(transform,position, transform.forward);
|
||||
RaycastHit hitInfo; // struct, stores data on ray collision
|
||||
|
||||
hitInfo.distance // distance from origin to collision point
|
||||
hitInfo.collider // collider of the hit object
|
||||
hitInfo.transform // transform og the hit object
|
||||
hitInfo.collider.gameObject // reference to the object hit by the ray
|
||||
hitInfo.collider.gameObject // reference to the object hit by the ray
|
||||
hitInfo.normal // normal bector og the hit surface
|
||||
hitInfo.point // actual point of collision
|
||||
|
||||
// static method, object must haave a collider dot the collision to happen, returns a BOOL
|
||||
Physics.Raycast(ray, out hitInfo); // update hitInfo based on ray collisions
|
||||
Physics.Raycast(ray, out hitInfo, float maxRayDistance); // limit the ray length
|
||||
Physics.Raycast(ray, out hitInfo, Mask mask); // specify with which layers the ray can interact, layer must be allpied to object's mask
|
||||
Physics.Raycast(ray, out hitInfo, Mask mask, QueryTriggerInteraction.Ignore); // ignore collision if "is trigger" is enabled on other objects
|
||||
|
||||
// detect a collision
|
||||
if (Physics.Raycast(ray, out hitInfo))
|
||||
{
|
||||
//collision happened
|
||||
|
||||
// draw the ray ingame for debuggng
|
||||
Debug.DrawLine(ray.origin, hitInfo.point, Color.red); // draw red line if collision happens
|
||||
}
|
||||
else
|
||||
{
|
||||
Debug.DrawLine(ray.origin, ray.origin + ray.direction * 100, Color.blue); // draw blue line if collision happens, arrival point is 100 units from the origin sinche the ray goes to infinity
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Detect mouse pointed point in-game
|
||||
|
||||
```cs
|
||||
public Camera gameCamera;
|
||||
|
||||
void Update()
|
||||
{
|
||||
// ray goint ftom cametra through a screen point
|
||||
Ray ray = gameCamera.ScreenPointToRay(Input.mousePosition); // Input.mousePosition is the postion of the mouse in pixels (screen points)
|
||||
RaycastHit hitInfo; // place pointed by the mouse
|
||||
|
||||
Physics.Raycast(ray, out hitInfo) // update pointed position
|
||||
}
|
||||
```
|
||||
|
||||
## 2D Raycasting
|
||||
|
||||
```cs
|
||||
|
||||
void Start()
|
||||
{
|
||||
Physisc2D.queriesStartColliders = false; // avoid collision with collider of the ray generator gameObject
|
||||
}
|
||||
|
||||
void Update()
|
||||
{
|
||||
// returns a RaycastHit2D, needs an origin and direction separatedly
|
||||
Raycast2D hitInfo = Physics2D.Raycast(Vector2 origin, Vector2 direction);
|
||||
Raycast2D hitInfo = Physics2D.Raycast(Vector2 origin, Vector2 direction, float maxRayDistance);
|
||||
Raycast2D hitInfo = Physics2D.Raycast(Vector2 origin, Vector2 direction, float maxRayDistance);
|
||||
Raycast2D hitInfo = Physics2D.Raycast(Vector2 origin, Vector2 direction, float minDepth, float maxDepth); // set range of z-coord values in which detect hits (sprites depth)
|
||||
|
||||
//! the ray starts from INSIDE the gameObject and can collider with it's collider
|
||||
|
||||
// detect collision
|
||||
if (hitInfo.collider != null) {
|
||||
// collision happened
|
||||
Debug.DrawLine(transform.position, hitInfo.point)
|
||||
}
|
||||
}
|
||||
```
|
135
.NET/Unity/Scripting.md
Normal file
135
.NET/Unity/Scripting.md
Normal file
|
@ -0,0 +1,135 @@
|
|||
# Unity C# Scripting
|
||||
|
||||
## Logging
|
||||
|
||||
```c#
|
||||
Debug.Log(string); //output message to console (more powerful and flexible than print())
|
||||
Print(string); //output message to console
|
||||
```
|
||||
|
||||
## Scripts
|
||||
|
||||
```c#
|
||||
public class ClassName : MonoBehaviour {
|
||||
|
||||
// Start is called before the first frame update
|
||||
void Start()
|
||||
{
|
||||
|
||||
}
|
||||
|
||||
// Update is called once per frame
|
||||
void Update()
|
||||
{
|
||||
Time.deltaTime; // time since last frame
|
||||
}
|
||||
|
||||
// FixedUpdate is calles every x seconds (not influenced by FPS instability)
|
||||
// used for physics calculations which sould be FPS independant
|
||||
void FixedUpdate()
|
||||
{
|
||||
Time.fixedDeltaTime; // fixed amount of time
|
||||
Time.timeDelta; // if called inside FIxedUpadate() behaves like fixedDeltaTime
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Script comunication
|
||||
|
||||
Referencing data in a sctipt from another.
|
||||
|
||||
```cs
|
||||
//example of a script to be referenced in another
|
||||
Using System;
|
||||
|
||||
public class Player : MonoBheaviour {
|
||||
|
||||
public float health = 10;
|
||||
public event Action OnPlayerDeath; //event of type Action, needs using System
|
||||
|
||||
void Start() {
|
||||
|
||||
}
|
||||
|
||||
void Update() {
|
||||
|
||||
if (health <= 0) {
|
||||
if (OnPlayerDeath != null) {
|
||||
OnPleyerDeath(); // invoke Action (if no subscribers event will be NULL, can cause errors)
|
||||
}
|
||||
|
||||
Destroy(GameObject); // needs to be notified
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
```cs
|
||||
// example of script needing a reference to another
|
||||
public class GameUI : MonoBehaviour {
|
||||
|
||||
Player player; //instance of referenced GameObject to be founf by its type
|
||||
|
||||
void Start(){
|
||||
GameObject playerObj = GameObject.Find("Player"); //reference to game object
|
||||
GameObject playerObj = GameObject.FindGameObjectWithTag("Tag"); //reference to game object
|
||||
player = playerObj.GetComponent<Player>(); // get script attached to the GameObject
|
||||
|
||||
player = FindObjectOfType<Player>(); // get reference to an object
|
||||
|
||||
// on event invocation all subscribet methods will be called
|
||||
player.OnPlayerDeath += GameOver; // subscribe method to event
|
||||
}
|
||||
|
||||
void Update() {
|
||||
DrawHealthBar(plyer.health); // call method passing data of player GameObject
|
||||
}
|
||||
|
||||
void DrawHealthbar(float playerHealth) {
|
||||
// implementation
|
||||
}
|
||||
|
||||
public void GameOver() {
|
||||
//game over screen
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Screen
|
||||
|
||||
### 2D Screen Measures
|
||||
|
||||
Aspect Ratio = `(screen_width [px]) / (screen_height [px])`
|
||||
Orhograpic Size `[world units]` = `(screen_height [world units] / 2)`
|
||||
Aspect Ratio * Orhograpic Size = `(screen_width [world units] / 2)`
|
||||
Screen Width `[world units]` = `(AspectRatio * OrhograpicSize * 2)`
|
||||
|
||||
```cs
|
||||
screenWidth = Camera.main.aspect * Camera.main.orthographicSize * 2;
|
||||
```
|
||||
|
||||
## Scriptable Objects
|
||||
|
||||
Class to store data in stand alone assets, used to keep data out of scripts.
|
||||
Can be used as a template.
|
||||
|
||||
```c#
|
||||
[CreateAssetMenu(menuName = "ScriptableObjectName")] //enable creation of scriptable object
|
||||
public class ScriptableObjectName : ScriptableObject {
|
||||
//data structure here
|
||||
}
|
||||
```
|
||||
|
||||
### Game Object Serialization
|
||||
|
||||
```c#
|
||||
[SeralizeField] type variable; //access game object from code
|
||||
```
|
||||
|
||||
### Game Object Data Access
|
||||
|
||||
```c#
|
||||
public type GetVariable(){
|
||||
return variable;
|
||||
}
|
||||
```
|
87
.NET/Unity/Vector, Tranfrorm, Space.md
Normal file
87
.NET/Unity/Vector, Tranfrorm, Space.md
Normal file
|
@ -0,0 +1,87 @@
|
|||
# Vector, Tranfrorm, Space
|
||||
|
||||
## Vector2, Vector3, Vector4
|
||||
|
||||
[Vector3 Docs](https://docs.unity3d.com/ScriptReference/Vector3.html)
|
||||
|
||||
Used to store positions, velocities and directions.
|
||||
|
||||
Magnitude = `sqrt(Math.pow(x, 2) + Math.pow(y, 2))`
|
||||
Direction = `(x / Magnitude, y / Magnitude)`
|
||||
|
||||
The direction is calculated by normalizing the vector to make it become a unit vector (*versor*).
|
||||
|
||||
```cs
|
||||
Vector3.x // x coord of vector
|
||||
Vector3.y // x coord of vector
|
||||
Vector3.z // x coord of vector
|
||||
|
||||
Vector3.magnitude
|
||||
Vector3.normalized
|
||||
|
||||
Vector3.up // Vector3(0, 1, 0)
|
||||
Vector3.down // Vector3(0, -1, 0)
|
||||
Vector3.left // Vector3(-1, 0, 0)
|
||||
Vector3.right // Vector3(1, 0, 0)
|
||||
Vector3.forward // Vector3(0, 0, 1)
|
||||
Vector3.back // Vector3(0, 0, -1)
|
||||
Vector3.one // Vector3(1, 1, 1)
|
||||
Vector3.zero // Vector3(0, 0, 0)
|
||||
Vector3.one // Vector3(1, 1, 1)
|
||||
```
|
||||
|
||||
### Operations
|
||||
|
||||
```cs
|
||||
Vector3(x, y, z) * n = Vecotr3(xn, yn, yz);
|
||||
Vector3(x, y, z) / n = Vecotr3(x / n, y / n, y / z);
|
||||
|
||||
Vector3(x1, y1, z1) + Vector3(x2, y2, z2) = Vector3(x1 + x2, y1 + y2, z1 + z2);
|
||||
Vector3(x1, y1, z1) - Vector3(x2, y2, z2) = Vector3(x1 - x2, y1 - y2, z1 - z2);
|
||||
|
||||
Quaternion.Euler(Vector3) // convert a Vector3 to a Quaternion
|
||||
```
|
||||
|
||||
### Movement
|
||||
|
||||
Speed = value m/s
|
||||
Velocity = Direction * Speed
|
||||
|
||||
MovementInFrame = Speed * timeSinceLastFrame
|
||||
|
||||
## Transform
|
||||
|
||||
[Transform Docs](https://docs.unity3d.com/ScriptReference/Transform.html)
|
||||
|
||||
```cs
|
||||
// propetries
|
||||
transfrom.position // Vector3 - global position
|
||||
transfrom.localPosition // Vector3 - local position
|
||||
transform.rotation // Quaternion - global rotation
|
||||
transfrom.parent // Transform - parent of the object
|
||||
|
||||
transform.localScale = Vector3; // set object dimensions
|
||||
|
||||
// methods
|
||||
transform.Rotate(Vector3 * Time.deltaTime * speed, Space); // set rotation using vectors in selected space (Space.Self or Space.World)
|
||||
transform.Translate(Vector3 * Time.deltaTime * speed, Space); // set movement in selected space
|
||||
```
|
||||
|
||||
### Local, GLobal & Object Spcace
|
||||
|
||||
**Local Space**: Applies transformation relative to the *local* coordinate system (`Space.Self`).
|
||||
**Global Space**: Applies transformation relative to the *world* coordinate system (`Space.World`)
|
||||
|
||||
### Parenting
|
||||
|
||||
Changing the parent will make position, scale and rotation of the child object retalive to the parent but keep the world space's position, rotation and scale the same.
|
||||
|
||||
Setting the parentele by script:
|
||||
|
||||
```cs
|
||||
public class ParentScript : MonoBehaviour {
|
||||
public Transform childTransform; // reference to the child object transfrom
|
||||
|
||||
childTrandform.parent = transfrom; // when evaluated at runtime sets current object as parent of another
|
||||
}
|
||||
```
|
35
.NET/Xamarin/App.xaml.cs.md
Normal file
35
.NET/Xamarin/App.xaml.cs.md
Normal file
|
@ -0,0 +1,35 @@
|
|||
# App.xaml.cs
|
||||
|
||||
This `App` class is defined as `public` and derives from the Xamarin.Forms `Application` class.
|
||||
The constructor has just one responsibility: to set the `MainPage` property of the `Application` class to an object of type `Page`.
|
||||
|
||||
```cs
|
||||
using System;
|
||||
using Xamarin.Forms;
|
||||
using Xamarin.Forms.Xaml;
|
||||
|
||||
namespace AppName
|
||||
{
|
||||
public partial class App : Application
|
||||
{
|
||||
public App()
|
||||
{
|
||||
InitializeComponent();
|
||||
|
||||
MainPage = new MainPage();
|
||||
}
|
||||
|
||||
protected override void OnStart()
|
||||
{
|
||||
}
|
||||
|
||||
protected override void OnSleep()
|
||||
{
|
||||
}
|
||||
|
||||
protected override void OnResume()
|
||||
{
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
51
.NET/Xamarin/App.xaml.md
Normal file
51
.NET/Xamarin/App.xaml.md
Normal file
|
@ -0,0 +1,51 @@
|
|||
# App.xaml
|
||||
|
||||
## Root Tag
|
||||
|
||||
The `<Application>` tag begins with two XML namespace declarations, both of which are URIs.
|
||||
|
||||
The default namespace belongs to **Xamarin**. This is the XML namespace (`xmlns`) for elements in the file with no prefix, such as the `ContentPage` tag.
|
||||
The URI includes the year that this namespace came into being and the word *forms* as an abbreviation for Xamarin.Forms.
|
||||
|
||||
The second namespace is associated with a prefix of `x` by convention, and it belongs to **Microsoft**. This namespace refers to elements and attributes that are intrinsic to XAML and are found in every XAML implementation.
|
||||
The word *winfx* refers to a name once used for the .NET Framework 3.0, which introduced WPF and XAML.
|
||||
|
||||
The `x:Class` attribute can appear only on the root element of a XAML file. It specifies the .NET namespace and name of a derived class. The base class of this derived class is the root element.
|
||||
|
||||
In other words, this `x:Class` specification indicates that the `App` class in the `AppName` namespace derives from `Application`.
|
||||
That’s exactly the same information as the `App` class definition in the `App.xaml.cs` file.
|
||||
|
||||
```xml
|
||||
<?xml version="1.0" encoding="utf-8" ?>
|
||||
<Application xmlns="http://xamarin.com/schemas/2014/forms"
|
||||
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
|
||||
x:Class="AppName.App">
|
||||
</Application>
|
||||
```
|
||||
|
||||
## Shared Resources
|
||||
|
||||
```xml
|
||||
<?xml version="1.0" encoding="utf-8" ?>
|
||||
<Application xmlns="http://xamarin.com/schemas/2014/forms"
|
||||
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
|
||||
x:Class="AppName.App">
|
||||
|
||||
<!-- collection of shared resources definiions -->
|
||||
<Application.Resources>
|
||||
|
||||
<!-- Application resource dictionary -->
|
||||
<ResourceDictionary>
|
||||
<!-- Key-Value Pair -->
|
||||
<Type x:Key="DictKey">value<Type>
|
||||
</ResourceDictionary>
|
||||
|
||||
<!-- define a reusable style -->
|
||||
<Style x:Key="Style Name" TargetType="Element Type">
|
||||
<!-- set poperties of the style -->
|
||||
<Setter Property="PropertyName" Value="PropertyValue">
|
||||
</Style>
|
||||
|
||||
</Application.Resources>
|
||||
</Application>
|
||||
```
|
22
.NET/Xamarin/Page.xaml.cs.md
Normal file
22
.NET/Xamarin/Page.xaml.cs.md
Normal file
|
@ -0,0 +1,22 @@
|
|||
# Page.xaml.cs
|
||||
|
||||
```cs
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.ComponentModel;
|
||||
using System.Linq;
|
||||
using System.Text;
|
||||
using System.Threading.Tasks;
|
||||
using Xamarin.Forms;
|
||||
|
||||
namespace AppName
|
||||
{
|
||||
public partial class PageName : ContentPage
|
||||
{
|
||||
public PageName()
|
||||
{
|
||||
InitializeComponent();
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
86
.NET/Xamarin/Page.xaml.md
Normal file
86
.NET/Xamarin/Page.xaml.md
Normal file
|
@ -0,0 +1,86 @@
|
|||
# Page.xaml
|
||||
|
||||
## Anatomy of an app
|
||||
|
||||
The modern user interface is constructed from visual objects of various sorts. Depending on the operating system, these visual objects might go by different names—controls, elements, views, widgets—but they are all devoted to the jobs of presentation or interaction or both.
|
||||
|
||||
In Xamarin.Forms, the objects that appear on the screen are collectively called *visual elements* (`VisualElement` class).
|
||||
|
||||
They come in three main categories:
|
||||
|
||||
- page (`Page` class)
|
||||
- layout (`Layout` class)
|
||||
- view (`View` class)
|
||||
|
||||
A Xamarin.Forms application consists of one or more pages. A page usually occupies all (or at least a large area) of the screen.
|
||||
Some applications consist of only a single page, while others allow navigating between multiple pages.
|
||||
|
||||
On each page, the visual elements are organized in a parent-child hierarchy.
|
||||
Some layouts have a single child, but many layouts have multiple children that the layout arranges within itself. These children can be other layouts or views.
|
||||
|
||||
The term *view* in Xamarin.Forms denotes familiar types of presentation and interactive objects.
|
||||
|
||||
## Pages
|
||||
|
||||
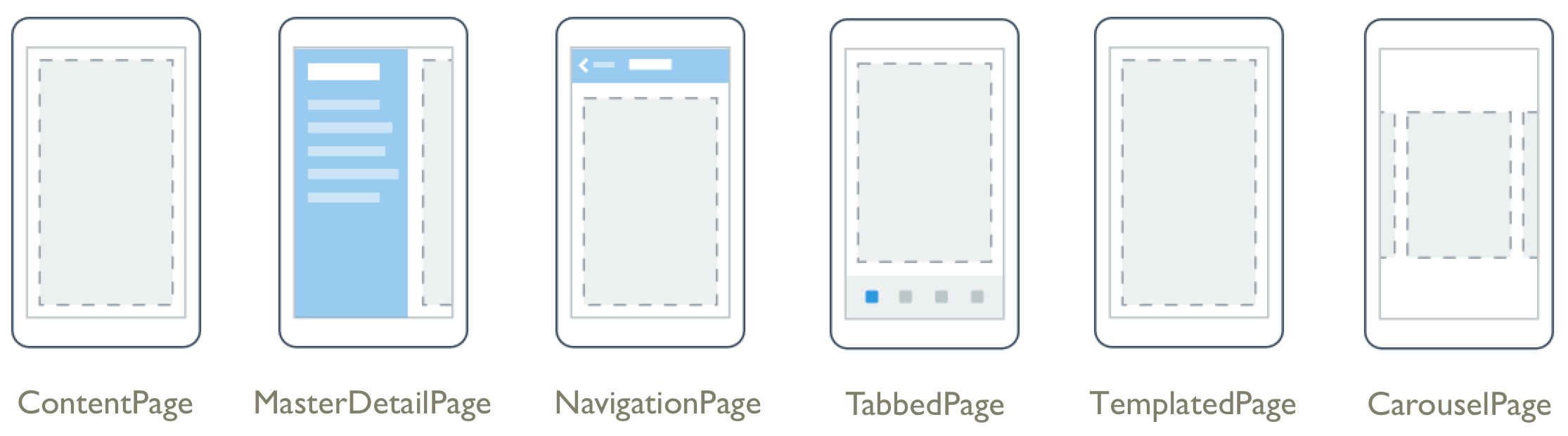
|
||||
|
||||
```xml
|
||||
<?xml version="1.0" encoding="utf-8" ?>
|
||||
|
||||
<!-- Sets the Content property to a single View -->
|
||||
<ContentPage></ContentPage>
|
||||
|
||||
<!-- Manages two panes of information (Master and Detail property) -->
|
||||
<MasterDetailPage></MasterDetailPage>
|
||||
|
||||
<!-- Manages navigation among other pages using a stack-based architecture -->
|
||||
<NavigationPage></NavigationPage>
|
||||
|
||||
<!-- Allows navigation among child pages using tabs -->
|
||||
<TabbedPage></TabbedPage>
|
||||
|
||||
<!-- Displays full-screen content with a control template -->
|
||||
<TemplatePage></TemplatePage>
|
||||
|
||||
<!-- Allows navigation among child pages through finger swiping -->
|
||||
<CarouselPage></CarouselPage>
|
||||
```
|
||||
|
||||
## Layouts
|
||||
|
||||
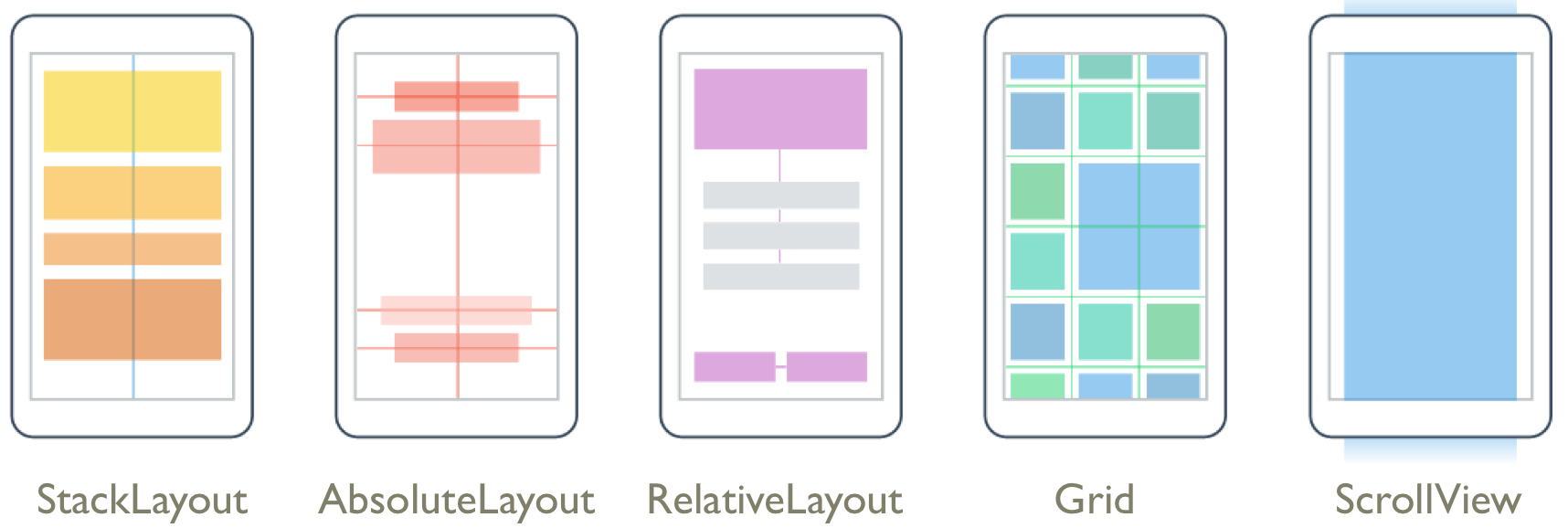
|
||||
|
||||
- StackLayout: Organizes views linearly, either horizontally or vertically.
|
||||
- AbsoluteLayout: Organizes views by setting coordinates & size in terms of absolute values or ratios.
|
||||
- RelativeLayout: Organizes views by setting constraints relative to their parent’s dimensions & position.
|
||||
- Grid: Organizes views in a grid of Rows and Columns
|
||||
- FlexLayout: Organizes views horizontally or vertically with wrapping.
|
||||
- ScrollView: Layout that's capable of scrolling its content.
|
||||
|
||||
### Grid Layout
|
||||
|
||||
```xml
|
||||
<!-- "<num>*" makes the dimesions proportional -->
|
||||
<Gird.RowDefinitions>
|
||||
<!-- insert a row in the layout -->
|
||||
<RowDefinition Height="2*"/>
|
||||
</Gird.RowDefinitions>
|
||||
|
||||
<Grid.ColumnDefinitions>
|
||||
<!-- insert column in layout -->
|
||||
<ColumnDefinition Width=".5*"/>
|
||||
</Grid.ColumnDefinitions>
|
||||
```
|
||||
|
||||
## [Views](https://docs.microsoft.com/en-us/xamarin/xamarin-forms/xaml/xaml-controls "XAML Views")
|
||||
|
||||
```xml
|
||||
<Image Source="" BackgroundColor="" [LayoutPosition]/>
|
||||
<!-- source contains reference to imagefile in Xamarin.[OS]/Resources/drawable -->
|
||||
|
||||
<!-- box to insert text -->
|
||||
<Editor Placeholder="placeholder text" [LayoutPosition]/>
|
||||
|
||||
<!-- clickable button -->
|
||||
<Button Text="button text" BackgroundColor="" Clicked="function_to_call" [LayoytPosition]/>
|
||||
```
|
35
.NET/Xamarin/ViewModel.cs.md
Normal file
35
.NET/Xamarin/ViewModel.cs.md
Normal file
|
@ -0,0 +1,35 @@
|
|||
# ViewModel.cs
|
||||
|
||||
```cs
|
||||
using System;
|
||||
using System.Collections.Generic;
|
||||
using System.Collections.ObjectModel;
|
||||
using System.ComponentModel;
|
||||
using System.Text;
|
||||
using Xamarin.Forms;
|
||||
|
||||
namespace AppName.ViewModels
|
||||
{
|
||||
class PageNameViewModel : INotifyPropertyChanged
|
||||
{
|
||||
public MainPageViewModel()
|
||||
{
|
||||
}
|
||||
|
||||
public event PropertyChangedEventHandler PropertyChanged; // event handler to notify changes
|
||||
|
||||
public string field; // needed, if substituted by ExprBody will cause infinite loop of access with the binding
|
||||
public string Property
|
||||
{
|
||||
get => field;
|
||||
set
|
||||
{
|
||||
field = value;
|
||||
|
||||
var args = new PropertyChangedEventArgs(nameof(Property)); // EVENT: let view know that the Property has changed
|
||||
PropertyChanged?.Invoke(this, args); // Ivoke event to notify the view
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
41
.NET/Xamarin/Xamarin.Essentials.md
Normal file
41
.NET/Xamarin/Xamarin.Essentials.md
Normal file
|
@ -0,0 +1,41 @@
|
|||
# Xamarin.Essentials
|
||||
|
||||
Android, iOS, and UWP offer unique operating system and platform APIs that developers have access to all in C# leveraging Xamarin.
|
||||
**Xamarin.Essentials** provides a *single cross-platform API* that works with any Xamarin.Forms, Android, iOS, or UWP application that can be accessed from shared code no matter how the user interface is created.
|
||||
|
||||
- [Accelerometer](https://docs.microsoft.com/en-us/xamarin/essentials/accelerometer): Retrieve acceleration data of the device in three dimensional space.
|
||||
- [App Information](https://docs.microsoft.com/en-us/xamarin/essentials/app-information): Find out information about the application.
|
||||
- [App Theme](https://docs.microsoft.com/en-us/xamarin/essentials/app-theme): Detect the current theme requested for the application.
|
||||
- [Barometer](https://docs.microsoft.com/en-us/xamarin/essentials/barometer): Monitor the barometer for pressure changes.
|
||||
- [Battery](https://docs.microsoft.com/en-us/xamarin/essentials/battery): Easily detect battery level, source, and state.
|
||||
- [Clipboard](https://docs.microsoft.com/en-us/xamarin/essentials/clipboard): Quickly and easily set or read text on the clipboard.
|
||||
- [Color Converters](https://docs.microsoft.com/en-us/xamarin/essentials/color-converters): Helper methods for System.Drawing.Color.
|
||||
- [Compass](https://docs.microsoft.com/en-us/xamarin/essentials/compass): Monitor compass for changes.
|
||||
- [Connectivity](https://docs.microsoft.com/en-us/xamarin/essentials/connectivity): Check connectivity state and detect changes.
|
||||
- [Detect Shake](https://docs.microsoft.com/en-us/xamarin/essentials/detect-shake): Detect a shake movement of the device.
|
||||
- [Device Display Information](https://docs.microsoft.com/en-us/xamarin/essentials/device-display): Get the device's screen metrics and orientation.
|
||||
- [Device Information](https://docs.microsoft.com/en-us/xamarin/essentials/device-information): Find out about the device with ease.
|
||||
- [Email](https://docs.microsoft.com/en-us/xamarin/essentials/email): Easily send email messages.
|
||||
- [File System Helpers](https://docs.microsoft.com/en-us/xamarin/essentials/file-system-helpers): Easily save files to app data.
|
||||
- [Flashlight](https://docs.microsoft.com/en-us/xamarin/essentials/flashlight): A simple way to turn the flashlight on/off.
|
||||
- [Geocoding](https://docs.microsoft.com/en-us/xamarin/essentials/geocoding): Geocode and reverse geocode addresses and coordinates.
|
||||
- [Geolocation](https://docs.microsoft.com/en-us/xamarin/essentials/geolocation): Retrieve the device's GPS location.
|
||||
- [Gyroscope](https://docs.microsoft.com/en-us/xamarin/essentials/gyroscope): Track rotation around the device's three primary axes.
|
||||
- [Launcher](https://docs.microsoft.com/en-us/xamarin/essentials/launcher): Enables an application to open a URI by the system.
|
||||
- [Magnetometer](https://docs.microsoft.com/en-us/xamarin/essentials/magnetometer): Detect device's orientation relative to Earth's magnetic field.
|
||||
- [MainThread](https://docs.microsoft.com/en-us/xamarin/essentials/main-thread): Run code on the application's main thread.
|
||||
- [Maps](https://docs.microsoft.com/en-us/xamarin/essentials/maps): Open the maps application to a specific location.
|
||||
- [Open Browser](https://docs.microsoft.com/en-us/xamarin/essentials/open-browser): Quickly and easily open a browser to a specific website.
|
||||
- [Orientation Sensor](https://docs.microsoft.com/en-us/xamarin/essentials/orientation-sensor): Retrieve the orientation of the device in three dimensional space.
|
||||
- [Permissions](https://docs.microsoft.com/en-us/xamarin/essentials/permissions): Check and request permissions from users.
|
||||
- [Phone Dialer](https://docs.microsoft.com/en-us/xamarin/essentials/phone-dialer): Open the phone dialer.
|
||||
- [Platform Extensions](https://docs.microsoft.com/en-us/xamarin/essentials/platform-extensions): Helper methods for converting Rect, Size, and Point.
|
||||
- [Preferences](https://docs.microsoft.com/en-us/xamarin/essentials/preferences): Quickly and easily add persistent preferences.
|
||||
- [Secure Storage](https://docs.microsoft.com/en-us/xamarin/essentials/secure-storage): Securely store data.
|
||||
- [Share](https://docs.microsoft.com/en-us/xamarin/essentials/share): Send text and website uris to other apps.
|
||||
- [SMS](https://docs.microsoft.com/en-us/xamarin/essentials/sms): Create an SMS message for sending.
|
||||
- [Text-to-Speech](https://docs.microsoft.com/en-us/xamarin/essentials/text-to-speech): Vocalize text on the device.
|
||||
- [Unit Converters](https://docs.microsoft.com/en-us/xamarin/essentials/unit-converters): Helper methods to convert units.
|
||||
- [Version Tracking](https://docs.microsoft.com/en-us/xamarin/essentials/version-tracking): Track the applications version and build numbers.
|
||||
- [Vibrate](https://docs.microsoft.com/en-us/xamarin/essentials/vibrate): Make the device vibrate.
|
||||
- [Web Authenticator](https://docs.microsoft.com/en-us/xamarin/essentials/web-authenticator): Start web authentication flows and listen for a callback.
|
437
Android/Activity.kt.md
Normal file
437
Android/Activity.kt.md
Normal file
|
@ -0,0 +1,437 @@
|
|||
# Android App Activity.kt
|
||||
|
||||
## Logging
|
||||
|
||||
```kotlin
|
||||
Log.i("tag", "logValue") //info log
|
||||
Log.d("tag", "logValue") //debug log
|
||||
Log.w("tag", "logValue") //warning log
|
||||
Log.e("tag", "logValue") //error log
|
||||
Log.c("tag", "logValue") //critical log
|
||||
```
|
||||
|
||||
## Activity Life Cycle
|
||||
|
||||

|
||||
|
||||
```kotlin
|
||||
package com.its.<appname>
|
||||
|
||||
import androidx.appcompat.app.AppCompatActivity
|
||||
import android.os.Bundle
|
||||
|
||||
class MainActivity : AppCompatActivity() {
|
||||
|
||||
//entry point of the activity
|
||||
override fun onCreate(savedInstanceState: Bundle?) {
|
||||
super.onCreate(savedInstanceState)
|
||||
setContentView(R.layout.<activity_xml>)
|
||||
}
|
||||
|
||||
override fun onStart() {
|
||||
}
|
||||
|
||||
override fun onResume() {
|
||||
}
|
||||
|
||||
override fun onPause() {
|
||||
}
|
||||
|
||||
override fun onStop() {
|
||||
}
|
||||
|
||||
override fun onRestart() {
|
||||
}
|
||||
|
||||
override fun onDestroy() {
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Passing data between activities
|
||||
|
||||
In *lauching* activity:
|
||||
|
||||
```kotlin
|
||||
private fun openActivity() {
|
||||
|
||||
//target to open Intent(opener, opened)
|
||||
val intent = Intent(this, Activity::class.java)
|
||||
|
||||
//pass data to lauched activity
|
||||
intent.putExtra("identifier", value)
|
||||
|
||||
startActivity(intent) //launch another activity
|
||||
}
|
||||
```
|
||||
|
||||
In *launched* activity:
|
||||
|
||||
```kotlin
|
||||
val identifier = intent.get<Type>Extra("identifier")
|
||||
```
|
||||
|
||||
## Hooks
|
||||
|
||||
### Resources Hooks
|
||||
|
||||
```kotlin
|
||||
R.<resourceType>.<resourceName> //access to reource
|
||||
|
||||
ContextCompat.getColor(this, colorResource) //extract color from resources
|
||||
getString(stringResource) //extract string from resources
|
||||
```
|
||||
|
||||
### XML hooks
|
||||
|
||||
Changes in xml made in kotlin are applied after the app is drawn thus overwriting instructions in xml.
|
||||
|
||||
In `activity.xml`:
|
||||
|
||||
```xml
|
||||
<View
|
||||
android:id="@+id/<id>"/>
|
||||
```
|
||||
|
||||
in `Activity.kt`:
|
||||
|
||||
```kotlin
|
||||
var element = findViewById(R.id.<id>) //old method
|
||||
|
||||
<id>.poperty = value //access and modify view contents
|
||||
```
|
||||
|
||||
## Activity Components
|
||||
|
||||
### Snackbar
|
||||
|
||||
Componed derived from material design. If using old API material design dependency must be set in gradle.
|
||||
|
||||
In `build.gradle (Module:app)`:
|
||||
|
||||
```gradle
|
||||
dependencies {
|
||||
implementation 'com.google.android.material:material:<sem_ver>'
|
||||
}
|
||||
```
|
||||
|
||||
In `Activity.kt`:
|
||||
|
||||
```kotlin
|
||||
import com.google.android.material.snackbar.Snackbar
|
||||
|
||||
Snackbar
|
||||
.make(activityID, message, Snackbar.TIME_ALIVE) //create snackbar
|
||||
.setAction("Button Name", { action }) //add button to snackbar
|
||||
.show()
|
||||
```
|
||||
|
||||
## Opening External Content
|
||||
|
||||
### Opening URLs
|
||||
|
||||
```kotlin
|
||||
val url = "https://www.google.com"
|
||||
val intent = Intent(Intent.ACTION_VIEW)
|
||||
|
||||
intent.setData(Uri.parse(url))
|
||||
startActivity(intent)
|
||||
```
|
||||
|
||||
### Sharing Content
|
||||
|
||||
```kotlin
|
||||
val intent = Intent(Intent.ACTION_SEND)
|
||||
|
||||
intent.setType("text/plain") //specifying shared content type
|
||||
intent.putExtra(Intent.EXTRA_MAIL, "mail@address") //open mail client and precompile field if share w/ mail
|
||||
intent.putExtra(Intent.EXTRA_SUBJECT, "subject")
|
||||
intent.putExtra(Intent.EXTRA_TEXT, "text") //necessary since type is text
|
||||
startActivity(Initent.startChooser(intent, "chooser title")) //let user choose the share app
|
||||
```
|
||||
|
||||
### App Google Maps
|
||||
|
||||
[Documentation](https://developers.google.com/maps/documentation/urls/android-intents)
|
||||
|
||||
```kotlin
|
||||
val uri = Uri.parse("geo: <coordinates>")
|
||||
val intent = Intent(Intent.ACTION_VIEW, uri)
|
||||
intent.setPackage("com.google.android.apps.maps") //app to be opened
|
||||
startActivity(intent)
|
||||
```
|
||||
|
||||
### Make a call (wait for user)
|
||||
|
||||
Preset phone number for the call, user needs to press call button to initiate dialing.
|
||||
|
||||
```kotlin
|
||||
fun call() {
|
||||
val intent = Intent(Intent.ACTION_DIAL)
|
||||
intent.setData(Uri.parse("tel: <phone number>"))
|
||||
startActivity(intent)
|
||||
}
|
||||
|
||||
```
|
||||
|
||||
### Make a call (directly)
|
||||
|
||||
In `AndroidManifest.xml`:
|
||||
|
||||
```xml
|
||||
<uses-permission android:name="android.permission.CALL_PHONE" />
|
||||
```
|
||||
|
||||
```kotlin
|
||||
//https://developer.android.com/training/permissions/requesting
|
||||
|
||||
//intercept OS response to permission popup
|
||||
override fun onRequestPermissionResult(requestCode: Int, permissins: Array<out String>, grantResults: IntArray) {
|
||||
super.onRequestPermissionsResult(requestCode, permissions, grantResults)
|
||||
}
|
||||
|
||||
fun checkCallPermission() {
|
||||
//check if permission to make a call has been granted
|
||||
if (ContextCompact.checkSelfPermission(context!!, android.Manifest.permission.CALL_PHONE) != PackageManager.PERMISSION_GRANTED) {
|
||||
// i permission has not been granted request it (opens OS popup, no listener aviable)
|
||||
ActivityCompat.requestPermissions(context!!, arrrayOf(android.Manifest.permission.CALL_PHONE), requestCode) //request code neeeds to be specific for the permission
|
||||
} else {
|
||||
call() //if permission has been already given
|
||||
}
|
||||
}
|
||||
|
||||
@SuppressLint("MissingPermission") // suppress warnig of unhandled permission (handled in checkCallPermission)
|
||||
fun call() {
|
||||
val intent = Intent(Intent.ACTION_DIAL, Uri.parse("tel: <phone number>"))
|
||||
startActivity(intent)
|
||||
}
|
||||
```
|
||||
|
||||
## Lists (with RecyclerView)
|
||||
|
||||
A [LayoutManager][1] is responsible for measuring and positioning item views within a `RecyclerView` as well as determining the policy for when to recycle item views that are no longer visible to the user.
|
||||
By changing the `LayoutManager` a `RecyclerView` can be used to implement a standard vertically *scrolling list*, a *uniform grid*, *staggered grids*, *horizontally scrolling collections* and more.
|
||||
Several stock layout managers are provided for general use.
|
||||
|
||||
[Adapters][2] provide a binding from an app-specific data set to views that are displayed within a `RecyclerView`.
|
||||
|
||||
[1]:https://developer.android.com/reference/kotlin/androidx/recyclerview/widget/RecyclerView.LayoutManager
|
||||
[2]:https://developer.android.com/reference/kotlin/androidx/recyclerview/widget/RecyclerView.Adapter
|
||||
|
||||
```kotlin
|
||||
var array: ArrayList<T>? = null //create ArrayList of elements to be displayed
|
||||
var adapter: RecyclerViewItemAdapter? = null //create adapter to draw the list in the Activity
|
||||
|
||||
array.add(item) //add item to ArrayList
|
||||
|
||||
// create LayoutManager for the recyclerView
|
||||
val layoutManager = <ViewGroup>LayoutManager(context, <ViewGroup>LayoutManager.VERTICAL, reverseLayout: Bool)
|
||||
recycleView.LayoutManager = layoutManager // assign LayoutManager for the recyclerView
|
||||
|
||||
// handle adapter var containing null value
|
||||
if (array != null) {
|
||||
adapter = RecyclerViewItemAdapter(array!!) // valorize adapter with a adaper object
|
||||
recyclerView.adapter = adapter // assing adapter to the recyclerView
|
||||
}
|
||||
|
||||
// add or remom item
|
||||
|
||||
// tell the adapter that something is changed
|
||||
adapter?.notifyDataSetChanged()
|
||||
```
|
||||
|
||||
## WebView
|
||||
|
||||
[WebView Docs](https://developerandroid.com/reference/android/webkit/WebView)
|
||||
|
||||
```kotlin
|
||||
webView.webViewClient = object : WebViewClient() {
|
||||
|
||||
// avoid openiing browsed by default, open webview instead
|
||||
override fun shouldOverrideUrlLoading(view: WebView?, url: String?): Boolean {
|
||||
view?.loadUrl(url) // handle every url
|
||||
return true
|
||||
}
|
||||
|
||||
override fun shouldOverrideUrlLoading(view: WebView?, url: String?): Boolean {
|
||||
|
||||
// stay in WebView until website changes
|
||||
if (Uri.parse(url).host == "www.website.domain") {
|
||||
return false
|
||||
}
|
||||
|
||||
Intent(Intent.ACTION_VIEW, Uri.parse(url).apply){
|
||||
startActivity(this) // open br br owser/app when the website changes to external URL
|
||||
}
|
||||
return true
|
||||
}
|
||||
|
||||
webView.settings.javaScriptEnabled = true // enable javascript
|
||||
webView.addJavaScriptInterface(webAppInterface(this, "Android")) // create bridge between js on website an app
|
||||
|
||||
// if in webView use android back butto to go to previous webpage (if possible)
|
||||
override fun onKeyDown(keyCode: Int, event: KeyEvent?) :Boolean {
|
||||
if (keyCode == KeyEvent.KEYCODE_BACK && webVew,canGoBack()) { // if previous url exists & back button pressed
|
||||
webView.goBack() // go to previous URL
|
||||
return true
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Web Requests (Using Volley)
|
||||
|
||||
[Volley Docs](https://developer.android.com/training/volley)
|
||||
|
||||
### Import & Permissions
|
||||
|
||||
Import in `build.gradle`:
|
||||
|
||||
```kotlin
|
||||
implementation 'com.android.volley:volley:1.1.1'
|
||||
```
|
||||
|
||||
Perrmissions in `AndroidManifest.xml`:
|
||||
|
||||
```xml
|
||||
<uses-permission android:name="android.permission.INTERNET" />
|
||||
```
|
||||
|
||||
### Make the request
|
||||
|
||||
Subsequnt requests sould be delayed to avoid allowing the user to make too frequent requests.
|
||||
|
||||
```kotlin
|
||||
private lateinit var queue: RequestQueue
|
||||
|
||||
override fun onCreate(savedInstanceState: Bundle?){
|
||||
queue = Volley.newRequestQueue(context)
|
||||
}
|
||||
|
||||
// response is a String
|
||||
private fun simpleRequest() {
|
||||
|
||||
var url = "www.website.domain"
|
||||
|
||||
var stringRequest = StringRequest(Request.Method.GET, url, Response.Listener<String> {
|
||||
Log.d()
|
||||
},
|
||||
Response.ErrorListener{
|
||||
Log.e()
|
||||
})
|
||||
|
||||
stringRequest.TAG = "TAG" // assign a tag to the request
|
||||
|
||||
queue.add(stringRequest) // add request to the queue
|
||||
}
|
||||
|
||||
// response is JSON Object
|
||||
private fun getJsonObjectRequest(){
|
||||
// GET -> jsonRequest = null
|
||||
// POST -> jsonRequest = JsonObject
|
||||
var stringRequest = JsonObjectRequest(Request.Method.GET, url, jsonRequest, Response.Listener<String> {
|
||||
Log.d()
|
||||
// read end use JSON
|
||||
},
|
||||
Response.ErrorListener{
|
||||
Log.e()
|
||||
})
|
||||
|
||||
queue.add(stringRequest) // add request to the queue
|
||||
}
|
||||
|
||||
// response is array of JSON objects
|
||||
private fun getJSONArrayRequest(){
|
||||
// GET -> jsonRequest = null
|
||||
// POST -> jsonRequest = JsonObject
|
||||
var stringRequest = JsonArrayRequest(Request.Method.GET, url, jsonRequest, Response.Listener<String> {
|
||||
Log.d()
|
||||
// read end use JSON
|
||||
},
|
||||
Response.ErrorListener{
|
||||
Log.e()
|
||||
})
|
||||
|
||||
queue.add(stringRequest) // add request to the queue
|
||||
}
|
||||
|
||||
|
||||
override fun onStop() {
|
||||
super.onStop()
|
||||
queue?.cancellAll("TAG") // delete all request with a particular tag when the activity is closed (avoid crash)
|
||||
}
|
||||
```
|
||||
|
||||
### Parse JSON Request
|
||||
|
||||
```kotlin
|
||||
Response.Litener { response ->
|
||||
var value = response.getSting("key")
|
||||
}
|
||||
```
|
||||
|
||||
## Data Persistance
|
||||
|
||||
### Singleton
|
||||
|
||||
Object instantiated during app init and is destroid only on app closing. It can be used for data persistance since is not affected by the destruction of an activity.
|
||||
|
||||
```kotlin
|
||||
// Context: Interface to global information about an application environment.
|
||||
class Singleton consturctor(context: Context) {
|
||||
|
||||
companion object {
|
||||
|
||||
@Volatile
|
||||
private var INSTANCE: Singleton? = null
|
||||
|
||||
// syncronized makes sure that all instances of the singleton are actually the only existing one
|
||||
fun getInstance(context: Contecxt) = INSTANCE ?: syncronized(this) {
|
||||
INSTANCE ?: Singleton(context).also {
|
||||
INSTANCE = it
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### SharedPreferences
|
||||
|
||||
[SharedPreferences Docs](https://developer.android.com/training/data-storage/shared-preferences)
|
||||
|
||||
**Get a handle to shared preferences**:
|
||||
|
||||
- `getSharedPreferences()` — Use this if you need multiple shared preference files identified by name, which you specify with the first parameter. You can call this from any Context in your app.
|
||||
- `getPreferences()` — Use this from an Activity if you need to use only one shared preference file for the activity. Because this retrieves a default shared preference file that belongs to the activity, you don't need to supply a name.
|
||||
|
||||
```kotlin
|
||||
val sharedPref = activity?.getSharedPreferences( getString(R.string.preference_file_key), Context.MODE_PRIVATE )
|
||||
val sharedPref = activity?.getPreferences(Context.MODE_PRIVATE)
|
||||
```
|
||||
|
||||
**Write to shared preferences**:
|
||||
|
||||
To write to a shared preferences file, create a `SharedPreferences.Editor` by calling `edit()` on your SharedPreferences.
|
||||
|
||||
Pass the keys and values to write with methods such as `putInt()` and `putString()`. Then call `apply()` or `commit()` to save the changes.
|
||||
|
||||
```kotlin
|
||||
val sharedPref = activity?.getPreferences(Context.MODE_PRIVATE) ?: return
|
||||
with (sharedPref.edit()) {
|
||||
putInt(getString(R.string.key), value)
|
||||
commit() // or apply()
|
||||
}
|
||||
```
|
||||
|
||||
`apply()` changes the in-memory `SharedPreferences` object immediately but writes the updates to disk *asynchronously*.
|
||||
Alternatively, use `commit()` to write the data to disk *synchronously*. But because *commit()* is synchronous, avoid calling it from your main thread because it could pause the UI rendering.
|
||||
|
||||
**Read from shared preferences**:
|
||||
|
||||
To retrieve values from a shared preferences file, call methods such as getInt() and getString(), providing the key for the wanted value, and optionally a default value to return if the key isn't present.
|
||||
|
||||
```kotlin
|
||||
val sharedPref = activity?.getPreferences(Context.MODE_PRIVATE) ?: return
|
||||
val defaultValue = resources.getInteger(R.integer.default_value_key)
|
||||
val value = sharedPref.getInt(getString(R.string.key), defaultValue)
|
||||
```
|
86
Android/Adapter.kt.md
Normal file
86
Android/Adapter.kt.md
Normal file
|
@ -0,0 +1,86 @@
|
|||
# RecycleView Cell Adapter
|
||||
|
||||
[Adapters][1] provide a binding from an app-specific data set to views that are displayed within a `RecyclerView`.
|
||||
|
||||
[1]:https://developer.android.com/reference/kotlin/androidx/recyclerview/widget/RecyclerView.Adapter
|
||||
|
||||
```kotlin
|
||||
package <package>
|
||||
|
||||
import android.content.Context
|
||||
import android.util.Log
|
||||
import android.view.LayoutInflater
|
||||
import android.view.ViewGroup
|
||||
import android.widget.Button
|
||||
import android.widget.TextView
|
||||
import androidx.recyclerview.widget.RecyclerView
|
||||
|
||||
class RecipeAdapter : RecyclerView.Adapter<RecipeAdapter.ViewHolder> {
|
||||
|
||||
private var mDataset: ArrayList<Recipe> // RecyclerView data
|
||||
|
||||
class ViewHolder : RecyclerView.ViewHolder {
|
||||
var viewGroup: ViewGroup? = null
|
||||
constructor(v: ViewGroup?) : super(v!!) {
|
||||
viewGroup = v
|
||||
}
|
||||
}
|
||||
|
||||
//adapter contructor, takes list of data
|
||||
constructor(myDataset: ArrayList<Recipe>/*, mContext: Context?*/){
|
||||
mDataset = myDataset
|
||||
//mContenxt = mContext
|
||||
}
|
||||
|
||||
|
||||
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
|
||||
val v : ViewGroup = LayoutInflater.from(parent.context).inflate(R.layout.view_recipe_item, parent, false) as ViewGroup
|
||||
|
||||
val vh = ViewHolder(v)
|
||||
return vh
|
||||
}
|
||||
|
||||
override fun getItemCount(): Int {
|
||||
return mDataset.size
|
||||
}
|
||||
|
||||
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
|
||||
|
||||
val mItem : Recipe = mDataset.get(position)
|
||||
|
||||
val titleText = holder.viewGroup?.findViewById<TextView>(R.id.titleText)
|
||||
titleText?.text = mItem.title
|
||||
|
||||
Log.d("Adapter","Title: "+mItem.title)
|
||||
|
||||
val deleteButton = holder.viewGroup!!.findViewById<Button>(R.id.deleteButton)
|
||||
deleteButton.setOnClickListener { removeItem(position) }
|
||||
|
||||
//Click
|
||||
holder.viewGroup?.setOnClickListener {
|
||||
mListener?.select(position)
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
|
||||
private fun removeItem(position: Int) {
|
||||
mDataset.removeAt(position)
|
||||
notifyItemRemoved(position)
|
||||
notifyItemRangeChanged(position, mDataset.size)
|
||||
}
|
||||
|
||||
/*
|
||||
* Callback
|
||||
* */
|
||||
private var mListener: AdapterCallback? = null
|
||||
|
||||
interface AdapterCallback {
|
||||
fun select(position: Int)
|
||||
}
|
||||
|
||||
fun setOnCallback(mItemClickListener: AdapterCallback) {
|
||||
this.mListener = mItemClickListener
|
||||
}
|
||||
}
|
||||
```
|
9
Android/Firebase.md
Normal file
9
Android/Firebase.md
Normal file
|
@ -0,0 +1,9 @@
|
|||
# [Firebase](https://firebase.google.com/) notes
|
||||
|
||||
> A comprehensive app development platform
|
||||
|
||||
[Firebase Docs](https://firebase.google.com/docs)
|
||||
|
||||
**Warning**: Google Analitycs can cause conflicts with the GDPR if user data is used in the app.
|
||||
|
||||
To link Firebase with the app it's necessary to download `google-services.json`.
|
154
Android/Fragment.md
Normal file
154
Android/Fragment.md
Normal file
|
@ -0,0 +1,154 @@
|
|||
# Fragments & FragmentActivity
|
||||
|
||||
[Documentation](https://developer.android.com/guide/components/fragments)
|
||||
|
||||
A **Fragment** represents a behavior or a portion of user interface in a `FragmentActivity`. It's possible to combine multiple fragments in a single activity to build a multi-pane UI and reuse a fragment in multiple activities.
|
||||
Think of a fragment as a *modular section of an activity*, which has its *own* lifecycle, receives its *own* input events, and which you can add or remove while the activity is running (sort of like a "sub activity" that you can reuse in different activities).
|
||||
|
||||

|
||||
|
||||
A fragment must always be hosted in an activity and the fragment's lifecycle is *directly affected* by the host activity's lifecycle.
|
||||
|
||||

|
||||
|
||||
## Minimal Fragment Functions
|
||||
|
||||
Usually, you should implement at least the following lifecycle methods:
|
||||
|
||||
`onCreate()`
|
||||
The system calls this when creating the fragment. Within your implementation, you should initialize essential components of the fragment that you want to retain when the fragment is paused or stopped, then resumed.
|
||||
|
||||
`onCreateView()`
|
||||
The system calls this when it's time for the fragment to draw its user interface for the first time. To draw a UI for your fragment, you must return a View from this method that is the root of your fragment's layout. You can return null if the fragment does not provide a UI.
|
||||
|
||||
`onPause()`
|
||||
The system calls this method as the first indication that the user is leaving the fragment (though it doesn't always mean the fragment is being destroyed). This is usually where you should commit any changes that should be persisted beyond the current user session (because the user might not come back).
|
||||
|
||||
## Fragment Subclasses
|
||||
|
||||
`DialogFragment`
|
||||
Displays a floating dialog. Using this class to create a dialog is a good alternative to using the dialog helper methods in the Activity class, because you can incorporate a fragment dialog into the back stack of fragments managed by the activity, allowing the user to return to a dismissed fragment.
|
||||
|
||||
`ListFragment`
|
||||
Displays a list of items that are managed by an adapter (such as a SimpleCursorAdapter), similar to ListActivity. It provides several methods for managing a list view, such as the onListItemClick() callback to handle click events. (Note that the preferred method for displaying a list is to use RecyclerView instead of ListView. In this case you would need to create a fragment that includes a RecyclerView in its layout. See Create a List with RecyclerView to learn how.)
|
||||
|
||||
`PreferenceFragmentCompat`
|
||||
Displays a hierarchy of Preference objects as a list. This is used to create a settings screen for your application.
|
||||
|
||||
## Fragment Insertion in Layout (Method 1)
|
||||
|
||||
In `Activity.xml`:
|
||||
|
||||
```xml
|
||||
<!-- Activity.xml boilerplate -->
|
||||
|
||||
<!-- Fragment drawn at Activity start cannot be drawn on event -->
|
||||
<fragment
|
||||
android:name="com.<app>.<Fragment>"
|
||||
android:layout_width="match_parent"
|
||||
android:layout_height="match_parent"
|
||||
android:id="@+id/fragment_id" />
|
||||
|
||||
<!-- Activity.xml boilerplate -->
|
||||
```
|
||||
|
||||
In `Fragment.kt`:
|
||||
|
||||
```kotlin
|
||||
package <package>
|
||||
|
||||
import android.os.Bundle
|
||||
import androidx.fragment.app.Fragment
|
||||
import android.view.LayoutInflater
|
||||
import android.view.View
|
||||
import android.view.ViewGroup
|
||||
|
||||
/**
|
||||
* A simple [Fragment] subclass.
|
||||
*/
|
||||
class FirstFragment : Fragment() {
|
||||
|
||||
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle? ): View? {
|
||||
// Inflate the layout for this fragment (draw and insert fragment in Activity)
|
||||
return inflater.inflate(R.layout.<fragment_xml>, container, false)
|
||||
}
|
||||
|
||||
//called after fragment is drawn (ACTIONS GO HERE)
|
||||
override fun onViewCreated(view: View, savedInstanceState: Bundle?) {
|
||||
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Fragment Insertion in Layout (Method 2)
|
||||
|
||||
```xml
|
||||
<!-- Activity.xml boilerplate -->
|
||||
<layout
|
||||
android:id="@+id/containerID"
|
||||
android:layout_width="match_parent"
|
||||
android:layout_height="match_parent" />
|
||||
|
||||
<!-- Activity.xml boilerplate -->
|
||||
```
|
||||
|
||||
In `Activity.kt`:
|
||||
|
||||
```kotlin
|
||||
override fun onCreate(savedInstanceState: Bundle?) {
|
||||
super.onCreate(savedInstanceState)
|
||||
setContentView(R.layout.<activity_xml>)
|
||||
|
||||
val fragmentID = FragmentClass()
|
||||
|
||||
//insert fragment from kotlin (can be triggered by event)
|
||||
supportFragmentManager
|
||||
.beginTransaction()
|
||||
.add(R.id.<containerID>, fragmentID)
|
||||
.commit()
|
||||
|
||||
//replace fragment from kotlin (can be triggered by event)
|
||||
supportFragmentManager
|
||||
.beginTransaction()
|
||||
.replace(R.id.<containerID>, fragmentID)
|
||||
.addToBackStack(null) // remember order of loaded fragment (keep alive previous fragments)
|
||||
.commit()
|
||||
}
|
||||
```
|
||||
|
||||
## Passing Values from Activity to Fragment
|
||||
|
||||
In `Activity.kt`:
|
||||
|
||||
```kotlin
|
||||
override fun onCreate(savedInstanceState: Bundle?) {
|
||||
super.onCreate(savedInstanceState)
|
||||
setContentView(R.layout.<activity_xml>)
|
||||
|
||||
val fragmentID = FragmentClass()
|
||||
|
||||
val bundle = Bundle()
|
||||
bundle.putString("key", "value") //passed value
|
||||
fragmentID.arguments = bundle
|
||||
|
||||
//insert fragment from kotlin (can be triggered by event)
|
||||
supportFragmentManager
|
||||
.beginTransaction()
|
||||
.add(R.id.<containerID>, fragmentID)
|
||||
.commit()
|
||||
}
|
||||
```
|
||||
|
||||
In `Fragment.kt`:
|
||||
|
||||
```kotlin
|
||||
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle? ): View? {
|
||||
|
||||
value = arguments?.getString("key") //read passed value
|
||||
|
||||
// Inflate the layout for this fragment (draw and insert fragment in Activity)
|
||||
return inflater.inflate(R.layout.<fragment_xml>, container, false)
|
||||
}
|
||||
```
|
||||
|
||||
.png?width=640&name=The%20activity%20and%20fragment%20lifecycles%20(1).png)
|
35
Android/Gradle & Building.md
Normal file
35
Android/Gradle & Building.md
Normal file
|
@ -0,0 +1,35 @@
|
|||
# Gradle & Building
|
||||
|
||||
## `build.gradle` (Module)
|
||||
|
||||
```gradle
|
||||
android {
|
||||
|
||||
defaultconfig{
|
||||
|
||||
applicationId <string> // app package name
|
||||
|
||||
minSdkVersion <int> // min API version
|
||||
targetSdkVersion <int> // actual API version
|
||||
|
||||
versionCode <int> // unique code for each release
|
||||
versionName "<string>"
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Distribuiting the app
|
||||
|
||||
### APK vs Bundle
|
||||
|
||||
- APK: android executable, contains all assets of the app (multiplr versions for each device). The user downloads all the assets.
|
||||
- Bundle: split the assets based on dedvice specs. The users donwloads only the assest needed for the target device.
|
||||
|
||||
### APK generation & [App Signing](https://developer.android.com/studio/publish/app-signing)
|
||||
|
||||
- **Key store path**: location where the keystore file will be stored.
|
||||
- **Key store password**: secure password for the keystore (**to be rememberd**).
|
||||
- **Key alias**: identifying name for the key (**to be rememberd**).
|
||||
- **Key password**: secure password for the key (**to be rememberd**).
|
||||
|
||||
The ketstore identifies the app and it's developers. It's needed for APK generation and releases distribution.
|
84
Android/MapsActivity.md
Normal file
84
Android/MapsActivity.md
Normal file
|
@ -0,0 +1,84 @@
|
|||
# Maps Activity
|
||||
|
||||
[Google Maps Docs](https://developers.google.com/maps/documentation/android-sdk/intro)
|
||||
|
||||
Activity sould be **Google Maps Activity**.
|
||||
|
||||
In `google_maps_api.xml`:
|
||||
|
||||
```xml
|
||||
<resources>
|
||||
<string name="google_maps_key" templateMergeStartegy="preserve", translateble="false">API_KEY</string>
|
||||
</resources>
|
||||
```
|
||||
|
||||
## Activity Layout (xml)
|
||||
|
||||
```xml
|
||||
<!-- a fragment to contain the map -->
|
||||
<fragment xmlns:android="http://schemas.android.com/apk/res/android"
|
||||
xmlns:map="http://schemas.android.com/apk/res-auto"
|
||||
xmlns:tools="http://schemas.android.com/tools"
|
||||
android:id="@+id/<fragment_id>"
|
||||
android:name="com.google.android.gms.maps.SupportMapFragment"
|
||||
android:layout_width="match_parent"
|
||||
android:layout_height="match_parent"
|
||||
tools:context=".<Activity>" />
|
||||
```
|
||||
|
||||
## Activity Class (kotlin)
|
||||
|
||||
```kotlin
|
||||
import androidx.appcompat.app.AppCompatActivity
|
||||
import android.os.Bundle
|
||||
|
||||
import com.google.android.gms.maps.CameraUpdateFactory
|
||||
import com.google.android.gms.maps.GoogleMap
|
||||
import com.google.android.gms.maps.OnMapReadyCallback
|
||||
import com.google.android.gms.maps.SupportMapFragment
|
||||
import com.google.android.gms.maps.model.LatLng
|
||||
import com.google.android.gms.maps.model.Marker
|
||||
import com.google.android.gms.maps.model.MarkerOptions
|
||||
|
||||
class MapsActivity : AppCompatActivity(), OnMapReadyCallback,
|
||||
GoogleMap.OnInfoWindowClickListener,
|
||||
GoogleMap.OnMarkerClickListener,
|
||||
GoogleMap.OnCameraIdleListener{
|
||||
|
||||
private lateinit var mMap: GoogleMap // declare but not valorize
|
||||
|
||||
override fun onCreate(savedInstanceState: Bundle?) {
|
||||
super.onCreate(savedInstanceState)
|
||||
setContentView(R.layout.<activity_xml>)
|
||||
|
||||
// add the map to a fragment
|
||||
val mapFragment = supportFragmentManager.findFragmentById(R.id.<fragment_id>) as SupportMapFragment
|
||||
mapFragment.getMapAsync(this)
|
||||
|
||||
}
|
||||
|
||||
override fun onMapReady(googleMap: GoogleMap) {
|
||||
mMap = googleMap
|
||||
mMap.setOnMarkerClickListener(this)
|
||||
mMap.setOnInfoWindowClickListener(this)
|
||||
|
||||
// Add a marker and move the camera
|
||||
val location = LatLng(-34.0, 151.0) // set loaction with latitude and longitude
|
||||
mMap.addMarker(MarkerOptions().position(location).title("Marker in ...")) // ad the marker to the map with a name and position
|
||||
|
||||
mMap.moveCamera(CameraUpdateFactory.newLatLng(location)) // move camera to the marker
|
||||
}
|
||||
|
||||
override fun onInfoWindowClick(p0: Marker?) {
|
||||
|
||||
}
|
||||
|
||||
override fun onMarkerClick(p0: Marker?): Boolean {
|
||||
|
||||
}
|
||||
|
||||
override fun onCameraIdle() {
|
||||
|
||||
}
|
||||
}
|
||||
```
|
101
Android/SQLite.md
Normal file
101
Android/SQLite.md
Normal file
|
@ -0,0 +1,101 @@
|
|||
# SQLite in Android
|
||||
|
||||
[Kotlin SQLite Documentation](https://developer.android.com/training/data-storage/sqlite)
|
||||
[NoSQL DB Realm Docs](https://realm.io/docs)
|
||||
|
||||
## Database Helper
|
||||
|
||||
Create database in memory and its tables.
|
||||
|
||||
```kotlin
|
||||
class DatabaseHelper(
|
||||
context: Context?,
|
||||
name: String?,
|
||||
factory: SQLiteDatabase.CursorFactory?,
|
||||
version: Int
|
||||
) : SQLiteOpenHelper(context, name, factory, version) { // super constructor?
|
||||
|
||||
// called on db creation if it does not exists (app installation)
|
||||
override fun onCreate() {
|
||||
sqLiteDatabase?.execSQL("CREATE TABLE ...") // create table
|
||||
}
|
||||
|
||||
override fun onUpgrade(sqLiteDatabase: SQLiteDatabase?, ...) {
|
||||
// table update logic
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## AppSingleton
|
||||
|
||||
Make data persistent.
|
||||
|
||||
```kotlin
|
||||
class AppSingleton consturctor(context: Context) {
|
||||
|
||||
var dataabse: SQLiteDatabase? = null // point to database file in memory
|
||||
|
||||
companion object {
|
||||
|
||||
@Volatile
|
||||
private var INSTANCE: AppSingleton? = null
|
||||
|
||||
// syncronized makes sure that all instances of the singleton are actually the only existing one
|
||||
fun getInstance(context: Contecxt) = INSTANCE ?: syncronized(this) {
|
||||
INSTANCE ?: Singleton(context).also {
|
||||
INSTANCE = it
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
// called to create DB in device memory
|
||||
fun openDatabase(context: Context?) {
|
||||
var databaseHelper = DatabaseHelper(context, "AppDB_Name.sqlite", null, version) {
|
||||
database = databaseHelper.writeDatabase //?
|
||||
}
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Activity
|
||||
|
||||
```kotlin
|
||||
override fun onCreate() {
|
||||
|
||||
// either creates or reads DB
|
||||
AppSingleton.getInstance().openDatabase(this) // context is this
|
||||
|
||||
val controller = ModelController()
|
||||
controller.insert(model) // insert data from object
|
||||
}
|
||||
```
|
||||
|
||||
## ModelController
|
||||
|
||||
Controller to handle data from the objects and interact with the Database
|
||||
|
||||
```kotlin
|
||||
sqLiteDatabse = AppISngleton.getInstance().database // reference to the database from the singleton
|
||||
|
||||
contentValues = ContentValues() // dict like structure to insert data in DB
|
||||
contentValuest.put("DB_Column", value) // put data in the structure
|
||||
|
||||
// insertion query V1
|
||||
sqLiteDatabase?.insert("table", null, contentValue)
|
||||
|
||||
//insertion query V2
|
||||
sqLiteDatabase?.rawQuey("INSERT INTO ...")
|
||||
|
||||
// returns a Cursor()
|
||||
val cursor = sqLiteDatabase?.query("table", ArrayOf(columns_to_read), "WHERE ...", "GROUP BY ...", "HAVING ...", "ORDER BY ...")
|
||||
|
||||
try {
|
||||
if (cursor != null) {
|
||||
while(cursor.moveToNext()) { // loop through cursor data
|
||||
data = cursor.getString(cursor.getColumnIndex("DB_Column")) // read data from the cursor
|
||||
}
|
||||
}
|
||||
} finally {
|
||||
cursor?.close()
|
||||
}
|
||||
```
|
215
Android/activity.xml.md
Normal file
215
Android/activity.xml.md
Normal file
|
@ -0,0 +1,215 @@
|
|||
# Activity.xml
|
||||
|
||||
## Resources
|
||||
|
||||
### Colors, Style, Strings
|
||||
|
||||
These resorsces are located in `app/src/main/res/values/<resource_type>.xml`
|
||||
`@color/colorName` -> access to *color definition* in `colors.xml`
|
||||
`@string/stringName` -> access to *string definition* in `strings.xml` (useful for localization)
|
||||
`@style/styleName` -> access to *style definition* in `styles.xml`
|
||||
|
||||
In `colors.xml`:
|
||||
|
||||
```xml
|
||||
<?xml version="1.0" encoding="utf-8"?>
|
||||
<resources>
|
||||
<!-- app bar color -->
|
||||
<color name="colorPrimary">#6200EE</color>
|
||||
<!-- status bar color -->
|
||||
<color name="colorPrimaryDark">#3700B3</color>
|
||||
|
||||
<color name="colorAccent">#03DAC5</color>
|
||||
|
||||
<!-- other color definitions -->
|
||||
</resources>
|
||||
```
|
||||
|
||||
In `strings.xml`:
|
||||
|
||||
```xml
|
||||
<resources>
|
||||
<string name="app_name"> AppName </string>
|
||||
|
||||
<!-- other strings definitions -->
|
||||
</resources>
|
||||
```
|
||||
|
||||
In `styles.xml`:
|
||||
|
||||
```xml
|
||||
<resources>
|
||||
|
||||
<!-- Base application theme. -->
|
||||
<style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar">
|
||||
<!-- Customize your theme here. -->
|
||||
<item name="colorPrimary">@color/colorPrimary</item>
|
||||
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
|
||||
<item name="colorAccent">@color/colorAccent</item>
|
||||
</style>
|
||||
|
||||
</resources>
|
||||
```
|
||||
|
||||
## View & View Group
|
||||
|
||||
A **View** contains a specific element of the layout.
|
||||
A **View Group** or **Layout** is a container of Views.
|
||||
|
||||
```xml
|
||||
<View settings/>
|
||||
|
||||
<ViewGroup settings>
|
||||
...
|
||||
</ViewGroup>
|
||||
|
||||
```
|
||||
|
||||
`android:layout_width`, `android:layout_heigth`:
|
||||
|
||||
* fixed value (dp, sp)
|
||||
* match_parent
|
||||
* wrap_content
|
||||
|
||||
## ViewGroups
|
||||
|
||||
### [RelativeLayout](https://developer.android.com/reference/android/widget/RelativeLayout.LayoutParams)
|
||||
|
||||
The relative layout containing the view uses the value of these layout parameters to determine where to position the view on the screen.
|
||||
If the view is not contained within a relative layout, these attributes are ignored.
|
||||
|
||||
```xml
|
||||
<RelativeLayout
|
||||
android:layout_width="match_parent"
|
||||
android:layout_height="match_parent">
|
||||
|
||||
</RelativeLayout>
|
||||
```
|
||||
|
||||
`android:layout_above`: Positions the bottom edge of this view above the given anchor view ID.
|
||||
`android:layout_alignBaseline`: Positions the baseline of this view on the baseline of the given anchor view ID.
|
||||
`android:layout_alignBottom`: Makes the bottom edge of this view match the bottom edge of the given anchor view ID.
|
||||
`android:layout_alignEnd`: Makes the end edge of this view match the end edge of the given anchor view ID.
|
||||
`android:layout_alignLeft`: Makes the left edge of this view match the left edge of the given anchor view ID.
|
||||
`android:layout_alignParentBottom`: If true, makes the bottom edge of this view match the bottom edge of the parent.
|
||||
`android:layout_alignParentEnd`: If true, makes the end edge of this view match the end edge of the parent.
|
||||
`android:layout_alignParentLeft`: If true, makes the left edge of this view match the left edge of the parent.
|
||||
`android:layout_alignParentRight`: If true, makes the right edge of this view match the right edge of the parent.
|
||||
`android:layout_alignParentStart`: If true, makes the start edge of this view match the start edge of the parent.
|
||||
`android:layout_alignParentTop`: If true, makes the top edge of this view match the top edge of the parent.
|
||||
`android:layout_alignRight`: Makes the right edge of this view match the right edge of the given anchor view ID.
|
||||
`android:layout_alignStart`: Makes the start edge of this view match the start edge of the given anchor view ID.
|
||||
`android:layout_alignTop`: Makes the top edge of this view match the top edge of the given anchor view ID.
|
||||
`android:layout_alignWithParentIfMissing`: If set to true, the parent will be used as the anchor when the anchor cannot be be found for layout_toLeftOf, layout_toRightOf, etc.
|
||||
`android:layout_below`: Positions the top edge of this view below the given anchor view ID.
|
||||
`android:layout_centerHorizontal`: If true, centers this child horizontally within its parent.
|
||||
`android:layout_centerInParent`: If true, centers this child horizontally and vertically within its parent.
|
||||
`android:layout_centerVertical`: If true, centers this child vertically within its parent.
|
||||
`android:layout_toEndOf`: Positions the start edge of this view to the end of the given anchor view ID.
|
||||
`android:layout_toLeftOf`: Positions the right edge of this view to the left of the given anchor view ID.
|
||||
`android:layout_toRightOf`: Positions the left edge of this view to the right of the given anchor view ID.
|
||||
`android:layout_toStartOf`: Positions the end edge of this view to the start of the given anchor view ID.
|
||||
|
||||
### [LinearLayout](https://developer.android.com/reference/android/widget/LinearLayout.LayoutParams)
|
||||
|
||||
Layout that arranges other views either horizontally in a single column or vertically in a single row.
|
||||
|
||||
```xml
|
||||
<LinearLayout
|
||||
android:layout_width="match_parent"
|
||||
android:layout_height="match_parent"
|
||||
android:orientation="vertical"> <!-- or horizontal -->
|
||||
|
||||
</LinearLayout>
|
||||
```
|
||||
|
||||
`android:layout_weight`: Indicates how much of the extra space in the LinearLayout is allocated to the view associated with these LayoutParams.
|
||||
`android:layout_margin`: Specifies extra space on the left, top, right and bottom sides of this view.
|
||||
`android:layout_marginBottom`: Specifies extra space on the bottom side of this view.
|
||||
`android:layout_marginEnd`: Specifies extra space on the end side of this view.
|
||||
`android:layout_marginHorizontal`: Specifies extra space on the left and right sides of this view.
|
||||
`android:layout_marginLeft`: Specifies extra space on the left side of this view.
|
||||
`android:layout_marginRight`: Specifies extra space on the right side of this view.
|
||||
`android:layout_marginStart`: Specifies extra space on the start side of this view.
|
||||
`android:layout_marginTop`: Specifies extra space on the top side of this view.
|
||||
`android:layout_marginVertical`: Specifies extra space on the top and bottom sides of this view.
|
||||
`android:layout_height`: Specifies the basic height of the view.
|
||||
`android:layout_width`: Specifies the basic width of the view.
|
||||
|
||||
## Views
|
||||
|
||||
```xml
|
||||
<!--ID is necessary for identification -->
|
||||
<View
|
||||
android:id="@+id/uniquieId"
|
||||
android:layout_width="value"
|
||||
android:layout_height="value"
|
||||
/>
|
||||
```
|
||||
|
||||
### TextView
|
||||
|
||||
To add `...` to truncate a string:
|
||||
|
||||
```xml
|
||||
<!-- maxLines + ellipsize works only in API 22+ -->
|
||||
<TextView
|
||||
maxLines="n"
|
||||
ellipsize="end" />
|
||||
```
|
||||
|
||||
### ScrollView
|
||||
|
||||
The scroll view can only have one child. If the child is a layout than the layout can contain many elements.
|
||||
|
||||
```xml
|
||||
<ScrollView
|
||||
android:id="@+id/uniquieId"
|
||||
android:layout_width="value"
|
||||
android:layout_height="value">
|
||||
<!-- single child -->
|
||||
</ScrollView>
|
||||
```
|
||||
|
||||
### RecyclerView
|
||||
|
||||
List of items
|
||||
|
||||
In `build.gradle`:
|
||||
|
||||
```gradle
|
||||
dependencies {
|
||||
implementation "androidx.recyclerview:recyclerview:<version>"
|
||||
// For control over item selection of both touch and mouse driven selection
|
||||
implementation "androidx.recyclerview:recyclerview-selection:<version>"
|
||||
}
|
||||
```
|
||||
|
||||
In `activity.xml`:
|
||||
|
||||
```xml
|
||||
<!-- VIewGroup for a list of items -->
|
||||
<androidx.recycleview.widget.RecycleView
|
||||
android:id="@+id/uniquieId"
|
||||
android:layout_width="value"
|
||||
android:layout_height="value">
|
||||
```
|
||||
|
||||
In `recyclerViewItem.xml`:
|
||||
|
||||
```xml
|
||||
<!-- list item layout -->
|
||||
```
|
||||
|
||||
### WebView
|
||||
|
||||
```xml
|
||||
<WebView
|
||||
android:id="@+id/uniquieId"
|
||||
android:layout_width="value"
|
||||
android:layout_height="value">
|
||||
```
|
||||
|
||||
## Constraints
|
||||
|
||||
If a view is *anchored on both sides* and the width/height is set to `0dp` the view will expand to touch the elements to which is anchored.
|
200
Bash/Bash Commands.md
Normal file
200
Bash/Bash Commands.md
Normal file
|
@ -0,0 +1,200 @@
|
|||
# Bash Commands
|
||||
|
||||
**NOTE**: Square brackets (`[]`) denotes optional commands/flags/arguments. Uppercase denotes placeholders for arguments.
|
||||
|
||||
## Basic Commands
|
||||
|
||||
### Elevated Priviledges and Users
|
||||
|
||||
[sudo vs su](https://unix.stackexchange.com/questions/35338/su-vs-sudo-s-vs-sudo-i-vs-sudo-bash/35342)
|
||||
|
||||
```bash
|
||||
sudo su # login as root (user must be sudoer, root password not required) DANGEROUS
|
||||
sudo -s # act as root and inherit current user enviroment (env as is now, along current dir and env vars) SAFE (can modify user enviroment)
|
||||
sudo -i # act as root and and use a clean enviroment (goes to user's home, runs .bashrc) SAFEST
|
||||
sudo COMMAND # run a command w\ root permissions
|
||||
sudo -u USER COMMAND # run command as user
|
||||
|
||||
su # become root (must know root password) DANGEROUS
|
||||
su - USER # change user and load it's home folder
|
||||
su USER # change user but dont load it's home folder
|
||||
```
|
||||
|
||||
### Getting Info
|
||||
|
||||
```sh
|
||||
man COMMAND # show command manual
|
||||
help COMMAND # show command info
|
||||
whatis COMMAND # one-line command explanation
|
||||
apropos COMMAND # search related commands
|
||||
which COMMAND # locate a command
|
||||
history # list of used commands
|
||||
|
||||
id # Print user and group information for the specified USER, or (when USER omitted) for the current user
|
||||
```
|
||||
|
||||
### Moving & Showing Directory Contents
|
||||
|
||||
```sh
|
||||
pwd # print working (current) directory
|
||||
ls [option]... [FILE]... # list directory contents ("list storage")
|
||||
cd rel_path # change directory to path (rel_path must be inside current directory)
|
||||
cd abs_path # change directory to path
|
||||
cd .. # change directory to parent directory
|
||||
cd ~ # go to /home
|
||||
cd - # go to previous directory
|
||||
pushd PATH # go from current directory to path
|
||||
popd # return to previous directory (before pushd)
|
||||
```
|
||||
|
||||
### Creating, Reading, Copying, Moving, Modifying Files And Directories
|
||||
|
||||
```sh
|
||||
touch FILE # change FILE timestamp fi exists, create file otherwise
|
||||
cat [FILE] # concatenate files and print on statndard output (FD 1)
|
||||
cat >> FILE # append following content ot file (Ctrl+D to stop)
|
||||
file FILE # discover file extension and format
|
||||
stat FILE # display file or file system status
|
||||
|
||||
tail # output the last part of a file
|
||||
tail [-nNUM] # output the last NUM lines
|
||||
|
||||
more # filter for paging through text one screenful at a time
|
||||
less # opposite of more (display big file in pages), navigate with arrow keys or spacebar
|
||||
|
||||
cut # remove sections from each line of files
|
||||
cut -[d --delimiter=DELIM] # use DELIM instead of TAB for field delimiter
|
||||
cut [-f --fields=LIST] # select only these fields
|
||||
|
||||
df # report file system disk space usage
|
||||
|
||||
rm FILE # remove file or directories
|
||||
rm DIRECTORY -r # remove directory an all its contents (recursive)
|
||||
rmdir DIRECTORY # remove directory only if is empty
|
||||
|
||||
mkdir DIRECTORY # make directories
|
||||
|
||||
mv SOURCE DESTINATION # move or raneme files
|
||||
mv SOURCE DIRECTORY # move FILE to DIRECTORY
|
||||
|
||||
cp SOURCE DESTINATION # copy SOURCE to DESTIANTION
|
||||
```
|
||||
|
||||
### Files Permissions & Ownership
|
||||
|
||||

|
||||
|
||||
```sh
|
||||
chmod MODE FILE # change file (or directory) permissions
|
||||
chmod OCTAL-MODE FILE # change file (or directory) permissions
|
||||
|
||||
chown [OPTION]... [OWNER][:[GROUP]] FILE... # change file owner and group
|
||||
chgrp [OPTION]... GROUP FILE... # change group ownership
|
||||
```
|
||||
|
||||
**File Permissions**:
|
||||
|
||||
- `r`: Read. Can see file content
|
||||
- `w`: Write. Can modify file content
|
||||
- `x`: Execute. Can execute file
|
||||
|
||||
**Directory Permissions**:
|
||||
|
||||
- `r`: Read. Can see dir contents
|
||||
- `w`: CRUD. Can create, rename and delete files
|
||||
- `x`: Search. Can access and navigate inside the dir. Necessary to operate on files
|
||||
|
||||
***Common* Octal Files Permissions**:
|
||||
|
||||
- `777`: (`rwxrwxrwx`) No restrictions on permissions. Anybody may do anything. Generally not a desirable setting.
|
||||
- `755`: (`rwxr-xr-x`) The file's owner may read, write, and execute the file. All others may read and execute the file. This setting is common for programs that are used by all users.
|
||||
- `700`: (`rwx------`) The file's owner may read, write, and execute the file. Nobody else has any rights. This setting is useful for programs that only the owner may use and must be kept private from others.
|
||||
- `666`: (`rw-rw-rw-`) All users may read and write the file.
|
||||
- `644`: (`rw-r--r--`) The owner may read and write a file, while all others may only read the file. A common setting for data files that everybody may read, but only the owner may change.
|
||||
- `600`: (`rw-------`) The owner may read and write a file. All others have no rights. A common setting for data files that the owner wants to keep private.
|
||||
|
||||
***Common* Octal Directory Permissions**:
|
||||
|
||||
- `777`: (`rwxrwxrwx`) No restrictions on permissions. Anybody may list files, create new files in the directory and delete files in the directory. Generally not a good setting.
|
||||
- `755`: (`rwxr-xr-x`) The directory owner has full access. All others may list the directory, but cannot create files nor delete them. This setting is common for directories that you wish to share with other users.
|
||||
- `700`: (`rwx------`) The directory owner has full access. Nobody else has any rights. This setting is useful for directories that only the owner may use and must be kept private from others.
|
||||
|
||||
### Finding Files And Directories
|
||||
|
||||
```sh
|
||||
find [path] [expression] # search file in directory hierarchy
|
||||
find [start-position] -type f -name FILENAME # search for a file named "filename"
|
||||
find [start-position] -type d -name DIRNAME # search for a directory named "dirname"
|
||||
find [path] -exec <command> {} \; # ececute command on found items (identified by {})
|
||||
|
||||
[ -f "path" ] # test if a file exists
|
||||
[ -d "path" ] # test if a folder exists
|
||||
```
|
||||
|
||||
### Other
|
||||
|
||||
```sh
|
||||
tee # copy standard input and write to standard output AND files simultaneously
|
||||
tee [FILE]
|
||||
command | sudo tee FILE # operate on file w/o using shell as su
|
||||
|
||||
echo # display a line of text
|
||||
echo "string" > FILE # write lin of text to file
|
||||
echo "string" >> FILE # append line o ftext to end of file (EOF)
|
||||
|
||||
wget URL # download repositories to linux machine
|
||||
|
||||
curl # dovnlaod the contents of a URL
|
||||
curl [-I --head] # Fetch the headers only
|
||||
|
||||
ps [-ax] # display processes
|
||||
kill <PID> # kill process w/ Process ID <PID>
|
||||
killall PROCESS # kill process by nane
|
||||
|
||||
grep # search through a string using a REGEX
|
||||
grep [-i] # grep ignore case
|
||||
|
||||
source script.sh # load script as a command
|
||||
diff FILES # compare files line by line
|
||||
|
||||
# sudo apt install shellcheck
|
||||
shellcheck FILE # shell linter
|
||||
|
||||
xargs [COMMAND] # build and execute command lines from standard input
|
||||
# xargs reads items form the standard input, delimites by blanks or newlines, and executes the COMMAND one or more times with the items as argumests
|
||||
watch [OPTIONS] COMMAND # execute a program periodically, showing output fullscreen
|
||||
watch -n SECONDS COMMAND # execute command every SECONDS seconds (no less than 0.1 seconds)
|
||||
```
|
||||
|
||||
## Data Wrangling
|
||||
|
||||
**Data wrangling** is the process of transforming and mapping data from one "raw" data form into another format with the intent of making it more appropriate and valuable for a variety of downstream purposes such as analytics.
|
||||
|
||||
```bash
|
||||
sed # stream editor for filtering and transforming text
|
||||
sed -E "s/REGEX/replacement/" # subsitute text ONCE (-E uses modern REGEX)
|
||||
sed -E "s/REGEX/replacement/g" # subsitute text multiple times (every match)
|
||||
|
||||
wc [FILE] # print newline, word and byte countd for each file
|
||||
wc [-m --chars] FILE # print character count
|
||||
wc [-c --bytes] FILE # print bytes count
|
||||
wc [-l --lines] FILE # print lines count
|
||||
wc [-w --words] FILE # print word count
|
||||
|
||||
sort [FILE] # sort lines of a text file
|
||||
|
||||
uniq [INPUT [OUTPUT]] # report or omit repeated lines (from INPUT to OUTPUT)
|
||||
uniq [-c --count] # prefix lines w/ number of occurrences
|
||||
uniq [-d --repeated] # plrint only duplicare lines, one for each group
|
||||
uniq [-D] # plrint only duplicare lines
|
||||
|
||||
paste [FILE] # merge lines of files
|
||||
paste [-d --delimiters=LIST] # use delimiters from LIST
|
||||
paste [- --serial] # paste one file at a time instead of in parallel
|
||||
|
||||
awk '{program}' # pattern scanning and processing language
|
||||
awk [-f --file PROGRAM_FILE] # read program source from PROGRAM_FILE instead of from first argument
|
||||
|
||||
bc [-hlwsqv long-options] [FILE] # arbitrary precision calculator language
|
||||
bc [-l --mathlib] [FILE] # use standard math library
|
||||
```
|
256
Bash/Bash Scripting.md
Normal file
256
Bash/Bash Scripting.md
Normal file
|
@ -0,0 +1,256 @@
|
|||
# Bash Cheat Sheet
|
||||
|
||||
[Bash Manual](https://www.gnu.org/software/bash/manual/)
|
||||
|
||||
`Ctrl+Shift+C`: copy
|
||||
`Ctrl+Shift+C`: paste
|
||||
|
||||
## Bash Use Modes
|
||||
|
||||
Interactive mode --> shell waits for user's commands
|
||||
Non-interactive mode --> shell runs scripts
|
||||
|
||||
## File & Directories Permissons
|
||||
|
||||
File:
|
||||
|
||||
- `r`: Read. Can see file content
|
||||
- `w`: Write. Can modify file content
|
||||
- `x`: Execute. Can execute file
|
||||
|
||||
Directory:
|
||||
|
||||
- `r`: Read. Can see dir contents
|
||||
- `w`: CRD. Can create, rename and delete files
|
||||
- `x`: Search. Can access and navigate inside the dir. Necessary to operate on files
|
||||
|
||||
## File Descriptors
|
||||
|
||||
`FD 0` "standard input" --> Channel for standard input (default keyboard)
|
||||
`FD 1` "standard output" --> Channel for the default output (default screen)
|
||||
`FD 2` "standard error" --> Channel for error messages, info messages, prompts (default keyboard)
|
||||
File descriptors chan be joined to create streams that lead to files, devices or other processes.
|
||||
|
||||
Bash gets commands by reading lines.
|
||||
As soon as it's read enough lines to compose a complete command, bash begins running that command.
|
||||
Usually, commands are just a single line long. An interactive bash session reads lines from you at the prompt.
|
||||
Non-interactive bash processes read their commands from a file or stream.
|
||||
Files with a hashbang as their first line (and the executable permission) can be started by your system's kernel like any other program.
|
||||
|
||||
### First Line Of Bash
|
||||
|
||||
`#!/bin/env bash`
|
||||
Hashbang indicating whitch interpreter to use
|
||||
|
||||
### Simple Command
|
||||
|
||||
```bash
|
||||
[ var=value ... ] command [ arg ... ] [ redirection ... ] # [.] is optional component
|
||||
```
|
||||
|
||||
### Pipelines (commands concatenation)
|
||||
|
||||
```bash
|
||||
command | file.ext # link the first process' standard output to the second process' standard input
|
||||
command |& file.ext # link the first process' standard output & standard error to the second process' standard input
|
||||
```
|
||||
|
||||
### Lists (sequence of commands)
|
||||
|
||||
```bash
|
||||
command_1; command_2; ... # execute command in sequence, one after the other
|
||||
command_1 || command_2 || ... # execute successive commands only if preceding ones fail
|
||||
```
|
||||
|
||||
### COMPOUND COMMANDs (multiple commands as one)
|
||||
|
||||
```bash
|
||||
# block of commands executed as one
|
||||
<keyword>
|
||||
command_1; command_2; ...
|
||||
<end_keyword>
|
||||
|
||||
{command_1; command_2; ...} # sequence of commands executed as one
|
||||
```
|
||||
|
||||
### Functions (blocks of easely reusable code)
|
||||
|
||||
`function_name () {compound_command}`
|
||||
Bash does not accept func arguments, parentheses must be empty
|
||||
|
||||
## Command names & Running programs
|
||||
|
||||
To run a command, bash uses the name of your command and performs a search for how to execute that command.
|
||||
In order, bash will check whether it has a function or builtin by that name.
|
||||
Failing that, it will try to run the name as a program.
|
||||
If bash finds no way to run your command, it will output an error message.
|
||||
|
||||
## The path to a program
|
||||
|
||||
When bash needs to run a program, it uses the command name to perform a search.
|
||||
Bash searches the directories in your PATH environment variable, one by one, until it finds a directory that contains a program with the name of your command.
|
||||
To run a program that is not installed in a PATH directory, use the path to that program as your command's name.
|
||||
|
||||
## Command arguments & Quoting literals
|
||||
|
||||
To tell a command what to do, we pass it arguments. In bash, arguments are tokens, that are separated from each other by blank space.
|
||||
To include blank space in an argument's value, you need to either quote the argument or escape the blank space within.
|
||||
Failing that, bash will break your argument apart into multiple arguments at its blank space.
|
||||
Quoting arguments also prevents other symbols in it from being accidentally interpreted as bash code.
|
||||
|
||||
## Managing a command's input and output using redirection
|
||||
|
||||
By default, new commands inherit the shell's current file descriptors.
|
||||
We can use redirections to change where a command's input comes from and where its output should go to.
|
||||
File redirection allows us to stream file descriptors to files.
|
||||
We can copy file descriptors to make them share a stream. There are also many other more advanced redirection operators.
|
||||
|
||||
### Redirections
|
||||
|
||||
```bash
|
||||
[x]>file # make FD x write to file
|
||||
[x]<file # make FD x read from file
|
||||
|
||||
[x]>&y # make FD x write to FD y's stream
|
||||
[x]<&y # make FD x read from FD y's stream
|
||||
&>file # make both FD 1 (standard output) & FD 2 (standard error) write to file
|
||||
|
||||
[x]>>file # make FD x append to end of file
|
||||
x>&-, x<&- # close FD x (stream disconnected from FD x)
|
||||
[x]>&y-, [x]<&y- # replace FD x with FD y
|
||||
[x]<>file # open FD x for both reading and writing to file
|
||||
```
|
||||
|
||||
## Pathname Expansion (filname pattern [glob] matching)
|
||||
|
||||
`*` matches any kind of text (even no text).
|
||||
`?` matches any single character.
|
||||
`[characters]` mathces any single character in the given set.
|
||||
`[[:classname:]]` specify class of characters to match.
|
||||
`{}` expand list of arguments (applies command to each one)
|
||||
|
||||
`shopt -s extglob` enables extended globs (patterns)
|
||||
|
||||
`+(pattern [| pattern ...])` matches when any of the patterns in the list appears, once or many times over. ("at least one of ...").
|
||||
`*(pattern [| pattern ...])` matches when any of the patterns in the list appears, once, not at all, or many times over. ("however many of ...").
|
||||
`?(pattern [| pattern ...])` matches when any of the patterns in the list appears, once, not at all, or many times over. ("however many of ...").
|
||||
`@(pattern [| pattern ...])` matches when any of the patterns in the list appears just once. ("one of ...").
|
||||
`!(pattern [| pattern ...])` matches only when none of the patterns in the list appear. ("none of ...").
|
||||
|
||||
## Command Substituition
|
||||
|
||||
With Command Substitution, we effectively write a command within a command, and we ask bash to expand the inner command into its output and use that output as argument data for the main command.
|
||||
|
||||
```bash
|
||||
$(inner_command) # $ --> value-expansion prefix
|
||||
```
|
||||
|
||||
## Shell Variables
|
||||
|
||||
```bash
|
||||
varname=value # variable assignement
|
||||
varname="$(command)" # command sobstituition, MUST be double-quoted
|
||||
"$varname", "${varname}" # variable expansion, MUST be double-quoted (name substituited w/ varaible content)
|
||||
|
||||
$$ # pid
|
||||
$# # number of arguments passed
|
||||
$@ # all arguments passed
|
||||
${n} # n-th argument passed to the command
|
||||
$0 # name of the script
|
||||
$_ # last argument passed to the command
|
||||
$? # error message of the last (previous) comman
|
||||
!! # executes last command used (echo !! prints the last command)
|
||||
```
|
||||
|
||||
## Parameter Expansion Modifiers (in double-quotes)
|
||||
|
||||
`${parameter#pattern}` removes the shortest string that matches the pattern if it's at the start of the value.
|
||||
`${parameter##pattern}` removes the longest string that matches the pattern if it's at the start of the value.
|
||||
`${parameter%pattern}` removes the shortest string that matches the pattern if it's at the end of the value.
|
||||
`${parameter%%pattern}` removes the longest string that matches the pattern if it's at the end of the value.
|
||||
`${parameter/pattern/replacement}` replaces the first string that matches the pattern with the replacement.
|
||||
`${parameter//pattern/replacement}` replaces each string that matches the pattern with the replacement.
|
||||
`${parameter/#pattern/replacement}` replaces the string that matches the pattern at the beginning of the value with the replacement.
|
||||
`${parameter/%pattern/replacement}` replaces the string that matches the pattern at the end of the value with the replacement.
|
||||
`${#parameter}` expands the length of the value (in bytes).
|
||||
`${parametr:start[:length]}` expands a part of the value, starting at start, length bytes long.
|
||||
Counts from the end rather than the beginning by using a (space followed by a) negative value.
|
||||
`${parameter[^|^^|,|,,][pattern]}` expands the transformed value, either upper-casing or lower-casing the first or all characters that match the pattern.
|
||||
Omit the pattern to match any character.
|
||||
|
||||
## Decision Statements
|
||||
|
||||
### If Statement
|
||||
|
||||
Only the final exit code after executing the entire list is relevant for the branch's evaluation.
|
||||
|
||||
```bash
|
||||
if command_list; then
|
||||
command_list;
|
||||
elif command_list; then
|
||||
command_list;
|
||||
else command_list;
|
||||
fi
|
||||
```
|
||||
|
||||
### Test Command
|
||||
|
||||
`[[ arggument_1 <operator> argument_2 ]]`
|
||||
|
||||
### Arithmetic expansion and evaluation
|
||||
|
||||
`(( expression ))`
|
||||
|
||||
### Comparison Operators
|
||||
|
||||
```bash
|
||||
[[ "$a" -eq "$b" ]] # is equal to
|
||||
[[ "$a" -ne "$b" ]] # in not equal to
|
||||
[[ "$a" -gt "$b" ]] # greater than
|
||||
[[ "$a" -ge "$b" ]] # greater than or equal to
|
||||
[[ "$a" -lt "$b" ]] # less than
|
||||
[[ "$a" -le "$b" ]] # less than or equal to
|
||||
```
|
||||
|
||||
### Arithmetic Comperison Operators
|
||||
|
||||
```bash
|
||||
(("$a" > "$b")) # greater than
|
||||
(("$a" >= "$b")) # greater than or equal to
|
||||
(("$a" < "$b")) # less than
|
||||
(("$a" <= "$b")) # less than or equal to
|
||||
```
|
||||
|
||||
### String Compatison Operators
|
||||
|
||||
```bash
|
||||
[ "$a" = "$b" ] # is equal to (whitespace atoun operator)
|
||||
|
||||
[[ $a == z* ]] # True if $a starts with an "z" (pattern matching)
|
||||
[[ $a == "z*" ]] # True if $a is equal to z* (literal matching)
|
||||
[ $a == z* ] # File globbing and word splitting take place
|
||||
[ "$a" == "z*" ] # True if $a is equal to z* (literal matching)
|
||||
|
||||
[ "$a" != "$b" ] # is not equal to, pattern matching within a [[ ... ]] construct
|
||||
|
||||
[[ "$a" < "$b" ]] # is less than, in ASCII alphabetical order
|
||||
[ "$a" \< "$b" ] # "<" needs to be escaped within a [ ] construct.
|
||||
|
||||
[[ "$a" > "$b" ]] # is greater than, in ASCII alphabetical order
|
||||
[ "$a" \> "$b" ] # ">" needs to be escaped within a [ ] construct.
|
||||
```
|
||||
|
||||
## Commands short circuit evaluation
|
||||
|
||||
```bash
|
||||
command_1 || command_2 # if command_1 fails executes command_2
|
||||
command_1 && command_2 # executes command_2 only if command_1 succedes
|
||||
```
|
||||
|
||||
## Loops
|
||||
|
||||
```bash
|
||||
for var in iterable ; do
|
||||
# command here
|
||||
done
|
||||
```
|
862
C++/C++.md
Normal file
862
C++/C++.md
Normal file
|
@ -0,0 +1,862 @@
|
|||
# C/C++ Cheat Sheet
|
||||
|
||||
## Naming convention
|
||||
|
||||
C++ element | Case
|
||||
-------------|------------
|
||||
class | PascalCase
|
||||
variable | camelCase
|
||||
method | camelCase
|
||||
|
||||
## Library Import
|
||||
|
||||
`#include <iostream>`
|
||||
C++ libs encapsulate C libs.
|
||||
A C library can be used with the traditional name `<lib.h>` or with the prefix _**c**_ and without `.h` as `<clib>`.
|
||||
|
||||
### Special Operators
|
||||
|
||||
Operator | Operator Name
|
||||
-----------|----------------------------------------------------
|
||||
`::` | global reference operator
|
||||
`&` | address operator (returns a memory address)
|
||||
`*` | deferentiation operator (returns the pointed value)
|
||||
|
||||
### Constant Declaration
|
||||
|
||||
```cpp
|
||||
#define constant_name value
|
||||
const type constant_name = value;
|
||||
```
|
||||
|
||||
### Console pausing before exit
|
||||
|
||||
```cpp
|
||||
#include <cstdlib>
|
||||
system("pause");
|
||||
getchar(); // waits imput from keyboard, if is last instruction will prevent closing console until satisfied
|
||||
```
|
||||
|
||||
### Namespace definition
|
||||
|
||||
Can be omitted and replaced by namespce`::`
|
||||
`using namespace <namespace>;`
|
||||
|
||||
### Main Function
|
||||
|
||||
```cpp
|
||||
int main() {
|
||||
//code here
|
||||
return 0;
|
||||
}
|
||||
```
|
||||
|
||||
### Variable Declaration
|
||||
|
||||
```cpp
|
||||
type var_name = value; //c-like initialization
|
||||
type var_name (value); //constructor initialization
|
||||
type var_name {value}; //uniform initialization
|
||||
type var_1, var_2, ..., var_n;
|
||||
```
|
||||
|
||||
### Type Casting
|
||||
|
||||
`(type) var;`
|
||||
`type(var);`
|
||||
|
||||
### Variable Types
|
||||
|
||||
Type | Value Range | Byte
|
||||
-------------------------|-----------------------------------|------
|
||||
`short` | -32768 to 32765 | 1
|
||||
`unsigned short` | 0 to 65535 | 1
|
||||
`int` | -2147483648 to 2147483647 | 4
|
||||
`unisgned int` | 0 to 4294967295 | 4
|
||||
`long` | -2147483648 to 2147483647 | 4
|
||||
`unsigned long` | 0 to 4294967295 | 4
|
||||
`long long` | | 8
|
||||
`float` | +/- 3.4e +/- 38 (~7 digits) | 4
|
||||
`double` | +/- 1.7e +/- 308 (~15 digits) | 8
|
||||
`long double` | | 16 (?)
|
||||
|
||||
Type | Value
|
||||
-------------------------|-----------------------------
|
||||
`bool` | true or false
|
||||
`char` | ascii characters
|
||||
`string` | sequence of ascii characters
|
||||
`NULL` | empty value
|
||||
|
||||
### Integer Numerals
|
||||
|
||||
Example | Type
|
||||
---------|------------------------
|
||||
`75` | decimal
|
||||
`0113` | octal (zero prefix)
|
||||
`0x4` | hexadecimal (0x prefix)
|
||||
`75` | int
|
||||
`75u` | unsigned int
|
||||
`75l` | long
|
||||
`75ul` | unsigned long
|
||||
`75lu` | unsigned long
|
||||
|
||||
### FLOATING POINT NUMERALS
|
||||
|
||||
Example | Type
|
||||
------------|-------------
|
||||
`3.14159L` | long double
|
||||
`60.22e23f` | flaot
|
||||
|
||||
Code | Value
|
||||
----------|---------------
|
||||
`3.14159` | 3.14159
|
||||
`6.02e23` | 6.022 * 10^23
|
||||
`1.6e-19` | 1.6 * 10^-19
|
||||
`3.0` | 3.0
|
||||
|
||||
### Character/String Literals
|
||||
|
||||
`'z'` single character literal
|
||||
`"text here"` string literal
|
||||
|
||||
### Special Characters
|
||||
|
||||
Escape Character | Character
|
||||
-------------------|-----------------------------
|
||||
`\n` | neweline
|
||||
`\r` | carriage return
|
||||
`\t` | tab
|
||||
`\v` | vertical tab
|
||||
`\b` | backspace
|
||||
`\f` | form feed
|
||||
`\a` | alert (beep)
|
||||
`\'` | single quote (')
|
||||
`\"` | double quote (")
|
||||
`\?` | question mark (?)
|
||||
`\\` | backslash (\)
|
||||
`\0` | string termination character
|
||||
|
||||
### Screen Output
|
||||
|
||||
```cpp
|
||||
cout << expression; // print line on screen (no automatic newline)
|
||||
cout << expressin_1 << expreeeion_2; // concatenation of outputs
|
||||
cout << expression << "\n"; // print line on screen
|
||||
cout << expression << endl; // print line on screen
|
||||
|
||||
//Substitutes variable to format specifier
|
||||
#include <stdio.h>
|
||||
printf("text %<fmt_spec>", variable); // has problems, use PRINTF_S
|
||||
printf_s("text %<fmt_spec>", variable);
|
||||
```
|
||||
|
||||
### Input
|
||||
|
||||
```cpp
|
||||
#iclude <iostream>
|
||||
cin >> var; //space terminates value
|
||||
cin >> var_1 >> var_2;
|
||||
|
||||
//if used after cin >> MUST clear buffer with cin.ignore(), cin.sync() or std::ws
|
||||
getline(stream, string, delimiter) //read input from stream (usially CIN) and store in in string, a different delimiter character can be set.
|
||||
|
||||
#include <stdio.h>
|
||||
scanf("%<fmt_spec>", &variable); // has problems, use SCANF_S
|
||||
scanf_s("%<fmt_spec>", &variable); //return number of succescully accepted inputs
|
||||
```
|
||||
|
||||
### Fromat Specifiers %[width].[lenght][specifier]
|
||||
|
||||
Specifier | Specified Format
|
||||
------------|-----------------------------------------
|
||||
`%d`, `%i` | singed decimal integer
|
||||
`%u` | unsigned decimal integer
|
||||
`%o` | unsigned octal
|
||||
`%x` | unsigned hexadecimal integer
|
||||
`%X` | unsigned hexadecimal integer (UPPERCASE)
|
||||
`%f` | decimal floating point (lowercase)
|
||||
`%F` | decimal floating point (UPPERCASE)
|
||||
`%e` | scientific notation (lowercase)
|
||||
`%E` | scientific notation (UPPERCASE)
|
||||
`%a` | hexadecimal floating point (lowercase)
|
||||
`%A` | hexadecimal floating point (UPPERCASE)
|
||||
`%c` | character
|
||||
`%s` | string
|
||||
`%p` | pointer address
|
||||
|
||||
### CIN input validation
|
||||
|
||||
```cpp
|
||||
if (cin.fail()) // if cin fails to get an input
|
||||
{
|
||||
cin.clear(); // reset cin status (modified by cin.fail() ?)
|
||||
cin.ignore(n, '\n'); //remove n characters from budder or until \n
|
||||
|
||||
//error message here
|
||||
}
|
||||
|
||||
if (!(cin >> var)) // if cin fails to get an input
|
||||
{
|
||||
cin.clear(); // reset cin status (modified by cin.fail() ?)
|
||||
cin.ignore(n, '\n'); //remove n characters from budder or until \n
|
||||
|
||||
//error message here
|
||||
}
|
||||
```
|
||||
|
||||
### Cout Format Specifier
|
||||
|
||||
```cpp
|
||||
#include <iomanip>
|
||||
cout << stew(print_size) << setprecision(num_digits) << var; //usage
|
||||
|
||||
setbase(base) //set numberica base [dec, hex, oct]
|
||||
setw(print_size) //set the total number of characters to display
|
||||
setprecision(num_digits) //sets the number of decimal digits to siaplay
|
||||
setfill(character) //use character to fill space between words
|
||||
```
|
||||
|
||||
### Arithmetic Operators
|
||||
|
||||
Operator | Operation
|
||||
---------|---------------
|
||||
a `+` b | sum
|
||||
a `-` b | subtraction
|
||||
a `*` b | multiplication
|
||||
a `/` b | division
|
||||
a `%` b | modulo
|
||||
a`++` | increment
|
||||
a`--` | decrement
|
||||
|
||||
### Comparison Operators
|
||||
|
||||
Operator | Operation
|
||||
---------|--------------------------
|
||||
a `==` b | equal to
|
||||
a `!=` b | not equal to
|
||||
a `>` b | greater than
|
||||
a `<` b | lesser than
|
||||
a `>=` b | greater than or equal to
|
||||
a `<=` b | lesser than or equal to
|
||||
|
||||
### Logical Operator
|
||||
|
||||
Operator | Operation
|
||||
---------------------|-----------------------
|
||||
`!`a, `not` a | logical negation (NOT)
|
||||
a `&&` b, a `and` b | logical AND
|
||||
a `||` b, a `or` b | logical OR
|
||||
|
||||
### Conditional Ternary Operator
|
||||
|
||||
`condition ? result_1 : result_2`
|
||||
If condition is true evaluates to result_1, and otherwise to result_2
|
||||
|
||||
### Bitwise Operators
|
||||
|
||||
Operator | Operation
|
||||
-----------------------|---------------------
|
||||
`~`a, `compl` a | bitwise **NOT**
|
||||
a `&` b, a `bitand` b | bitwise **AND**
|
||||
a `|` b, a `bitor` b | bitwise **OR**
|
||||
a `^` b, a `xor` b, | bitwise **XOR**
|
||||
a `<<` b | bitwise left shift
|
||||
a `>>` b | bitwise right shift
|
||||
|
||||
### Compound Assignement O
|
||||
|
||||
Operator | Operation
|
||||
------------|------------
|
||||
a `+=` b | a = a + b
|
||||
a `-=` b | a = a - b
|
||||
a `*=` b | a = a * b
|
||||
a `/=` b | a = a / b
|
||||
a `%=` b | a = a % b
|
||||
a `&=` b | a = a & b
|
||||
a `|=` b | a = a | b
|
||||
a `^=` b | a = a ^ b
|
||||
a `<<=` b | a = a << b
|
||||
a `>>=` b | a = a >> b
|
||||
|
||||
### Operator Precedence
|
||||
|
||||
1. `!`
|
||||
2. `*`, `/`, `%`
|
||||
3. `+`, `-`
|
||||
4. `<`, `<=`, `<`, `>=`
|
||||
5. `==`, `!=`
|
||||
6. `&&`
|
||||
7. `||`
|
||||
8. `=`
|
||||
|
||||
### Mathematical Functions
|
||||
|
||||
```cpp
|
||||
#include <cmath>
|
||||
abs(x); // absolute value
|
||||
labs(x); //absolute value if x is long, result is long
|
||||
fabs(x); //absolute value if x i float, result is float
|
||||
sqrt(x); // square root
|
||||
ceil(x); // ceil function (next initeger)
|
||||
floor(x); // floor function (integer part of x)
|
||||
log(x); // natural log of x
|
||||
log10(x); // log base 10 of x
|
||||
exp(x); // e^x
|
||||
pow(x, y); // x^y
|
||||
sin(x);
|
||||
cos(x);
|
||||
tan(x);
|
||||
asin(x); //arcsin(x)
|
||||
acos(x); //arccos(x)
|
||||
atan(x); //arctan(x)
|
||||
atan2(x, y); //arctan(x / y)
|
||||
sinh(x); //hyperbolic sin(x)
|
||||
cosh(x); //hyperbolic cos(x)
|
||||
tanh(x); //hyperbolic tan(X)
|
||||
```
|
||||
|
||||
### Character Classification
|
||||
|
||||
```cpp
|
||||
isalnum(c); //true if c is alphanumeric
|
||||
isalpha(c); //true if c is a letter
|
||||
isdigit(c); //true if char is 0 1 2 3 4 5 6 7 8 9
|
||||
iscntrl(c); //true id c is DELETE or CONTROL CHARACTER
|
||||
isascii(c); //true if c is a valid ASCII character
|
||||
isprint(c); //true if c is printable
|
||||
isgraph(c); //true id c is printable, SPACE excluded
|
||||
islower(c); //true if c is lowercase
|
||||
isupper(c); //true if c is uppercase
|
||||
ispunct(c); //true if c is punctuation
|
||||
isspace(c); //true if c is SPACE
|
||||
isxdigit(c); //true if c is HEX DIGIT
|
||||
```
|
||||
|
||||
### Character Functions
|
||||
|
||||
```cpp
|
||||
#include <ctype.n>
|
||||
|
||||
tolower(c); //transfromas charatcer in lowercase
|
||||
toupper(c); //transform character in uppercase
|
||||
```
|
||||
|
||||
### Random Numbers Between max-min (int)
|
||||
|
||||
```cpp
|
||||
#include <time>
|
||||
#include <stdlib.h>
|
||||
srand(time(NULL)); //initzialize seed
|
||||
var = rand() //random number
|
||||
var = (rand() % max + 1) //random numbers between 0 & max
|
||||
var = (rand() % (max - min + 1)) + min //random numbers between min & max
|
||||
```
|
||||
|
||||
### Flush Output Buffer
|
||||
|
||||
```cpp
|
||||
#include <stdio.h>
|
||||
fflush(FILE); // empty output buffer end write its content on argument passed
|
||||
```
|
||||
|
||||
**Do not use stdin** to empty INPUT buffers. It's undefined C behaviour.
|
||||
|
||||
## STRINGS (objects)
|
||||
|
||||
```cpp
|
||||
#include <string>
|
||||
|
||||
string string_name = "string_content"; //string declaration
|
||||
string string_name = string("strintg_content"); // string creation w/ constructor
|
||||
|
||||
string.length //returns the length of the string
|
||||
|
||||
//if used after cin >> MUST clear buffer with cin.ignore(), cin.sync() or std::ws
|
||||
getline(source, string, delimiter); //string input, source can be input stream (usually cin)
|
||||
|
||||
printf_s("%s", string.c_str()); //print the string as a char array, %s --> char*
|
||||
|
||||
string_1 + string_2; // string concatenation
|
||||
string[pos] //returns char at index pos
|
||||
```
|
||||
|
||||
### String Functions
|
||||
|
||||
```cpp
|
||||
string.c_str() //transfroms the string in pointer to char[] (char array aka C string) ter,iomated by '\0'
|
||||
|
||||
strlen(string); //return lenght (num of chars) of the string
|
||||
strcat(destination, source); //appends chars of string2 to string1
|
||||
strncat(string1, string2, nchar); //appends the first n chars of string 2 to string1
|
||||
strcpy(string1, string2.c_str()); //copies string2 into string1 char by char
|
||||
strncpy(string1, string2, n); //copy first n chars from string2 to string1
|
||||
strcmp(string1, string2); //compares string1 w/ string2
|
||||
strncmp(string1, string2, n); //compares first n chars
|
||||
//returns < 0 if string1 precedes string2
|
||||
//returns 0 if string1 == string2
|
||||
// returns > 0 if string1 succedes string2
|
||||
strchr(string, c); //returns index of c in string if it exists, NULL otherwise
|
||||
strstr(string1, string2); //returns pointer to starting index of string1 in string2
|
||||
strpbrk(string, charSet); //Returns a pointer to the first occurrence of any character from strCharSet in str, or a NULL pointer if the two string arguments have no characters in common.
|
||||
```
|
||||
|
||||
### String Conversion
|
||||
|
||||
```cpp
|
||||
atof(string); //converts string in double if possible
|
||||
atoi(string); //converts string in integer if possible
|
||||
atol(string); //converts string in long if possible
|
||||
```
|
||||
|
||||
### String Methods
|
||||
|
||||
```C++
|
||||
string.at(pos); // returns char at index pos
|
||||
string.substr(start, end); // returns substring between indexes START and END
|
||||
string.c_str(); //reads string char by char
|
||||
string.find(substring); // The zero-based index of the first character in string object that matches the requested substring or characters
|
||||
```
|
||||
|
||||
## VECTORS
|
||||
|
||||
```cpp
|
||||
#include <vector>
|
||||
vector<type> vector_name = {values}; //variable length array
|
||||
```
|
||||
|
||||
## Selection Statements
|
||||
|
||||
### Simple IF
|
||||
|
||||
```cpp
|
||||
if (condition)
|
||||
//single istruction
|
||||
|
||||
|
||||
if (condition) {
|
||||
//code here
|
||||
}
|
||||
```
|
||||
|
||||
### Simple IF-ELSE
|
||||
|
||||
```cpp
|
||||
if (condition) {
|
||||
//code here
|
||||
} else {
|
||||
//code here
|
||||
}
|
||||
```
|
||||
|
||||
## IF-ELSE mutli-branch
|
||||
|
||||
```cpp
|
||||
if (condition) {
|
||||
//code here
|
||||
} else if (condition) {
|
||||
//code here
|
||||
} else {
|
||||
//code here
|
||||
}
|
||||
```
|
||||
|
||||
### Switch
|
||||
|
||||
```cpp
|
||||
switch (expression) {
|
||||
case constant_1:
|
||||
//code here
|
||||
break;
|
||||
|
||||
case constant_2:
|
||||
//code here
|
||||
break;
|
||||
|
||||
default:
|
||||
//code here
|
||||
}
|
||||
```
|
||||
|
||||
## Loop Statements
|
||||
|
||||
### While Loop
|
||||
|
||||
```cpp
|
||||
while (condition) {
|
||||
//code here
|
||||
}
|
||||
```
|
||||
|
||||
### Do While
|
||||
|
||||
```cpp
|
||||
do {
|
||||
//code here
|
||||
} while (condition);
|
||||
```
|
||||
|
||||
### For Loop
|
||||
|
||||
```cpp
|
||||
for (initialization; condition; increase) {
|
||||
//code here
|
||||
}
|
||||
```
|
||||
|
||||
### Range-Based For Loop
|
||||
|
||||
```cpp
|
||||
for (declaration : range) {
|
||||
//code here
|
||||
}
|
||||
```
|
||||
|
||||
### Break Statement
|
||||
|
||||
`break;` leaves a loop, even if the condition for its end is not fulfilled.
|
||||
|
||||
### Continue Statement
|
||||
|
||||
`continue;` causes the program to skip the rest of the loop in the current iteration.
|
||||
|
||||
## Functions
|
||||
|
||||
Functions **must** be declared **before** the main function.
|
||||
It is possible to declare functions **after** the main only if the *prototype* is declared **before** the main.
|
||||
To return multiple variables those variables can be passed by reference so that their values is adjourned in the main.
|
||||
|
||||
### Function Prototype (before main)
|
||||
|
||||
`type function_name(type argument1, ...);`
|
||||
|
||||
### Standard Function
|
||||
|
||||
```cpp
|
||||
type functionName (parameters) { //parametri formali aka arguents
|
||||
//code here
|
||||
return <expression>;
|
||||
}
|
||||
```
|
||||
|
||||
### Void Function (aka procedure)
|
||||
|
||||
```cpp
|
||||
void functionName (parameters) {
|
||||
//code here
|
||||
}
|
||||
```
|
||||
|
||||
### Arguments passed by reference without pointers
|
||||
|
||||
Passing arguments by reference causes modifications made inside the funtion to be propagated to the values outside.
|
||||
Passing arguments by values copies the values to the arguments: changes remain inside the function.
|
||||
|
||||
```cpp
|
||||
type functionName (type &argument1, ...) {
|
||||
//code here
|
||||
return <expression>;
|
||||
}
|
||||
```
|
||||
|
||||
`functionName (arguments);`
|
||||
|
||||
### Arguments passed by reference with pointers
|
||||
|
||||
Passing arguments by reference causes modifications made inside the funtion to be propagated to the values outside.
|
||||
Passing arguments by values copies the values to the arguments: changes remain inside the function.
|
||||
|
||||
```cpp
|
||||
type function_name (type *argument_1, ...) {
|
||||
instructions;
|
||||
return <expression>;
|
||||
}
|
||||
```
|
||||
|
||||
`function_name (&argument_1, ...);`
|
||||
|
||||
## Arrays
|
||||
|
||||
```cpp
|
||||
type arrayName[dimension]; //array declaration
|
||||
type arrayName[dimension] = {value1, value2, ...}; //array declaration & inizialization, values number must match dimension
|
||||
|
||||
array[index] //item access, index starts at 0 (zero)
|
||||
array[index] = value; //value assignement at position index
|
||||
```
|
||||
|
||||
## String as array of Chars
|
||||
|
||||
```cpp
|
||||
char string[] = "text"; //converts string in char array, string length determines dimesion of the array
|
||||
string str = string[] //a array of chars is automatically converted to a string
|
||||
```
|
||||
|
||||
## Array as function parameter
|
||||
|
||||
The dimesion is not specified because it is determined by the passed array.
|
||||
The array is passed by reference.
|
||||
|
||||
```cpp
|
||||
type function(type array[]){
|
||||
//code here
|
||||
}
|
||||
|
||||
//array is not modificable inside the function (READ ONLY)
|
||||
type function(const type array[]){
|
||||
//code here
|
||||
}
|
||||
|
||||
function(array); //array passed w/out square brackets []
|
||||
```
|
||||
|
||||
### Multi-Dimensional Array (Matrix)
|
||||
|
||||
```cpp
|
||||
type matrix[rows][columns];
|
||||
matrix[i][j] //element A_ij of the matrix
|
||||
```
|
||||
|
||||
### Matrix as function parameter
|
||||
|
||||
```cpp
|
||||
//matrix passed by reference, second dimension is mandatory
|
||||
type function(type matrix[][columns]){
|
||||
//code here
|
||||
};
|
||||
|
||||
//martix values READ ONLY
|
||||
type function(const type matric[][columns]){
|
||||
//code here
|
||||
}
|
||||
|
||||
type function(type matrix[][dim2]...[dimN]){
|
||||
//code here
|
||||
}
|
||||
```
|
||||
|
||||
## Record (struct)
|
||||
|
||||
List of non omogeneous items
|
||||
|
||||
### Struct Definition (before functions, outside main)
|
||||
|
||||
```cpp
|
||||
struct structName {
|
||||
type field1;
|
||||
type field2;
|
||||
type field3;
|
||||
type field4;
|
||||
};
|
||||
|
||||
structName variable; //STRUCT variable
|
||||
variable.field //field access
|
||||
```
|
||||
|
||||
## Pointers
|
||||
|
||||
Pointers hold memory adresses of declared variables, they should be initialized to NULL.
|
||||
|
||||
```cpp
|
||||
type *pointer = &variable; //pointer init and assignement
|
||||
type *pointer = NULL;
|
||||
type *pointer = otherPointer;
|
||||
type **pointerToPointer = &pointer; // pointerToPointer -> pointer -> variable
|
||||
```
|
||||
|
||||
`&variable` extracts the address, the pointer holds the address of the variable.
|
||||
pointer type and variable type **must** match.
|
||||
(*) --> "value pointed to by"
|
||||
|
||||
```cpp
|
||||
pointer //addres of pointed value (value of variable)
|
||||
*pointer //value of pointed variable
|
||||
**pointer //value pointed by *pointer (pointer to pointer)
|
||||
```
|
||||
|
||||
### Pointer to array
|
||||
|
||||
```cpp
|
||||
type *pointer;
|
||||
type array[dim] = {};
|
||||
|
||||
|
||||
pointer = array; //point to array (pointer points to first "cell" of array)
|
||||
pointer++; //change pointed value to successive "cell" of array
|
||||
```
|
||||
|
||||
### Pointers, Arrays & Functions
|
||||
|
||||
```cpp
|
||||
func(array) //pass entire array to function (no need to use (&) to extract address)
|
||||
|
||||
type func(type* array){
|
||||
array[index] //acces to item of array at index
|
||||
}
|
||||
```
|
||||
|
||||
### Pointer to Struct
|
||||
|
||||
```cpp
|
||||
(*structPointer).field //acces to field value
|
||||
structPointer->structField //acces to field value
|
||||
```
|
||||
|
||||
## Dynamic Structures
|
||||
|
||||
Dynamic structures are structures without a fixed number of items.
|
||||
|
||||
Every item in a dynamic structure is called **node**.
|
||||
Every node is composed by two parts:
|
||||
|
||||
* the value (item)
|
||||
* pointer to successive node
|
||||
|
||||
**Lists** are *linear* dinamyc structures in which is only defined the preceding and succeding item. A List is a group of homogeneous items (all of the same type).
|
||||
|
||||
**Trees**, **Graphs** are non *linear* dynamic structures in which an item cha have multiple successors.
|
||||
|
||||
### Stack
|
||||
|
||||
A **Stack** is a list in with nodes can be extracted from one *side* only (*LIFO*).
|
||||
The extraction of an item from the *top* is called **pop**
|
||||
|
||||
```cpp
|
||||
// node structure
|
||||
struct Node {
|
||||
type value;
|
||||
stack *next;
|
||||
}
|
||||
```
|
||||
|
||||
#### Node Instertion
|
||||
|
||||
```cpp
|
||||
Node *stackNode; //current node
|
||||
Node* head = NULL; //pointer to head of stack
|
||||
|
||||
int nodeValue;
|
||||
//assign value to nodeValue
|
||||
|
||||
stackNode = new Node; //create new node
|
||||
|
||||
stackNode->value = nodevalue; //valorize node
|
||||
stackNode->next = head; //update node poiter to old head adding it to the stack
|
||||
|
||||
head = stackNode; //update head to point to new first node
|
||||
```
|
||||
|
||||
#### Node Deletion
|
||||
|
||||
```cpp
|
||||
stackNode = head->next; //memorize location of second node
|
||||
delete head; //delete first node
|
||||
head = stackNode; //update head to point to new first node
|
||||
```
|
||||
|
||||
#### Passing Head To Functions
|
||||
|
||||
```cpp
|
||||
type function(Node** head) //value of head passed by address (head is Node*)
|
||||
{
|
||||
*head = ... //update value of head (pointed variable/object/Node)
|
||||
}
|
||||
```
|
||||
|
||||
### Queue
|
||||
|
||||
A **Queue** is a list in which nodes enter from one side and can be extracted only from the other side (*FIFO*).
|
||||
|
||||
### Linked List
|
||||
|
||||
A **Linked List** is list in which nodes can be extracted from each side and from inside the linked list.
|
||||
|
||||
Linked lists can be *linear*, *circular* or *bidirectional*.
|
||||
In circular linked lists the last node points to the first.
|
||||
|
||||
Nodes of bidirectional linked lists are composed by three parts:
|
||||
|
||||
* the value (item)
|
||||
* pointer to successive node
|
||||
* pointer to previous item
|
||||
|
||||
Thus the first and last node will have a component empty since they only point to a single node.
|
||||
|
||||
### Dynamic Memory Allocation
|
||||
|
||||
C/C++ does not automatically free allocated memory when nodes are deleted. It must be done manuallty.
|
||||
|
||||
In **C++**:
|
||||
|
||||
* `new` is used to allocate memory dynamically.
|
||||
* `delete` is use to free the dinamically allocated memory.
|
||||
|
||||
In **C**:
|
||||
|
||||
* `malloc()` returns a void pointer if the allocation is successful.
|
||||
* `free()` frees the memory
|
||||
|
||||
```C
|
||||
list *pointer = (list*)malloc(sizeof(list)); //memory allocation
|
||||
free(pointer) //freeing of memory
|
||||
```
|
||||
|
||||
`malloc()` returns a *void pointer* thus the list must be casted to a void type with `(list*)`
|
||||
|
||||
## Files
|
||||
|
||||
The object oriented approach is based on the use of *streams*.
|
||||
A **Stream** can be considered a stream of data that passes sequentially from a source to a destination.
|
||||
|
||||
The avaiable classes in C++ to operate on files are:
|
||||
|
||||
* `ifstream` for the input (reading)
|
||||
* `ofstream` for the output (writing)
|
||||
* `fstream` for input or output
|
||||
|
||||
### File Opening
|
||||
|
||||
Filename can be string literal or CharArray (use `c_str()`).
|
||||
|
||||
```cpp
|
||||
ifstream file;
|
||||
file.open("filename"); //read from file
|
||||
|
||||
ofstream file;
|
||||
file.open("filename"); //writo to file
|
||||
|
||||
|
||||
fstream file;
|
||||
file.open("filename", ios::in); //read form file
|
||||
file.open("filename", ios::out); //write to file
|
||||
file.open("filename", ios::app); //append to file
|
||||
file.open("filename", ios::trunc); //overwrite file
|
||||
file.open("filename", ios::nocreate); //opens file only if it exists, error otherwise. Does not create new file
|
||||
file.open("filename", ios::noreplace); //opens file only if it not exists, error otherwise. If it not exists the file is created.
|
||||
file.open("filename", ios::binary); //opens file in binary format
|
||||
```
|
||||
|
||||
If file opening fails the stream has value 0, otherwise the value is the assigned memory address.
|
||||
Opening modes can be conbined with the OR operator: `ios::mode | ios::mode`.
|
||||
|
||||
### File Reading & Writing
|
||||
|
||||
To write to and read from a file the `>>` and `<<` operators are used.
|
||||
|
||||
```cpp
|
||||
file.open("filename", ios::in | ios::out);
|
||||
|
||||
file << value << endl; //write to file
|
||||
|
||||
string line;
|
||||
do {
|
||||
getline(file, line); //read file line by line
|
||||
//code here
|
||||
} while (!file.eof()); // or !EOF
|
||||
```
|
||||
|
||||
### Stream state & Input errors
|
||||
|
||||
Once a stream is in a **state of error** it will remain so until the status flags are *explicitly resetted*. The input operations on such a stream are *void* until the reset happens.
|
||||
To clear the status of a stream the `clear()` method is used.
|
||||
Furthermore, when an error on the stream happens **the stream is not cleared** of it's charaters contents.
|
||||
To clear the stream contents the `ignore()` method is used.
|
1131
CSS/CSS.md
Normal file
1131
CSS/CSS.md
Normal file
File diff suppressed because it is too large
Load diff
8
CSS/Programming Notes.code-workspace
Normal file
8
CSS/Programming Notes.code-workspace
Normal file
|
@ -0,0 +1,8 @@
|
|||
{
|
||||
"folders": [
|
||||
{
|
||||
"path": ".."
|
||||
}
|
||||
],
|
||||
"settings": {}
|
||||
}
|
483
CSS/resets/destyle.css
Normal file
483
CSS/resets/destyle.css
Normal file
|
@ -0,0 +1,483 @@
|
|||
/*! destyle.css v1.0.11 | MIT License | https://github.com/nicolas-cusan/destyle.css */
|
||||
|
||||
/* Reset box-model
|
||||
========================================================================== */
|
||||
|
||||
* {
|
||||
box-sizing: border-box;
|
||||
}
|
||||
|
||||
::before,
|
||||
::after {
|
||||
box-sizing: inherit;
|
||||
}
|
||||
|
||||
/* Document
|
||||
========================================================================== */
|
||||
|
||||
/**
|
||||
* 1. Correct the line height in all browsers.
|
||||
* 2. Prevent adjustments of font size after orientation changes in iOS.
|
||||
* 3. Remove gray overlay on links for iOS.
|
||||
*/
|
||||
|
||||
html {
|
||||
line-height: 1.15; /* 1 */
|
||||
-webkit-text-size-adjust: 100%; /* 2 */
|
||||
-webkit-tap-highlight-color: transparent; /* 3*/
|
||||
}
|
||||
|
||||
/* Sections
|
||||
========================================================================== */
|
||||
|
||||
/**
|
||||
* Remove the margin in all browsers.
|
||||
*/
|
||||
|
||||
body {
|
||||
margin: 0;
|
||||
}
|
||||
|
||||
/**
|
||||
* Render the `main` element consistently in IE.
|
||||
*/
|
||||
|
||||
main {
|
||||
display: block;
|
||||
}
|
||||
|
||||
/* Vertical rhythm
|
||||
========================================================================== */
|
||||
|
||||
p,
|
||||
table,
|
||||
blockquote,
|
||||
address,
|
||||
pre,
|
||||
iframe,
|
||||
form,
|
||||
figure,
|
||||
dl {
|
||||
margin: 0;
|
||||
}
|
||||
|
||||
/* Headings
|
||||
========================================================================== */
|
||||
|
||||
h1,
|
||||
h2,
|
||||
h3,
|
||||
h4,
|
||||
h5,
|
||||
h6 {
|
||||
font-size: inherit;
|
||||
line-height: inherit;
|
||||
font-weight: inherit;
|
||||
margin: 0;
|
||||
}
|
||||
|
||||
/* Lists (enumeration)
|
||||
========================================================================== */
|
||||
|
||||
ul,
|
||||
ol {
|
||||
margin: 0;
|
||||
padding: 0;
|
||||
list-style: none;
|
||||
}
|
||||
|
||||
/* Lists (definition)
|
||||
========================================================================== */
|
||||
|
||||
dt {
|
||||
font-weight: bold;
|
||||
}
|
||||
|
||||
dd {
|
||||
margin-left: 0;
|
||||
}
|
||||
|
||||
/* Grouping content
|
||||
========================================================================== */
|
||||
|
||||
/**
|
||||
* 1. Add the correct box sizing in Firefox.
|
||||
* 2. Show the overflow in Edge and IE.
|
||||
*/
|
||||
|
||||
hr {
|
||||
box-sizing: content-box; /* 1 */
|
||||
height: 0; /* 1 */
|
||||
overflow: visible; /* 2 */
|
||||
border: 0;
|
||||
border-top: 1px solid;
|
||||
margin: 0;
|
||||
clear: both;
|
||||
color: inherit;
|
||||
}
|
||||
|
||||
/**
|
||||
* 1. Correct the inheritance and scaling of font size in all browsers.
|
||||
* 2. Correct the odd `em` font sizing in all browsers.
|
||||
*/
|
||||
|
||||
pre {
|
||||
font-family: monospace, monospace; /* 1 */
|
||||
font-size: inherit; /* 2 */
|
||||
}
|
||||
|
||||
address {
|
||||
font-style: inherit;
|
||||
}
|
||||
|
||||
/* Text-level semantics
|
||||
========================================================================== */
|
||||
|
||||
/**
|
||||
* Remove the gray background on active links in IE 10.
|
||||
*/
|
||||
|
||||
a {
|
||||
background-color: transparent;
|
||||
text-decoration: none;
|
||||
color: inherit;
|
||||
}
|
||||
|
||||
/**
|
||||
* 1. Remove the bottom border in Chrome 57-
|
||||
* 2. Add the correct text decoration in Chrome, Edge, IE, Opera, and Safari.
|
||||
*/
|
||||
|
||||
abbr[title] {
|
||||
border-bottom: none; /* 1 */
|
||||
text-decoration: underline; /* 2 */
|
||||
text-decoration: underline dotted; /* 2 */
|
||||
}
|
||||
|
||||
/**
|
||||
* Add the correct font weight in Chrome, Edge, and Safari.
|
||||
*/
|
||||
|
||||
b,
|
||||
strong {
|
||||
font-weight: bolder;
|
||||
}
|
||||
|
||||
/**
|
||||
* 1. Correct the inheritance and scaling of font size in all browsers.
|
||||
* 2. Correct the odd `em` font sizing in all browsers.
|
||||
*/
|
||||
|
||||
code,
|
||||
kbd,
|
||||
samp {
|
||||
font-family: monospace, monospace; /* 1 */
|
||||
font-size: inherit; /* 2 */
|
||||
}
|
||||
|
||||
/**
|
||||
* Add the correct font size in all browsers.
|
||||
*/
|
||||
|
||||
small {
|
||||
font-size: 80%;
|
||||
}
|
||||
|
||||
/**
|
||||
* Prevent `sub` and `sup` elements from affecting the line height in
|
||||
* all browsers.
|
||||
*/
|
||||
|
||||
sub,
|
||||
sup {
|
||||
font-size: 75%;
|
||||
line-height: 0;
|
||||
position: relative;
|
||||
vertical-align: baseline;
|
||||
}
|
||||
|
||||
sub {
|
||||
bottom: -0.25em;
|
||||
}
|
||||
|
||||
sup {
|
||||
top: -0.5em;
|
||||
}
|
||||
|
||||
/* Embedded content
|
||||
========================================================================== */
|
||||
|
||||
/**
|
||||
* Remove the border on images inside links in IE 10.
|
||||
*/
|
||||
|
||||
img {
|
||||
border-style: none;
|
||||
vertical-align: bottom;
|
||||
}
|
||||
|
||||
embed,
|
||||
object,
|
||||
iframe {
|
||||
border: 0;
|
||||
vertical-align: bottom;
|
||||
}
|
||||
|
||||
/* Forms
|
||||
========================================================================== */
|
||||
|
||||
/**
|
||||
* Reset form fields to make them styleable
|
||||
* 1. Reset radio and checkbox to preserve their look in iOS.
|
||||
*/
|
||||
|
||||
button,
|
||||
input,
|
||||
optgroup,
|
||||
select,
|
||||
textarea {
|
||||
-webkit-appearance: none;
|
||||
appearance: none;
|
||||
vertical-align: middle;
|
||||
color: inherit;
|
||||
font: inherit;
|
||||
border: 0;
|
||||
background: transparent;
|
||||
padding: 0;
|
||||
margin: 0;
|
||||
outline: 0;
|
||||
border-radius: 0;
|
||||
text-align: inherit;
|
||||
}
|
||||
|
||||
[type='checkbox'] {
|
||||
/* 1 */
|
||||
-webkit-appearance: checkbox;
|
||||
appearance: checkbox;
|
||||
}
|
||||
|
||||
[type='radio'] {
|
||||
/* 1 */
|
||||
-webkit-appearance: radio;
|
||||
appearance: radio;
|
||||
}
|
||||
|
||||
/**
|
||||
* Show the overflow in IE.
|
||||
* 1. Show the overflow in Edge.
|
||||
*/
|
||||
|
||||
button,
|
||||
input {
|
||||
/* 1 */
|
||||
overflow: visible;
|
||||
}
|
||||
|
||||
/**
|
||||
* Remove the inheritance of text transform in Edge, Firefox, and IE.
|
||||
* 1. Remove the inheritance of text transform in Firefox.
|
||||
*/
|
||||
|
||||
button,
|
||||
select {
|
||||
/* 1 */
|
||||
text-transform: none;
|
||||
}
|
||||
|
||||
/**
|
||||
* Correct the inability to style clickable types in iOS and Safari.
|
||||
*/
|
||||
|
||||
button,
|
||||
[type='button'],
|
||||
[type='reset'],
|
||||
[type='submit'] {
|
||||
cursor: pointer;
|
||||
-webkit-appearance: none;
|
||||
appearance: none;
|
||||
}
|
||||
|
||||
button[disabled],
|
||||
[type='button'][disabled],
|
||||
[type='reset'][disabled],
|
||||
[type='submit'][disabled] {
|
||||
cursor: default;
|
||||
}
|
||||
|
||||
/**
|
||||
* Remove the inner border and padding in Firefox.
|
||||
*/
|
||||
|
||||
button::-moz-focus-inner,
|
||||
[type='button']::-moz-focus-inner,
|
||||
[type='reset']::-moz-focus-inner,
|
||||
[type='submit']::-moz-focus-inner {
|
||||
border-style: none;
|
||||
padding: 0;
|
||||
}
|
||||
|
||||
/**
|
||||
* Restore the focus styles unset by the previous rule.
|
||||
*/
|
||||
|
||||
button:-moz-focusring,
|
||||
[type='button']:-moz-focusring,
|
||||
[type='reset']:-moz-focusring,
|
||||
[type='submit']:-moz-focusring {
|
||||
outline: 1px dotted ButtonText;
|
||||
}
|
||||
|
||||
/**
|
||||
* Reset to invisible
|
||||
*/
|
||||
|
||||
fieldset {
|
||||
margin: 0;
|
||||
padding: 0;
|
||||
border: 0;
|
||||
min-width: 0;
|
||||
}
|
||||
|
||||
/**
|
||||
* 1. Correct the text wrapping in Edge and IE.
|
||||
* 2. Correct the color inheritance from `fieldset` elements in IE.
|
||||
* 3. Remove the padding so developers are not caught out when they zero out
|
||||
* `fieldset` elements in all browsers.
|
||||
*/
|
||||
|
||||
legend {
|
||||
color: inherit; /* 2 */
|
||||
display: table; /* 1 */
|
||||
max-width: 100%; /* 1 */
|
||||
padding: 0; /* 3 */
|
||||
white-space: normal; /* 1 */
|
||||
}
|
||||
|
||||
/**
|
||||
* Add the correct vertical alignment in Chrome, Firefox, and Opera.
|
||||
*/
|
||||
|
||||
progress {
|
||||
vertical-align: baseline;
|
||||
}
|
||||
|
||||
/**
|
||||
* Remove the default vertical scrollbar in IE 10+.
|
||||
*/
|
||||
|
||||
textarea {
|
||||
overflow: auto;
|
||||
}
|
||||
|
||||
/**
|
||||
* 1. Remove the padding in IE 10.
|
||||
*/
|
||||
|
||||
[type='checkbox'],
|
||||
[type='radio'] {
|
||||
padding: 0; /* 1 */
|
||||
}
|
||||
|
||||
/**
|
||||
* Correct the cursor style of increment and decrement buttons in Chrome.
|
||||
*/
|
||||
|
||||
[type='number']::-webkit-inner-spin-button,
|
||||
[type='number']::-webkit-outer-spin-button {
|
||||
height: auto;
|
||||
}
|
||||
|
||||
/**
|
||||
* 1. Correct the outline style in Safari.
|
||||
*/
|
||||
|
||||
[type='search'] {
|
||||
outline-offset: -2px; /* 1 */
|
||||
}
|
||||
|
||||
/**
|
||||
* Remove the inner padding in Chrome and Safari on macOS.
|
||||
*/
|
||||
|
||||
[type='search']::-webkit-search-decoration {
|
||||
-webkit-appearance: none;
|
||||
}
|
||||
|
||||
/**
|
||||
* 1. Correct the inability to style clickable types in iOS and Safari.
|
||||
* 2. Change font properties to `inherit` in Safari.
|
||||
*/
|
||||
|
||||
::-webkit-file-upload-button {
|
||||
-webkit-appearance: button; /* 1 */
|
||||
font: inherit; /* 2 */
|
||||
}
|
||||
|
||||
/**
|
||||
* Clickable labels
|
||||
*/
|
||||
|
||||
label[for] {
|
||||
cursor: pointer;
|
||||
}
|
||||
|
||||
/* Interactive
|
||||
========================================================================== */
|
||||
|
||||
/*
|
||||
* Add the correct display in Edge, IE 10+, and Firefox.
|
||||
*/
|
||||
|
||||
details {
|
||||
display: block;
|
||||
}
|
||||
|
||||
/*
|
||||
* Add the correct display in all browsers.
|
||||
*/
|
||||
|
||||
summary {
|
||||
display: list-item;
|
||||
}
|
||||
|
||||
/* Table
|
||||
========================================================================== */
|
||||
|
||||
table {
|
||||
border-collapse: collapse;
|
||||
border-spacing: 0;
|
||||
}
|
||||
|
||||
caption {
|
||||
text-align: left;
|
||||
}
|
||||
|
||||
td,
|
||||
th {
|
||||
vertical-align: top;
|
||||
}
|
||||
|
||||
th {
|
||||
text-align: left;
|
||||
font-weight: bold;
|
||||
}
|
||||
|
||||
/* Misc
|
||||
========================================================================== */
|
||||
|
||||
/**
|
||||
* Add the correct display in IE 10+.
|
||||
*/
|
||||
|
||||
template {
|
||||
display: none;
|
||||
}
|
||||
|
||||
/**
|
||||
* Add the correct display in IE 10.
|
||||
*/
|
||||
|
||||
[hidden] {
|
||||
display: none;
|
||||
}
|
||||
|
349
CSS/resets/normalize.css
vendored
Normal file
349
CSS/resets/normalize.css
vendored
Normal file
|
@ -0,0 +1,349 @@
|
|||
/*! normalize.css v8.0.1 | MIT License | github.com/necolas/normalize.css */
|
||||
|
||||
/* Document
|
||||
========================================================================== */
|
||||
|
||||
/**
|
||||
* 1. Correct the line height in all browsers.
|
||||
* 2. Prevent adjustments of font size after orientation changes in iOS.
|
||||
*/
|
||||
|
||||
html {
|
||||
line-height: 1.15; /* 1 */
|
||||
-webkit-text-size-adjust: 100%; /* 2 */
|
||||
}
|
||||
|
||||
/* Sections
|
||||
========================================================================== */
|
||||
|
||||
/**
|
||||
* Remove the margin in all browsers.
|
||||
*/
|
||||
|
||||
body {
|
||||
margin: 0;
|
||||
}
|
||||
|
||||
/**
|
||||
* Render the `main` element consistently in IE.
|
||||
*/
|
||||
|
||||
main {
|
||||
display: block;
|
||||
}
|
||||
|
||||
/**
|
||||
* Correct the font size and margin on `h1` elements within `section` and
|
||||
* `article` contexts in Chrome, Firefox, and Safari.
|
||||
*/
|
||||
|
||||
h1 {
|
||||
font-size: 2em;
|
||||
margin: 0.67em 0;
|
||||
}
|
||||
|
||||
/* Grouping content
|
||||
========================================================================== */
|
||||
|
||||
/**
|
||||
* 1. Add the correct box sizing in Firefox.
|
||||
* 2. Show the overflow in Edge and IE.
|
||||
*/
|
||||
|
||||
hr {
|
||||
box-sizing: content-box; /* 1 */
|
||||
height: 0; /* 1 */
|
||||
overflow: visible; /* 2 */
|
||||
}
|
||||
|
||||
/**
|
||||
* 1. Correct the inheritance and scaling of font size in all browsers.
|
||||
* 2. Correct the odd `em` font sizing in all browsers.
|
||||
*/
|
||||
|
||||
pre {
|
||||
font-family: monospace, monospace; /* 1 */
|
||||
font-size: 1em; /* 2 */
|
||||
}
|
||||
|
||||
/* Text-level semantics
|
||||
========================================================================== */
|
||||
|
||||
/**
|
||||
* Remove the gray background on active links in IE 10.
|
||||
*/
|
||||
|
||||
a {
|
||||
background-color: transparent;
|
||||
}
|
||||
|
||||
/**
|
||||
* 1. Remove the bottom border in Chrome 57-
|
||||
* 2. Add the correct text decoration in Chrome, Edge, IE, Opera, and Safari.
|
||||
*/
|
||||
|
||||
abbr[title] {
|
||||
border-bottom: none; /* 1 */
|
||||
text-decoration: underline; /* 2 */
|
||||
text-decoration: underline dotted; /* 2 */
|
||||
}
|
||||
|
||||
/**
|
||||
* Add the correct font weight in Chrome, Edge, and Safari.
|
||||
*/
|
||||
|
||||
b,
|
||||
strong {
|
||||
font-weight: bolder;
|
||||
}
|
||||
|
||||
/**
|
||||
* 1. Correct the inheritance and scaling of font size in all browsers.
|
||||
* 2. Correct the odd `em` font sizing in all browsers.
|
||||
*/
|
||||
|
||||
code,
|
||||
kbd,
|
||||
samp {
|
||||
font-family: monospace, monospace; /* 1 */
|
||||
font-size: 1em; /* 2 */
|
||||
}
|
||||
|
||||
/**
|
||||
* Add the correct font size in all browsers.
|
||||
*/
|
||||
|
||||
small {
|
||||
font-size: 80%;
|
||||
}
|
||||
|
||||
/**
|
||||
* Prevent `sub` and `sup` elements from affecting the line height in
|
||||
* all browsers.
|
||||
*/
|
||||
|
||||
sub,
|
||||
sup {
|
||||
font-size: 75%;
|
||||
line-height: 0;
|
||||
position: relative;
|
||||
vertical-align: baseline;
|
||||
}
|
||||
|
||||
sub {
|
||||
bottom: -0.25em;
|
||||
}
|
||||
|
||||
sup {
|
||||
top: -0.5em;
|
||||
}
|
||||
|
||||
/* Embedded content
|
||||
========================================================================== */
|
||||
|
||||
/**
|
||||
* Remove the border on images inside links in IE 10.
|
||||
*/
|
||||
|
||||
img {
|
||||
border-style: none;
|
||||
}
|
||||
|
||||
/* Forms
|
||||
========================================================================== */
|
||||
|
||||
/**
|
||||
* 1. Change the font styles in all browsers.
|
||||
* 2. Remove the margin in Firefox and Safari.
|
||||
*/
|
||||
|
||||
button,
|
||||
input,
|
||||
optgroup,
|
||||
select,
|
||||
textarea {
|
||||
font-family: inherit; /* 1 */
|
||||
font-size: 100%; /* 1 */
|
||||
line-height: 1.15; /* 1 */
|
||||
margin: 0; /* 2 */
|
||||
}
|
||||
|
||||
/**
|
||||
* Show the overflow in IE.
|
||||
* 1. Show the overflow in Edge.
|
||||
*/
|
||||
|
||||
button,
|
||||
input { /* 1 */
|
||||
overflow: visible;
|
||||
}
|
||||
|
||||
/**
|
||||
* Remove the inheritance of text transform in Edge, Firefox, and IE.
|
||||
* 1. Remove the inheritance of text transform in Firefox.
|
||||
*/
|
||||
|
||||
button,
|
||||
select { /* 1 */
|
||||
text-transform: none;
|
||||
}
|
||||
|
||||
/**
|
||||
* Correct the inability to style clickable types in iOS and Safari.
|
||||
*/
|
||||
|
||||
button,
|
||||
[type="button"],
|
||||
[type="reset"],
|
||||
[type="submit"] {
|
||||
-webkit-appearance: button;
|
||||
}
|
||||
|
||||
/**
|
||||
* Remove the inner border and padding in Firefox.
|
||||
*/
|
||||
|
||||
button::-moz-focus-inner,
|
||||
[type="button"]::-moz-focus-inner,
|
||||
[type="reset"]::-moz-focus-inner,
|
||||
[type="submit"]::-moz-focus-inner {
|
||||
border-style: none;
|
||||
padding: 0;
|
||||
}
|
||||
|
||||
/**
|
||||
* Restore the focus styles unset by the previous rule.
|
||||
*/
|
||||
|
||||
button:-moz-focusring,
|
||||
[type="button"]:-moz-focusring,
|
||||
[type="reset"]:-moz-focusring,
|
||||
[type="submit"]:-moz-focusring {
|
||||
outline: 1px dotted ButtonText;
|
||||
}
|
||||
|
||||
/**
|
||||
* Correct the padding in Firefox.
|
||||
*/
|
||||
|
||||
fieldset {
|
||||
padding: 0.35em 0.75em 0.625em;
|
||||
}
|
||||
|
||||
/**
|
||||
* 1. Correct the text wrapping in Edge and IE.
|
||||
* 2. Correct the color inheritance from `fieldset` elements in IE.
|
||||
* 3. Remove the padding so developers are not caught out when they zero out
|
||||
* `fieldset` elements in all browsers.
|
||||
*/
|
||||
|
||||
legend {
|
||||
box-sizing: border-box; /* 1 */
|
||||
color: inherit; /* 2 */
|
||||
display: table; /* 1 */
|
||||
max-width: 100%; /* 1 */
|
||||
padding: 0; /* 3 */
|
||||
white-space: normal; /* 1 */
|
||||
}
|
||||
|
||||
/**
|
||||
* Add the correct vertical alignment in Chrome, Firefox, and Opera.
|
||||
*/
|
||||
|
||||
progress {
|
||||
vertical-align: baseline;
|
||||
}
|
||||
|
||||
/**
|
||||
* Remove the default vertical scrollbar in IE 10+.
|
||||
*/
|
||||
|
||||
textarea {
|
||||
overflow: auto;
|
||||
}
|
||||
|
||||
/**
|
||||
* 1. Add the correct box sizing in IE 10.
|
||||
* 2. Remove the padding in IE 10.
|
||||
*/
|
||||
|
||||
[type="checkbox"],
|
||||
[type="radio"] {
|
||||
box-sizing: border-box; /* 1 */
|
||||
padding: 0; /* 2 */
|
||||
}
|
||||
|
||||
/**
|
||||
* Correct the cursor style of increment and decrement buttons in Chrome.
|
||||
*/
|
||||
|
||||
[type="number"]::-webkit-inner-spin-button,
|
||||
[type="number"]::-webkit-outer-spin-button {
|
||||
height: auto;
|
||||
}
|
||||
|
||||
/**
|
||||
* 1. Correct the odd appearance in Chrome and Safari.
|
||||
* 2. Correct the outline style in Safari.
|
||||
*/
|
||||
|
||||
[type="search"] {
|
||||
-webkit-appearance: textfield; /* 1 */
|
||||
outline-offset: -2px; /* 2 */
|
||||
}
|
||||
|
||||
/**
|
||||
* Remove the inner padding in Chrome and Safari on macOS.
|
||||
*/
|
||||
|
||||
[type="search"]::-webkit-search-decoration {
|
||||
-webkit-appearance: none;
|
||||
}
|
||||
|
||||
/**
|
||||
* 1. Correct the inability to style clickable types in iOS and Safari.
|
||||
* 2. Change font properties to `inherit` in Safari.
|
||||
*/
|
||||
|
||||
::-webkit-file-upload-button {
|
||||
-webkit-appearance: button; /* 1 */
|
||||
font: inherit; /* 2 */
|
||||
}
|
||||
|
||||
/* Interactive
|
||||
========================================================================== */
|
||||
|
||||
/*
|
||||
* Add the correct display in Edge, IE 10+, and Firefox.
|
||||
*/
|
||||
|
||||
details {
|
||||
display: block;
|
||||
}
|
||||
|
||||
/*
|
||||
* Add the correct display in all browsers.
|
||||
*/
|
||||
|
||||
summary {
|
||||
display: list-item;
|
||||
}
|
||||
|
||||
/* Misc
|
||||
========================================================================== */
|
||||
|
||||
/**
|
||||
* Add the correct display in IE 10+.
|
||||
*/
|
||||
|
||||
template {
|
||||
display: none;
|
||||
}
|
||||
|
||||
/**
|
||||
* Add the correct display in IE 10.
|
||||
*/
|
||||
|
||||
[hidden] {
|
||||
display: none;
|
||||
}
|
54
CSS/resets/reset.css
Normal file
54
CSS/resets/reset.css
Normal file
|
@ -0,0 +1,54 @@
|
|||
/* http://meyerweb.com/eric/tools/css/reset/
|
||||
v2.0 | 20110126
|
||||
License: none (public domain)
|
||||
*/
|
||||
|
||||
html, body, div, span, applet, object, iframe,
|
||||
h1, h2, h3, h4, h5, h6, p, blockquote, pre,
|
||||
a, abbr, acronym, address, big, cite, code,
|
||||
del, dfn, em, img, ins, kbd, q, s, samp,
|
||||
small, strike, strong, sub, sup, tt, var,
|
||||
b, u, i, center,
|
||||
dl, dt, dd, ol, ul, li,
|
||||
fieldset, form, label, legend,
|
||||
table, caption, tbody, tfoot, thead, tr, th, td,
|
||||
article, aside, canvas, details, embed,
|
||||
figure, figcaption, footer, header, hgroup,
|
||||
menu, nav, output, ruby, section, summary,
|
||||
time, mark, audio, video {
|
||||
margin: 0;
|
||||
padding: 0;
|
||||
border: 0;
|
||||
font-size: 100%;
|
||||
font: inherit;
|
||||
vertical-align: baseline;
|
||||
}
|
||||
|
||||
/* HTML5 display-role reset for older browsers */
|
||||
article, aside, details, figcaption, figure,
|
||||
footer, header, hgroup, menu, nav, section {
|
||||
display: block;
|
||||
}
|
||||
|
||||
body {
|
||||
line-height: 1;
|
||||
}
|
||||
|
||||
ol, ul {
|
||||
list-style: none;
|
||||
}
|
||||
|
||||
blockquote, q {
|
||||
quotes: none;
|
||||
}
|
||||
|
||||
blockquote:before, blockquote:after,
|
||||
q:before, q:after {
|
||||
content: '';
|
||||
content: none;
|
||||
}
|
||||
|
||||
table {
|
||||
border-collapse: collapse;
|
||||
border-spacing: 0;
|
||||
}
|
409
Database/MongoDB.md
Normal file
409
Database/MongoDB.md
Normal file
|
@ -0,0 +1,409 @@
|
|||
# MongoDB Cheat Sheet
|
||||
|
||||
## Terminologia & concetti base
|
||||
|
||||
Il database è un contenitore di **collezioni** (tabelle in DB relazionali). Le collezioni sono mini contenitori di **documenti** (record in DB relazionali).
|
||||
|
||||
I documenti sono *schema-less* ovvero hanno una struttura dinamica ed essa può cambiare tra documenti all'interno della stessa collezione.
|
||||
La struttura di un documento è quella del JSON.
|
||||
|
||||
### Tipi di dati
|
||||
|
||||
| Tipo | Documento | Funzione |
|
||||
|-------------------|------------------------------------------------|-------------------------|
|
||||
| Text | `"Text"` |
|
||||
| Boolean | `true` |
|
||||
| Number | `42` |
|
||||
| Objectid | `"_id": {"$oid": "<id>"}` | `ObjectId("<id>")` |
|
||||
| ISODate | `"key": {"$date": "YYYY-MM-DDThh:mm:ss.sssZ"}` | `ISODate("YYYY-MM-DD")` |
|
||||
| Timestamp | | `Timestamp(11421532)` |
|
||||
| Embedded Document | `{"a": {...}}` |
|
||||
| Embedded Array | `{"b": [...]}` |
|
||||
|
||||
E' obbligatorio per ogni documento avere un campo `_id` univoco.
|
||||
MongoDB di occupa di creare un `ObjectId()` in automatico.
|
||||
|
||||
### Uso Database
|
||||
|
||||
Per creare un database è sufficiente effettuare uno switch verso un db non-esistente (creazione implicita): `use [database]`
|
||||
il db non viene creato finchè non si inserisce un dato.
|
||||
|
||||
```sh
|
||||
show dbs # list all databases
|
||||
use <database> # use a particular database
|
||||
show collections # list all collection for the current database
|
||||
|
||||
dbs.dropDatabase() # delete current database
|
||||
```
|
||||
|
||||
## Uso Collezioni
|
||||
|
||||
```sh
|
||||
db.createCollection(name, {options}) # creazione collezione
|
||||
db.<collection>.insertOne({document}) # creazione implicita collezione
|
||||
```
|
||||
|
||||
## Operazioni CRUD
|
||||
|
||||
### Filters
|
||||
|
||||
Base Syntax: `{ "outerKey.innerKey": "value" }`
|
||||
Comparison: `{ key: { $operator : "value"} }`
|
||||
|
||||
| Operator | Math Symbol |
|
||||
|----------|-------------|
|
||||
| `$gt` | > |
|
||||
| `$gte` | => |
|
||||
| `$lt` | < |
|
||||
| `$lte` | <= |
|
||||
| `$eq` | == |
|
||||
| `$ne` | != |
|
||||
|
||||
Field Exists: `{ key: {$exists: true} }`
|
||||
Logical `Or`: `{ $or: [ {filter_1}, {filter_2}, ... ] }`
|
||||
Membership: `{ key: { $in: [value_1, value_2, ...] } }` or `{ key: { $nin: [value_1, value_2, ...] } }`
|
||||
|
||||
### Create
|
||||
|
||||
È possibile inserire documenti con il comando `insertOne()` (un documento alla volta) o `insertMany()` (più documenti).
|
||||
|
||||
Risultati inserimento:
|
||||
|
||||
- errore -> rollback
|
||||
- successo -> salvataggio intero documneto
|
||||
|
||||
```sh
|
||||
# explicit collection creation, all options are otional
|
||||
db.createCollection( <name>,
|
||||
{
|
||||
capped: <boolean>,
|
||||
autoIndexId: <boolean>,
|
||||
size: <number>,
|
||||
max: <number>,
|
||||
storageEngine: <document>,
|
||||
validator: <document>,
|
||||
validationLevel: <string>,
|
||||
validationAction: <string>,
|
||||
indexOptionDefaults: <document>,
|
||||
viewOn: <string>,
|
||||
pipeline: <pipeline>,
|
||||
collation: <document>,
|
||||
writeConcern: <document>
|
||||
}
|
||||
)
|
||||
|
||||
db.createCollection("name", { capped: true, size: max_bytes, max: max_docs_num } ) # creation of a capped collection
|
||||
# SIZE: int - will be rounded to a multiple of 256
|
||||
|
||||
# implicit creation at doc insertion
|
||||
db.<collection>.insertOne({ document }, options) # insert a document in a collection
|
||||
db.<collection>.insertMany([ { document }, { document }, ... ], options) # insert multiple docs
|
||||
db.<collection>.insert()
|
||||
```
|
||||
|
||||
Se `insertMany()` causa un errore il processo di inserimento si arresta. Non viene eseguito il rollback dei documenti già inseriti.
|
||||
|
||||
### Read
|
||||
|
||||
```sh
|
||||
db.<collection>.findOne() # find only one document
|
||||
db.<collection>.find(filter) # show selected documents
|
||||
db.<collection>.find(filter, {key: 1}) # show selected values form documents (1 or true => show, 0 or false => dont show, cant mix 0 and 1)
|
||||
db.<collection>.find(filter, {_id: 0, key: 1}) # only _id can be set to 0 with other keys at 1
|
||||
db.<collection>.find().pretty() # show documents formatted
|
||||
db.<collection>.find().limit(n) # show n documents
|
||||
db.<collection>.find().limit(n).skip(k) # show n documents skipping k docs
|
||||
db.<collection>.find().count() # number of found docs
|
||||
db.<collection>.find().sort({key1: 1, ... , key_n: -1}) # show documents sorted by specified keys in ascending (1) or descending (-1) order
|
||||
|
||||
# GeoJSON - https://docs.mongodb.com/manual/reference/operator/query/near/index.html
|
||||
db.<collection>.find(
|
||||
{
|
||||
<location field>: {
|
||||
$near: {
|
||||
$geometry: { type: "Point", coordinates: [ <longitude> , <latitude> ] },
|
||||
$maxDistance: <distance in meters>,
|
||||
$minDistance: <distance in meters>
|
||||
}
|
||||
}
|
||||
}
|
||||
)
|
||||
|
||||
db.<collection>.find().hint( { <field>: 1 } ) # specify the index
|
||||
db.<collection>.find().hint( "index-name" ) # specify the index using the index name
|
||||
|
||||
db.<collection>.find().hint( { $natural : 1 } ) # force the query to perform a forwards collection scan
|
||||
db.<collection>.find().hint( { $natural : -1 } ) # force the query to perform a reverse collection scan
|
||||
```
|
||||
|
||||
### Update
|
||||
|
||||
[Update Operators](https://docs.mongodb.com/manual/reference/operator/update/ "Update Operators Documentation")
|
||||
|
||||
```sh
|
||||
db.<collection>.updateOne(filter, $set: {key: value}) # add or modify values
|
||||
db.<collection>.updateOne(filter, $set: {key: value}, {upsert: true}) # add or modify values, if attribute doesent exists create it
|
||||
|
||||
db.<collection>.updateMany(filter, update)
|
||||
|
||||
db.<collection>.replaceOne(filter, { document }, options)
|
||||
```
|
||||
|
||||
### Delete
|
||||
|
||||
```sh
|
||||
db.<collection>.deleteOne(filter, options)
|
||||
db.<collection>.deleteMany(filter, options)
|
||||
|
||||
db.<collection>.drop() # delete whole collection
|
||||
db.dropDatabase() # delete entire database
|
||||
```
|
||||
|
||||
## Mongoimport Tool
|
||||
|
||||
Utility to import all docs into a specified collection.
|
||||
If the collection alredy exists `--drop` deletes it before reuploading it.
|
||||
**WARNING**: CSV separators must be commas (`,`)
|
||||
|
||||
```sh
|
||||
mongoimport -h <host:port> –d <database> –c <collection> --drop --jsonArray <souce_file>
|
||||
|
||||
mongoimport --host <HOST:PORT> --ssl --username <USERNAME> --password <PASSWORD> --authenticationDatabase admin --db <DATABASE> --collection <COLLECTION> --type <FILETYPE> --file <FILENAME>
|
||||
|
||||
# if file is CSV and first line is header
|
||||
mongoimport ... --haderline
|
||||
```
|
||||
|
||||
## Mongoexport Tool
|
||||
|
||||
Utility to export documents into a specified file.
|
||||
|
||||
```sh
|
||||
mongoexport -h <host:port> –d <database> –c <collection> <souce_file>
|
||||
|
||||
mongoexport --host <host:port> --ssl --username <username> --password <PASSWORD> --authenticationDatabase admin --db <DATABASE> --collection <COLLECTION> --type <FILETYPE> --out <FILENAME>
|
||||
```
|
||||
|
||||
## Mongodump & Mongorestore
|
||||
|
||||
`mongodump` exports the content of a running server into `.bson` files.
|
||||
|
||||
`mongorestore` Restore backups generated with `mongodump` to a running server.
|
||||
|
||||
## Relations
|
||||
|
||||
**Nested / Embedded Documents**:
|
||||
|
||||
- Group data locically
|
||||
- Optimal for data belonging together that do not overlap
|
||||
- Should avoid nesting too deep or making too long arrays (max doc size 16 mb)
|
||||
|
||||
```json
|
||||
{
|
||||
_id: Objectid()
|
||||
key: "value"
|
||||
key: "value"
|
||||
|
||||
innerDocument: {
|
||||
key: "value"
|
||||
key: "value"
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
**References**:
|
||||
|
||||
- Divide data between collections
|
||||
- Optimal for related but shared data used in relations or stand-alone
|
||||
- Allows to overtake nidification and size limits
|
||||
|
||||
NoSQL databases do not have relations and references. It's the app that has to handle them.
|
||||
|
||||
```json
|
||||
{
|
||||
key: "value"
|
||||
references: ["id1", "id2"]
|
||||
}
|
||||
|
||||
// referenced
|
||||
{
|
||||
_id: "id1"
|
||||
key: "value"
|
||||
}
|
||||
```
|
||||
|
||||
## [Indexes](https://docs.mongodb.com/manual/indexes/ "Index Documentation")
|
||||
|
||||
Indexes support the efficient execution of queries in MongoDB.
|
||||
|
||||
Without indexes, MongoDB must perform a *collection scan* (*COLLSCAN*): scan every document in a collection, to select those documents that match the query statement.
|
||||
If an appropriate index exists for a query, MongoDB can use the index to limit the number of documents it must inspect (*IXSCAN*).
|
||||
|
||||
Indexes are special data structures that store a small portion of the collection’s data set in an easy to traverse form. The index stores the value of a specific field or set of fields, ordered by the value of the field. The ordering of the index entries supports efficient equality matches and range-based query operations. In addition, MongoDB can return sorted results by using the ordering in the index.
|
||||
|
||||
Indexes *slow down writing operations* since the index must be updated at every writing.
|
||||
|
||||
 using an index")
|
||||
|
||||
### [Index Types](https://docs.mongodb.com/manual/indexes/#index-types)
|
||||
|
||||
- **Normal**: Fields sorted by name
|
||||
- **Compound**: Multiple Fields sorted by name
|
||||
- **Multykey**: values of sorted arrays
|
||||
- **Text**: Ordered text fragments
|
||||
- **Geospatial**: ordered geodata
|
||||
|
||||
**Sparse** indexes only contain entries for documents that have the indexed field, even if the index field contains a null value. The index skips over any document that is missing the indexed field.
|
||||
|
||||
### Diagnosys and query planning
|
||||
|
||||
```sh
|
||||
db.<collection>.find({...}).explain() # explain won't accept other functions
|
||||
db.explain().<collection>.find({...}) # can accept other functions
|
||||
db.explain("executionStats").<collection>.find({...}) # more info
|
||||
```
|
||||
|
||||
### Index Creation
|
||||
|
||||
```sh
|
||||
db.<collection>.createIndex( <key and index type specification>, <options> )
|
||||
|
||||
db.<collection>.createIndex( { <field>: <type>, <field>: <type>, ... } ) # normal, compound or multikey (field is array) index
|
||||
db.<collection>.createIndex( { <field>: "text" } ) # text index
|
||||
db.<collection>.createIndex( { <field>: 2dsphere } ) # geospatial 2dsphere index
|
||||
|
||||
# sparse index
|
||||
db.<collection>.createIndex(
|
||||
{ <field>: <type>, <field>: <type>, ... },
|
||||
{ sparse: true } # sparse option
|
||||
)
|
||||
|
||||
# custom name
|
||||
db.<collection>.createIndex(
|
||||
{ <key and index type specification>, },
|
||||
{ name: "index-name" } # name option
|
||||
)
|
||||
```
|
||||
|
||||
### [Index Management](https://docs.mongodb.com/manual/tutorial/manage-indexes/)
|
||||
|
||||
```sh
|
||||
# view all db indexes
|
||||
db.getCollectionNames().forEach(function(collection) {
|
||||
indexes = db[collection].getIndexes();
|
||||
print("Indexes for " + collection + ":");
|
||||
printjson(indexes);
|
||||
});
|
||||
db.<collection>.getIndexes() # view collenction's index
|
||||
|
||||
db.<collection>.dropIndexes() # drop all indexes
|
||||
db.<collection>.dropIndex( { "index-name": 1 } ) # drop a specific index
|
||||
```
|
||||
|
||||
## Database Profiling
|
||||
|
||||
Profiling Levels:
|
||||
|
||||
- `0`: no profiling
|
||||
- `1`: data on operations slower than `slowms`
|
||||
- `2`: data on all operations
|
||||
|
||||
Logs are saved in the `system.profile` *capped* collection.
|
||||
|
||||
```sh
|
||||
db.setProgilingLevel(n) # set profiler level
|
||||
db.setProfilingLevel(1, { slowms: <ms> })
|
||||
db.getProfilingStatus() # check profiler satus
|
||||
|
||||
db.system.profile.find().limit(n).sort( {} ).pretty() # see logs
|
||||
db.system.profile.find().limit(n).sort( { ts : -1 } ).pretty() # sort by decreasing timestamp
|
||||
```
|
||||
|
||||
## Roles and permissions
|
||||
|
||||
**Authentication**: identifies valid users
|
||||
**Authorization**: identifies what a user can do
|
||||
|
||||
- **userAdminAnyDatabase**: can admin every db in the istance (role must be created on admin db)
|
||||
- **userAdmin**: can admin the specific db in which is created
|
||||
- **readWrite**: can read and write in the specific db in which is created
|
||||
- **read**: can read the specific db in which is created
|
||||
|
||||
```sh
|
||||
# create users in the current MongoDB instance
|
||||
db.createUser(
|
||||
{
|
||||
user: "dbAdmin",
|
||||
pwd: "password",
|
||||
roles:[
|
||||
{
|
||||
role: "userAdminAnyDatabase",
|
||||
db:"admin"
|
||||
}
|
||||
]
|
||||
},
|
||||
{
|
||||
user: "username",
|
||||
pwd: "password",
|
||||
roles:[
|
||||
{
|
||||
role: "role",
|
||||
db: "database"
|
||||
}
|
||||
]
|
||||
}
|
||||
)
|
||||
```
|
||||
|
||||
## Sharding
|
||||
|
||||
**Sharding** is a MongoDB concept through which big datasests are subdivided in smaller sets and distribuited towards multiple instances of MongoDB.
|
||||
It's a technique used to improve the performances of large queries towards large quantities of data that require al lot of resources from the server.
|
||||
|
||||
A collection containing several documents is splitted in more smaller collections (*shards*)
|
||||
Shards are implemented via cluster that are none other a group of MongoDB instances.
|
||||
|
||||
Shard components are:
|
||||
|
||||
- Shards (min 2), instances of MongoDB that contain a subset of the data
|
||||
- A config server, instasnce of MongoDB which contains metadata on the cluster, that is the set of instances that have the shard data.
|
||||
- A router (or `mongos`), instance of MongoDB used to redirect the user instructions from the client to the correct server.
|
||||
|
||||

|
||||
|
||||
### [Replica set](https://docs.mongodb.com/manual/replication/)
|
||||
|
||||
A **replica set** in MongoDB is a group of `mongod` processes that maintain the `same dataset`. Replica sets provide redundancy and high availability, and are the basis for all production deployments.
|
||||
|
||||
## Aggregations
|
||||
|
||||
Sequence of operations applied to a collection as a *pipeline* to get a result: `db.collection.aggregate(pipeline, options)`.
|
||||
|
||||
[Aggragations Stages][AggrStgs]:
|
||||
|
||||
- `$lookup`: Right Join
|
||||
- `$match`: Where
|
||||
- `$sort`: Order By
|
||||
- `$project`: Select *
|
||||
- ...
|
||||
|
||||
[AggrStgs]: https://docs.mongodb.com/manual/reference/operator/aggregation-pipeline/
|
||||
|
||||
Example:
|
||||
|
||||
```sh
|
||||
db.collection.aggregate([
|
||||
{
|
||||
$lookup: {
|
||||
from: <collection to join>,
|
||||
localField: <field from the input documents>,
|
||||
foreignField: <field from the documents of the "from" collection>,
|
||||
as: <output array field>
|
||||
}
|
||||
},
|
||||
{ $match: { <query> } },
|
||||
{ $sort: { ... } },
|
||||
{ $project: { ... } },
|
||||
{ ... }
|
||||
])
|
||||
```
|
109
Database/Redis.md
Normal file
109
Database/Redis.md
Normal file
|
@ -0,0 +1,109 @@
|
|||
# [Redis](https://redis.io/)
|
||||
|
||||
Redis is in the family of databases called **key-value stores**.
|
||||
|
||||
The essence of a key-value store is the ability to store some data, called a value, inside a key. This data can later be retrieved only if we know the exact key used to store it.
|
||||
|
||||
Often Redis it is called a *data structure* server because it has outer key-value shell, but each value can contain a complex data structure, such as a string, a list, a hashes, or ordered data structures called sorted sets as well as probabilistic data structures like *hyperloglog*.
|
||||
|
||||
## [Redis Commands](https://redis.io/commands)
|
||||
|
||||
### Server Startup
|
||||
|
||||
```bash
|
||||
redis-server # start the server
|
||||
redis-cli
|
||||
```
|
||||
|
||||
### [Key-Value Pairs](https://redis.io/commands#generic)
|
||||
|
||||
```sh
|
||||
SET <key> <value> [ EX <seconds> ] # store a key-value pair, TTL optional
|
||||
GET <key> # read a key content
|
||||
EXISTS <key> # check if a key exists
|
||||
DEL <key> # delete a key-value pair
|
||||
|
||||
INCR <key> # atomically increment a number stored at a given key
|
||||
INCRBY <key> <amount> # increment the number contained inside a key by a specific amount
|
||||
DECR <key>
|
||||
DECRBY <key> <amount>
|
||||
|
||||
# re-setting the key will make it permanent (TTL -1)
|
||||
EXPIRE <key> <seconds> # make the key expire after <second> seconds
|
||||
TTL <key> # see remaining seconds before expiry
|
||||
PEXPIRE <key> <seconds> # make the key expire after <second> milli-seconds
|
||||
PTTL <key> # see remaining milli-seconds before expiry
|
||||
PERSIST <key> # make the key permanent
|
||||
```
|
||||
|
||||
### [Lists](https://redis.io/commands#list)
|
||||
|
||||
A list is a series of ordered values.
|
||||
|
||||
```sh
|
||||
RPUSH <key> <value1> <value2> ... # add one or more values to the end of the list
|
||||
LPUSH <key> <value1> <value2> ... # add one or more values to the start of a list
|
||||
|
||||
LLEN # number of items in the list
|
||||
LRANGE <key> <start_index> <end_index> # return a subset of the list, end index included. Negative indexes caout backwards from the end
|
||||
|
||||
LPOP # remove and return the first item fro the list
|
||||
RPOP # remove and return the last item fro the list
|
||||
```
|
||||
|
||||
### [Sets](https://redis.io/commands#set)
|
||||
|
||||
A set is similar to a list, except it does not have a specific order and each element may only appear once.
|
||||
|
||||
```sh
|
||||
SADD <key> <value1> <value2> ... # add one or more values to the set (retunr 0 if values are alredy inside)
|
||||
SREM <key> <value> # remove the given member from the set, return 1 or 0 to signal if the member was actually there or not.
|
||||
SPOP <key> <value> # remove and return value from the set
|
||||
|
||||
SISMEMBER <key> <value> # test if value is in the set
|
||||
SMEMBERS <key> # lis of all set items
|
||||
|
||||
SUINION <key1> <key2> ... # combine two or more sets and return the list of all elements.
|
||||
```
|
||||
|
||||
### [Sorted Sets](https://redis.io/commands#sorted_set)
|
||||
|
||||
Sets are a very handy data type, but as they are unsorted they don't work well for a number of problems. This is why Redis 1.2 introduced Sorted Sets.
|
||||
|
||||
A sorted set is similar to a regular set, but now each value has an associated score. This score is used to sort the elements in the set.
|
||||
|
||||
```sh
|
||||
ZADD <key> <score> <value> # add a value with it's score
|
||||
|
||||
ZRANGE <key> <start_index> <end_index> # return a subset of the sortedSet
|
||||
|
||||
...
|
||||
```
|
||||
|
||||
### [Hashes](https://redis.io/commands#hash)
|
||||
|
||||
Hashes are maps between string fields and string values, so they are the perfect data type to represent objects.
|
||||
|
||||
```sh
|
||||
HSET <key> <field> <value> [ <field> <value> ... ] # set the string of a hash field
|
||||
HSETNX <key> <field> <value> # set the value of a hash field, only if the field does not exist
|
||||
|
||||
HEXISTS <key> <field> # determine if a hash field exists
|
||||
|
||||
HLEN <key> # get the number of fields in a hash
|
||||
HSTRLEN <key> <field> # get the length of the value of a hash field
|
||||
HGETALL <key> # get all fields and values in a hash
|
||||
HGET <key> <field> # get data on a single field
|
||||
HKEYS <key> # get all the fields in a hash
|
||||
HVALS <key> # get all the values in a hash
|
||||
|
||||
HDEL <key> <field_1> <field_2> ... # delete one or more field hases
|
||||
|
||||
HMGET <key> <field> [<field> ...] # get the values of all the given hash fields
|
||||
HMSET <key> <field> <value> [<field> <value> ...] # set multiple hash fields to multiple values
|
||||
|
||||
HINCRBY <key> <field> <amount> # increment the integer value of a hash field by the given number
|
||||
HINCRBYFLOAT <key> <field> <amount> # increment the float value of a hash field by the given amount
|
||||
|
||||
HSCAN <key> <cursor> [MATCH <pattern>] [COUNT <count>] # incrementally iterate hash fields and associated values
|
||||
```
|
284
Database/SQL.md
Normal file
284
Database/SQL.md
Normal file
|
@ -0,0 +1,284 @@
|
|||
# SQL
|
||||
|
||||
`mysql -u root`: avvio mysql come utente root
|
||||
|
||||
## DDL
|
||||
|
||||
```sql
|
||||
show databases; -- mostra database
|
||||
CREATE DATABASE <database>; -- dataabse creation
|
||||
use <database_name>; -- usa un database particolare
|
||||
exit; -- exit mysql
|
||||
|
||||
show tables; -- mostra tabelle del database
|
||||
|
||||
-- INLINE COMMENT
|
||||
/* MULTI-LINE COMMENT */
|
||||
```
|
||||
|
||||
### Table Creation
|
||||
|
||||
```sql
|
||||
CREATE TABLE <table_name>
|
||||
(<field_name> <field_type> <option>,
|
||||
...);
|
||||
```
|
||||
|
||||
### PRIMARY KEY from multiple fields
|
||||
|
||||
```sql
|
||||
CREATE TABLE <table_name>(
|
||||
...,
|
||||
PRIMARY KEY (<field1>, ...),
|
||||
);
|
||||
```
|
||||
|
||||
### Table Field Options
|
||||
|
||||
```sql
|
||||
PRIMARY KEY -- marks primary key as field option
|
||||
NOT NULL -- marks a necessary field
|
||||
REFERENCES <table> (<field>) -- adds foreign key reference
|
||||
UNIQUE (<field>) -- set field as unique (MySQL)
|
||||
<field> UNIQUE -- T-SQL
|
||||
```
|
||||
|
||||
### Table Modification
|
||||
|
||||
```sql
|
||||
ALTER TABLE <table>
|
||||
ADD PRIMARY KEY (<field>, ...), -- definition of PK after table creation
|
||||
ADD <field_name> <field_type> <option>; -- addition of a new field, field will have no value in the table
|
||||
|
||||
ALTER TABLE <table>
|
||||
CHANGE <field_name> <new_name> <new_type>;
|
||||
ALTER COLUMN <field_name> <new_name> <new_type>; -- T-SQL
|
||||
|
||||
ALTER TABLE <table>
|
||||
DROP <field>;
|
||||
|
||||
ALTER TABLE <table>
|
||||
ADD FOREIGN KEY (<field>) REFERENCES <TABLE> (<FIELD>);
|
||||
```
|
||||
|
||||
## DML
|
||||
|
||||
### Data Insertion
|
||||
|
||||
```sql
|
||||
INSERT INTO <table> (field_1, ...) VALUES (value_1, ...), (value_1, ...);
|
||||
INSERT INTO <table> VALUES (value_1, ...), (value_1, ...); -- field order MUST respest tables's columns order
|
||||
```
|
||||
|
||||
### Data Update
|
||||
|
||||
```sql
|
||||
UPDATE <table> SET <field> = <value>, <field> = <value>, ... WHERE <condition>;
|
||||
```
|
||||
|
||||
### Data Elimination
|
||||
|
||||
```sql
|
||||
DELETE FROM <table> WHERE <condition>
|
||||
DELETE FROM <table> -- empty the table
|
||||
```
|
||||
|
||||
## Data Selection
|
||||
|
||||
`*` Indica tutti i campi
|
||||
|
||||
```sql
|
||||
SELECT * FROM <table>; -- show table contents
|
||||
SHOW columns FROM <table>; -- show table columns
|
||||
DESCRIBE <table>; -- shows table
|
||||
```
|
||||
|
||||
### Alias Tabelle
|
||||
|
||||
```sql
|
||||
SELECT <field/funzione> as <alias>; -- mostra <field/funzione> con nome <alias>
|
||||
```
|
||||
|
||||
### Selezione Condizionale
|
||||
|
||||
```sql
|
||||
SELECT * FROM <table> WHERE <condition>; -- mostra elementi che rispettano la condizione
|
||||
AND, OR, NOT -- connettivi logici
|
||||
|
||||
SELECT * FROM <table> WHERE <field> Between <value_1> AND <value_2>;
|
||||
```
|
||||
|
||||
### Ordinamento
|
||||
|
||||
```sql
|
||||
SELECT * FROM <table> ORDER BY <field>, ...; -- mostra tabella ordinata in base a colonna <field>
|
||||
SELECT * FROM <table> ORDER BY <field>, ... DESC; -- mostra tabella ordinata decrescente in base a colonna <field>
|
||||
SELECT * FROM <table> ORDER BY <field>, ... LIMIT n; -- mostra tabella ordinata in base a colonna <field>, mostra n elementi
|
||||
SELECT TOP(n) * FROM <table> ORDER BY <field>, ...; -- T-SQL
|
||||
```
|
||||
|
||||
## Raggruppamento
|
||||
|
||||
```sql
|
||||
SELECT * FROM <table> GROUP BY <field>;
|
||||
SELECT * FROM <table> GROUP BY <field> HAVING <condition>;
|
||||
SELECT DISTINCT <field> FROM <table>; -- mostra elementi senza riperizioni
|
||||
```
|
||||
|
||||
### Ricerca caratteri in valori
|
||||
|
||||
`%` 0+ caratteri
|
||||
|
||||
```sql
|
||||
SELECT * FROM <table> WHERE <field> LIKE '<char>%'; -- seleziona elemnti in <field> inizianti per <char>
|
||||
SELECT * FROM <table> WHERE <field> LIKE '%<char>'; -- seleziona elemnti in <field> terminanti per <char>
|
||||
SELECT * FROM <table> WHERE <field> LIKE '%<char>%'; -- seleziona elemnti in <field> contenenti <char>
|
||||
SELECT * FROM <table> WHERE <field> NOT LIKE '%<char>%'; -- seleziona elemnti in <field> non contenenti <char>
|
||||
```
|
||||
|
||||
### Selection from multiple tables
|
||||
|
||||
```sql
|
||||
SELECT a.<field>, b.<field> FROM <table> AS a, <table> AS b
|
||||
WHERE a.<field> ...;
|
||||
```
|
||||
|
||||
## Funzioni
|
||||
|
||||
```sql
|
||||
SELECT COUNT(*) FROM <field>; -- conta numero elemneti nel campo
|
||||
SELECT MIN(*) FROM <table>; -- restituisce il valore minimo
|
||||
SELECT MAX(*) FROM <table>; -- restituisce valore massimo
|
||||
SELECT AVG(*) FROM <table>; -- media valori del campo
|
||||
ALL (SELECT ...)
|
||||
ANY (SELECT ...)
|
||||
```
|
||||
|
||||
## Query Annidate
|
||||
|
||||
```sql
|
||||
SELECT * FROM <table> WHERE EXISTS (SELECT * FROM <table>) -- selected field existing in subquery
|
||||
SELECT * FROM <table> WHERE NOT EXISTS (SELECT * FROM <table>) -- selected field not existing in subquery
|
||||
```
|
||||
|
||||
## New table from data
|
||||
|
||||
Create new table with necessary fields:
|
||||
|
||||
```sql
|
||||
CREATE TABLE <table> (
|
||||
(<field_name> <field_type> <option>,
|
||||
...);
|
||||
)
|
||||
```
|
||||
|
||||
Fill fields with data from table:
|
||||
|
||||
```sql
|
||||
INSERT INTO <table>
|
||||
SELECT <fields> FROM <TABLE> WHERE <condition>;
|
||||
```
|
||||
|
||||
## Join
|
||||
|
||||
Permette di legare due tabelle correlando i dati, le tabelle devono avere almeno un campo in comune.
|
||||
Primary key deve comparire in altra tabella come foreign key.
|
||||
|
||||
```sql
|
||||
SELECT * FROM <table1> JOIN <table2> ON <table1>.<field> = <table2>.<field>; -- seleziono tutti gli elementi che hanno una relarione tra le due tabelle
|
||||
SELECT * FROM <table1> LEFT JOIN <table2> ON <condition>; -- seleziona tutti gli elementi di table1 e i eventuali elementi richiamati dal join
|
||||
SELECT * FROM <table1> RIGHT JOIN <tabl2> ON <condition> -- -- seleziona tutti gli elementi di table2 e i eventuali elementi richiamati dal join
|
||||
```
|
||||
|
||||
[Inner Join, Left Join, Right Join, Full Outer Join](https://www.diffen.com/difference/Inner_Join_vs_Outer_Join)
|
||||
|
||||
## Multiple Join
|
||||
|
||||
```sql
|
||||
SELECT * FROM <table1>
|
||||
JOIN <table2> ON <table1>.<field> = <table2>.<field>
|
||||
JOIN <table3> ON <table2>.<field> = <table3>.<field>;
|
||||
```
|
||||
|
||||
[char, nchar, varchar, nvarchar](https://stackoverflow.com/questions/176514/what-is-the-difference-between-char-nchar-varchar-and-nvarchar-in-sql-server)
|
||||
|
||||
***
|
||||
|
||||
## T-SQL (MSSQL Server)
|
||||
|
||||
### T-SQL Insert From table
|
||||
|
||||
```sql
|
||||
USE [<db_name>]
|
||||
|
||||
SET IDENTITY_INSERT [<destination_table>] ON
|
||||
|
||||
INSERT INTO <table> (field_1, ...)
|
||||
|
||||
SELECT (field_1, ...) FROM <source_table>
|
||||
|
||||
SET IDENTITY_INSERT [<destination_table>] OFF
|
||||
```
|
||||
|
||||
### T-SQL Parametric Query
|
||||
|
||||
```sql
|
||||
-- variable declaration
|
||||
DECLARE @var_name <type>
|
||||
|
||||
-- init variable (input parameter)
|
||||
SET @var_name = <value>
|
||||
|
||||
-- use in query (memorize data)
|
||||
SELECT @var_name = COUNT(*) -- query won't show results in the "table view" sice param is used in SELECT
|
||||
FROM <table> ...
|
||||
|
||||
-- display message (query won't show results in the "table view")
|
||||
PRINT 'Text: ' + @var_name
|
||||
PRINT 'Text: ' + CONVERT(type, @var_name) -- convert data before printing
|
||||
GO
|
||||
```
|
||||
|
||||
### T-SQL View
|
||||
|
||||
A view represents a virtual table. Join multiple tables in a view and use the view to present the data as if the data were coming from a single table.
|
||||
|
||||
```sql
|
||||
CREATE VIEW <name> AS
|
||||
SELECT * FROM <table> ...
|
||||
```
|
||||
|
||||
### T-SQL Stored Procedure
|
||||
|
||||
[Stored Procedure How-To](https://docs.microsoft.com/en-us/sql/relational-databases/stored-procedures/create-a-stored-procedure "Create a Stored Procedure - Microsoft Docs")
|
||||
[T-SQL Stored Procedure](https://docs.microsoft.com/en-us/sql/t-sql/statements/create-procedure-transact-sql)
|
||||
|
||||
Stored Procedure Definition:
|
||||
|
||||
```sql
|
||||
CREATE PROCEDURE <Procedure_Name>
|
||||
-- Add the parameters for the stored procedure here
|
||||
<@Param1> <Datatype_For_Param1> = <Default_Value_For_Param1>,
|
||||
<@Param2> <Datatype_For_Param2>
|
||||
AS
|
||||
BEGIN
|
||||
-- SET NOCOUNT ON added to prevent extra result sets from interfering with SELECT statements.
|
||||
SET NOCOUNT ON; -- dont return number of selected rows
|
||||
|
||||
-- Insert statements for procedure here
|
||||
SELECT ...
|
||||
END
|
||||
GO
|
||||
```
|
||||
|
||||
Stored Procedure call in query:
|
||||
|
||||
```sql
|
||||
USE <database>
|
||||
GO
|
||||
|
||||
-- Stored Procedure call
|
||||
EXECUTE <Procedure_Name>
|
||||
-- or
|
||||
EXEC <Procedure_Name>
|
||||
```
|
417577
Git/ProGit.pdf
Normal file
417577
Git/ProGit.pdf
Normal file
File diff suppressed because one or more lines are too long
254
Git/git.md
Normal file
254
Git/git.md
Normal file
|
@ -0,0 +1,254 @@
|
|||
# Git Cheatsheet
|
||||
|
||||
## Glossary
|
||||
|
||||
**GIT**: an open source, distributed version-control system
|
||||
**GITHUB**: a platform for hosting and collaborating on Git repositories
|
||||
|
||||
**TREE**: directory that maps names to blobs or trees
|
||||
**BLOB**: any file
|
||||
**COMMIT**: snapshot of the entire repository + metadata, identified it's SHA-1 hash
|
||||
|
||||
**HEAD**: represents the current working directory, the HEAD pointer can be moved to different branches, tags, or commits when using git checkout
|
||||
**BRANCH**: a lightweight movable pointer to a commit
|
||||
**CLONE**: a local version of a repository, including all commits and branches
|
||||
**REMOTE**: a common repository on GitHub that all team member use to exchange their changes
|
||||
|
||||
**FORK**: a copy of a repository on GitHub owned by a different user
|
||||
**PULL REQUEST**: a place to compare and discuss the differences introduced on a branch with reviews, comments, integrated tests, and more
|
||||
|
||||
**REPOSITORY**: collection of files and folder of a project aka repo
|
||||
**STAGING AREA/STASH**: area of temporary snapshots (not yet commits)
|
||||
|
||||
## Data Model
|
||||
|
||||
Data Model Structure:
|
||||
|
||||
```txt
|
||||
<root> (tree)
|
||||
|
|
||||
|_ foo (tree)
|
||||
| |_ bar.txt (blob, contents = "hello world")
|
||||
|
|
||||
|_ baz.txt (blob, contents = "git is wonderful")
|
||||
```
|
||||
|
||||
Data Model as pseudocode:
|
||||
|
||||
```py
|
||||
# a file is a bunch of bytes
|
||||
blob = array<byte>
|
||||
|
||||
# a directory contains named files and directories
|
||||
tree = map<string, tree | file>
|
||||
|
||||
# a commit has parents, metadata, and the top-level tree
|
||||
commit = struct {
|
||||
parent: array<commit>
|
||||
author: string
|
||||
message: string
|
||||
snapshot: tree
|
||||
}
|
||||
|
||||
# an object is either a blob, tree or commit
|
||||
object = map<string, blob | tree | commit>
|
||||
|
||||
# commit identified by it's hash (unmutable)
|
||||
def store(object):
|
||||
id = sha1(object) # hash repo
|
||||
objects<id> = object # store repo w/ index hash
|
||||
|
||||
# load the commit
|
||||
def load(id):
|
||||
return objects<id>
|
||||
|
||||
# human-readable names for SHA-1 hashes (mutable)
|
||||
references = map<string, string>
|
||||
|
||||
# bind a regerence to a hash
|
||||
def update_reference(name, id):
|
||||
references<name> = id
|
||||
|
||||
def read_reference(name):
|
||||
return references<name>
|
||||
|
||||
def load_reference(name_or_id):
|
||||
if name_or_id in references:
|
||||
return load(references<name_or_id>)
|
||||
else:
|
||||
return load(name_or_id)
|
||||
```
|
||||
|
||||
## Commands
|
||||
|
||||
`git help <command>`: get help for a git command
|
||||
|
||||
### Create Repository
|
||||
|
||||
`git init [<project_name>]`: initialize a brand new Git repository and begins tracking
|
||||
`.gitignore`: specify intentionally untracked files to ignore
|
||||
|
||||
### Config
|
||||
|
||||
`git config --global user.name "<name>"`: set name attached to commits
|
||||
`git config --global user.email "<email address>"`: set email attached to commits
|
||||
`git config --global color.ui auto`: enable colorization of command line output
|
||||
|
||||
### Making Changes
|
||||
|
||||
`git status`: shows the status of changes as untracked, modified, or staged
|
||||
`git add <filename1 filename2 ...>`: add files to the staging area
|
||||
`git add -p <files>`: interacively stage chuncks of a file
|
||||
|
||||
`git stash`: temporarily remove modifications to working directory (must be staged to be stashed)
|
||||
`git stash pop`: recuperate stashed changes
|
||||
|
||||
`git blame <file>`: show who last edited which line
|
||||
|
||||
`git commit`: save the snapshot to the project history
|
||||
`git commit -m "message"`: commit and provide a message
|
||||
`git commit -a`: automatically notice any modified (but not new) files and commit
|
||||
|
||||
`git diff <filename>`: show difference since the last commit
|
||||
`git diff <commit> <filename>`: show differences in a file since a particular snapshot
|
||||
`git diff <reference_1> <reference_2> <filename>`: show differences in a file between two snapshots
|
||||
`git diff --cached`: show what is about to be committed
|
||||
`git diff <firts-branch>...<second-branch>`: show content diff between two branches
|
||||
|
||||
`git bisect`: binary search history (e.g. for regressions)
|
||||
|
||||
### Remotes
|
||||
|
||||
`git remote`: list remotes
|
||||
`git remote -v`: list remotes names and URLs
|
||||
`git remote show <remote>`: inspect the remote
|
||||
|
||||
`git remote add <remote> <url | path>`: add a remote
|
||||
`git branch --set-upstream-to=<remote>/<remote branch>`: set up correspondence between local and remote branch
|
||||
|
||||
`git push <remote> <branch>`: send objects to remote
|
||||
`git push <remote> <local branch>:<remote branch>`: send objects to remote, and update remote reference
|
||||
|
||||
`git fetch [<remote>]`: retrieve objects/references from a remote
|
||||
`git pull`: update the local branch with updates from its remote counterpart, same as `git fetch; git merge`
|
||||
|
||||
`git fetch && git show <remote>/<branch>`: show incoming changes
|
||||
|
||||
`git clone <url> [<folder_name>]`: download repository and repo history from remote
|
||||
`git clone --shallow`: clone only repo files, not history of commits
|
||||
|
||||
`git remote remove <remote>`: remove the specified remote
|
||||
`git remote rename <old_name> <new_name>`: rename a remote
|
||||
|
||||
### Viewing Project History
|
||||
|
||||
`git log`: show history of changes
|
||||
`git log -p`: show history of changes and complete differences
|
||||
`git log --stat --summary`: show overview of the change
|
||||
`git log --follow <file>`: list version history fo file, including renames
|
||||
`git log --all --graph --decorate`: visualizes history as a DAG
|
||||
`git log --oneline`: compact log
|
||||
|
||||
`git show <commit>`: output metadata and content changes of commit
|
||||
`git cat-file -p <commit>`: output commit metadata
|
||||
|
||||
### Tag
|
||||
|
||||
Git supports two types of tags: *lightweight* and *annotated*.
|
||||
|
||||
A lightweight tag is very much like a branch that doesn’t change—it’s just a pointer to a specific commit.
|
||||
|
||||
Annotated tags, however, are stored as full objects in the Git database.
|
||||
They’re checksummed;contain the tagger name, email, and date; have a tagging message; and can be signed and verifiedwith GNU Privacy Guard (GPG).
|
||||
It’s generally recommended creating annotated tags so it's possible to have all this information.
|
||||
|
||||
`git tag`: list existing tags
|
||||
`git tag -l|--list <pattern>`: list existing tags mathcing a wildard or pattern
|
||||
|
||||
`git tag <tag> [<commit_hash>]`: create a *lightweight* tag on the commit
|
||||
`git tag -a <tag> [<commit_hash> -m <message>]`: create am *annotated* tag on the commit
|
||||
|
||||
`git push <remote> <tagname>`: push a tag to the remote
|
||||
`git push <remote> --tags`: push commits and their tags (both types) to the remote
|
||||
|
||||
`git tag -d <tagname>`: delete a tag
|
||||
`git push <remote> :refs/tags<tagname>:`: remove a tag from the remote
|
||||
`git push <remote> --delete <tagname>`: remove a tag from the remote
|
||||
|
||||
`git checkout <tag>`: checkout a tag - **WARNING**: will go into *detached HEAD*
|
||||
|
||||
### Branching And Merging
|
||||
|
||||
`git branch`: shows branches
|
||||
`git branch -v`: show branch + last commit
|
||||
|
||||
`git branch <branch-name>`: create new branch
|
||||
`git checkout -b <branch-name>`: create a branch and switches to it, same as `git branch <name>; git checkout <name>`
|
||||
`git branch`: show list of all existing branches (* indicates current)
|
||||
`git checkout <branch-name>`: change current branch (update HEAD) and update working directory
|
||||
`git branch -d <branch-name>`: delete specified branch
|
||||
|
||||
`git merge <branch-name>`: merges into current branch
|
||||
`git merge --continue`: continue previous merge after solving a merge conflinct
|
||||
`git mergetool`: use a fancy tool to help resolve merge conflicts
|
||||
`git rebase`: rebase set of patches onto a new base
|
||||
`git rebase -i`: interactive rebasing
|
||||
|
||||
`gitk`: show graph of history (git for windows only)
|
||||
|
||||
### Undo & [Rewriting History](https://www.themoderncoder.com/rewriting-git-history/)
|
||||
|
||||
`git commit --amend`: replace last commit by creating a new one (can add files or rewrite commit message)
|
||||
`git commit --amend --no-edit`: replace last commit by creating a new one (can add files or rewrite commit message)
|
||||
`git reset HEAD <file>`: unstage a file
|
||||
`git reset <commit>`: undo all commits after specified commit, preserving changes locally
|
||||
`git checkout <file>`: discard changes
|
||||
`git checkout -- <file>`: discard changes, no output to screen
|
||||
`git reset --hard`: discard all changes since last commit
|
||||
`git reset --hard <commit>`: discard all history and changes back to specified commit
|
||||
`git rebase -i HEAD~<n>`: modify (reword, edit, drop, squash, merge, ...) *n* commits
|
||||
|
||||
**WARNING**: Changing history can have nasty side effects
|
||||
|
||||
---
|
||||
|
||||
## How To
|
||||
|
||||
### Rebase Branches
|
||||
|
||||
```ps1
|
||||
git checkout <primary_branch>
|
||||
git pull # get up to date
|
||||
|
||||
git checkout <feature_branch>
|
||||
git rebase <primary_branch> # rebase commits on master (moves branch start point on last master commit)
|
||||
|
||||
git chechout <primary_branch>
|
||||
git rebase <feature_branch> # moves commits from the branch on top of master
|
||||
```
|
||||
|
||||

|
||||
|
||||
### Clone Branches
|
||||
|
||||
```ps1
|
||||
git clone <repo> # clone the repo
|
||||
|
||||
git branch -r # show remote branches
|
||||
git checkout <branch> # checkout remote branch (omit <remote>/)
|
||||
|
||||
git pull # clone branch
|
||||
```
|
||||
|
||||
### [Sync Forks][github sync frok guide]
|
||||
|
||||
[github sync frok guide](https://docs.github.com/en/free-pro-team@latest/github/collaborating-with-issues-and-pull-requests/syncing-a-fork)
|
||||
|
||||
```ps1
|
||||
git fetch upstream # Fetch the branches and their respective commits from the upstream repository
|
||||
git checkout main # chechout fork's main primary branch
|
||||
|
||||
git merge upstream/main # Merge the changes from the upstream default branch into the local default branch
|
||||
|
||||
git push # update fork on GitHub
|
||||
```
|
608
HTML/HTML.md
Normal file
608
HTML/HTML.md
Normal file
|
@ -0,0 +1,608 @@
|
|||
# HTML Cheat Sheet
|
||||
|
||||
## Terminology
|
||||
|
||||
**Web design**: The process of planning, structuring and creating a website.
|
||||
**Web development**: The process of programming dynamic web applications.
|
||||
**Front end**: The outwardly visible elements of a website or application.
|
||||
**Back end**: The inner workings and functionality of a website or application.
|
||||
|
||||
## Anatomy of an HTML Element
|
||||
|
||||
**Element**: Building blocks of web pages, an individual component of HTML.
|
||||
**Tag**: Opening tag marks the beginning of an element & closing tag marks the end.
|
||||
Tags contain characters that indicate the tag's purpose content.
|
||||
|
||||
`<tagname> content </tagname>`
|
||||
|
||||
**Container Element**: An element that can contain other elements or content.
|
||||
**Stand Alone Element**: An element that cannot contain anything else.
|
||||
**Attribute**: Provides additional information about the HTML element. Placed inside an opening tag, before the right angle bracket.
|
||||
**Value**: Value is the value assigned to a given attribute. Values must be contained inside quotation marks (“”).
|
||||
|
||||
## The Doctype
|
||||
|
||||
The first thing on an HTML page is the doctype, which tells the browser which version of the markup language the page is using.
|
||||
|
||||
### XHTML 1.0 Strict
|
||||
|
||||
```html
|
||||
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN"
|
||||
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
|
||||
```
|
||||
|
||||
### HTML4 Transitional
|
||||
|
||||
```html
|
||||
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
|
||||
"http://www.w3.org/TR/html4/loose.dtd">
|
||||
```
|
||||
|
||||
### HTML5
|
||||
|
||||
`<!doctype html>`
|
||||
|
||||
## The HTML Element
|
||||
|
||||
After `<doctype>`, the page content must be contained between tags.
|
||||
|
||||
```html
|
||||
<!doctype html>
|
||||
<html lang="en">
|
||||
<!-- page contents -->
|
||||
</html>
|
||||
```
|
||||
|
||||
### The HEAD Element
|
||||
|
||||
The head contains the title of the page & meta information about the page. Meta information is not visible to the user, but has many purposes, like providing information to search engines.
|
||||
UTF-8 is a character encoding capable of encoding all possible characters, or code points, defined by Unicode. The encoding is variable-length and uses 8-bit code units.
|
||||
|
||||
XHTML and HTML4: `<meta http-equiv="Content-Type" content="text/html; charset=utf-8"></meta>`
|
||||
|
||||
Per essere compatibile con XHTML i meta-tag devono essere sempre chiusi.
|
||||
|
||||
HTML5: `<meta charset="utf-8">`
|
||||
|
||||
### HTML Shiv (Polyfill)
|
||||
|
||||
Used to make old browsers understand newer HTML5 and newer components.
|
||||
|
||||
```html
|
||||
<!--[if lt IE 9]>
|
||||
<script src="https://cdnjs.cloudflare.com/ajax/libs/html5shiv/3.7.3/html5shiv.js"></script>
|
||||
<![endif]-->
|
||||
```
|
||||
|
||||
## The BODY Element
|
||||
|
||||
The body contains the actual content of the page. Everything that is contained in the body is visible to the user.
|
||||
|
||||
```html
|
||||
<body>
|
||||
<!-- page contents -->
|
||||
</body>
|
||||
```
|
||||
|
||||
## JavaScript
|
||||
|
||||
XHTML and older: `<script src="js/scripts.js" type="text/javascript"></script>`
|
||||
HTML5: `<script src="js/scripts.js"></script>` (HTML5 spect states that `type` attribute is redundant and shoul be omitted)
|
||||
The `<script>` tag is used to define a client-side script (JavaScript).
|
||||
The `<script>` element either contains scripting statements, or it points to an external script file through the src attribute.
|
||||
|
||||
### Local, Remote or Inline JavaScript
|
||||
|
||||
**Remote**: `<script src="//ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script>`
|
||||
**Local**: `<script src="js/main.js"></script>`
|
||||
**Inline**: `<script> javascript-code-here </script>`
|
||||
|
||||
## Forms
|
||||
|
||||
Forms allow to collect data from the user:
|
||||
|
||||
* signing up and logging in to websites
|
||||
* entering personal information
|
||||
* filtering content (using dropdowns, checkboxes)
|
||||
* performing a search
|
||||
* uploading files
|
||||
|
||||
Forms contain elements called controls (text inputs, checkboxes, radio buttons, submit buttons).
|
||||
When users complete a form the data is usually submitted to a web server for processing.
|
||||
|
||||
### [Form Validation](https://developer.mozilla.org/en-US/docs/Learn/Forms/Form_validation)
|
||||
|
||||
Validation is a mechanism to ensure the correctness of user input.
|
||||
|
||||
Uses of Validation:
|
||||
|
||||
* Make sure that all required information has been entered
|
||||
* Limit the information to certain types (e.g. only numbers)
|
||||
* Make sure that the information follows a standard (e.g. email, credit card number)
|
||||
* Limit the information to a certain length
|
||||
* Other validation required by the application or the back-end services
|
||||
|
||||
#### Front-End Validation
|
||||
|
||||
The application should validate all information to make sure that it is complete, free of errors and conforms to the specifications required by the back-end.
|
||||
It should contain mechanisms to warn users if input is not complete or correct.
|
||||
It should avoid to send ‘bad’ data to the back-end.
|
||||
|
||||
### Back-End Validation
|
||||
|
||||
It should never trust that the front-end has done validation since some clever users can bypass the front-end mechanisms easily.
|
||||
Back-end services can receive data from other services, not necessarily front-end, that don’t perform validation.
|
||||
|
||||
#### Built-In Validation
|
||||
|
||||
Not all browsers validate in the same way and some follow the specs partially. Some browsers don’t have validation at all (older desktop browsers, some mobile browsers).
|
||||
Apart from declaring validation intention with HTML5 developers don’t have much control over what the browser actually does.
|
||||
Before using build-in validation make sure that it’s supported by the target browsers.
|
||||
|
||||
#### Validation with JavaScript
|
||||
|
||||
* Gives the developer more control.
|
||||
* The developer can make sure it works on all target browsers.
|
||||
* Requires a lot of custom coding, or using a library (common practice).
|
||||
|
||||
---
|
||||
|
||||
## General structure of HTML page
|
||||
|
||||
```html
|
||||
<!-- HTML Boilerplate -->
|
||||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<!-- meta tag -->
|
||||
<meta charset="utf-8">
|
||||
<title></title>
|
||||
<meta name="description" content="">
|
||||
<meta name="author" content="">
|
||||
|
||||
<!-- adapt page dimensions to device -->
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
|
||||
<!-- external style sheet here -->
|
||||
<link rel="stylesheet" href="path/style-sheet.css">
|
||||
|
||||
<!-- script if necessary -->
|
||||
<script src="_.js" type="text/javascript"></script>
|
||||
|
||||
<!-- script is executed only after the page finishes loading-->
|
||||
<script src="_.js" defer type="text/javascript"></script>
|
||||
</head>
|
||||
<body>
|
||||
|
||||
<!-- end of body -->
|
||||
<script src="_.js" type="text/javascript"></script>
|
||||
</body>
|
||||
</html>
|
||||
```
|
||||
|
||||
Attributes describe additional characteristics of an HTML element.
|
||||
`<tagname attribute="value"> content </tagname>`
|
||||
|
||||
### Meta Tag Structure
|
||||
|
||||
`<meta name="info-name" content="info-content">`
|
||||
|
||||
### Paragraph
|
||||
|
||||
Paragraphs allow to format the content in a readable fashion.
|
||||
|
||||
```html
|
||||
<p> paragraph-content </p>
|
||||
<p> paragraph-content </p>
|
||||
```
|
||||
|
||||
### Headings
|
||||
|
||||
Heading numbers indicates hierarchy, not size.
|
||||
|
||||
```html
|
||||
<h1> Heading 1 </h1>
|
||||
<h2> Heading 2 </h2>
|
||||
```
|
||||
|
||||
### Fromatted Text
|
||||
|
||||
With semantic value:
|
||||
|
||||
* Emphasized text (default cursive): `<em></em>`
|
||||
* Important text (default bold): `<strong></strong>`
|
||||
|
||||
Without semantic value, used as last resort:
|
||||
|
||||
* Italic text: `<i></i>`
|
||||
* Bold text: `<b></b>`
|
||||
|
||||
## Elements
|
||||
|
||||
`<br/>`: Line break (carriage return). It's not good practice to put line breacks inside paragraphs.
|
||||
|
||||
`<hr>`: horizontal rule (line). Used to define a thematic change in the content.
|
||||
|
||||
### Links/Anchor
|
||||
|
||||
Surround content to turn into links.
|
||||
|
||||
```html
|
||||
<!-- Link to absolute URL -->
|
||||
<a href="uri/url" title="content-title" taget="_self"> text/image </a>
|
||||
|
||||
<!-- links to relative URL -->
|
||||
<a href="//example.com">Scheme-relative URL</a>
|
||||
<a href="/en-US/docs/Web/HTML">Origin-relative URL</a>
|
||||
<a href="./file">Directory-relative URL</a>
|
||||
|
||||
<!-- Link to element on the same page -->
|
||||
<a href="#element-id"></a>
|
||||
|
||||
<!-- Link to top of page -->
|
||||
<a href="#top"> Back to Top </a>
|
||||
|
||||
<!-- link to email -->
|
||||
<a href="mailto:address@domain">address@domain</a>
|
||||
|
||||
<!-- link to telephone number -->
|
||||
<a href="tel:+39(111)2223334">+39 111 2223334</a>
|
||||
|
||||
<!-- download link -->
|
||||
<a href="./folder/filename" download>Download</a>
|
||||
```
|
||||
|
||||
`target`:
|
||||
|
||||
* `_blank`: opens linked document in a new window or *tab*
|
||||
* `_self`: opens linked document in the same frame as it was clicked (DEFAULT)
|
||||
* `_parent`: opens the linked document in the parent frame
|
||||
* `_top`: opens linked document in the full body of the window
|
||||
* `frame-name`: opens the linked document in the specified frame
|
||||
|
||||
### Images
|
||||
|
||||
```html
|
||||
<img src="image-location" alt="brief-description"/> <!-- image element -->
|
||||
<!-- alt should be always be populated for accessibility and SEO purposes -->
|
||||
```
|
||||
|
||||
```html
|
||||
<!-- supported by modern browsers -->
|
||||
<figure>
|
||||
<img src="img-location" alt="description">
|
||||
<figcaption> caption of the figure </figcaption>
|
||||
</figure>
|
||||
```
|
||||
|
||||
### Unordered list (bullet list)
|
||||
|
||||
```html
|
||||
<ul>
|
||||
<li></li> <!-- list element -->
|
||||
<li></li>
|
||||
</ul>
|
||||
```
|
||||
|
||||
### Ordered list (numebred list)
|
||||
|
||||
```html
|
||||
<ol>
|
||||
<li></li>
|
||||
<li></li>
|
||||
</ol>
|
||||
```
|
||||
|
||||
### Description list (list of terms and descriptions)
|
||||
|
||||
```html
|
||||
<dl>
|
||||
<dt>term</dt> <!-- define term/name -->
|
||||
<dd>definition</dd> <!-- describe term/name -->
|
||||
<dt>term</dt>
|
||||
<dd>definition</dd>
|
||||
</dl>
|
||||
```
|
||||
|
||||
### Tables
|
||||
|
||||
```html
|
||||
<table>
|
||||
<thead> <!-- table intestation (head) row -->
|
||||
<th></th> <!-- table intestation, one for each column-->
|
||||
<th></th>
|
||||
</thead>
|
||||
<tbody> <!-- table content (body) -->
|
||||
<tr> <!-- table row -->
|
||||
<td></td> <!-- table cell -->
|
||||
<td></td>
|
||||
</tr>
|
||||
</tbody>
|
||||
</table>
|
||||
```
|
||||
|
||||
### Character Codes
|
||||
|
||||
Code | Character
|
||||
---------|-----------------
|
||||
`©` | Copyrigth
|
||||
`<` | less than (`<`)
|
||||
`>` | greater than (`>`)
|
||||
`&` | ampersand (`&`)
|
||||
|
||||
### Block Element
|
||||
|
||||
Used to group elements together into sections, eventually to style them differently.
|
||||
|
||||
```html
|
||||
<div>
|
||||
<!-- content here -->
|
||||
</div>
|
||||
```
|
||||
|
||||
### Inline Element
|
||||
|
||||
Used to apply a specific style inline.
|
||||
|
||||
```html
|
||||
<p> non-styled content <span class="..."> styled content </span> non-styled content </p>
|
||||
```
|
||||
|
||||
### HTML5 new tags
|
||||
|
||||
```html
|
||||
<header></header>
|
||||
<nav></nav>
|
||||
<main></main>
|
||||
<section></section>
|
||||
<article></article>
|
||||
<aside></aside>
|
||||
<footer></footer>
|
||||
```
|
||||
|
||||
## HTML Forms
|
||||
|
||||
```html
|
||||
<form action="data-reciever" target="" method="http-method">
|
||||
<!-- ALL form elements go here -->
|
||||
</form>
|
||||
```
|
||||
|
||||
Target:
|
||||
|
||||
* `_blank`: submitted result will open in a new browser tab
|
||||
* `_self`: submitted result will open in the same page (*default*)
|
||||
|
||||
Method:
|
||||
|
||||
* `get`: data sent via get method is visible in the browser's address bar
|
||||
* `post`: data sent via post in not visible to the user
|
||||
|
||||
PROs & CONs of `GET` method:
|
||||
|
||||
* Data sent by the GET method is displayed in the URL
|
||||
* It is possible to bookmark the page with specific query string values
|
||||
* Not suitable for passing sensitive information such as the username and password
|
||||
* The length of the URL is limited
|
||||
|
||||
PROs & CONs of `POST` method:
|
||||
|
||||
* More secure than GET; information is never visible in the URL query string or in the server logs
|
||||
* Has a much larger limit on the amount of data that can be sent
|
||||
* Can send text data as well as binary data (uploading a file)
|
||||
* Not possible to bookmark the page with the query
|
||||
|
||||
### Basic Form Elements
|
||||
|
||||
```html
|
||||
<form action="" method="">
|
||||
<label for="target-identifier">label-here</label>
|
||||
<input type="input-type" name="input-name" value="value-sent" id="target-identifier">
|
||||
</form>
|
||||
```
|
||||
|
||||
Input Attributes:
|
||||
|
||||
* `name`: assigns a name to the form control (used by JavaScript and queries)
|
||||
* `value`: value to be sent to the server when the option is selected
|
||||
* `id`: identifier for CSS and linking tags
|
||||
* `checked`: intially selected or not (radiobutton, checkboxes, ...)
|
||||
* `selected`: default selection of a dropdown
|
||||
|
||||
### Text Field
|
||||
|
||||
One line areas that allow the user to input text.
|
||||
The `<label>` tag is used to define the labels for `<input>` elements.
|
||||
|
||||
```html
|
||||
<form>
|
||||
<label for="identifier">Label:</label>
|
||||
<input type="text" name="label-name" id="identifier" placeholder="placeholder-text">
|
||||
</form>
|
||||
|
||||
<!-- SAME AS -->
|
||||
|
||||
<form>
|
||||
<label>Label:
|
||||
<input type="text" name="label-name" id="identifier" placeholder="placeholder-text">
|
||||
</label>
|
||||
</form>
|
||||
```
|
||||
|
||||
Text inputs can display a placeholder text that will disappear as soon as some text is entered
|
||||
|
||||
### Password Field
|
||||
|
||||
```html
|
||||
<form>
|
||||
<label for="identifier">Password:</label>
|
||||
<input type="passwrod" name="user-password" id="identifier">
|
||||
</form>
|
||||
```
|
||||
|
||||
### Radio Buttons
|
||||
|
||||
```html
|
||||
<form action="..." method="post" target="_blank">
|
||||
<label for="identifier">Radiobutton-Text</label>
|
||||
<input type="radio" name="option-name" id="identifier" value="option-value">
|
||||
<label for="identifier">Radiobutton-Text</label>
|
||||
<input type="radio" name="option-name" id="identifier" value="option-value" checked="checked">
|
||||
|
||||
<button type="submit">Button-Action</button>
|
||||
</form>
|
||||
```
|
||||
|
||||
### Checkboxes
|
||||
|
||||
```html
|
||||
<form>
|
||||
<label for="identifier">Option-Name</label>
|
||||
<input type="checkbox" name="" id="identifier">
|
||||
|
||||
<label for="identifier">Option-Name</label>
|
||||
<input type="checkbox" name="" id="identifier">
|
||||
|
||||
<label for="identifier">Option-Name</label>
|
||||
<input type="checkbox" name="" id="identifier" checked="checked">
|
||||
</form>
|
||||
```
|
||||
|
||||
### Dropdown Menus
|
||||
|
||||
```html
|
||||
<form>
|
||||
<label for="identifier">Label:</label>
|
||||
<select name="" id="identifier" multiple>
|
||||
<option value="value">Value</option>
|
||||
<option value="value">Value</option>
|
||||
<option value="value" selected>Value</option>
|
||||
</select>
|
||||
</form>
|
||||
```
|
||||
|
||||
Use `<select>` rather than radio buttons when the number of options to choose from is large
|
||||
`selected` is used rather than checked.
|
||||
Provides the ability to select multiple options.
|
||||
Conceptually, `<select>` becomes more similar to checkboxes.
|
||||
|
||||
### FILE Select
|
||||
|
||||
Upload a local file as an attachment
|
||||
|
||||
```html
|
||||
<form>
|
||||
<label for="file-select">Upload:</label>
|
||||
<input type="file" name="upload" value="file-select">
|
||||
</form>
|
||||
```
|
||||
|
||||
### Text Area
|
||||
|
||||
Multi line text input.
|
||||
|
||||
```html
|
||||
<form>
|
||||
<label for="identifier">Label:</label>
|
||||
<textarea name="label" rows="row-number" cols="column-number" id="identifier">pre-existing editable test</textarea>
|
||||
<!-- rows and columns should be defined in a CSS -->
|
||||
</form>
|
||||
```
|
||||
|
||||
### Submit & Reset
|
||||
|
||||
```html
|
||||
<form action="" method="POST">
|
||||
<input type="submit" value="">
|
||||
<input type="reset" value="">
|
||||
<!-- OR -->
|
||||
<button type="submit" value="">
|
||||
<button type="reset" value="">
|
||||
</form>
|
||||
```
|
||||
|
||||
`sumbit`: sends the form data to the location specified in the action attribute.
|
||||
`reset`: resets all forms controls to the default values.
|
||||
|
||||
### Button
|
||||
|
||||
```html
|
||||
<button type="button/reset/submit" value=""/>
|
||||
|
||||
<!-- can contain HTML -->
|
||||
<button type="button/reset/submit" value=""></button>
|
||||
```
|
||||
|
||||
### Fieldset
|
||||
|
||||
Group controls into categories. Particularly important for screen readers.
|
||||
|
||||
```html
|
||||
<fieldset>
|
||||
<legend>Color</legend>
|
||||
<input type="radio" name="colour" value="red" id="colour_red">
|
||||
<label for="colour_red">Red</label>
|
||||
<input type="radio" name="colour" value="green" id="colour_green">
|
||||
<label for="colour_green">Green</label>
|
||||
<input type="radio" name="colour" value="blue" id="colour_blue">
|
||||
<label for="colour_blue">Red</label>
|
||||
</fieldset>
|
||||
```
|
||||
|
||||
## HTML5 Input Types
|
||||
|
||||
Newer input types are useful for:
|
||||
|
||||
* validation
|
||||
* restricting user input
|
||||
* Using custom dialogs
|
||||
|
||||
Downsides:
|
||||
|
||||
* most are not supported by older browsers, especially Internet Explorer.
|
||||
* each browser has a different implementation so the user experience is not consistent.
|
||||
|
||||
### Email Field
|
||||
|
||||
Used to receive a valid e-mail address from the user. Most browsers can validate this without needing javascript.
|
||||
Older browsers don’t support this input type.
|
||||
|
||||
```html
|
||||
<form>
|
||||
<label for="user-email">Email:</label>
|
||||
<input type="email" name="user-email" id="form-email">
|
||||
<button type="submit">Send</button>
|
||||
</form>
|
||||
```
|
||||
|
||||
### More Input Types
|
||||
|
||||
```html
|
||||
<input type="email" id="email" name="email">
|
||||
<input type="url" id="url" name="url">
|
||||
<input type="number" name="" id="identifier" min="min-value" max="max-value" step="">
|
||||
<input type="search" id="identifier" name="">
|
||||
```
|
||||
|
||||
### [Using Built-In Form Validation](https://developer.mozilla.org/en-US/docs/Learn/Forms/Form_validation)
|
||||
|
||||
One of the most significant features of HTML5 form controls is the ability to validate most user data without relying on JavaScript.
|
||||
This is done by using validation attributes on form elements.
|
||||
|
||||
* `required`: Specifies whether a form field needs to be filled in before the form can be submitted.
|
||||
* `minlength`, `maxlength`: Specifies the minimum and maximum length of textual data (strings)
|
||||
* `min`, `max`: Specifies the minimum and maximum values of numerical input types
|
||||
* `type`: Specifies whether the data needs to be a number, an email address, or some other specific preset type.
|
||||
* `pattern`: Specifies a regular expression that defines a pattern the entered data needs to follow.
|
||||
|
||||
If the data entered in an form field follows all of the rules specified by the above attributes, it is considered valid. If not, it is considered invalid.
|
||||
|
||||
When an element is valid, the following things are true:
|
||||
|
||||
* The element matches the `:valid` CSS *pseudo-class*, which lets you apply a specific style to valid elements.
|
||||
* If the user tries to send the data, the browser will submit the form, provided there is nothing else stopping it from doing so (e.g. JavaScript).
|
||||
|
||||
When an element is invalid, the following things are true:
|
||||
|
||||
* The element matches the `:invalid` CSS *pseudo-class*, and sometimes other UI *pseudo-classes* (e.g. `:out-of-range`) depending on the error, which lets you apply a specific style to invalid elements.
|
||||
* If the user tries to send the data, the browser will block the form and display an error message.
|
24
HTML/boilerplate.html
Normal file
24
HTML/boilerplate.html
Normal file
|
@ -0,0 +1,24 @@
|
|||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<!-- meta tag -->
|
||||
<meta charset="utf-8">
|
||||
<title></title>
|
||||
<meta name="description" content="">
|
||||
<meta name="author" content="">
|
||||
|
||||
<!-- adapt page dimensions to device -->
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0">
|
||||
|
||||
<!-- external style sheet here -->
|
||||
<link rel="stylesheet" href="path/style-sheet.css">
|
||||
|
||||
<!-- script if necessary -->
|
||||
<script src="_.js" type="text/javascript"></script>
|
||||
</head>
|
||||
<body>
|
||||
|
||||
<!-- end of body -->
|
||||
<script src="_.js" type="text/javascript" defer async></script>
|
||||
</body>
|
||||
</html>
|
115
Java/DAO.md
Normal file
115
Java/DAO.md
Normal file
|
@ -0,0 +1,115 @@
|
|||
# Database Access Object
|
||||
|
||||
## DB
|
||||
|
||||
Connection to the DB.
|
||||
|
||||
```java
|
||||
package dao;
|
||||
|
||||
import java.sql.Connection;
|
||||
import java.sql.DriverManager;
|
||||
import java.sql.SQLException;
|
||||
|
||||
public class DB {
|
||||
|
||||
private Connection conn; // db connection obj
|
||||
|
||||
private final String URL = "jdbc:<dbms>:<db_url>/<database>";
|
||||
private final String USER = "";
|
||||
private final String PWD = "";
|
||||
|
||||
public DB() {
|
||||
this.conn = null;
|
||||
}
|
||||
|
||||
public void connect() {
|
||||
try {
|
||||
this.conn = DriverManager.getConnection(URL, USER, PWD);
|
||||
} catch (SQLException e) {
|
||||
e.printStackTrace();
|
||||
}
|
||||
}
|
||||
|
||||
public void disconnect() {
|
||||
if(conn != null) {
|
||||
try {
|
||||
this.conn.close();
|
||||
} catch (SQLException e) {
|
||||
e.printStackTrace();
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
public Connection getConn() {
|
||||
return conn;
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## `I<Type>DAO`
|
||||
|
||||
Interface for CRUD methods on a database.
|
||||
|
||||
```java
|
||||
package dao;
|
||||
|
||||
import java.sql.SQLException;
|
||||
import java.util.List;
|
||||
|
||||
public interface I<Type>DAO {
|
||||
|
||||
String RAW_QUERY = "SELECT * ..."
|
||||
public Type Query() throws SQLException;
|
||||
}
|
||||
|
||||
```
|
||||
|
||||
## `<Type>DAO`
|
||||
|
||||
Class implementing `I<Type>DAO` and handling the comunication with the db.
|
||||
|
||||
```java
|
||||
package dao;
|
||||
|
||||
import java.sql.ResultSet;
|
||||
import java.sql.SQLException;
|
||||
import java.sql.Statement;
|
||||
|
||||
public class <Type>DAO implements I<Type>DAO {
|
||||
|
||||
private <Type> results;
|
||||
|
||||
private Statement statement; // SQL instruction contenitor
|
||||
private ResultSet rs; // Query results contenitor
|
||||
private DB db;
|
||||
|
||||
public <Type>DAO() {
|
||||
db = new DB();
|
||||
}
|
||||
|
||||
@Override
|
||||
public Type Query() throws SQLException {
|
||||
// 1. connection
|
||||
db.connect();
|
||||
|
||||
// 2. instruction
|
||||
statement = db.getConn().createStatement(); // statement creation
|
||||
|
||||
// 3. query
|
||||
rs = statement.executeQuery(RAW_QUERY); // set the query
|
||||
|
||||
// 4. results
|
||||
while(rs.next()) {
|
||||
// create and valorize Obj
|
||||
// add to results
|
||||
}
|
||||
|
||||
// 5. disconnection
|
||||
db.disconnect()
|
||||
|
||||
// 6. return results
|
||||
return results;
|
||||
}
|
||||
}
|
||||
```
|
122
Java/Java Collection Framework.md
Normal file
122
Java/Java Collection Framework.md
Normal file
|
@ -0,0 +1,122 @@
|
|||
# Java Collection Framework - JCF
|
||||
|
||||
All classes that permit the handling of groups of objects constituite the Java Collection Framework.
|
||||
|
||||
A Collection is a *container* in which several objects are grouped in a *single entity*.
|
||||
|
||||
The **Java Collection Framework** is constituited by:
|
||||
|
||||
- **Interfaces** the define the operations of a generic collection. They can be split into two categories:
|
||||
- **Collection**: used to optimize operations of insertion, modification and deletion of elements in a group of objects.
|
||||
- **Map**: optimized for look-up operations.
|
||||
- **Classes** that implement the interfaces using different data structures.
|
||||
- **Algorithms** consistng in methods to operate over a collection.
|
||||
|
||||

|
||||
|
||||
## java.util.Collections
|
||||
|
||||
### Collection Functions
|
||||
|
||||
```java
|
||||
boolean add (Object o) e.g., <x>.add (<y>) //append to colelction, false if fails
|
||||
boolean add (int index, Object o) //insertion at given index
|
||||
boolean addAll (Collection c) //appends a collection to another
|
||||
void clear() //remove items from container
|
||||
boolean contains (Object o) //true if object is in collection
|
||||
boolean containsAll (Collection c) //true if all items of collectio are in another
|
||||
boolean isEmpty (Object o) e.g., if (<x>.isEmpty()) ... //true if collection is empty
|
||||
boolean remove (Object o) //remove object from collection
|
||||
Object remove (int index) //remove object at given index
|
||||
void removeAll (Collection c) //remove all items form collection
|
||||
int size () //number og items in collection
|
||||
Object [] toArray() //transform collection in array
|
||||
Iterator iterator() //returns iterator to iterate over the collection
|
||||
```
|
||||
|
||||
### Collections Methods
|
||||
|
||||
```java
|
||||
Collection<E>.forEach(Consumer<? super T> action);
|
||||
```
|
||||
|
||||
### Iterator
|
||||
|
||||
Abstracts the problem of iterating over all the elements of a collection;
|
||||
|
||||
- `public Iterator (Collection c)` creates the Iterator
|
||||
- `public boolean hasNext()` checks if there is a successive element
|
||||
- `public Object next()` extracts the successive element
|
||||
|
||||
### ArrayList
|
||||
|
||||
**Note**: ArrayLists can't contain *primitive* values. *Use wrapper classes* instead.
|
||||
|
||||
```java
|
||||
import java.util.ArrayList;
|
||||
ArrayList<Type> ArrayListName = new ArrayList<Type>(starting_dim); //resizable array
|
||||
ArrayList<Type> ArrayListName = new ArrayList<Type>(); //resizable array
|
||||
ArrayList<Type> ArrayListName = new ArrayList<>(); //resizable array (JAVA 1.8+)
|
||||
|
||||
|
||||
ArrayListName.add(item); //append item to collection
|
||||
ArrayListName.add(index, item); // add item at positon index, shift all item from index and successive towards the end af the ArrayList
|
||||
ArrayListName.set(index, item); // substitute EXISTING item
|
||||
ArrayListName.get(index); //access to collection item
|
||||
ArrayListName.remove(item) //remove first occurence of item from collection
|
||||
ArrayListName.remove(index) //remove item at position index
|
||||
ArrayListName.clear() //emties the ArrayList
|
||||
ArrayListName.contains(object); // check if object is in the ArrayList
|
||||
ArrayListName.IndexOf(object); // returns the index of the object
|
||||
ArrayListName.isEmpty(); // check wether the list is empty
|
||||
|
||||
ArrayListName.size(); //dimension of the ArrayList
|
||||
ArrayListName.tirmToSize(); // reduce ArrayList size to minimum needed
|
||||
// ArrayList size doubles when a reseize is needed.
|
||||
|
||||
//run through to the collection with functional programming (JAVA 1.8+)
|
||||
ArrayListName.forEach(item -> function(v));
|
||||
```
|
||||
|
||||
### Collection Sorting
|
||||
|
||||
To sort a collection it's items must implement `Comparable<T>`:
|
||||
|
||||
```java
|
||||
class ClassName implements Comparable<ClassName> {
|
||||
|
||||
@override
|
||||
public int compareTo(Classname other){
|
||||
//compare logic
|
||||
return <int>;
|
||||
}
|
||||
}
|
||||
|
||||
List<ClassName> list;
|
||||
//valorize List
|
||||
Collections.sort(list); //"natuarl" sorting uses compareTo()
|
||||
```
|
||||
|
||||
Otherwise a `Comparator()` must be implemented:
|
||||
|
||||
```java
|
||||
class Classname {
|
||||
//code here
|
||||
}
|
||||
|
||||
// Interface object (!) implements directly a method
|
||||
Comparator<ClassName> comparator = new Comparator<ClassName>() {
|
||||
|
||||
@Override
|
||||
public int compare(ClassName o1, Classname o2) {
|
||||
//compare logic
|
||||
return <int>;
|
||||
}
|
||||
};
|
||||
|
||||
List<ClassName> list;
|
||||
//valorize List
|
||||
Collections.sort(list, comparator); //"natuarl" sorting uses compareTo()
|
||||
```
|
||||
|
||||
`Comparator<T>` and `Conmparable<T>` are functional interfaces
|
115
Java/Java Web/JSP.md
Normal file
115
Java/Java Web/JSP.md
Normal file
|
@ -0,0 +1,115 @@
|
|||
# Java Server Pages Cheat Sheet
|
||||
|
||||
Java embedded in html.
|
||||
Codice + JSP salvati in file `.war`
|
||||
|
||||
[TEMP Notes Source](https://github.com/maboglia/CorsoJava/blob/master/appunti/057_JSP_appunti.md#corso-jsp---le-direttive)
|
||||
|
||||
## Directives
|
||||
|
||||
Le direttive Le direttive permettono di definire la struttura di tutto il documento JSP. Indicano gli aspetti principali del *servlet* in cui sarà convertito il file JSP.
|
||||
|
||||
Sono processati al momento della conversione in servlet, a compile-time.
|
||||
|
||||
Le direttive esistenti sono: *page*, *include* e *taglib*.
|
||||
|
||||
- `<%@ page attribute="value" %>`
|
||||
- `<%@ include file="path" %>`
|
||||
- `<%@ taglib ...%>`
|
||||
|
||||
Introdotte dal simbolo `@` possono contenere diversi attributi, in alcuni casi concatenabili come per la direttiva import.
|
||||
|
||||
### Attributi Direttiva `Page`
|
||||
|
||||
**`import="package.class"`**
|
||||
Lista di package o classes, separati da virgola, che saranno importati per essere utilizzati nel codice java.
|
||||
|
||||
**`session ="true | false"`**
|
||||
Specifica se la pagina fa parte di una sessione HTTP. Se si inizializza a true, è disponibile l'oggetto implicito sessione.
|
||||
|
||||
**`buffer ="dimensione-kb"`**
|
||||
Specifica la dimensione di un buffer di uscita di tipo stream, per il client.
|
||||
|
||||
**`errorPage="url"`**
|
||||
Specifica una pagina JSP che sarà processata nel caso si verifichi un errore.
|
||||
|
||||
**`isErrorPage="true|false"`**
|
||||
Indica che la pagina è una pagina di errore JSP e può mostrare a video l'output dell'errore verificatosi. Per default è settata false.
|
||||
|
||||
**`contentType="MIME-Type"`**, **`contentType="MIME-Type; charset=Character-Set"`**
|
||||
valore MIME di default è text/html
|
||||
|
||||
### Direttiva `Include`
|
||||
|
||||
Indica al motore JSP di includere il contenuto del file corrispondente, inserendolo al posto della direttiva nella pagina JSP. Il contenuto del file incluso è analizzato al momento della traduzione del file JSP e si include una copia del file stesso nel servlet generato. Una volta incluso, se si modifica il file non sarà ricompilato nel servlet. Il tipo di file da includere può essere un file html (*statico*) o un file jsp (*dinamico*).
|
||||
|
||||
```jsp
|
||||
<html>
|
||||
<head>
|
||||
<title> pagina di prova Direttive </title>
|
||||
</head>
|
||||
<body>
|
||||
<h1>pagina di prova Direttive inclusione</h1>
|
||||
<%@ include file="/hello_world.html" %>
|
||||
<%@ include file=”/login.jsp” %>
|
||||
</body>
|
||||
</html>
|
||||
```
|
||||
|
||||
### Direttiva `Taglib`
|
||||
|
||||
Permette estendere i marcatori di JSP con etichette o marcatori generati dall'utente (etichette personalizzate).
|
||||
|
||||
Sintassi: `<%@ taglib uri="taglibraryURI" prefix="tagPrefix" %>`
|
||||
|
||||
Esempi librerie standard:
|
||||
|
||||
- JSTL core
|
||||
- JSTL sql
|
||||
- JSTL function
|
||||
|
||||
## Implicit Objects
|
||||
|
||||
JSP utilizza gli oggetti impliciti (built-in).
|
||||
|
||||
Gli oggetti impliciti sono oggetti istanziati automaticamente dall’ambiente JSP, non dobbiamo preoccuparci di importarli e istanziarli.
|
||||
Per utilizzarli è sufficiente usare la sintassi `nomeOggetto.nomeMetodo`.
|
||||
|
||||
Oggetti disponibili per l’uso in pagine JSP:
|
||||
|
||||
- **out**: per scrivere codice HTML nella risposta (System.out di Java)
|
||||
- **session**: dati specifici della sessione utente corrente
|
||||
- **request**: richiesta HTTP ricevuta e i suoi attributi, header, cookie, parametri, etc.
|
||||
- **page**: la pagina e le sue proprietà.
|
||||
- **config**: dati di configurazione
|
||||
- **response**: risposta HTTP e le sue proprietà.
|
||||
- **application**: dati condivisi da tutte le pagine della web application
|
||||
- **exception**: eventuali eccezioni lanciate dal server; utile per pagine di errore
|
||||
- **pageContext**: dati di contesto per l’esecuzione della pagina
|
||||
|
||||
Gli oggetti impliciti possono essere:
|
||||
|
||||
- oggetti legati alla servlet relativa alla pagina JSP
|
||||
- oggetti legati all’input e all’output della pagina JSP
|
||||
- oggetti che forniscono informazioni sul contesto in cui la JSP viene eseguita
|
||||
- oggetti risultanti da eventuali errori
|
||||
|
||||
Ambito Definisce dove e per quanto tempo saranno accessibili gli oggetti (oggetti impliciti, JavaBeans, ...):
|
||||
|
||||
- di pagina: l'oggetto è accessibile dal servlet che rappresenta la pagina
|
||||
- di richiesta: l'oggetto viene creato e poi distrutto dopo l'uso
|
||||
- di sessione: l'oggetto è accessibile durante tutta la sessione
|
||||
- di applicazione: l'oggetto è accessibile dal servlet che rappresenta la pagina
|
||||
|
||||
---
|
||||
|
||||
## Code in JSP Page
|
||||
|
||||
`<% /* code here */ %>` is used to embed java code in a JSP page.
|
||||
`<%= var %>` is used to get a value from a variable to be displayed.
|
||||
|
||||
### Request Parameters
|
||||
|
||||
```java
|
||||
Type variable = request.getParameter("request_parameter"); // parameter of a GET request
|
||||
```
|
51
Java/Java Web/Servlet.md
Normal file
51
Java/Java Web/Servlet.md
Normal file
|
@ -0,0 +1,51 @@
|
|||
# Servlet
|
||||
|
||||
A Java servlet is a Java software component that extends the capabilities of a server.
|
||||
Although servlets can respond to many types of requests, they most commonly implement web containers for hosting web applications on web servers and thus qualify as a server-side servlet web API.
|
||||
|
||||
## Basic Structure
|
||||
|
||||
```java
|
||||
package <package>;
|
||||
|
||||
import java.io.IOException;
|
||||
import javax.servlet.ServletException;
|
||||
import javax.servlet.annotation.WebServlet;
|
||||
import javax.servlet.http.HttpServlet;
|
||||
import javax.servlet.http.HttpServletRequest;
|
||||
import javax.servlet.http.HttpServletResponse;
|
||||
|
||||
@WebServlet("/route")
|
||||
public class <className> extends HttpServlet {
|
||||
private static final long serialVersionUID = 1L;
|
||||
|
||||
/** handle get request */
|
||||
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
|
||||
|
||||
// GET REQUEST: load and display page (forward to JSP)
|
||||
// OPTIONAL: add data to request (setAttribute())
|
||||
|
||||
}
|
||||
|
||||
/** handle post request */
|
||||
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
|
||||
|
||||
// POST REQUEST: add stuff to DB, page, ...
|
||||
|
||||
doGet(request, response); // return same page with new conted added (default case)
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Servlet Instructions
|
||||
|
||||
```java
|
||||
request.getParameter() // read request parameter
|
||||
|
||||
response.setContentType("text/html"); // to return HTML in the response
|
||||
|
||||
response.getWriter().append(""); //append content to the http response
|
||||
|
||||
request.setAttribute(attributeName, value); // set http attribute of the request
|
||||
request.getRequestDispatcher("page.jsp").forward(request, response); // redirect the request to another page
|
||||
```
|
1231
Java/Java.md
Normal file
1231
Java/Java.md
Normal file
File diff suppressed because it is too large
Load diff
203
Java/Spring Framework/Spring Project Structure.md
Normal file
203
Java/Spring Framework/Spring Project Structure.md
Normal file
|
@ -0,0 +1,203 @@
|
|||
# Spring Project
|
||||
|
||||
## Libs
|
||||
|
||||
- MySql Driver
|
||||
- Spring Data JPA (data persistance)
|
||||
- Spring Boot Dev Tools
|
||||
- Jersey (Serializzazione)
|
||||
- Spring Web
|
||||
|
||||
## application.properties
|
||||
|
||||
```ini
|
||||
spring.datasource.url=DB_url
|
||||
spring.datasource.username=user
|
||||
spring.datasource.password=password
|
||||
|
||||
spring.jpa.show-sql=true
|
||||
|
||||
server.port=server_port
|
||||
```
|
||||
|
||||
## Package `entities`
|
||||
|
||||
Model of a table of the DB
|
||||
|
||||
```java
|
||||
package <base_pakcage>.entities;
|
||||
|
||||
import javax.persistence.Entity;
|
||||
import javax.persistence.GeneratedValue;
|
||||
import javax.persistence.GenerationType;
|
||||
import javax.persistence.Id;
|
||||
import javax.persistence.Table;
|
||||
|
||||
@Entity // set as DB Entity (DB record Java implementation)
|
||||
public class Entity {
|
||||
|
||||
@Id // set as Primary Key
|
||||
@GeneratedValue(strategy = GenerationType.IDENTITY) // id is autoincremented by the DB
|
||||
private int id;
|
||||
|
||||
// no constructor (Spring requirement)
|
||||
|
||||
// table columns attributes
|
||||
|
||||
// getters & setters
|
||||
|
||||
// toString()
|
||||
}
|
||||
```
|
||||
|
||||
## Package `dal`
|
||||
|
||||
Spring Interface for DB connection and CRUD operations.
|
||||
|
||||
```java
|
||||
package <base_package>.dal // or .repository
|
||||
|
||||
import org.springframework.data.repository.JpaRepository;
|
||||
import org.springframework.data.repository.Query;
|
||||
import org.springframework.data.repository.query.Param;
|
||||
|
||||
// interface for spring Hibernate JPA
|
||||
// CrudRepository<Entity, PK_Type>
|
||||
public interface IEntityDAO extends JpaRepository<Entity, Integer> {
|
||||
|
||||
// custom query
|
||||
@Query("FROM <Entity> WHERE param = :param")
|
||||
Type query(@Param("param") Type param);
|
||||
}
|
||||
```
|
||||
|
||||
## Package `services`
|
||||
|
||||
Interfaces and method to access the Data Access Layer (DAL).
|
||||
|
||||
In `IEntityService.java`:
|
||||
|
||||
```java
|
||||
package <base_package>.services;
|
||||
|
||||
import java.util.List;
|
||||
|
||||
import org.springframework.beans.factory.annotation.Autowired;
|
||||
|
||||
import <base_package>.Entity;
|
||||
import <base_package>.IEntityRepository;
|
||||
|
||||
// CRUD method implemented on Entity
|
||||
public interface IEntityService {
|
||||
|
||||
String FIND_ALL = "SELECT * FROM <table>"
|
||||
String FIND_ONE = "SELECT * FROM <table> WHERE id = ?"
|
||||
|
||||
List<Entity> findAll();
|
||||
Entity findOne(int id);
|
||||
void addEntity(Entity e);
|
||||
void updateEntity(int id, Entity e);
|
||||
void deleteEntity(Entity e);
|
||||
void deleteEntity(int id);
|
||||
|
||||
}
|
||||
```
|
||||
|
||||
In `EntityService.java`:
|
||||
|
||||
```java
|
||||
package com.lamonacamarcello.libri.services;
|
||||
|
||||
import java.util.List;
|
||||
|
||||
import org.springframework.beans.factory.annotation.Autowired;
|
||||
import org.springframework.stereotype.Service;
|
||||
|
||||
import <base_package>.entities.Entity;
|
||||
import <base_package>.repos.ILibriRepository;
|
||||
|
||||
// implementation of IEntityService
|
||||
@Service
|
||||
public class EntityService implements IEntityService {
|
||||
|
||||
@Autowired // connection to db (obj created by spring as needed: Inversion Of Control)
|
||||
private IEntityDAO repo;
|
||||
|
||||
@Override
|
||||
public List<Entity> findAll() {
|
||||
return repo.findAll();
|
||||
}
|
||||
|
||||
@Override
|
||||
public Entity findOne(int id) {
|
||||
return repo.findById(id).get();
|
||||
}
|
||||
|
||||
@Override
|
||||
public void addEntity(Entity e) {
|
||||
repo.save(e);
|
||||
}
|
||||
|
||||
@Override
|
||||
public void updateEntity(int id, Entity e) {
|
||||
}
|
||||
|
||||
@Override
|
||||
public void deleteEntity(Entity e) {
|
||||
}
|
||||
|
||||
@Override
|
||||
public void deleteEntity(int id) {
|
||||
}
|
||||
|
||||
// custom query
|
||||
Type query(Type param) {
|
||||
return repo.query(param);
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Package `integration`
|
||||
|
||||
REST API routes & endpoints to supply data as JSON.
|
||||
|
||||
```java
|
||||
package com.lamonacamarcello.libri.integration;
|
||||
|
||||
import java.util.List;
|
||||
|
||||
import org.springframework.beans.factory.annotation.Autowired;
|
||||
import org.springframework.web.bind.annotation.GetMapping;
|
||||
import org.springframework.web.bind.annotation.PathVariable;
|
||||
import org.springframework.web.bind.annotation.RestController;
|
||||
|
||||
import <base_package>.entities.Entity;
|
||||
import <base_package>.services.IEntityService;
|
||||
|
||||
@RestController // returns data as JSON
|
||||
@RequestMapping("/api") // controller route
|
||||
public class EntityRestCtrl {
|
||||
|
||||
@Autowired // connection to service (obj created by spring as needed: Inverion Of Control)
|
||||
private IEntityService service;
|
||||
|
||||
@GetMapping("entities") // site route
|
||||
public List<Entity> findAll(){
|
||||
return service.findAll();
|
||||
}
|
||||
|
||||
@GetMapping("/entities/{id}")
|
||||
public Entity findOne(@PathVariable("id") int id) { // use route variable
|
||||
return service.findOne(id);
|
||||
}
|
||||
|
||||
@PostMapping("/entities")
|
||||
public void addEntity(@RequestBody Entity e) { // added entity is in the request body
|
||||
return service.addEntity(e)
|
||||
}
|
||||
|
||||
// PUT / PATCH -> updateEntity(id, e)
|
||||
|
||||
// DELETE -> deleteEntity(id)
|
||||
}
|
||||
```
|
45
Java/Spring Framework/pom.xml.md
Normal file
45
Java/Spring Framework/pom.xml.md
Normal file
|
@ -0,0 +1,45 @@
|
|||
# pom.xml
|
||||
|
||||
File specifing project dependencies.
|
||||
|
||||
```xml
|
||||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
|
||||
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
|
||||
<modelVersion>major.minor.patch</modelVersion>
|
||||
|
||||
<parent>
|
||||
<groupId>org.springframework.boot</groupId>
|
||||
<artifactId>spring-boot-starter-parent</artifactId>
|
||||
<version>major.minor.patch.RELEASE</version>
|
||||
<relativePath/> <!-- lookup parent from repository -->
|
||||
</parent>
|
||||
|
||||
<groupId>base_package</groupId>
|
||||
<artifactId>Project_Name</artifactId>
|
||||
<version>major.minor.patch</version>
|
||||
<name>Project_Name</name>
|
||||
<description>...</description>
|
||||
|
||||
<properties>
|
||||
<java.version>11</java.version>
|
||||
</properties>
|
||||
|
||||
<dependencies>
|
||||
<dependency>
|
||||
<groupId>org.springframework.boot</groupId>
|
||||
<artifactId>spring-boot-starter-data-jpa</artifactId>
|
||||
</dependency>
|
||||
</dependencies>
|
||||
|
||||
<build>
|
||||
<plugins>
|
||||
<plugin>
|
||||
<groupId>org.springframework.boot</groupId>
|
||||
<artifactId>spring-boot-maven-plugin</artifactId>
|
||||
</plugin>
|
||||
</plugins>
|
||||
</build>
|
||||
|
||||
</project>
|
||||
```
|
112
JavaScript/AJAX.md
Normal file
112
JavaScript/AJAX.md
Normal file
|
@ -0,0 +1,112 @@
|
|||
# AJAX
|
||||
|
||||
**AJAX**: Asynchronous JavaScript and XML
|
||||
|
||||
AJAX Interaction:
|
||||
|
||||
1. An event occurs in a web page (the page is loaded, a button is clicked)
|
||||
2. 2.An `XMLHttpRequest` object is created by JavaScript
|
||||
3. 3.The `XMLHttpRequest` object sends a request to a web server
|
||||
4. 4.The server processes the request
|
||||
5. 5.The server sends a response back to the web page
|
||||
6. 6.The response is read by JavaScript
|
||||
7. 7.Proper action (like page update) is performed by JavaScript
|
||||
|
||||
## XMLHttpRequest
|
||||
|
||||
```js
|
||||
var request = new XMLHttpRequest();
|
||||
|
||||
request.addEventListener(event, function() {...});
|
||||
|
||||
request.open("HttpMethod", "path/to/api", true); // third parameter is asynchronicity (true = asynchronous)
|
||||
reqest.setRequestHeader(key, value) // HTTP Request Headers
|
||||
reqest.send()
|
||||
```
|
||||
|
||||
To check the status use `XMLHttpRequest.status` and `XMLHttpRequest.statusText`.
|
||||
|
||||
### XMLHttpRequest Events
|
||||
|
||||
**loadstart**: fires when the process of loading data has begun. This event always fires first
|
||||
**progress**: fires multiple times as data is being loaded, giving access to intermediate data
|
||||
**error**: fires when loading has failed
|
||||
**abort**: fires when data loading has been canceled by calling abort()
|
||||
**load**: fires only when all data has been successfully read
|
||||
**loadend**: fires when the object has finished transferring data always fires and will always fire after error, abort, or load
|
||||
**timeout**: fires when progression is terminated due to preset time expiring
|
||||
**readystatechange**: fires when the readyState attribute of a document has changed
|
||||
|
||||
**Alternative `XMLHttpRequest` using `onLoad`**:
|
||||
|
||||
```js
|
||||
var request = new XMLHttpRequest();
|
||||
request.open('GET', 'myservice/username?id=some-unique-id');
|
||||
request.onload = function(){
|
||||
if(request.status ===200){
|
||||
console.log("User's name is "+ request.responseText);
|
||||
} else {
|
||||
console.log('Request failed. Returned status of '+ request.status);
|
||||
}
|
||||
};
|
||||
request.send();
|
||||
```
|
||||
|
||||
**Alternative `XMLHttpRequest` using `readyState`**:
|
||||
|
||||
```js
|
||||
var request = new XMLHttpRequest(), method ='GET', url ='https://developer.mozilla.org/';
|
||||
|
||||
request.open(method, url, true);
|
||||
request.onreadystatechange = function(){
|
||||
if(request.readyState === XMLHttpRequest.DONE && request.status === 200){
|
||||
console.log(request.responseText);
|
||||
}
|
||||
};
|
||||
request.send();
|
||||
```
|
||||
|
||||
`XMLHttpRequest.readyState` values:
|
||||
`0` `UNSENT`: Client has been created. `open()` not called yet.
|
||||
`1` `OPENED`: `open()` has been called.
|
||||
`2` `HEADERS_RECEIVED`: `send()` has been called, and headers and status are available.
|
||||
`3` `LOADING`: Downloading; `responseText` holds partial data.
|
||||
`4` `DONE`: The operation is complete.
|
||||
|
||||
### `XMLHttpRequest` Browser compatibility
|
||||
|
||||
Old versions of IE don’t implement XMLHttpRequest. You must use the ActiveXObject if XMLHttpRequest is not available
|
||||
|
||||
```js
|
||||
var request =window.XMLHttpRequest ? new XMLHttpRequest() : new ActiveXObject('Microsoft.XMLHTTP');
|
||||
|
||||
// OR
|
||||
|
||||
var request;
|
||||
if(window.XMLHttpRequest){
|
||||
// code for modern browsers
|
||||
request = new XMLHttpRequest();
|
||||
} else {
|
||||
// code for old IE browsers
|
||||
request = new ActiveXObject('Microsoft.XMLHTTP');
|
||||
}
|
||||
```
|
||||
|
||||
## Status & Error Handling
|
||||
|
||||
Always inform the user when something is loading. Check the status and give feedback (a loader or message)
|
||||
Errors and responses need to be handled. There is no guarantee that HTTP requests will always succeed.
|
||||
|
||||
### Cross Domain Policy
|
||||
|
||||
Cross domain requests have restrictions.
|
||||
|
||||
Examples of outcome for requests originating from: `http://store.company.com/dir/page.htmlCross-origin`
|
||||
|
||||
| URL | Outcome | Reason |
|
||||
|-------------------------------------------------|---------|--------------------|
|
||||
| `http://store.company.com/dir2/other.html` | success |
|
||||
| `http://store.company.com/dir/inner/other.html` | success |
|
||||
| `https://store.company.com/secure.html` | failure | Different protocol |
|
||||
| `http://store.company.com:81/dir/other.html` | failure | Different port |
|
||||
| `http://news.company.com/dir/other.html` | failure | Different host |
|
164
JavaScript/DOM.md
Normal file
164
JavaScript/DOM.md
Normal file
|
@ -0,0 +1,164 @@
|
|||
# Document Object Model (DOM)
|
||||
|
||||
The **Document Object Model** is a *map* of the HTML document. Elements in an HTML document can be accessed, changed, deleted, or added using the DOM.
|
||||
The document object is *globally available* in the browser. It allows to access and manipulate the DOM of the current web page.
|
||||
|
||||
## DOM Access
|
||||
|
||||
### Selecting Nodes from the DOM
|
||||
|
||||
`getElementById()` and `querySelector()` return a single element.
|
||||
`getElementsByClassName()`, `getElementsByTagName()`, and `querySelectorAll()` return a collection of elements.
|
||||
|
||||
```js
|
||||
Javascript
|
||||
// By Id
|
||||
var node = document.getElementById('id');
|
||||
|
||||
// By Tag Name
|
||||
var node = document.getElementsByTagName('tag');
|
||||
|
||||
// By Class Name
|
||||
var node = document.getElementsByClassName('class');
|
||||
|
||||
// By CSS Query
|
||||
var node = document.querySelector('css-selector');
|
||||
var node = document.querySelectorAll('css-selector');
|
||||
```
|
||||
|
||||
## Manupulating the DOM
|
||||
|
||||
### Manipulating a node's attributes
|
||||
|
||||
It's possible access and change the attributes of a DOM node using the *dot notation*.
|
||||
|
||||
```js
|
||||
// Changing the src of an image:
|
||||
var image = document.getElementById('id');
|
||||
var oldImageSource = image.src;
|
||||
image.src = 'image-url';
|
||||
|
||||
//Changing the className of a DOM node:
|
||||
var node = document.getElementById('id');
|
||||
node.className = 'new-class';
|
||||
```
|
||||
|
||||
### Manipulating a node’s style
|
||||
|
||||
It's possible to access and change the styles of a DOM nodes via the **style** property.
|
||||
CSS property names with a `-` must be **camelCased** and number properties must have a unit.
|
||||
|
||||
```css
|
||||
body {
|
||||
color: red;
|
||||
background-color: pink;
|
||||
padding-top: 10px;
|
||||
}
|
||||
```
|
||||
|
||||
```js
|
||||
var pageNode = document.body;
|
||||
pageNode.style.color = 'red';
|
||||
pageNode.style.backgroundColor = 'pink';
|
||||
pageNode.style.paddingTop = '10px';
|
||||
```
|
||||
|
||||
### Manipulating a node’s contents
|
||||
|
||||
Each DOM node has an `innerHTML` attribute. It contains the HTML of all its children.
|
||||
|
||||
```js
|
||||
var pageNode = document.body;
|
||||
console.log(pageNode.innerHTML);
|
||||
|
||||
// Set innerHTML to replace the contents of the node:
|
||||
pageNode.innerHTML = "<h1>Oh, no! Everything is gone!</h1>";
|
||||
|
||||
// Or add to innerHTML instead:
|
||||
pageNode.innerHTML += "P.S. Please do write back.";
|
||||
```
|
||||
|
||||
To change the actual text of a node, `textContent` may be a better choice:
|
||||
|
||||
`innerHTML`:
|
||||
|
||||
- Works in older browsers
|
||||
- **More powerful**: can change code
|
||||
- **Less secure**: allows cross-site scripting (XSS)
|
||||
|
||||
`textContent`:
|
||||
|
||||
- Doesn't work in IE8 and below
|
||||
- **Faster**: the browser doesn't have to parse HTML
|
||||
- **More secure**: won't execute code
|
||||
|
||||
### Reading Inputs From A Form
|
||||
|
||||
In `page.html`:
|
||||
|
||||
```html
|
||||
<input type="" id="identifier" value="">
|
||||
```
|
||||
|
||||
In `script.js`:
|
||||
|
||||
```js
|
||||
var formNode = document.getEleementById("Identifier");
|
||||
var value = formNode.value;
|
||||
```
|
||||
|
||||
## Creating & Removing DOM Nodes
|
||||
|
||||
The document object also allows to create new nodes from scratch.
|
||||
|
||||
```js
|
||||
// create node
|
||||
document.createElement('tagName');
|
||||
document.createTextNode('text');
|
||||
|
||||
domNode.appendChild(childToAppend); // insert childTaAppend after domNode
|
||||
|
||||
// insert node before domNode
|
||||
domNode.insertBEfore(childToInsert, domnode);
|
||||
domNode.parentNode.insertBefore(childToInsert, nodeAfterChild);
|
||||
|
||||
// remove a node
|
||||
domnNod.removeChild(childToRemove);
|
||||
node.parentNode.removeChild(node);
|
||||
```
|
||||
|
||||
Example:
|
||||
|
||||
```js
|
||||
var body = document.body;
|
||||
|
||||
var newImg = document.createElement('img');
|
||||
newImg.src = 'http://placekitten.com/400/300';
|
||||
newImg.style.border = '1px solid black';
|
||||
|
||||
body.appendChild(newImg);
|
||||
|
||||
var newParagraph = document.createElement('p');
|
||||
var newText = document.createTextNode('Squee!');
|
||||
|
||||
newParagraph.appendChild(newText);
|
||||
|
||||
body.appendChild(newParagraph);
|
||||
```
|
||||
|
||||
### Creating DOM Nodes with Constructor Functions
|
||||
|
||||
```js
|
||||
function Node(params) {
|
||||
this.node = document.createElement("tag");
|
||||
|
||||
this.node.attrubute = value;
|
||||
|
||||
// operations on the node
|
||||
|
||||
return this.node;
|
||||
}
|
||||
|
||||
var node = Node(params);
|
||||
domElement.appendChild(node);
|
||||
```
|
81
JavaScript/Events & Animation.md
Normal file
81
JavaScript/Events & Animation.md
Normal file
|
@ -0,0 +1,81 @@
|
|||
# Events & Animation
|
||||
|
||||
## Events
|
||||
|
||||
Event Types:
|
||||
|
||||
- **Mouse Events**: `mousedown`, `mouseup`, `click`, `dbclick`, `mousemove`, `mouseover`, `mousewheel`, `mouseout`, `contextmenu`, ...
|
||||
- **Touch Events**: `touchstart`, `touchmove`, `touchend`, `touchcancel`, ...
|
||||
- **Keyboard Events**: `keydown`, `keypress`, `keyup`, ...
|
||||
- **Form Events**: `focus`, `blur`, `change`, `submit`, ...
|
||||
- **Window Events**: `scroll`, `resize`, `hashchange`, `load`, `unload`, ...
|
||||
|
||||
### Managing Event Listeners
|
||||
|
||||
```js
|
||||
var domNode = document.getElementById("id");
|
||||
|
||||
var onEvent = function(event) { // parameter contains info on the triggered event
|
||||
// logic here
|
||||
}
|
||||
|
||||
domNode.addEventListener(eventType, calledback);
|
||||
domNode.renoveEventListener(eventType, callback);
|
||||
```
|
||||
|
||||
### Bubbling & Capturing
|
||||
|
||||
Events in Javascript propagate through the DOM tree.
|
||||
|
||||
[Bubbling and Capturing](https://javascript.info/bubbling-and-capturing)
|
||||
[What Is Event Bubbling in JavaScript? Event Propagation Explained](https://www.sitepoint.com/event-bubbling-javascript/)
|
||||
|
||||
### Dispatching Custom Events
|
||||
|
||||
Event Options:
|
||||
|
||||
- `bubbles` (bool): whether the event propagates through bubbling
|
||||
- `cancellable` (bool): if `true` the "default action" may be prevented
|
||||
|
||||
```js
|
||||
let event = new Event(type [,options]); // create the event, type can be custom
|
||||
domNode.dispatchEvent(event); // launch the event
|
||||
```
|
||||
|
||||
## Animation
|
||||
|
||||
The window object is the assumed global object on a page.
|
||||
|
||||
Animation in JavascriptThe standard way to animate in JS is to use window methods.
|
||||
It's possible to animate CSS styles to change size, transparency, position, color, etc.
|
||||
|
||||
```js
|
||||
//Calls a function once after a delay
|
||||
window.setTimeout(callbackFunction, delayMilliseconds);
|
||||
|
||||
//Calls a function repeatedly, with specified interval between each call
|
||||
window.setInterval(callbackFunction, delayMilliseconds);
|
||||
|
||||
//To stop an animation store the timer into a variable and clear it
|
||||
window.clearTimeout(timer);
|
||||
window.clearInterval(timer);
|
||||
```
|
||||
|
||||
### Element Position & dimensions
|
||||
|
||||
[StackOverflow](https://stackoverflow.com/a/294273/8319610)
|
||||
[Wrong dimensions at runtime](https://stackoverflow.com/a/46772849/8319610)
|
||||
|
||||
```js
|
||||
> console.log(document.getElementById('id').getBoundingClientRect());
|
||||
DOMRect {
|
||||
bottom: 177,
|
||||
height: 54.7,
|
||||
left: 278.5,
|
||||
right: 909.5,
|
||||
top: 122.3,
|
||||
width: 631,
|
||||
x: 278.5,
|
||||
y: 122.3,
|
||||
}
|
||||
```
|
959
JavaScript/JavaScript.md
Normal file
959
JavaScript/JavaScript.md
Normal file
|
@ -0,0 +1,959 @@
|
|||
# JavaScript Cheat Sheet
|
||||
|
||||
## Basics
|
||||
|
||||
### Notable javascript engines
|
||||
|
||||
- **Chromium**: `V8` from Google
|
||||
- **Firefox**: `SpiderMonkey` from Mozilla
|
||||
- **Safari**: `JavaScriptCore` from Apple
|
||||
- **Internet Explorer**: `Chakra` from Microsoft
|
||||
|
||||
### Comments
|
||||
|
||||
```javascript
|
||||
//single line comment
|
||||
/*multiline comment*/
|
||||
```
|
||||
|
||||
### File Header
|
||||
|
||||
```javascript
|
||||
/**
|
||||
* @file filename.js
|
||||
* @author author's name
|
||||
* purpose of file
|
||||
*
|
||||
* detailed explanantion of what the file does on multiple lines
|
||||
*/
|
||||
```
|
||||
|
||||
### Naming Conventions
|
||||
|
||||
Elements | Case
|
||||
----------|-----------
|
||||
variable | camelCase
|
||||
|
||||
### Modern Mode
|
||||
|
||||
If located at the top of the script the whole script works the “modern” way (enables post-ES5 functionalities).
|
||||
`"use strict"`
|
||||
|
||||
### Pop-Up message
|
||||
|
||||
Interrupts script execution until closure, **to be avoided**
|
||||
|
||||
```javascript
|
||||
alert("message");
|
||||
```
|
||||
|
||||
### Print message to console
|
||||
|
||||
`console.log(value);`
|
||||
|
||||
## Variables
|
||||
|
||||
### Declaration & Initialization
|
||||
|
||||
[var vs let vs const](https://www.freecodecamp.org/news/var-let-and-const-whats-the-difference/)
|
||||
|
||||
Variable names can only contain numbers, digits, underscores and $. Varieble names are camelCase.
|
||||
|
||||
`let`: Block-scoped; access to variable restricted to the nearest enclosing block.
|
||||
`var`: Function-scoped
|
||||
|
||||
`let variable1 = value1, variable2 = value2;`
|
||||
`var variable1 = value1, variable2 = value2;`
|
||||
|
||||
### Scope
|
||||
|
||||
Variabled declared with `let` are in local to the code block in which are declared.
|
||||
Variabled declared with `var` are local only if declared in a function.
|
||||
|
||||
```js
|
||||
function func(){
|
||||
variable = value; // implicitly declared as a global variable
|
||||
var variable = value; // local variable
|
||||
}
|
||||
|
||||
var a = 10; // a is 10
|
||||
let b = 10; // b is 10
|
||||
{
|
||||
var x = 2, a = 2; // a is 2
|
||||
let y = 2, b = 2; // b is 2
|
||||
}
|
||||
// a is 2, b is 10
|
||||
// x can NOT be used here
|
||||
// y CAN be used here
|
||||
```
|
||||
|
||||
### Constants
|
||||
|
||||
Hard-coded values are UPPERCASE and snake_case, camelCase otherwise.
|
||||
`const CONSTANT = value;`
|
||||
|
||||
## Data Types
|
||||
|
||||
`Number`, `String`, `Boolean`, etc are *built-in global objects*. They are **not** types. **Do not use them for type checking**.
|
||||
|
||||
### Numeric data types
|
||||
|
||||
Only numeric type is `number`.
|
||||
|
||||
```javascript
|
||||
let number = 10; //integer numbers
|
||||
number = 15.7; //floating point numbers
|
||||
number = Infinity; //mathematical infinity
|
||||
number = - Infinity;
|
||||
number = 1234567890123456789012345678901234567890n; //BigInt, value > 2^53, "n" at the end
|
||||
number = "text" / 2; //NaN --> not a number.
|
||||
```
|
||||
|
||||
[Rounding Decimals in JavaScript](https://www.jacklmoore.com/notes/rounding-in-javascript/)
|
||||
[Decimal.js](https://github.com/MikeMcl/decimal.js)
|
||||
|
||||
Mathematical expression will *never* cause an error. At worst the result will be NaN.
|
||||
|
||||
### String data type
|
||||
|
||||
```javascript
|
||||
let string = "text";
|
||||
let string$ = 'text';
|
||||
let string_ = `text ${expression}`; //string interpolation (needs backticks)
|
||||
|
||||
string.length; // length of the string
|
||||
let char = string.charAt(index); // extaraction of a single character by position
|
||||
string[index]; // char extraction by property access
|
||||
let index = strinf.indexOf(substring); // start index of substring in string
|
||||
```
|
||||
|
||||
Property access is unpredictable:
|
||||
|
||||
- does not work in IE7 or earlier
|
||||
- makes strings look like arrays (confusing)
|
||||
- if no character is found, `[ ]` returns undefined, `charAt()` returns an empty string
|
||||
- Is read only: `string[index] = "value"` does not work and gives no errors
|
||||
|
||||
### [Slice vs Substring vs Substr](https://stackoverflow.com/questions/2243824/what-is-the-difference-between-string-slice-and-string-substring)
|
||||
|
||||
If the parameters to slice are negative, they reference the string from the end. Substring and substr doesn´t.
|
||||
|
||||
```js
|
||||
string.slice(begin [, end]);
|
||||
string.substring(from [, to]);
|
||||
string.substr(start [, length]);
|
||||
```
|
||||
|
||||
### Boolean data type
|
||||
|
||||
```javascript
|
||||
let boolean = true;
|
||||
let boolean_ = false;
|
||||
```
|
||||
|
||||
### Null data type
|
||||
|
||||
```javascript
|
||||
let _ = null;
|
||||
```
|
||||
|
||||
### Undefined
|
||||
|
||||
```javascript
|
||||
let $; //value is "undefined"
|
||||
$ = undefined;
|
||||
```
|
||||
|
||||
### Typeof()
|
||||
|
||||
```javascript
|
||||
typeof x; //returns the type of the variable x as a string
|
||||
typeof(x); //returns the type of the variable x as a string
|
||||
```
|
||||
|
||||
The result of typeof null is "object". That’s wrong.
|
||||
It is an officially recognized error in typeof, kept for compatibility. Of course, null is not an object.
|
||||
It is a special value with a separate type of its own. So, again, this is an error in the language.
|
||||
|
||||
### Type Casting
|
||||
|
||||
```javascript
|
||||
String(value); //converts value to string
|
||||
|
||||
Number(value); //converst value to a number
|
||||
Number(undefined); //--> NaN
|
||||
Number(null); //--> 0
|
||||
Number(true); //--> 1
|
||||
Number(false); //--> 0
|
||||
Number(String); //Whitespaces from the start and end are removed. If the remaining string is empty, the result is 0. Otherwise, the number is “read” from the string. An error gives NaN.
|
||||
|
||||
Boolean(value); //--> true
|
||||
Boolean(0); //--> false
|
||||
Boolean(""); //--> false
|
||||
Boolean(null); //--> false
|
||||
Boolean(undefined); //--> false
|
||||
Boolean(NaN); //--> false
|
||||
|
||||
|
||||
//numeric type checking the moronic way
|
||||
typeof var_ == "number"; // typeof returns a string with the name of the type
|
||||
```
|
||||
|
||||
### Type Checking
|
||||
|
||||
```js
|
||||
isNaN(var); // converts var in number and then check if is NaN
|
||||
|
||||
Number("A") == NaN; //false ?!?
|
||||
|
||||
```
|
||||
|
||||
### Dangerous & Stupid Implicit Type Casting
|
||||
|
||||
```js
|
||||
2 + 'text'; //"2text", implicit conversion and concatenation
|
||||
1 + "1"; //"11", implicit conversion and concatention
|
||||
"1" + 1; //"11", implicit conversion and concatention
|
||||
+"1"; //1, implicit conversion
|
||||
+"text"; // NaN
|
||||
1 == "1"; //true
|
||||
1 === "1"; //false
|
||||
1 == true; //true
|
||||
0 == false; //true
|
||||
"" == false; //true
|
||||
```
|
||||
|
||||
## Operators
|
||||
|
||||
Operator | Operation
|
||||
---------------|----------------
|
||||
`(...)` | grouping
|
||||
a`.`b | member access
|
||||
`new` a(...) | object creation
|
||||
a `in` b | membership
|
||||
|
||||
### Mathemetical Operators
|
||||
|
||||
Operator | Operation
|
||||
-----------|----------------
|
||||
a `+` b | addition
|
||||
a `-` b | subtraction
|
||||
a `*` b | multiplication
|
||||
a `**` b | a^b
|
||||
a `/` b | division
|
||||
a `%` b | modulus
|
||||
|
||||
### Unary Increment Operators
|
||||
|
||||
Operator | Operation
|
||||
--------------|------------------
|
||||
`--`variable | prefix decrement
|
||||
`++`variable | prefix incremente
|
||||
variable`--` | postfiz decrement
|
||||
variable`++` | ostfix increment
|
||||
|
||||
### Logical Operators
|
||||
|
||||
Operator | Operation
|
||||
---------|----------------
|
||||
a `&&` b | logical **AND**
|
||||
a `||` b | logical **OR**
|
||||
`!`a | logical **NOT**
|
||||
|
||||
### Comparison Operators
|
||||
|
||||
Operator | Operation
|
||||
----------|--------------------
|
||||
a `<` b | less than
|
||||
a `<=` b | less or equal to
|
||||
a `>` b | greater than
|
||||
a `>=` b | greater or equal to
|
||||
a `==` b | equaltity
|
||||
a `!=` b | inequality
|
||||
a `===` b | strict equality
|
||||
a `!==` b | strict inequality
|
||||
|
||||
### Bitwise Logical Operators
|
||||
|
||||
Operator | Operation
|
||||
-----------|-----------------------------
|
||||
a `&` b | bitwise AND
|
||||
a `|` b | bitwise OR
|
||||
a `^` b | bitwise XOR
|
||||
`~`a | bitwise NOT
|
||||
a `<<` b | bitwise left shift
|
||||
a `>>` b | bitwise rigth sigt
|
||||
a `>>>` b | bitwise unsigned rigth shift
|
||||
|
||||
### Compound Operators
|
||||
|
||||
Operator | Operation
|
||||
------------|-------------
|
||||
a `+=` b | a = a + b
|
||||
a `-=` b | a = a - b
|
||||
a `*=` b | a = a * b
|
||||
a `**=` b | a = a ** b
|
||||
a `/=` b | a = a / b
|
||||
a `%=` b | a = a % b
|
||||
a `<<=` b | a = a << b
|
||||
a `>>=` b | a = a >> b
|
||||
a `>>>=` b | a = a >>> b
|
||||
a `&=` b | a = a & b
|
||||
a `^=` b | a = a ^ b
|
||||
a `|=` b | a = a ! b
|
||||
|
||||
## Decision Statements
|
||||
|
||||
### IF-ELSE
|
||||
|
||||
```javascript
|
||||
if (condition) {
|
||||
//code here
|
||||
} else {
|
||||
//code here
|
||||
}
|
||||
```
|
||||
|
||||
### IF-ELSE Multi-Branch
|
||||
|
||||
```javascript
|
||||
if (condition) {
|
||||
//code here
|
||||
} else if (condition) {
|
||||
//code here
|
||||
} else {
|
||||
//code here
|
||||
}
|
||||
```
|
||||
|
||||
### Ternary Operator
|
||||
|
||||
`condition ? instruction1 : istruction2;`
|
||||
Ff TRUE execute instruction1, execute instruction2 otherwise.
|
||||
|
||||
### Switch Statement
|
||||
|
||||
```javascript
|
||||
switch (expression) {
|
||||
case expression:
|
||||
//code here
|
||||
break;
|
||||
|
||||
default:
|
||||
//code here
|
||||
break;
|
||||
}
|
||||
```
|
||||
|
||||
## Loops
|
||||
|
||||
### While Loop
|
||||
|
||||
```javascript
|
||||
while (condition) {
|
||||
//code here
|
||||
}
|
||||
```
|
||||
|
||||
### Do-While Loop
|
||||
|
||||
```javascript
|
||||
do {
|
||||
//code here
|
||||
} while (condition);
|
||||
```
|
||||
|
||||
### For Loop
|
||||
|
||||
```javascript
|
||||
// baseic for
|
||||
for (begin; condition; step) { }
|
||||
|
||||
for (var variable in iterable) { } // for/in statement loops through the properties of an object
|
||||
for (let variable in iterable) { } // inistatiate a new variable at each iteration
|
||||
|
||||
// for/of statement loops through the values of an iterable objects
|
||||
// for/of lets you loop over data structures that are iterable such as Arrays, Strings, Maps, NodeLists, and more.
|
||||
for (var variable of iterable) { }
|
||||
for (let variable of iterable) { } // inistatiate a new variable at each iteration
|
||||
|
||||
// foreach (similar to for..of)
|
||||
itearble.forEach(() => { /* statements */ });
|
||||
```
|
||||
|
||||
### Break & Continue statements
|
||||
|
||||
`break;` exits the loop.
|
||||
`continue;` skip to next loop cycle.
|
||||
|
||||
```javascript
|
||||
labelname: for(begin; condition; step) {
|
||||
//code here
|
||||
}
|
||||
|
||||
break labelname; //breaks labelled loop and nested loops inside it
|
||||
```
|
||||
|
||||
## Arrays
|
||||
|
||||
```js
|
||||
let array = []; // empty array
|
||||
let array = ["text", 3.14, [1.41]]; // array declaration and initialization
|
||||
|
||||
array.length; // number of items in the array
|
||||
array[index]; // access to item by index
|
||||
array[index] = item; // change or add item by index
|
||||
|
||||
array.push(item); //add item to array
|
||||
array.pop(); // remove and return last item
|
||||
|
||||
array.join("separator"); // constuct a string from the items of the array, sepatated by SEPARATOR
|
||||
array.find(item => condition); // returns the value of the first element in the provided array that satisfies the provided testing function
|
||||
array.fill(value, start, end); // filla an array with the passed value
|
||||
|
||||
|
||||
// https://stackoverflow.com/a/37601776
|
||||
array.slice(start, end); // RETURN list of items between indexes start and end-1
|
||||
array.splice(start, deleteCount, [items_to_add]); // remove and RETURN items from array, can append a list of items. IN PLACE operation
|
||||
```
|
||||
|
||||
### `filter()` & `map()`, `reduce()`
|
||||
|
||||
```js
|
||||
let array = [ items ];
|
||||
|
||||
// execute an operation on each item, producing a new array
|
||||
array.map(function);
|
||||
array.map(() => operation);
|
||||
|
||||
array.filter(() => condition); // return an items only if the condition is true
|
||||
|
||||
// execute a reducer function on each element of the array, resulting in single output value
|
||||
array.reduce((x, y) => ...);
|
||||
```
|
||||
|
||||
## Spread Operator (...)
|
||||
|
||||
```js
|
||||
// arrays
|
||||
let array1 = [ 1, 2, 3, 4, 5, 6 ];
|
||||
let array2 = [ 7, 8, 9, 10 ];
|
||||
let copy = [ ...array1 ]; // shallow copy
|
||||
let copyAndAdd = [ 0, ...array1, 7 ]; // insert all values in new array
|
||||
let merge = [ ...array1, ...attay2 ]; // merge the arrays contents in new array
|
||||
|
||||
// objects
|
||||
let obj = { prop1: value1, prop2: value2 };
|
||||
let clone = { ...obj };
|
||||
let cloneAndAdd = { prop0: value0, ...obj, prop3: value3 };
|
||||
|
||||
// strings
|
||||
let alphabet = "abcdefghijklmnopqrstxyz"
|
||||
let letters = [ ...alphabet ]; // alphabet.split("")
|
||||
|
||||
//function arguments
|
||||
let func = (arg1 = val1, arg2 = val2) => expression;
|
||||
let args = [ value1, value2 ];
|
||||
func(arg0, ...args);
|
||||
```
|
||||
|
||||
## Dictionaries
|
||||
|
||||
```js
|
||||
let dict = { FirstName: "Chris", "one": 1, 1: "some value" };
|
||||
|
||||
|
||||
// add new or update property
|
||||
dict["Age"] = 42;
|
||||
|
||||
// direct property by name
|
||||
// because it's a dynamic language
|
||||
dict.FirstName = "Chris";
|
||||
```
|
||||
|
||||
### Iterating Key-Value pairs
|
||||
|
||||
```js
|
||||
for(let key in dict) {
|
||||
let value = dict[key];
|
||||
|
||||
// do something with "key" and "value" variables
|
||||
}
|
||||
|
||||
```
|
||||
|
||||
## Functions
|
||||
|
||||
### JSDOC documentation standard
|
||||
|
||||
```javascript
|
||||
/**
|
||||
* @param {type} parameter - description
|
||||
* @returns {type} parameter - description
|
||||
* */
|
||||
```
|
||||
|
||||
### Function Declaration
|
||||
|
||||
```javascript
|
||||
// ...args will contain extra parameters (rest argument)
|
||||
function functionName(parameter=default-value, ...args) {
|
||||
//code here
|
||||
return <expression>;
|
||||
}
|
||||
```
|
||||
|
||||
### Default Parameters (old versions)
|
||||
|
||||
```javascript
|
||||
function functionName(parameters) {
|
||||
if (parameter == undefined) {
|
||||
paremeter = value;
|
||||
}
|
||||
|
||||
//code here
|
||||
return <expression>;
|
||||
}
|
||||
```
|
||||
|
||||
### Function Expressions
|
||||
|
||||
```javascript
|
||||
let functionName = function(parameters) {
|
||||
//code here
|
||||
return expression;
|
||||
}
|
||||
```
|
||||
|
||||
### Arrow Functions
|
||||
|
||||
```javascript
|
||||
(input) => { /* statements */ }
|
||||
(input) => expression;
|
||||
input => expression; // parenthesis are optional
|
||||
() => expression; // no parameters syntax
|
||||
|
||||
// variants
|
||||
let func = (input) => {
|
||||
// code here
|
||||
};
|
||||
|
||||
let func = (input) => expression;
|
||||
let func = input => expression;
|
||||
|
||||
func(); // function call
|
||||
|
||||
// rerurn object literal
|
||||
let func = (value) => ({property: value});
|
||||
```
|
||||
|
||||
## Object Oriented Programming
|
||||
|
||||
An object is a collection of related data and/or functionality.
|
||||
|
||||
**Note**: It's not possible to transform a variable in an object simply by using the object assignement.
|
||||
|
||||
```js
|
||||
let variable = value;
|
||||
|
||||
// object literal
|
||||
let obj = {
|
||||
property: value,
|
||||
variable, // instead of variable: variable to use the variable's value -> variable: value
|
||||
|
||||
object: {
|
||||
...
|
||||
},
|
||||
|
||||
method: function() {
|
||||
// code here
|
||||
this.properyName; // reference to object property inside the object
|
||||
}
|
||||
|
||||
method; () => {
|
||||
obj.propertyName; // this is undefined here, use full object name
|
||||
}
|
||||
};
|
||||
|
||||
// access to property (non existant porperties will return Undefined)
|
||||
obj.property; // dot notation
|
||||
obj["property"]; // array notation
|
||||
|
||||
// property modification (will add property if missing)
|
||||
obj.property = value; // dot notation
|
||||
obj["property"] = value; // array notation
|
||||
|
||||
obj.func(); //method access
|
||||
|
||||
delete obj.propertyName; // delete property
|
||||
|
||||
Object.keys(obj); // list of all property names
|
||||
Object.entries(obj); // list contents as key-value pairs
|
||||
```
|
||||
|
||||
### Constructors and object instances
|
||||
|
||||
JavaScript uses special functions called **constructor functions** to define and initialize objects and their features.
|
||||
Notice that it has all the features you'd expect in a function, although it doesn't return anything or explicitly create an object — it basically just defines properties and methods.
|
||||
|
||||
```js
|
||||
// constructor function definition
|
||||
function Class(params) {
|
||||
this.property = param;
|
||||
|
||||
this.method = function(parms) { /* code here */ }
|
||||
}
|
||||
|
||||
let obj = new Class(params); // object instantiation
|
||||
|
||||
let obj = new Object(); // cretaes empty object
|
||||
let obj = new Object({
|
||||
// JSON
|
||||
});
|
||||
```
|
||||
|
||||
### Prototypes
|
||||
|
||||
Prototypes are the mechanism by which JavaScript objects *inherit* features from one another.
|
||||
|
||||
JavaScript is often described as a **prototype-based language**; to provide inheritance, objects can have a prototype object, which acts as a template object that it inherits methods and properties from.
|
||||
|
||||
An object's prototype object may also have a prototype object, which it inherits methods and properties from, and so on.
|
||||
This is often referred to as a **prototype chain**, and explains why different objects have properties and methods defined on other objects available to them.
|
||||
If a method is implemented on an object (and not it's prototype) then only that object will heve that method and not all the ones that come from the same prototype.
|
||||
|
||||
```js
|
||||
// constuctor function
|
||||
function Obj(param1, ...) {
|
||||
this.param1 = param1,
|
||||
...
|
||||
}
|
||||
|
||||
// method on the object
|
||||
Obj.prototype.method = function(params) {
|
||||
// code here (operate w/ this)
|
||||
}
|
||||
|
||||
let obj = new Obj(args); // object instantiation
|
||||
obj.method(); // call method from prototype
|
||||
```
|
||||
|
||||
### Extending with prototypes
|
||||
|
||||
```js
|
||||
// constructor function
|
||||
function DerivedObj(param1, param2, ...) {
|
||||
Obj.call(this, param1); // use prototype constructor
|
||||
this.param2 = param2;
|
||||
}
|
||||
|
||||
// extend Obj
|
||||
DerivedObj.prototype = Object.create(Obj.prototype);
|
||||
|
||||
// method on object
|
||||
DerivedObj.prototype.method = function() {
|
||||
// code here (operate w/ this)
|
||||
}
|
||||
|
||||
let dobj = new DerivedObj(args); // object instantiation
|
||||
dobj.method(); // call method from prototype
|
||||
```
|
||||
|
||||
### Classes (ES6+)
|
||||
|
||||
```js
|
||||
class Obj {
|
||||
constructor(param1, ...) {
|
||||
this.param1 = param1,
|
||||
...
|
||||
}
|
||||
|
||||
get param1() // getter
|
||||
{
|
||||
return this.param1;
|
||||
}
|
||||
|
||||
func() {
|
||||
// code here (operate w/ this)
|
||||
}
|
||||
|
||||
static func() { } // static method
|
||||
|
||||
// object instantiation
|
||||
let obj = new Obj(param1, ...);
|
||||
obj.func(); // call method
|
||||
```
|
||||
|
||||
### Extending with Classes
|
||||
|
||||
```js
|
||||
class DerivedObj extends Obj {
|
||||
constructor(param1, param2, ...){
|
||||
super(param1); // use superclass constructor
|
||||
this.param2 = param2;
|
||||
}
|
||||
|
||||
newFunc() { }
|
||||
}
|
||||
|
||||
let dobj = DerivedObj();
|
||||
dobj.newFunc();
|
||||
```
|
||||
|
||||
## Deconstruction
|
||||
|
||||
### Object deconstruction
|
||||
|
||||
```js
|
||||
let obj = {
|
||||
property: value,
|
||||
...
|
||||
}
|
||||
|
||||
let { var1, var2 } = obj; // extract values from object into variables
|
||||
let { property: var1, property2 : var2 } = obj; // extract props in variables w/ specified names
|
||||
let { property: var1, var2 = defalut_value } = obj; // use default values if object has less then expected props
|
||||
```
|
||||
|
||||
### Array Deconstrions
|
||||
|
||||
```js
|
||||
let array = [ 1, 2, 3, 4, 5, 6 ];
|
||||
let [first, , third, , seventh = "missing" ] = array; // extract specific values from array
|
||||
```
|
||||
|
||||
## Serialization
|
||||
|
||||
```js
|
||||
let object = {
|
||||
// ojectt attributes
|
||||
}
|
||||
|
||||
let json = JSON.stringify(object); // serialieze onbect in JSON
|
||||
|
||||
let json = { /* JSON */ };
|
||||
let object = JSON.parse(json); // deserialize to Object
|
||||
```
|
||||
|
||||
## Timing
|
||||
|
||||
### Timers
|
||||
|
||||
Function runs *once* after an interval of time.
|
||||
|
||||
```js
|
||||
// param1, param2, ... are the arguments passed to the function (IE9+)
|
||||
let timerId = setTimeout(func [, milliseconds, param1, param2, ... ]); // wait milliseconds before executing the code (params are read at execution time)
|
||||
|
||||
// works in IE9
|
||||
let timerId = setTimeout(function(){
|
||||
func(param1, param2);
|
||||
}, milliseconds);
|
||||
|
||||
// Anonymous functions with arguments
|
||||
let timerId = setTimeout(function(arg1, ...){
|
||||
// code here
|
||||
}, milliseconds, param1, ...);
|
||||
|
||||
clearTimeout(timerId) // cancel execution
|
||||
|
||||
// exemple of multiple consecutive schedules
|
||||
let list = [1 , 2, 3, 4, 5, 6, 7, 8, 9, 10, "A", "B", "C", "D", "E", "F", "G", "H", "I", "J", -1, -2, -3, -4, -5, -6, -7, -8, -9, -10]
|
||||
function useTimeout(pos=0) {
|
||||
|
||||
setTimeout(function(){
|
||||
console.log(list[pos]);
|
||||
pos += 1; // update value for next call
|
||||
|
||||
if (pos < list.length) { // recursion exit condition
|
||||
useTimeout(pos); // schedule next call with new walue
|
||||
}
|
||||
}, 1_000, pos);
|
||||
}
|
||||
|
||||
useTimeout();
|
||||
```
|
||||
|
||||
### `let` vs `var` with `setTimeout`
|
||||
|
||||
```js
|
||||
// let instantitates a new variable for each iteration
|
||||
for (let i = 0; i < 3; ++i) {
|
||||
setTimeout(function() {
|
||||
console.log(i);
|
||||
}, i * 100);
|
||||
}
|
||||
// output: 0, 1, 2
|
||||
|
||||
for (var i = 0; i < 3; ++i) {
|
||||
setTimeout(function() {
|
||||
console.log(i);
|
||||
}, i * 100);
|
||||
}
|
||||
// output: 3, 3, 3
|
||||
```
|
||||
|
||||
### Preserving the context
|
||||
|
||||
```js
|
||||
let obj = {
|
||||
prop: value,
|
||||
|
||||
method1 : function() { /* statement */ }
|
||||
|
||||
method2 : function() {
|
||||
let self = this // memorize context inside method (otherwise callback will not know it)
|
||||
setTimeout(function() { /* code here (uses self) */ })
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
// better
|
||||
let obj = {
|
||||
prop: value,
|
||||
|
||||
method1 : function() { /* statement */ }
|
||||
|
||||
method2 : function() {
|
||||
setTimeout(() => { /* code here (uses this) */ }) // arrow func does not create new scope, this context preserved
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Intervals
|
||||
|
||||
Function runs regularly with a specified interval. JavaScript is **Single Threaded**.
|
||||
|
||||
```js
|
||||
// param1, param2, ... are the arguments passed to the function (IE9+)
|
||||
let timerId = setInterval(func, milliseconds [, param1, param2, ... ]); // (params are read at execution time)
|
||||
|
||||
// works in IE9
|
||||
let timerId = setInterval(function(){
|
||||
func(param1, param2);
|
||||
}, milliseconds);
|
||||
|
||||
// Anonymous functions with arguments
|
||||
let timerId = setInterval(function(arg1, ...){
|
||||
// code here
|
||||
}, milliseconds, param1, ...);
|
||||
|
||||
clearTimeout(timerId); // cancel execution
|
||||
```
|
||||
|
||||
## DateTime
|
||||
|
||||
A date consists of a year, a month, a day, an hour, a minute, a second, and milliseconds.
|
||||
|
||||
There are generally 4 types of JavaScript date input formats:
|
||||
|
||||
- **ISO Date**: `"2015-03-25"`
|
||||
- Short Date: `"03/25/2015"`
|
||||
- Long Date: `"Mar 25 2015"` or `"25 Mar 2015"`
|
||||
- Full Date: `"Wednesday March 25 2015"`
|
||||
|
||||
```js
|
||||
// constructors
|
||||
new Date();
|
||||
new Date(milliseconds);
|
||||
new Date(dateString);
|
||||
new Date(year, month, day, hours, minutes, seconds, milliseconds);
|
||||
|
||||
// accepts parameters similar to the Date constructor, but treats them as UTC. It returns the number of milliseconds since January 1, 1970, 00:00:00 UTC.
|
||||
Date.UTC(year, month, day, hours, minutes, seconds, milliseconds);
|
||||
|
||||
//static methods
|
||||
Date.now(); // returns the number of milliseconds elapsed since January 1, 1970 00:00:00 UTC.
|
||||
|
||||
// methods
|
||||
let date = new Date();
|
||||
date.toSting(); // returns a string representing the specified Date object
|
||||
date.toUTCString();
|
||||
date.toDateString();
|
||||
date.toTimeString(); // method returns the time portion of a Date object in human readable form in American English.
|
||||
|
||||
|
||||
// get date
|
||||
|
||||
dare.getMonth();
|
||||
date.getMinutes();
|
||||
date.getFullYear();
|
||||
|
||||
// set date
|
||||
date.setFullYear(2020, 0, 14);
|
||||
date.setDate(date.getDate() + 50);
|
||||
|
||||
// parse valid dates
|
||||
let msec = Date.parse("March 21, 2012");
|
||||
let date = new Date(msec);
|
||||
```
|
||||
|
||||
### Comparing Dates
|
||||
|
||||
Comparison operators work also on dates
|
||||
|
||||
```js
|
||||
let date1 = new Date();
|
||||
let date2 = new Date("May 24, 2017 10:50:00");
|
||||
|
||||
if(date1 > date2){
|
||||
console.log('break time');
|
||||
} else {
|
||||
console.log('stay in class');
|
||||
}
|
||||
```
|
||||
|
||||
## [Exports](https://developer.mozilla.org/en-US/docs/web/javascript/reference/statements/export)
|
||||
|
||||
[Firefox CORS not HTTP](https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS/Errors/CORSRequestNotHttp)
|
||||
|
||||
**NOTE**: Firefox 68 and later define the origin of a page opened using a `file:///` URI as unique. Therefore, other resources in the same directory or its subdirectories no longer satisfy the CORS same-origin rule. This new behavior is enabled by default using the `privacy.file_unique_origin` preference.
|
||||
|
||||
```json
|
||||
"privacy.file_unique_origin": "false"
|
||||
```
|
||||
|
||||
In `page.html`
|
||||
|
||||
```html
|
||||
<!-- must specyfy module as type for importer and source -->
|
||||
<script src="scripts/module.js"></script>
|
||||
<script src="scripts/script.js"></script>
|
||||
```
|
||||
|
||||
In `module.js`:
|
||||
|
||||
```js
|
||||
// exporting indivisual fratures
|
||||
export default function() {} // one per module
|
||||
export func = () => expression; // zero or more per module
|
||||
|
||||
// Export list
|
||||
export { name1, name2, …, nameN };
|
||||
|
||||
// Renaming exports
|
||||
export { variable1 as name1, variable2 as name2, …, nameN };
|
||||
|
||||
// Exporting destructured assignments with renaming
|
||||
export const { name1, name2: bar } = o;
|
||||
|
||||
// re-export
|
||||
export { func } from "other_script.js"
|
||||
```
|
||||
|
||||
In `script.js`:
|
||||
|
||||
```js
|
||||
import default_func_alias, { func as alias } from "./module.js"; // import default and set alias
|
||||
import { default as default_func_alias, func as alias } from "./module.js"; // import default and set alias
|
||||
|
||||
// use imported functions
|
||||
default_func_alias();
|
||||
alias();
|
||||
```
|
||||
|
||||
```js
|
||||
import * from "./module.js"; // import all
|
||||
|
||||
module.function(); // use imported content with fully qualified name
|
||||
```
|
220
JavaScript/jQuery.md
Normal file
220
JavaScript/jQuery.md
Normal file
|
@ -0,0 +1,220 @@
|
|||
# jQuery Library
|
||||
|
||||
## Including jQuery
|
||||
|
||||
### Download and link the file
|
||||
|
||||
```html
|
||||
<head>
|
||||
<script src="jquery-3.2.1.min.js"></script>
|
||||
</head>
|
||||
```
|
||||
|
||||
### Use a CDN
|
||||
|
||||
```html
|
||||
<head>
|
||||
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
|
||||
</head>
|
||||
|
||||
<!-- OR -->
|
||||
|
||||
<head>
|
||||
<script src="https://ajax.aspnetcdn.com/ajax/jQuery/jquery-3.5.1.min.js"></script>
|
||||
</head>
|
||||
```
|
||||
|
||||
### What is a CDN
|
||||
|
||||
A **content delivery network** or **content distribution network** (CDN) is a large distributed system of servers deployed in multiple data centers across the Internet.
|
||||
The goal of a CDN is to serve content to end-users with high availability and high performance.
|
||||
CDNs serve a large fraction of the Internet content today, including web objects (text, graphics and scripts), downloadable objects (media files, software, documents), applications (e-commerce, portals), live streaming media, on-demand streaming media, and social networks.
|
||||
|
||||
## HTML Manipulation
|
||||
|
||||
### [Finding DOM elements](https://api.jquery.com/category/selectors/)
|
||||
|
||||
```js
|
||||
$('tag');
|
||||
$("#id");
|
||||
$(".class");
|
||||
```
|
||||
|
||||
### Manipulating DOM elements
|
||||
|
||||
```js
|
||||
$("p").addClass("special");
|
||||
```
|
||||
|
||||
```html
|
||||
<!-- before -->
|
||||
<p>Welcome to jQuery<p>
|
||||
|
||||
<!-- after -->
|
||||
<p class="special">Welcome to jQuery<p>
|
||||
```
|
||||
|
||||
### Reading Elements
|
||||
|
||||
```html
|
||||
<a id="yahoo" href="http://www.yahoo.com" style="font-size:20px;">Yahoo!</a>
|
||||
```
|
||||
|
||||
```js
|
||||
// find it & store it
|
||||
var link = $('a#yahoo');
|
||||
|
||||
// get info about it
|
||||
link.html(); // 'Yahoo!'
|
||||
link.attr('href'); // 'http://www.yahoo.com'
|
||||
link.css('font-size'); // '20px
|
||||
```
|
||||
|
||||
### Modifying Elements
|
||||
|
||||
```js
|
||||
// jQuery
|
||||
$('a').html('Yahoo!');
|
||||
$('a').attr('href', 'http://www.yahoo.com');
|
||||
$('a').css({'color': 'purple'});
|
||||
```
|
||||
|
||||
```html
|
||||
<!-- before -->
|
||||
<a href="http://www.google.com">Google</a>
|
||||
|
||||
<!-- after -->
|
||||
<a href="http://www.yahoo.com" style="color:purple">Yahoo</a>
|
||||
```
|
||||
|
||||
### Create, Store, Manipulate and inject
|
||||
|
||||
```js
|
||||
let paragraph = $('<p class="intro">Welcome<p>'); // creat and store element
|
||||
|
||||
paragraph.css('propery', 'value'); // manipulate element
|
||||
|
||||
$("body").append(paragraph); // inject in DOM
|
||||
```
|
||||
|
||||
### Regular DOM Nodes to jQuery Objects
|
||||
|
||||
```js
|
||||
var paragraphs = $('p'); // an array
|
||||
var aParagraph = paragraphs[0]; // a regular DOM node
|
||||
var $aParagraph = $(paragraphs[0]); // a jQuery Object
|
||||
|
||||
// can also use loops
|
||||
for(var i = 0; i < paragraphs.length; i++) {
|
||||
var element = paragraphs[i];
|
||||
var paragraph = $(element);
|
||||
paragraph.html(paragraph.html() + ' WOW!');
|
||||
}
|
||||
```
|
||||
|
||||
## [Events](https://api.jquery.com/category/events/)
|
||||
|
||||
```js
|
||||
var onButtonClick = function() {
|
||||
console.log('clicked!');
|
||||
}
|
||||
|
||||
// with named callback & .on
|
||||
$('button').on('click', onButtonClick);
|
||||
|
||||
// with anonymous callback & .on
|
||||
$('button').on('click', function(){
|
||||
console.log('clicked!');
|
||||
});
|
||||
|
||||
// with .click & named callback
|
||||
$('button').click(onButtonClick);
|
||||
```
|
||||
|
||||
### Preventing Default Event
|
||||
|
||||
```js
|
||||
$('selector').on('event', function(event) {
|
||||
event.preventDefault();
|
||||
// custom logic
|
||||
});
|
||||
```
|
||||
|
||||
## Plugins
|
||||
|
||||
In the HTML, add a `<script>` ag that hotlinks to the CDN or source file:
|
||||
|
||||
```html
|
||||
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-validate/1.17.0/jquery.validate.min.js"><script>
|
||||
```
|
||||
|
||||
In the JavaScript call the jQuery puging on the DOM:
|
||||
|
||||
```js
|
||||
$("form").validate();
|
||||
```
|
||||
|
||||
**NOTE**: always link to the [minified](https://developers.google.com/speed/docs/insights/MinifyResources) js files.
|
||||
|
||||
## More jQuery
|
||||
|
||||
### Patters & Anti-Patterns
|
||||
|
||||
```js
|
||||
// Pattern: name variables with $var
|
||||
$myVar =$('#myNode');
|
||||
|
||||
// Pattern: store references to callback functions
|
||||
var callback = function(argument){
|
||||
// do something cool
|
||||
};
|
||||
|
||||
$(document).on('click', 'p', myCallback);
|
||||
|
||||
// Anti-pattern: anonymous functions
|
||||
$(document).on('click', 'p', function(argument){
|
||||
// do something anonymous
|
||||
});
|
||||
```
|
||||
|
||||
### Chaining
|
||||
|
||||
```js
|
||||
banner.css('color', 'red');
|
||||
banner.html('Welcome!');
|
||||
banner.show();
|
||||
|
||||
// same as:
|
||||
banner.css('color', 'red').html('Welcome!').show();
|
||||
|
||||
// same as:
|
||||
banner.css('color', 'red')
|
||||
.html('Welcome!')
|
||||
.show();
|
||||
```
|
||||
|
||||
### DOM Readiness
|
||||
|
||||
DOM manipulation and event binding doesn’t work if the `<script>` is in the `<head>`
|
||||
|
||||
```js
|
||||
$(document).ready(function() {
|
||||
// the DOM is fully loaded
|
||||
});
|
||||
|
||||
$(window).on('load', function(){
|
||||
// the DOM and all assets (including images) are loaded
|
||||
});
|
||||
```
|
||||
|
||||
## AJAX (jQuery `1.5`+)
|
||||
|
||||
```js
|
||||
$.ajax({
|
||||
method: 'POST',
|
||||
url: 'some.php',
|
||||
data: { name: 'John', location: 'Boston'}
|
||||
})
|
||||
.done(function(msg){alert('Data Saved: '+ msg);})
|
||||
.fail(function(jqXHR, textStatus){alert('Request failed: '+ textStatus);});
|
||||
```
|
198
Kotlin/kotlin.md
Normal file
198
Kotlin/kotlin.md
Normal file
|
@ -0,0 +1,198 @@
|
|||
# Kotlin Cheat Sheet
|
||||
|
||||
## Pakage & Imports
|
||||
|
||||
```kotlin
|
||||
package com.app.uniqueID
|
||||
|
||||
import <package>
|
||||
```
|
||||
|
||||
## Variable & Constants
|
||||
|
||||
```kotlin
|
||||
|
||||
var variable: Type //variable declaration
|
||||
var variable = value //type can be omitted if it can be deduced by initialization
|
||||
|
||||
val CONSTANT_NAME: Type = value //constant declaration
|
||||
```
|
||||
|
||||
### Nullable Variables
|
||||
|
||||
For a variable to hold a null value, it must be of a nullable type.
|
||||
Nulalble types are specified suffixing `?` to the variable type.
|
||||
|
||||
```kotlin
|
||||
var nullableVariable: Type? = null
|
||||
|
||||
nullableVariable?.method() //correct way to use
|
||||
//if var is null dont execute method() and return null
|
||||
|
||||
nullablevariavle!!.method() //unsafe way
|
||||
//!! -> ignore that var can be null
|
||||
```
|
||||
|
||||
## Decision Statements
|
||||
|
||||
### `If` - `Else If` - `Else`
|
||||
|
||||
```kotlin
|
||||
if (condition) {
|
||||
//code here
|
||||
} else if (condition) {
|
||||
//code here
|
||||
} else {
|
||||
//code here
|
||||
}
|
||||
```
|
||||
|
||||
### Conditional Expressions
|
||||
|
||||
```kotlin
|
||||
var variable: Type = if (condition) {
|
||||
//value to be assigned here
|
||||
} else if (condition) {
|
||||
//value to be assigned here
|
||||
} else {
|
||||
//value to be assigned here
|
||||
}
|
||||
```
|
||||
|
||||
### `When` Expression
|
||||
|
||||
Each branch in a `when` expression is represented by a condition, an arrow (`->`), and a result.
|
||||
If the condition on the left-hand side of the arrow evaluates to true, then the result of the expression on the right-hand side is returned.
|
||||
Note that execution does not fall through from one branch to the next.
|
||||
|
||||
```kotlin
|
||||
when (variable){
|
||||
condition -> value
|
||||
condition -> value
|
||||
else -> value
|
||||
}
|
||||
|
||||
//Smart casting
|
||||
when (variable){
|
||||
is Type -> value
|
||||
is Type -> value
|
||||
}
|
||||
|
||||
//instead of chain of if-else
|
||||
when {
|
||||
condition -> value
|
||||
condirion -> value
|
||||
else -> value
|
||||
}
|
||||
```
|
||||
|
||||
## Loops
|
||||
|
||||
### `For` Loop
|
||||
|
||||
```kotlin
|
||||
for (item in iterable){
|
||||
//code here
|
||||
}
|
||||
|
||||
//loop in a numerical range
|
||||
for(i in start..end) {
|
||||
//code here
|
||||
}
|
||||
```
|
||||
|
||||
## Functions
|
||||
|
||||
```kotlin
|
||||
fun functionName(parameter: Type): Type {
|
||||
//code here
|
||||
|
||||
return <expression>
|
||||
}
|
||||
```
|
||||
|
||||
### Simplifying Function Declarations
|
||||
|
||||
```kotlin
|
||||
fun functionName(parameter: Type): Type {
|
||||
return if (condition) {
|
||||
//returned value
|
||||
} else {
|
||||
//returned value
|
||||
}
|
||||
}
|
||||
|
||||
fun functionName(parameter: Type): Type = if (condition) {
|
||||
//returned value
|
||||
else {
|
||||
//returned value
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Anonymous Functions
|
||||
|
||||
```kotlin
|
||||
val anonymousFunction: (Type) -> Type = { input ->
|
||||
//code acting on input here
|
||||
}
|
||||
|
||||
val variableName: Type = anonymousFunction(input)
|
||||
```
|
||||
|
||||
### Higher-order Functions
|
||||
|
||||
A function can take another function as an argument. Functions that use other functions as arguments are called *higher-order* functions.
|
||||
This pattern is useful for communicating between components in the same way that you might use a callback interface in Java.
|
||||
|
||||
```kotlin
|
||||
fun functionName(parameter: Type, function: (Type) -> Type): Type {
|
||||
//invoke function
|
||||
return function(parameter)
|
||||
}
|
||||
```
|
||||
|
||||
## Object Oriented Programming
|
||||
|
||||
### Class
|
||||
|
||||
```kotlin
|
||||
//primary constructor
|
||||
class ClassName(private var attrivute: Type) {
|
||||
|
||||
}
|
||||
|
||||
class ClassName {
|
||||
|
||||
private var var1: Type
|
||||
|
||||
//secondary constructor
|
||||
constructor(parameter: Type) {
|
||||
this.var1 = parameter
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Companion Object
|
||||
|
||||
[Companion Object Docs](https://kotlinlang.org/docs/tutorials/kotlin-for-py/objects-and-companion-objects.html)
|
||||
|
||||
```kotlin
|
||||
class ClassName {
|
||||
|
||||
// in java: static
|
||||
companion object {
|
||||
// static components of the class
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Collections
|
||||
|
||||
### ArrayList
|
||||
|
||||
```kotlin
|
||||
var array:ArrayList<Type>? = null // List init
|
||||
|
||||
array.add(item) //add item to list
|
||||
```
|
79
Markdown.md
Normal file
79
Markdown.md
Normal file
|
@ -0,0 +1,79 @@
|
|||
# Markdown Notes
|
||||
|
||||
## Headings
|
||||
|
||||
```markdown
|
||||
Heading 1
|
||||
=========
|
||||
|
||||
Heading 2
|
||||
---------
|
||||
|
||||
# Heading 1
|
||||
## Headding 2
|
||||
### Heading 3
|
||||
```
|
||||
|
||||
## Text Formatting
|
||||
|
||||
```markdown
|
||||
*Italic* _Italic_
|
||||
**Bold** __Bold__
|
||||
|
||||
~GitHub's striketrough~
|
||||
```
|
||||
|
||||
## Links & Images
|
||||
|
||||
```markdown
|
||||
[link text](http://b.org "title")
|
||||
|
||||
[link text][anchor]
|
||||
[anchor]: http://b.org "title"
|
||||
|
||||

|
||||
|
||||
![alt attribute][anchor]
|
||||
[anchor]: http://url/b.jpg "title"
|
||||
```
|
||||
|
||||
```markdown
|
||||
> Blockquote
|
||||
|
||||
* unordered list - unordered list
|
||||
* unordered list - unordered list
|
||||
* unordered list - unordered list
|
||||
|
||||
1) ordered list 1. ordered list
|
||||
2) ordered list 2. ordered list
|
||||
3) ordered list 3. ordered list
|
||||
|
||||
- [ ] empty checkbox
|
||||
- [x] checked checkbox
|
||||
```
|
||||
|
||||
### Horizontal rule
|
||||
|
||||
```markdown
|
||||
--- ***
|
||||
```
|
||||
|
||||
## Code
|
||||
|
||||
```markdown
|
||||
`inline code`
|
||||
|
||||
```lang
|
||||
multi-line
|
||||
code block
|
||||
```
|
||||
```
|
||||
|
||||
## Table
|
||||
|
||||
```markdown
|
||||
| column label | column label | column label |
|
||||
|:-------------|:------------:|--------------:|
|
||||
| left-aligned | centered | right-alinged |
|
||||
| row contents | row contents | row contents |
|
||||
```
|
32
Node.js/Electron.js/Electron.md
Normal file
32
Node.js/Electron.js/Electron.md
Normal file
|
@ -0,0 +1,32 @@
|
|||
# [Electron](https://www.electronjs.org/)
|
||||
|
||||
## Installation
|
||||
|
||||
```txt
|
||||
electron-app/
|
||||
├── package.json
|
||||
├── main.js
|
||||
└── index.html
|
||||
```
|
||||
|
||||
```ps1
|
||||
npm i -D electron@latest # electron@beta for beta version
|
||||
```
|
||||
|
||||
```json
|
||||
{
|
||||
"name": "<app_name>",
|
||||
"version": "<SemVer>",
|
||||
"description": "",
|
||||
"main": "main.js",
|
||||
"scripts": {
|
||||
"start": "electron ."
|
||||
},
|
||||
"keywords": [],
|
||||
"author": "",
|
||||
"license": "",
|
||||
"devDependencies": {
|
||||
"electron": "<electron_version>"
|
||||
}
|
||||
}
|
||||
```
|
59
Node.js/Libs/express.md
Normal file
59
Node.js/Libs/express.md
Normal file
|
@ -0,0 +1,59 @@
|
|||
# [Express](https://expressjs.com/)
|
||||
|
||||
## Installation
|
||||
|
||||
```ps1
|
||||
npm install express --save
|
||||
```
|
||||
|
||||
## [Application](https://expressjs.com/en/4x/api.html#app)
|
||||
|
||||
```js
|
||||
const express = require("express");
|
||||
|
||||
const app = express();
|
||||
const PORT = 5555;
|
||||
|
||||
// correctly serve static contents
|
||||
app.use(express.static("/route/for/static/files", "path/to/static/files/folder"));
|
||||
|
||||
// HTTP GET
|
||||
app.get("/<route>:<param>", (req, res) => {
|
||||
console.log(`${req.connection.remoteAddress} requested ${req.url}`);
|
||||
});
|
||||
|
||||
// HTTP POST
|
||||
app.post("/<route>:<param>", (req, res) => {
|
||||
console.log(`${req.connection.remoteAddress} posted to ${req.url}`);
|
||||
});
|
||||
|
||||
// responds to all HTTP verbs
|
||||
app.all("/<route>:<param>", (req, res, next) => {
|
||||
next(); // handle successive matching request (valid also on .get() & .post())
|
||||
});
|
||||
|
||||
let server = app.listen(PORT, () => {
|
||||
console.log(`Express server listening at http://localhost:${PORT}`);
|
||||
});
|
||||
|
||||
server.on("error", () => {
|
||||
server.close();
|
||||
});
|
||||
```
|
||||
|
||||
## [Response](https://expressjs.com/en/4x/api.html#res)
|
||||
|
||||
```js
|
||||
Response.send([body]); // Sends the HTTP response.
|
||||
Response.sendFile(path); // Transfers the file at the given path.
|
||||
Response.json(body); // Sends a JSON response.
|
||||
Response.redirect([status,] path); // Redirects to the URL derived from the specified path, with specified status
|
||||
Response.end(); // Ends the response process
|
||||
```
|
||||
|
||||
## [Request](https://expressjs.com/en/4x/api.html#req)
|
||||
|
||||
```js
|
||||
Request.params.<param> // query params obj (GET)
|
||||
Request.body.<param> // body params (POST)
|
||||
```
|
68
Node.js/Node.js.md
Normal file
68
Node.js/Node.js.md
Normal file
|
@ -0,0 +1,68 @@
|
|||
# Node.js
|
||||
|
||||
Asyncronous JavaScript Engine
|
||||
|
||||
## Syllabus
|
||||
|
||||
Moduli:
|
||||
|
||||
- nvm, npm cli
|
||||
- tutti moduli standard
|
||||
- alcuni da npm (express?, cors?, nodemon?)
|
||||
|
||||
## NVM
|
||||
|
||||
### Windows
|
||||
|
||||
```ps1
|
||||
nvm list # show installed node versions
|
||||
nvm list available # list installable versions of node
|
||||
|
||||
nvm install <version> # install a version of node
|
||||
nvm install latest # install the latest version of node
|
||||
|
||||
nvm use <version> # set <version> as default one
|
||||
|
||||
nvm uninstall <version> # uninstall a version of node
|
||||
```
|
||||
|
||||
### Linux
|
||||
|
||||
```bash
|
||||
nvm ls # show installed node versions
|
||||
nvm ls-remote # list installable versions of node
|
||||
|
||||
nvm install <version> # install a version of node
|
||||
nvm install node # install the latest version of node
|
||||
nvm install --lts # install the latest LTS version of node
|
||||
|
||||
nvm use <version> # set <version> as default one
|
||||
|
||||
nvm uninstall <version> # uninstall a version of node
|
||||
```
|
||||
|
||||
## NPM
|
||||
|
||||
```ps1
|
||||
npm init # init a project
|
||||
npm install <module> # install a module as global
|
||||
npm install <module> -P|--save-prod # install a module as local (aka --save)
|
||||
npm install <module> -D|--save-dev # install a module as local dev dependency
|
||||
```
|
||||
|
||||
## Imports
|
||||
|
||||
```js
|
||||
const pkg = require("module"); // load the file as JS object with an alias
|
||||
const { component } = require("module"); // load only a component of the module (can lead to name collision)
|
||||
const alias = require("module").component // set alias for component
|
||||
```
|
||||
|
||||
## Exports
|
||||
|
||||
```js
|
||||
// definitions
|
||||
|
||||
module.exports = <variable/method/class/expression>; // dafoult export
|
||||
module.exports.exported_name = <variable/method/class/expression>;
|
||||
```
|
22
Node.js/Standard Packages/child_process.md
Normal file
22
Node.js/Standard Packages/child_process.md
Normal file
|
@ -0,0 +1,22 @@
|
|||
# Child Process Module
|
||||
|
||||
## Spawning
|
||||
|
||||
```js
|
||||
child_process.spawn(command, args, options); // spawn a child process
|
||||
|
||||
// On Windows, setting options.detached to true makes it possible for the child process to continue running after the parent exits
|
||||
child_process.spawn(command, args, {
|
||||
detached: true
|
||||
});
|
||||
```
|
||||
|
||||
## Using The System Shell
|
||||
|
||||
```js
|
||||
exec("command args", {'shell':'powershell.exe'}, (err, stdout, stderr) => {
|
||||
|
||||
});
|
||||
|
||||
execSync("command args", {'shell':'powershell.exe'});
|
||||
```
|
50
Node.js/Standard Packages/cluster.md
Normal file
50
Node.js/Standard Packages/cluster.md
Normal file
|
@ -0,0 +1,50 @@
|
|||
# Cluster Module
|
||||
|
||||
A single instance of Node.js runs in a single thread. To take advantage of multi-core systems, the user will sometimes want to launch a **cluster of Node.js processes** to handle the load.
|
||||
|
||||
The cluster module allows easy creation of child processes that all share server ports.
|
||||
|
||||
```js
|
||||
const cluster = require('cluster');
|
||||
const http = require('http');
|
||||
const numCPUs = require('os').cpus().length;
|
||||
|
||||
if (cluster.isMaster) {
|
||||
console.log(`Master ${process.pid} is running`);
|
||||
|
||||
// Fork workers. (Spawn a new worker process)
|
||||
for (let i = 0; i < numCPUs; i++) {
|
||||
cluster.fork();
|
||||
}
|
||||
|
||||
cluster.on('exit', (worker, code, signal) => {
|
||||
console.log(`worker ${worker.process.pid} died`);
|
||||
cluster.fork();
|
||||
});
|
||||
|
||||
} else {
|
||||
// Workers can share any TCP connection
|
||||
// In this case it is an HTTP server
|
||||
http.createServer((req, res) => {
|
||||
res.writeHead(200);
|
||||
res.end('hello world\n');
|
||||
}).listen(8000);
|
||||
|
||||
console.log(`Worker ${process.pid} started`);
|
||||
}
|
||||
```
|
||||
|
||||
## Cluster VS Worker Threads
|
||||
|
||||
Cluster (multi-processing):
|
||||
|
||||
- One process is launched on each CPU and can communicate via IPC.
|
||||
- Each process has it's own memory with it's own Node (v8) instance. Creating tons of them may create memory issues.
|
||||
- Great for spawning many HTTP servers that share the same port b/c the master main process will multiplex the requests to the child processes.
|
||||
|
||||
Worker Threads (multi-threading):
|
||||
|
||||
- One process total
|
||||
- Creates multiple threads with each thread having one Node instance (one event loop, one JS engine). Most Node API's are available to each thread except a few. So essentially Node is embedding itself and creating a new thread.
|
||||
- Shares memory with other threads (e.g. SharedArrayBuffer)
|
||||
- Great for CPU intensive tasks like processing data or accessing the file system. Because NodeJS is single threaded, synchronous tasks can be made more efficient with workers
|
34
Node.js/Standard Packages/dgram.md
Normal file
34
Node.js/Standard Packages/dgram.md
Normal file
|
@ -0,0 +1,34 @@
|
|||
# UDP Module
|
||||
|
||||
```js
|
||||
const socket = dgram.createSocket("udp4"); // connect to network interface
|
||||
|
||||
socket.on("error", (err) => { /* handle error */ });
|
||||
socket.on("message", (msg, rinfo) => {});
|
||||
socket.on("end", () => {});
|
||||
|
||||
socket.bind(port); // listen to port
|
||||
socket.on('listening', () => {});
|
||||
|
||||
socket.send(message, port, host, (err) => { /* handle error */ });
|
||||
```
|
||||
|
||||
## Multicasting
|
||||
|
||||
```js
|
||||
const socket = dgram.createSocket({ type: "udp4", reuseAddr: true });
|
||||
// When reuseAddr is true socket.bind() will reuse the address, even if another process has already bound a socket on it.
|
||||
|
||||
const multicastPort = 5555;
|
||||
const multicastAddress = "239.255.255.255"; // whatever ip
|
||||
|
||||
socket.bind(multicastPort);
|
||||
|
||||
socket.on("listening", () => {
|
||||
socket.addMembership(multicastAddress);
|
||||
})
|
||||
|
||||
socket.on("message", (msg, rinfo) => {
|
||||
console.log(`Got: "${msg.toString()}" from ${rinfo.address}`);
|
||||
});
|
||||
```
|
35
Node.js/Standard Packages/events.md
Normal file
35
Node.js/Standard Packages/events.md
Normal file
|
@ -0,0 +1,35 @@
|
|||
# Events Module
|
||||
|
||||
Much of the Node.js core API is built around an idiomatic *asynchronous event-driven architecture* in which certain kinds of objects (**emitters**) emit *named events* that cause `Function` objects (**listeners**) to be called.
|
||||
|
||||
All objects that emit events are instances of the `EventEmitter` class. These objects expose an `eventEmitter.on()` function that allows one or more functions to be attached to named events emitted by the object. Typically, event names are camel-cased strings but any valid JavaScript property key can be used.
|
||||
|
||||
When the EventEmitter object emits an event, all of the functions attached to that specific event are called *synchronously*. Any values returned by the called listeners are *ignored and discarded*.
|
||||
|
||||
```js
|
||||
const EventEmitter = require("events");
|
||||
|
||||
class CustomEmitter extends EventEmitter {} ;
|
||||
|
||||
const customEmitterObj = new CustomEmitter();
|
||||
|
||||
// add event listener
|
||||
cusomEmitterObj.on("event", (e) => {
|
||||
// e contains event object
|
||||
})
|
||||
|
||||
// single-use event listener (execute and remove listener)
|
||||
cusomEmitterObj.once("event", (e) => {
|
||||
// e contains event object
|
||||
})
|
||||
|
||||
customEmitterObj.removeListener("event", callback);
|
||||
customEmitterObj.removeAllListeners("event");
|
||||
|
||||
customEmitterObj.emit("event");
|
||||
customEmitterObj.emit("event", { /* event object */ });
|
||||
|
||||
customEmitterObj.eventNames(); // string[] of the events it listens to
|
||||
customEmitterObj.listenerCount("event"); // num of listeners for an event
|
||||
customEmitterObj.listeners(); // Function[], handlers of the events
|
||||
```
|
102
Node.js/Standard Packages/fs.md
Normal file
102
Node.js/Standard Packages/fs.md
Normal file
|
@ -0,0 +1,102 @@
|
|||
# fs (FIle System) Module
|
||||
|
||||
Async versions can acces file at the same time which can lead to conflicts in operations, erros can be handled in callback.
|
||||
Sync versions cannot interfere with each other, errors cause exceptions, handle in try-catch.
|
||||
|
||||
## Files
|
||||
|
||||
### Creating & Deleting Files
|
||||
|
||||
```js
|
||||
fs.writeFile(file, data, encoding="utf8", mode=0o666, (err) => {}); // create a file (async)
|
||||
fs.writeFileSync(file, data, encoding="utf8", mode=0o666); // create a file (sync)
|
||||
|
||||
// remove file
|
||||
fs.unlink(path, callback); // delete a file (async)
|
||||
fs.unlinkSync(path); // delete a file (sync)
|
||||
|
||||
// remove file or directory
|
||||
fs.rm(path, force=false, recursive=false, (err) => {});
|
||||
fs.rmSync(path, force=false, recursive=false);
|
||||
|
||||
// rename a file, if oldPath is a directory an error will be rised
|
||||
fs.rename(oldPath, newPath, (err) => {});
|
||||
fs.renameSync(oldPath, newPath);
|
||||
```
|
||||
|
||||
### Writing & Reading a File
|
||||
|
||||
```js
|
||||
// append contents to a file
|
||||
fs.appendFile(file, data, encoding="utf8", mode=0o666, (err) => {});
|
||||
fs.appendFileSync(file, data, encoding="utf8", mode=0o666);
|
||||
|
||||
// write contents into a file
|
||||
fs.write(fd, string, position, encoding="utf8", (err) => {});
|
||||
fs.writeSync(fd, string, position, encoding="utf8"); // returns num of bytes written
|
||||
|
||||
// read file contents
|
||||
fs.readFile(path, (err, data) => {});
|
||||
fs.readFileSync(path); // returns contents of the file
|
||||
```
|
||||
|
||||
### Managing Links
|
||||
|
||||
```js
|
||||
// make a new name for a file
|
||||
fs.link(existingPath, newPath, (err) => {});
|
||||
fs.linkSync(existingPath, newPath);
|
||||
|
||||
// make a new name for a file (symlink)
|
||||
fs.symlink(target, path, (err) => {});
|
||||
fs.symlink(target, path);
|
||||
```
|
||||
|
||||
### Managing Permissions
|
||||
|
||||
## Directories & `fs.Dir`
|
||||
|
||||
### Creating & Deleting Directories
|
||||
|
||||
```js
|
||||
// create a directory
|
||||
fs.mkdir(path, mode=0o777, (err) => {});
|
||||
fs.mkdirSync(path, mode=0o777);
|
||||
|
||||
// remove a directory
|
||||
fs.rmdir(path, recursive=false, (err) => {});
|
||||
fs.rmdirSync(path, recursive=false;
|
||||
```
|
||||
|
||||
### Reading Directory Contents
|
||||
|
||||
```js
|
||||
// read directrory contents
|
||||
fs.readdir(path, (err, files) => {}); // files is string[]
|
||||
fs.readdir(path, { withFileTypes: true }, (err, files) => {}); // files is Dirent[]
|
||||
fs.readdirSync(path); // returns string[] of files/directories
|
||||
fs.readdirSync(path, { withFileTypes: true }); // returns Dirent[] of files/directories
|
||||
```
|
||||
|
||||
### `fs.Dir`
|
||||
|
||||
```js
|
||||
// construct an fs.Dir object from an existing path
|
||||
fs.opendir(path, (err, dir) => {});
|
||||
fs.opendirSync(path); // return a fs.Dir object
|
||||
```
|
||||
|
||||
## fs.Stats
|
||||
|
||||
### Obtaining
|
||||
|
||||
```js
|
||||
fs.stat(path, {bigint: true}, (err, stats) => {});
|
||||
fs.statSync(path, {bigint: true}); // returns fs.Stats
|
||||
|
||||
fs.lstat(path, {bigint: true}, (err, stats) => {});
|
||||
fs.lstatSync(path, {bigint: true}); // returns fs.Stats
|
||||
|
||||
fs.fstat(path, {bigint: true}, (err, stats) => {});
|
||||
fs.fstatSync(path, {bigint: true}); // returns fs.Stats
|
||||
```
|
80
Node.js/Standard Packages/http(s).md
Normal file
80
Node.js/Standard Packages/http(s).md
Normal file
|
@ -0,0 +1,80 @@
|
|||
# HTTP(S) Module
|
||||
|
||||
## HTTTP(S) IncomingMessage (request)
|
||||
|
||||
### Making a request
|
||||
|
||||
```js
|
||||
https.request(
|
||||
{
|
||||
host: "www.website.com",
|
||||
method: "GET", // POST, ...
|
||||
path: "/page/?param=value"
|
||||
},
|
||||
(response) => { // respnse is IncomingMessage
|
||||
// do stuff
|
||||
}
|
||||
).end();
|
||||
```
|
||||
|
||||
### Reqest Methods & Properties
|
||||
|
||||
```js
|
||||
IncomingMessage.headers
|
||||
IncomingMessage.statusCode
|
||||
IncomingMessage.statusMessage
|
||||
IncomingMessage.url
|
||||
```
|
||||
|
||||
## HTTTP(S) ServerResponse (response)
|
||||
|
||||
### Response Methods & Properties
|
||||
|
||||
```js
|
||||
ServerResponse.writeHead(statusCode[, statusMessage][, headers]);
|
||||
```
|
||||
|
||||
## HTTP(S) Server
|
||||
|
||||
### Creating a server
|
||||
|
||||
```js
|
||||
const PORT = 8123;
|
||||
|
||||
// req is IncomingMessage
|
||||
// res is ServerResponse
|
||||
const server = http.createServer((req, res) => {
|
||||
|
||||
let body = "<html></html>"
|
||||
|
||||
res.writeHead(200, {
|
||||
"Content-Length": Buffer.byteLength(body),
|
||||
"Content-Type": "text/html; charset=utf8"
|
||||
});
|
||||
|
||||
res.end(body);
|
||||
|
||||
});
|
||||
|
||||
server.listen(PORT);
|
||||
```
|
||||
|
||||
### Reading a request search params
|
||||
|
||||
```js
|
||||
const http = require("http");
|
||||
const url = require("url");
|
||||
|
||||
const PORT = 8123;
|
||||
|
||||
const server = http.createServer((req, res) => {
|
||||
let url = new URL(req.url, "http://" + req.headers.host);
|
||||
let params = url.searchParams;
|
||||
|
||||
// ...
|
||||
|
||||
});
|
||||
|
||||
console.log("Listening on port: " + PORT);
|
||||
server.listen(PORT);
|
||||
```
|
26
Node.js/Standard Packages/net.md
Normal file
26
Node.js/Standard Packages/net.md
Normal file
|
@ -0,0 +1,26 @@
|
|||
# Net Module (Connection Oriented Networking - TCP/IP)
|
||||
|
||||
## Server (`net.Server`)
|
||||
|
||||
```js
|
||||
const net = require('net');
|
||||
|
||||
const server = net.createServer((socket) => { /* connect listener */ });
|
||||
|
||||
server.on('error', (err) => {
|
||||
// handle error
|
||||
});
|
||||
|
||||
server.listen(8124, () => { }); // listen for connection
|
||||
```
|
||||
|
||||
## Sockets (`net.Socket`)
|
||||
|
||||
```js
|
||||
|
||||
const client = net.createConnection({ host: "127.0.0.1", port: 8124 }, () => { /* 'connect' listener. */ });
|
||||
|
||||
socket.on("data", (buffer) => { /* operate on input buffer */ });
|
||||
socket.on("error", (err) => { /* handle error */ });
|
||||
socket.on("end", () => {}); // client disconnection
|
||||
```
|
20
Node.js/Standard Packages/os.md
Normal file
20
Node.js/Standard Packages/os.md
Normal file
|
@ -0,0 +1,20 @@
|
|||
# OS Module
|
||||
|
||||
## Information
|
||||
|
||||
```js
|
||||
os.arch(); // CPU Architecture
|
||||
os.platform(); // OS platform (aix, darwin, freebsd, linux, openbsd, sunos, win32)
|
||||
os.type(); // OS name (Linux, Windows_NT, )
|
||||
|
||||
os.release(); // OS release number
|
||||
os.version(); // OS kernel version
|
||||
|
||||
os.tmpdir(); // OS Temp directory
|
||||
os.homedir(); // CUrrent user home directory
|
||||
|
||||
os.hostname(); // PC Name
|
||||
|
||||
os.cpus(); // Array of CPU info
|
||||
os.networkInterfaces(); // Array of Network Interfacecs info
|
||||
```
|
5
Node.js/Standard Packages/path.md
Normal file
5
Node.js/Standard Packages/path.md
Normal file
|
@ -0,0 +1,5 @@
|
|||
# Path Module
|
||||
|
||||
```js
|
||||
path.join("folder1", "folder2", "filename"); // WIN: folder1\folder2\filename - LINUX: folder1/folder2/filename
|
||||
```
|
27
Node.js/Standard Packages/process.md
Normal file
27
Node.js/Standard Packages/process.md
Normal file
|
@ -0,0 +1,27 @@
|
|||
# Process Module
|
||||
|
||||
Provides information about, and control over, the current Node.js process
|
||||
|
||||
## Properties
|
||||
|
||||
```js
|
||||
process.argv // string[] containing the command-line arguments passed when the Node.js process was launched
|
||||
process.pid // PID of the process
|
||||
process.env // list of ENV Variables
|
||||
process.platform // identifier of the OS
|
||||
process.arch // processor architecture
|
||||
```
|
||||
|
||||
## Functions
|
||||
|
||||
```js
|
||||
process.resourecUsage(); // resource usage for the current process
|
||||
process.memoryUsage(); // memory usage of the Node.js process measured in bytes
|
||||
process.exit(code); // terminate the process synchronously with an exit status of code
|
||||
```
|
||||
|
||||
## Events
|
||||
|
||||
```js
|
||||
process.on("event", (code) => { });
|
||||
```
|
16
Node.js/Standard Packages/url.md
Normal file
16
Node.js/Standard Packages/url.md
Normal file
|
@ -0,0 +1,16 @@
|
|||
# Url Module
|
||||
|
||||
`http://user:pass@sub.example.com:8080/p/a/t/h?query=string#hash`
|
||||
|
||||

|
||||
|
||||
## Basics
|
||||
|
||||
```js
|
||||
|
||||
const url = new URL('/foo', 'https://example.org/');
|
||||
|
||||
URL.searchParams
|
||||
URL.searchParams.get("queryparam");
|
||||
URL.searchParams.has("queryparam");
|
||||
```
|
38
Node.js/Standard Packages/worker_threads.md
Normal file
38
Node.js/Standard Packages/worker_threads.md
Normal file
|
@ -0,0 +1,38 @@
|
|||
# Worker Threads Module
|
||||
|
||||
The `worker_threads` module enables the use of threads that execute JavaScript in parallel.
|
||||
|
||||
Workers (threads) are useful for performing CPU-intensive JavaScript operations. They do not help much with I/O-intensive work. The Node.js built-in asynchronous I/O operations are more efficient than Workers can be.
|
||||
|
||||
Unlike `child_process` or `cluster`, `worker_threads` can *share* memory. They do so by transferring `ArrayBuffer` instances or sharing `SharedArrayBuffer` instances.
|
||||
|
||||
```js
|
||||
const { Worker, isMainThread } = require("worker_threads");
|
||||
|
||||
if(isMainThread){
|
||||
console.log("Main Thread");
|
||||
|
||||
// start new inner thread executing this file
|
||||
new Worker(__filename);
|
||||
}
|
||||
else
|
||||
{
|
||||
// executed by inner threads
|
||||
console.log("Inner Thread Starting");
|
||||
}
|
||||
```
|
||||
|
||||
## Cluster VS Worker Threads
|
||||
|
||||
Cluster (**multi-processing**):
|
||||
|
||||
- One process is launched on each CPU and can communicate via IPC.
|
||||
- Each process has it's own memory with it's own Node (v8) instance. Creating tons of them may create memory issues.
|
||||
- Great for spawning many HTTP servers that share the same port b/c the master main process will multiplex the requests to the child processes.
|
||||
|
||||
Worker Threads (**multi-threading**):
|
||||
|
||||
- One process total
|
||||
- Creates multiple threads with each thread having one Node instance (one event loop, one JS engine). Most Node API's are available to each thread except a few. So essentially Node is embedding itself and creating a new thread.
|
||||
- Shares memory with other threads (e.g. SharedArrayBuffer)
|
||||
- Great for CPU intensive tasks like processing data or accessing the file system. Because NodeJS is single threaded, synchronous tasks can be made more efficient with workerss
|
30351
Notes For Professionals/BashNotesForProfessionals.pdf
Normal file
30351
Notes For Professionals/BashNotesForProfessionals.pdf
Normal file
File diff suppressed because it is too large
Load diff
107538
Notes For Professionals/CSharpNotesForProfessionals.pdf
Normal file
107538
Notes For Professionals/CSharpNotesForProfessionals.pdf
Normal file
File diff suppressed because it is too large
Load diff
BIN
Notes For Professionals/HTML5NotesForProfessionals.pdf
Normal file
BIN
Notes For Professionals/HTML5NotesForProfessionals.pdf
Normal file
Binary file not shown.
148259
Notes For Professionals/JavaNotesForProfessionals.pdf
Normal file
148259
Notes For Professionals/JavaNotesForProfessionals.pdf
Normal file
File diff suppressed because it is too large
Load diff
BIN
Notes For Professionals/KotlinNotesForProfessionals.pdf
Normal file
BIN
Notes For Professionals/KotlinNotesForProfessionals.pdf
Normal file
Binary file not shown.
BIN
Notes For Professionals/PowerShellNotesForProfessionals.pdf
Normal file
BIN
Notes For Professionals/PowerShellNotesForProfessionals.pdf
Normal file
Binary file not shown.
BIN
Notes For Professionals/PythonNotesForProfessionals.pdf
Normal file
BIN
Notes For Professionals/PythonNotesForProfessionals.pdf
Normal file
Binary file not shown.
BIN
Notes For Professionals/SQLNotesForProfessionals.pdf
Normal file
BIN
Notes For Professionals/SQLNotesForProfessionals.pdf
Normal file
Binary file not shown.
109
PHP/PHP Composer & Autoloading.md
Normal file
109
PHP/PHP Composer & Autoloading.md
Normal file
|
@ -0,0 +1,109 @@
|
|||
# Composer & Autoloading
|
||||
|
||||
## Autoloading
|
||||
|
||||
The function [spl_autoload_register()](https://www.php.net/manual/en/function.spl-autoload-register.php) allows to register a function that will be invoked when PHP needs to load a class/interface defined by the user.
|
||||
|
||||
In `autoload.php`:
|
||||
|
||||
```php
|
||||
# custom function
|
||||
function autoloader($class) {
|
||||
|
||||
|
||||
# __DIR__ -> path of calling file
|
||||
# $class -> className (hopefully same as file)
|
||||
# if class is in namesapce $class -> Namespace\className (hopefully folders mirror Namespace)
|
||||
|
||||
$class = str_replace("\\", DIRECTORY_SEPARATOR, $class); # avoid linux path separator issues
|
||||
|
||||
$fileName = sprintf("%s\\path\\%s.php", __DIR__, $class);
|
||||
# or
|
||||
$filename = sprintf("%s\\%s.php", __DIR__, $class); # if class is in namespaace
|
||||
|
||||
if (file_exists($fileName)) {
|
||||
include $fileName;
|
||||
}
|
||||
}
|
||||
|
||||
spl_autoload_register('autoloader'); // register function
|
||||
```
|
||||
|
||||
In `file.php`:
|
||||
|
||||
```php
|
||||
require "autoload.php";
|
||||
|
||||
# other code
|
||||
```
|
||||
|
||||
**NOTE**: will fuck up if namespaces exists.
|
||||
|
||||
### Multiple Autoloading
|
||||
|
||||
It's possible to resister multiple autoloading functions by calling `spl_autoloaad_register()` multiple times.
|
||||
|
||||
```php
|
||||
# prepend adds the function at the start of the queue
|
||||
# throws selects if errors in loading throw exceptions
|
||||
spl_autoload_register(callable $func, $throw=TRUE, $prepend=FALSE);
|
||||
|
||||
spl_autoload_functions() # return a list of registered functions.
|
||||
```
|
||||
|
||||
## [Composer](https://getcomposer.org/)
|
||||
|
||||
Open Source project for dependency management and autoloading of PHP libraries and classes.
|
||||
|
||||
Composer uses `composer.json` to define dependencies with third-party libraries.
|
||||
Libraries are downloaded through [Packagist](https://packagist.org/) and [GitHub](https://github.com/).
|
||||
|
||||
In `composer.json`:
|
||||
|
||||
```json
|
||||
{
|
||||
"require": {
|
||||
"php": ">=7.0",
|
||||
"monolog/monolog": "1.0.*"
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Installing Dependencies
|
||||
|
||||
In the same folder of `composer.json` execute `composer install`.
|
||||
|
||||
Composer will create:
|
||||
|
||||
- `vendor`: folder containing all requested libraries
|
||||
- `vendor\autoload.php`: file for class autoloading
|
||||
- `composer.lock`
|
||||
|
||||
In alternative `composer require <lib>` will add the library to the project and create a `composer.json` if missing.
|
||||
|
||||
**NOTE**: to ignore the php version use `composer <command> --ignore-platform-reqs`
|
||||
|
||||
### Updating Dependencies
|
||||
|
||||
To update dependencies use `composer update`. To update only the autoloading section use `composer dump-autoload`.
|
||||
|
||||
### [Autoloading Project Classes](https://getcomposer.org/doc/04-schema.md#autoload)
|
||||
|
||||
[PSR-4 Spec](https://www.php-fig.org/psr/psr-4/)
|
||||
|
||||
Composer can also autoload classes belonging to the current project. It's sufficient to add the `autoload` keyword in the JSON and specify the path and autoload mode.
|
||||
|
||||
```json
|
||||
{
|
||||
"autoload": {
|
||||
"psr-4": {
|
||||
"RootNamespace": "src/",
|
||||
"Namespace\\": "src/Namespace/",
|
||||
},
|
||||
"file": [
|
||||
"path/to/file.php",
|
||||
...
|
||||
]
|
||||
}
|
||||
}
|
||||
```
|
102
PHP/PHP DB.md
Normal file
102
PHP/PHP DB.md
Normal file
|
@ -0,0 +1,102 @@
|
|||
# Databases in PHP
|
||||
|
||||
## PHP Data Objects ([PDO][pdo])
|
||||
|
||||
[pdo]: https://www.php.net/manual/en/book.pdo.php
|
||||
|
||||
PDO is the PHP extension for database access through a single API. It supports various databases: MySQL, SQLite, PostgreSQL, Oracle, SQL Server, etc.
|
||||
|
||||
### Database Connection
|
||||
|
||||
```php
|
||||
$dsn = "mysql:dbname=<dbname>;host=<ip>";
|
||||
$user="<db_user>";
|
||||
$password="<db_password>";
|
||||
|
||||
try {
|
||||
$pdo = new PDO($dsn, $user, $password); # connect, can throw PDOException
|
||||
} catch (PDOException $e) {
|
||||
printf("Connection failed: %s\n", $e->getMessage()); # notify error
|
||||
exit(1);
|
||||
}
|
||||
```
|
||||
|
||||
### Queries
|
||||
|
||||
To execute a query it's necessaty to "prepare it" with *parameters*.
|
||||
|
||||
```php
|
||||
# literal string with markers
|
||||
$sql = 'SELECT fields
|
||||
FROM tables
|
||||
WHERE field <operator> :marker'
|
||||
|
||||
$stmt = $pdo->prepare($sql, $options_array); # returns PDOStatement, used to execute the query
|
||||
$stmt->execute([ ':marker' => value ]); # substitute marker with actual value
|
||||
|
||||
# fetchAll returns all mathces
|
||||
$result = $stmt->fetchAll(); # result as associtive array AND numeric array (PDO::FETCH_BOTH)
|
||||
$result = $stmt->fetchAll(PDO::FETCH_ASSOC); # result as associtive array
|
||||
$result = $stmt->fetchAll(PDO::FETCH_NUM); # result as array
|
||||
$result = $stmt->fetchAll(PDO::FETCH_OBJ); # result as object of stdClass
|
||||
$result = $stmt->fetchAll(PDO::FETCH_CLASS, ClassName::class); # result as object of a specific class
|
||||
```
|
||||
|
||||
### Parameter Binding
|
||||
|
||||
```php
|
||||
# bindValue
|
||||
$stmt = pdo->prepare(sql);
|
||||
$stmt->bindValue(':marker', value, PDO::PARAM_<type>);
|
||||
$stmt->execute();
|
||||
|
||||
# bindParam
|
||||
$stmt = pdo->prepare(sql);
|
||||
$variable = value;
|
||||
$stmt->bindParam(':marker', $variable); # type optional
|
||||
$stmt->execute();
|
||||
```
|
||||
|
||||
### PDO & Data Types
|
||||
|
||||
By default PDO converts all results into strings since it is a generic driver for multiple databases.
|
||||
Its possible to disable this behaviour setting `PDO::ATTR_STRINGIFY_FETCHES` and `PDO::ATTR_EMULATE_PREPARES` as `false`.
|
||||
|
||||
**NOTE**: `FETCH_OBJ` abd `FETCH_CLASS` return classes and don't have this bheaviour.
|
||||
|
||||
```php
|
||||
pdo->setAttribute()
|
||||
|
||||
$pdo->setAttribute(PDO::ATTR_STRINGIFY_FETCHES, false);
|
||||
$pdo->setAttribute(PDO::ATTR_EMULATE_PREPARES, false);
|
||||
|
||||
$stmt = $pdo->prepare($sql);
|
||||
$stmt->execute([':marker' => value]);
|
||||
$result = $stmt->fetchAll(PDO::FETCH_ASSOC);
|
||||
```
|
||||
|
||||
### PDO Debug
|
||||
|
||||
```php
|
||||
$stmt = $pdo->prepare($sql);
|
||||
$stmt->execute([':marker' => value]);
|
||||
$result = $stmt->fetchAll(PDO::FETCH_ASSOC);
|
||||
|
||||
$stmt->debugDumpParams(); # print the SQK query that has been sent to the database
|
||||
```
|
||||
|
||||
## [SQLite3](https://www.php.net/manual/en/book.sqlite3.php)
|
||||
|
||||
```php
|
||||
$db = SQLite3("db_file.sqlite3"); // connection
|
||||
|
||||
$stmt = $db->prepare("SELECT fields FROM tables WHERE field <operator> :marker"); // preapre query
|
||||
$stmt->bindParam(":marker", $variable); // param binding
|
||||
|
||||
$result = $stmt->execute(); // retireve records
|
||||
$result->finalize(); // close result set, recommended calling before another execute()
|
||||
|
||||
$records = $results->fetchArray(SQLITE3_ASSOC); // extract records as array (flase if no results)
|
||||
```
|
||||
|
||||
**NOTE**: Result set objects retrieved by calling `SQLite3Stmt::execute()` on the same statement object are not independent, but rather share the same underlying structure. Therefore it is recommended to call `SQLite3Result::finalize()`, before calling `SQLite3Stmt::execute()` on the same statement object again.
|
81
PHP/PHP Dependecy Injection.md
Normal file
81
PHP/PHP Dependecy Injection.md
Normal file
|
@ -0,0 +1,81 @@
|
|||
# Dependency Injection (PHP-DI), KISS, SOLID
|
||||
|
||||
## Dependency Injection
|
||||
|
||||
Explicit definition of a class dependencies with the injection through the constructor or *getters*/*setters*.
|
||||
|
||||
```php
|
||||
class Foo
|
||||
{
|
||||
public function __construct(PDO $pdo) // depends on PDO
|
||||
{
|
||||
$this->pdo = $pdo;
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Dependency Injection Container
|
||||
|
||||
The **Dependecy Injection Container** (DIC) allow to archive all the dependencies in a single `Container` class. Some offer automatic resolution of the dependecies.
|
||||
Containers aid the developer in the handling of the dependecies: get/has/set.
|
||||
|
||||
## [PHP-DI](https://php-di.org/)
|
||||
|
||||
The dependency injection container for humans. Installation: `composer require php-di/php-di`
|
||||
|
||||
- **Autowire** functionality: the ability of the container to create and inject the dependecy automatically.
|
||||
- Use of [Reflection](https://www.php.net/manual/en/intro.reflection.php)
|
||||
- Configuration of the container through annotations & PHP code.
|
||||
|
||||
```php
|
||||
class Foo
|
||||
{
|
||||
private $bar;
|
||||
public function __construct(Bar $bar) // depends on Bar
|
||||
{
|
||||
$this->bar = $bar;
|
||||
}
|
||||
}
|
||||
|
||||
class Bar{}
|
||||
|
||||
$container = new DI\Container(); // DI Container
|
||||
$foo = $container->get('Foo'); // get instance of Foo (automatic DI of Bar)
|
||||
```
|
||||
|
||||
## DIC Configuration
|
||||
|
||||
```php
|
||||
// Foo.php
|
||||
class Foo
|
||||
{
|
||||
public function __construct(PDO $pdo) // depends on PDO
|
||||
{
|
||||
$this->pdo = $pdo;
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
```php
|
||||
// config.php
|
||||
use Psr\Container\ContainerInterface;
|
||||
|
||||
// config "primitrive" dependencies (dependecy => construct & return)
|
||||
return [
|
||||
'dsn' => 'sqlite:db.sq3',
|
||||
PDO::class => function(ContainerInterface $c) {
|
||||
return new PDO($c->get('dsn'));
|
||||
},
|
||||
|
||||
...
|
||||
];
|
||||
```
|
||||
|
||||
```php
|
||||
$builder = new \DI\ContainerBuilder();
|
||||
$builder->addDefinitions("config.php"); // load config
|
||||
$container = $builder->build(); // construct container
|
||||
$cart = $container->get(Foo::class); // Instantiate & Inject
|
||||
```
|
||||
|
||||
**NOTE**: `get("className")` requires the explicit definition of `className` in the config file. `get(ClassName::class)` does not.
|
34
PHP/PHP PSR-7.md
Normal file
34
PHP/PHP PSR-7.md
Normal file
|
@ -0,0 +1,34 @@
|
|||
# PSR-7
|
||||
|
||||
## [PSR-7](https://www.php-fig.org/psr/psr-7/)
|
||||
|
||||
Standard of the PHP Framework Interop Group that defines common interafces for handling HTTP messages.
|
||||
|
||||
- `Psr\Http\Message\MessageInterface`
|
||||
- `Psr\Http\Message\RequestInterface`
|
||||
- `Psr\Http\Message\ResponseInterface`
|
||||
- `Psr\Http\Message\ServerRequestInterface`
|
||||
- `Psr\Http\Message\StreamInterface`
|
||||
- `Psr\Http\Message\UploadedFileInterface`
|
||||
- `Psr\Http\Message\UriInterface`
|
||||
|
||||
Example:
|
||||
|
||||
```php
|
||||
// empty array if not found
|
||||
$header = $request->getHeader('Accept');
|
||||
|
||||
// empty string if not found
|
||||
$header = $request->getHeaderLine('Accept');
|
||||
|
||||
// check the presence of a header
|
||||
if (! $request->hasHeader('Accept')) {}
|
||||
|
||||
// returns the parameters in a query string
|
||||
$query = $request->getQueryParams();
|
||||
```
|
||||
|
||||
### Immutability
|
||||
|
||||
PSR-7 requests are *immutable* objects; a change in the data will return a new instance of the object.
|
||||
The stream objects of PSR-7 are *not immutable*.
|
146
PHP/PHP Templates with Plates.md
Normal file
146
PHP/PHP Templates with Plates.md
Normal file
|
@ -0,0 +1,146 @@
|
|||
# Templates with Plates
|
||||
|
||||
## Template
|
||||
|
||||
To separate HTML code and PHP code it's possible to use **templates** with markers for variable substitution.
|
||||
Variables are created in the PHP code and are passed to the template in the **rendering** phase.
|
||||
The server transmitts only the `index.php` file to the user. The php file renders the templates as needed.
|
||||
|
||||
```html
|
||||
<html>
|
||||
<head>
|
||||
<title><?= $this->e($title)?></title>
|
||||
</head>
|
||||
|
||||
<body>
|
||||
<?= $this->section('content')?>
|
||||
</body>
|
||||
</html>
|
||||
```
|
||||
|
||||
## [Plates](https://platesphp.com/)
|
||||
|
||||
Plates is a templete engine for PHP. A templete engine permits to separate the PHP code (businnes logic) from the HTML pages.
|
||||
|
||||
Installation through composer: `composer require league/plates`.
|
||||
|
||||
```php
|
||||
# index.php
|
||||
require "vendor/autoload.php";
|
||||
|
||||
use League\Plates\Engine;
|
||||
|
||||
$templates = new Engine("path\\to\\templates");
|
||||
|
||||
echo $templates->render("template_name", [
|
||||
"key_1" => "value_1",
|
||||
"key_2" => "value_2"
|
||||
]);
|
||||
```
|
||||
|
||||
```php
|
||||
# template.php
|
||||
<html>
|
||||
<head>
|
||||
<title><?= $key_1?></title>
|
||||
</head>
|
||||
|
||||
<body>
|
||||
<h1>Hello <?= $key_2 ?></h1>
|
||||
</body>
|
||||
</html>
|
||||
```
|
||||
|
||||
Variables in the template are created through an associtve array `key => value`. The key (`"key"`) becomes a variable (`$key`) in the template.
|
||||
|
||||
### Layout
|
||||
|
||||
It's possible to create a layout (a model) for a group of pages to make that identical save for the contents.
|
||||
In a layout it's possible to create a section called **content** that identifies content that can be specified at runtime.
|
||||
|
||||
**NOTE**: Sinsce only the template has the data passed eventual loops have to be done there.
|
||||
|
||||
```php
|
||||
# index.php
|
||||
require 'vendor/autoload.php';
|
||||
use League\Plates\Engine;
|
||||
$template = new Engine('/path/to/templates');
|
||||
echo $template->render('template_name', [ "marker" => value, ... ]);
|
||||
```
|
||||
|
||||
```php
|
||||
# template.php
|
||||
|
||||
# set the layout used for this template
|
||||
<?php $this->layout("layout_name", ["marker" => value, ...]) ?> # pass values to the layout
|
||||
|
||||
# section contents
|
||||
<p> <?= $this->e($marker) ?> </p>
|
||||
```
|
||||
|
||||
```php
|
||||
# layout.php
|
||||
<html>
|
||||
<head>
|
||||
<title><?= $marker ?></title>
|
||||
</head>
|
||||
<body>
|
||||
<?= $this->section('content')?> # insert the section
|
||||
</body>
|
||||
</html>
|
||||
```
|
||||
|
||||
### Escape
|
||||
|
||||
It's necessary to verify that the output is in the necessary format.
|
||||
|
||||
Plates offerts `$this->escape()` or `$this->e()` to validate the output.
|
||||
In general the output validation allows toprevent [Cross-Site Scripting][owasp-xss] (XSS).
|
||||
|
||||
[owasp-xss]: https://owasp.org/www-community/attacks/xss/
|
||||
|
||||
### Folders
|
||||
|
||||
```php
|
||||
# index.php
|
||||
$templates->addFolder("alias", "path/to/template/folder"); # add a template folder
|
||||
echo $templates->render("folder::template"); # use a template in a specific folder
|
||||
```
|
||||
|
||||
### Insert
|
||||
|
||||
It's possible to inject templates in a layout or template. It is done by using the `insert()` function.
|
||||
|
||||
```php
|
||||
# layout.php
|
||||
<html>
|
||||
<head>
|
||||
<title><?=$this->e($title)?></title>
|
||||
</head>
|
||||
<body>
|
||||
<?php $this->insert('template::header') ?> # insert template
|
||||
|
||||
<?= $this->section('content')?> # page contents
|
||||
|
||||
<?php $this->insert('template::footer') ?> # insert template
|
||||
</body>
|
||||
</html>
|
||||
```
|
||||
|
||||
### Sections
|
||||
|
||||
It's possible to insert page contest from another template with the `section()` function.
|
||||
The content to be inserted must be surrounded with by the `start()` and `stop()` functions.
|
||||
|
||||
```php
|
||||
# template.php
|
||||
|
||||
<?php $this->start("section_name") ?> # start section
|
||||
# section contents (HTML)
|
||||
<?php $this->stop() ?> # stop section
|
||||
|
||||
# append to section is existing, create if not
|
||||
<?php $this->push("section_name") ?>
|
||||
# section contents (HTML)
|
||||
<?php $this->end() ?>
|
||||
```
|
230
PHP/PHP Unit Testing.md
Normal file
230
PHP/PHP Unit Testing.md
Normal file
|
@ -0,0 +1,230 @@
|
|||
# PHP Unit Test
|
||||
|
||||
## Installation & Configuration
|
||||
|
||||
### Dev-Only Installation
|
||||
|
||||
```ps1
|
||||
composer require --dev phpunit/phpunit
|
||||
```
|
||||
|
||||
```json
|
||||
"require-dev": {
|
||||
"phpunit/phpunit": "<version>"
|
||||
}
|
||||
```
|
||||
|
||||
### Config
|
||||
|
||||
PHPUnit can be configured in a XML file called `phpunit.xml`:
|
||||
|
||||
```xml
|
||||
<phpunit xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
|
||||
xsi:noNamespaceSchemaLocation="vendor/phpunit/phpunit/phpunit.xsd"
|
||||
bootstrap="vendor/autoload.php"
|
||||
colors="true">
|
||||
|
||||
<testsuites>
|
||||
<testsuit name="App\\Tests">
|
||||
<directory>./test<directory>
|
||||
</testsuit>
|
||||
</testsuites>
|
||||
|
||||
<filter>
|
||||
<whitelist processUncoveredFilesFromWhitelist="true">
|
||||
<directory suffix=".php">./src</directory>
|
||||
</whitelist>
|
||||
</filter>
|
||||
</phpunit>
|
||||
```
|
||||
|
||||
## Testing
|
||||
|
||||
### Test Structure
|
||||
|
||||
**PHPUnit** tests are grouped in classes suffixed with `Test`. Each class *extends* `PHPUnit\Framework\TestCase`. A test is a method of a *test class* prefized with `test`.
|
||||
PHPUnit is executed from the command line with `vendor/bin/phpunit --colors`.
|
||||
|
||||
```php
|
||||
namespace App;
|
||||
|
||||
class Filter
|
||||
{
|
||||
public function isEmail(string $email): bool
|
||||
{
|
||||
// @todo implement
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
```php
|
||||
namespace App\Test;
|
||||
use PHPUnit\Framework\TestCase;
|
||||
use App\Filter;
|
||||
|
||||
class FilterTest extends TestCase
|
||||
{
|
||||
public function testValidMail()
|
||||
{
|
||||
$filter = new Filter();
|
||||
$this->assertTrue($filter->isEmail("foo@bar.com"));
|
||||
}
|
||||
|
||||
public function testInvalidEmail()
|
||||
{
|
||||
$filter = new Filter();
|
||||
$this->assertFalse($filter->idEmail("foo"));
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### [PHPUnit Assertions](https://phpunit.readthedocs.io/en/9.3/assertions.html)
|
||||
|
||||
- `asseretTrue()`: verifies that the element is true
|
||||
- `assertFalse()`: verifies that the element is false
|
||||
- `assertEmpty()`: verifies that the element is empty
|
||||
- `assertEquals()`: verifies that the two elements are equal
|
||||
- `assertGreaterThan()`: verifies that the element is greter than ...
|
||||
- `assertContains()`: verifies that the element is contained in an array
|
||||
- `assertInstanceOf()`: verifies that the element is an instance of a specific class
|
||||
- `assertArrayHasKey(mixed $key, array $array)`: verify taht a sopecific key is in the array
|
||||
|
||||
### [PHPUnit Testing Exceptions](https://phpunit.readthedocs.io/en/9.3/writing-tests-for-phpunit.html#testing-exceptions)
|
||||
|
||||
```php
|
||||
public function testAggiungiEsameException(string $esame)
|
||||
{
|
||||
$this->expectException(Exception::class);
|
||||
$this->expectExceptionMessage("exception_message");
|
||||
|
||||
// execute code that sould throw an exception
|
||||
}
|
||||
|
||||
// https://github.com/sebastianbergmann/phpunit/issues/2484#issuecomment-648822531
|
||||
public function testExceptionNotTrown()
|
||||
{
|
||||
$exceptioWasTrown = false;
|
||||
|
||||
try
|
||||
{
|
||||
// code that should succeed
|
||||
}
|
||||
catch (EsameException $e)
|
||||
{
|
||||
$exceptioWasTrown = true;
|
||||
}
|
||||
|
||||
$this->assertFalse($exceptioWasTrown);
|
||||
}
|
||||
|
||||
// same as
|
||||
|
||||
/**
|
||||
* @dataProvider provideExamNames
|
||||
* @doesNotPerformAssertions
|
||||
*/
|
||||
public function testAggiungiEsameNoExceptions(string $esame)
|
||||
{
|
||||
$this->studente->aggiungiEsame($esame);
|
||||
}
|
||||
```
|
||||
|
||||
### Test Setup & Teardown
|
||||
|
||||
```php
|
||||
class ClassTest extends TestCase
|
||||
{
|
||||
// initialize the test
|
||||
public function setUp(): void
|
||||
{
|
||||
file_put_contents("/tmp/foo", "Test")
|
||||
}
|
||||
|
||||
// reset the test
|
||||
public function tearDown(): void
|
||||
{
|
||||
unlink("/tmp/foo")
|
||||
}
|
||||
|
||||
public function testFoo()
|
||||
{
|
||||
// use temp file
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
**NOTE**: `setUp()` and `tearDown()` are called *before* and *after* each test method.
|
||||
|
||||
### Data Provider
|
||||
|
||||
```php
|
||||
class DataTest extends TestCase
|
||||
{
|
||||
/**
|
||||
* @dataProvider provider
|
||||
*/
|
||||
public function testAdd($a, $b, $expected)
|
||||
{
|
||||
$this->assertEquals($expected, $a + $b);
|
||||
}
|
||||
|
||||
// test recieves array contents as input
|
||||
public function provider()
|
||||
{
|
||||
// must return array of arrays
|
||||
return [
|
||||
[0, 0, 0],
|
||||
[0, 1, 1]
|
||||
];
|
||||
}
|
||||
|
||||
// test recieves array of arrays as input
|
||||
public function provideArrayOfArrays()
|
||||
{
|
||||
return [
|
||||
[
|
||||
[
|
||||
[0, 0, 0],
|
||||
[0, 1, 1]
|
||||
]
|
||||
]
|
||||
];
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Mock Objects
|
||||
|
||||
```php
|
||||
class UnitTest extends TestCase
|
||||
{
|
||||
public function setUp()
|
||||
{
|
||||
// names of mock are independent from tested class variables
|
||||
$this->mock = $this->createMock(ClassName::class); // create a mock object of a class
|
||||
$this->returned = $this->createMock(ClassName::class); // mock of returned object
|
||||
|
||||
$this->mock->method("methodName") // simulate method on mock
|
||||
->with($this->equalTo(param), ...) // specify input params (one param per equalTo)
|
||||
->willReturn($this->returned); // specify return value
|
||||
}
|
||||
|
||||
public function testMethod()
|
||||
{
|
||||
$this->mock
|
||||
->method("methodName")
|
||||
->with($this->equalTo($arg)) // arg passed to the method
|
||||
->willReturn(value); // actual return value for THIS case
|
||||
// or
|
||||
->will($this->throwException(new Exception())); // method will throw exception
|
||||
|
||||
// assertions
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Code Coverage (needs [XDebug](https://xdebug.org/))
|
||||
|
||||
```ps1
|
||||
vendor/bin/phpunit --coverage-text # code cverage analisys in the terminal
|
||||
```
|
151
PHP/PHP Web.md
Normal file
151
PHP/PHP Web.md
Normal file
|
@ -0,0 +1,151 @@
|
|||
# PHP for the Web
|
||||
|
||||
## PHP Internal Web Server
|
||||
|
||||
Command Line Web Server in PHP, useful in testing phase. Limited since handles only one request at a time. **Do not use in production**.
|
||||
|
||||
```ps1
|
||||
PHP -S <ip:post> # start web server
|
||||
PHP -S <ip:post> -t /path/to/folder # execute in specified folder at specified address
|
||||
PHP -S <ip:post> file.php # redirect requests to single file
|
||||
```
|
||||
|
||||
## HTTP Methods
|
||||
|
||||
Handling of HTTP requests happend using the following global variables:
|
||||
|
||||
- `$_SERVER`: info on request headers, version, URL path and method (dict)
|
||||
- `$_GET`: parameters of get request (dict)
|
||||
- `$_POST`: parameters of post request (dict)
|
||||
- `$_COOKIE`
|
||||
- `$_FILES`: file to send to web app.
|
||||
|
||||
### `$_FILES`
|
||||
|
||||
```html
|
||||
<!-- method MUST BE post -->
|
||||
<!-- must have enctype="multipart/form-data" attribute -->
|
||||
<form name="invio_file" action="file.php" method="POST" enctype="multipart/form-data">
|
||||
<input type="file" name="photo" />
|
||||
<input type="submit" name="Invio" />
|
||||
</form>
|
||||
```
|
||||
|
||||
Files in `$_FILES` are memorized in a system temp folder. They can be moved with `move_uploaded_file()`
|
||||
|
||||
```php
|
||||
if (! isset($_FILES['photo']['error'])) {
|
||||
http_response_code(400); # send a response code
|
||||
echo'<h1>Non è stato inviato nessun file</h1>';
|
||||
exit();
|
||||
}
|
||||
|
||||
if ($_FILES['photo']['error'] != UPLOAD_ERR_OK) {
|
||||
http_response_code(400);
|
||||
echo'<h1>Il file inviato non è valido</h1>';
|
||||
exit();
|
||||
}
|
||||
|
||||
$path = '/path/to/' . $_FILES['photo']['name'];
|
||||
|
||||
if (! move_uploaded_file($_FILES['photo']['tmp_name'], $path)) {
|
||||
http_response_code(400);
|
||||
echo'<h1>Errore durante la scrittura del file</h1>';
|
||||
exit();
|
||||
}
|
||||
|
||||
echo'<h1>File inviato con successo</h1>';
|
||||
```
|
||||
|
||||
### `$_SERVER`
|
||||
|
||||
Request Header Access:
|
||||
|
||||
```php
|
||||
$_SERVER["REQUEST_METHOD"];
|
||||
$_SERVER["REQUEST_URI"];
|
||||
$_SERVER["SERVER_PROTOCOL"]; // HTTP Versions
|
||||
$_SERVER["HTTP_ACCEPT"];
|
||||
$_SERVER["HTTP_ACCEPT_ENCODING"];
|
||||
$_SERVER["HTTP_CONNECTION"];
|
||||
$_SERVER["HTTP_HOST"];
|
||||
$_SERVER["HTTP_USER_AGENT"];
|
||||
// others
|
||||
```
|
||||
|
||||
### `$_COOKIE`
|
||||
|
||||
[Cookie Laws](https://www.iubenda.com/it/cookie-solution)
|
||||
[Garante Privacy 8/5/2014](http://www.privacy.it/archivio/garanteprovv201405081.html)
|
||||
|
||||
All sites **must** have a page for the consensus about using cookies.
|
||||
|
||||
**Cookies** are HTTP headers used to memorize key-value info *on the client*. They are sent from the server to the client to keep track of info on the user that is visting the website.
|
||||
When a client recieves a HTTP response that contains `Set-Cookie` headers it has to memorize that info and reuse them in future requests.
|
||||
|
||||
```http
|
||||
Set-Cookie: <cookie-name>=<cookie-value>
|
||||
Set-Cookie: <cookie-name>=<cookie-value>; Expires=<date>
|
||||
Set-Cookie: <cookie-name>=<cookie-value>; Max-Age=<seconds>
|
||||
Set-Cookie: <cookie-name>=<cookie-value>; Domain=<domain-value>
|
||||
Set-Cookie: <cookie-name>=<cookie-value>; Path=<path-value>
|
||||
Set-Cookie: <cookie-name>=<cookie-value>; Secure
|
||||
Set-Cookie: <cookie-name>=<cookie-value>; HttpOnly
|
||||
```
|
||||
|
||||
Anyone can modify the contents of a cookie; for this reason cookies **must not contain** *personal or sensible info*.
|
||||
|
||||
When a clien has memorized a cookie, it is sent in successive HTTP requests through the `Cookie` header.
|
||||
|
||||
```http
|
||||
Cookie: <cookie-name>=<cookie-value>
|
||||
```
|
||||
|
||||
[PHP setcookie docs](https://www.php.net/manual/en/function.setcookie.php)
|
||||
|
||||
```php
|
||||
setcookie (
|
||||
string $name,
|
||||
[ string $value = "" ],
|
||||
[ int $expire = 0 ], // in seconds (time() + seconds)
|
||||
[ string $path = "" ],
|
||||
[ string $domain = "" ],
|
||||
[ bool $secure = false ], // use https
|
||||
[ bool $httponly = false ] // accessible only through http (no js, ...)
|
||||
)
|
||||
|
||||
// examle: memorize user-id 112 with 24h expiry for site example.com
|
||||
setcookie ("User-id", "112", time() + 3600*24, "/", "example.com");
|
||||
|
||||
// check if a cookie exists
|
||||
if(isset($_COOKIE["cookie_name"])) {}
|
||||
```
|
||||
|
||||
### [$_SESSION](https://www.php.net/manual/en/ref.session.php)
|
||||
|
||||
**Sessions** are info memorized *on the server* assoiciated to the client that makes an HTTP request.
|
||||
|
||||
PHP generates a cookie named `PHPSESSID` containing a *session identifier* and an *hash* generated from `IP + timestamp + pseudo-random number`.
|
||||
|
||||
To use the session it's necesary to recall the function `session_start()` at the beginning of a PHP script that deals with sesssions; after starting the session information in be savend in the `$_SESSION` array.
|
||||
|
||||
```php
|
||||
$_SESSION["key"] = value; // save data in session file (serialized data)
|
||||
|
||||
unset($_SESSION["key"]); // delete data from the session
|
||||
session_unset(); # remove all session data
|
||||
session_destroy(); # destroy all of the data associated with the current session.
|
||||
# It does not unset any of the global variables associated with the session, or unset the session cookie.
|
||||
```
|
||||
|
||||
Session data is be memorized in a file by *serializing* `$_SESSION`. Files are named as `sess_PHPSESSID` in a folder (`var/lib/php/sessions` in Linux).
|
||||
|
||||
It's possible to modify the memorization system of PHP serialization variables by:
|
||||
|
||||
- modifying `session.save_handler` in `php.ini`
|
||||
- writing as personalized handler with the function `session_set_save_handler()` and/or the class `SessionHandler`
|
||||
|
||||
## PHP Web Instructions
|
||||
|
||||
`http_response_code()` is used to return an HTTP response code. If no code is specified `200 OK` is returned.
|
||||
`header("Location: /route")` is used to make a redirect to another UTL.
|
926
PHP/PHP.md
Normal file
926
PHP/PHP.md
Normal file
|
@ -0,0 +1,926 @@
|
|||
# PHP CheatSheet
|
||||
|
||||
[PHP Docs](https://www.php.net/docs.php)
|
||||
|
||||
```php
|
||||
declare(strict_types=1); # activates variable type checking on function arguments
|
||||
# single line comment
|
||||
//single line comment
|
||||
/* multi line comment */
|
||||
```
|
||||
|
||||
## Include, Require
|
||||
|
||||
```php
|
||||
include "path\\file.php"; # import an external php file, E_WARNING if fails
|
||||
include_once "path\\file.php"; # imports only if not already loaded
|
||||
|
||||
require "path\\file.php"; # # import an external php file, E_COMPILE_ERROR if fails
|
||||
require_once "path\\file.php"; # imports only if not already loaded
|
||||
```
|
||||
|
||||
### Import configs from a file with `include`
|
||||
|
||||
In `config.php`:
|
||||
|
||||
```php
|
||||
//config.php
|
||||
|
||||
//store configuration options in associtive array
|
||||
return [
|
||||
setting => value,
|
||||
setting = value,
|
||||
]
|
||||
```
|
||||
|
||||
```php
|
||||
$config = include "config.php"; // retireve config wnd store into variable
|
||||
```
|
||||
|
||||
## Namespace
|
||||
|
||||
[PSR-4 Spec](https://www.php-fig.org/psr/psr-4/)
|
||||
|
||||
```php
|
||||
namespace Foo\Bar\Baz; # set nemespace for all file contents, \ for nested namespaces
|
||||
|
||||
use <PHP_Class> # using a namespace hides standard php classes (WHY?!?)
|
||||
|
||||
# namespace for only a block of code
|
||||
namespace Foo\Bar\Baz {
|
||||
function func() {
|
||||
# coded here
|
||||
}
|
||||
};
|
||||
|
||||
|
||||
Foo\Bar\Baz\func(); # use function from Foo\Bar\Baz withous USE instruction
|
||||
|
||||
use Foo\Bar\Baz\func; # import namespace
|
||||
func(); # use function from Foo\Bar\Baz
|
||||
|
||||
use Foo\Bar\Baz\func as f; # use function with an alias
|
||||
f(); # use function from Foo\Bar\Baz
|
||||
|
||||
use Foo\Bar\Baz as fbb # use namespace with alias
|
||||
fnn\func(); # use function from Foo\Bar\Baz
|
||||
```
|
||||
|
||||
## Basics
|
||||
|
||||
```php
|
||||
declare(strict_types=1); # activates type checking
|
||||
# single line comment
|
||||
//single line comment
|
||||
/* multi line comment */
|
||||
```
|
||||
|
||||
### Screen Output
|
||||
|
||||
```php
|
||||
echo "string"; # string output
|
||||
echo 'string\n'; # raw string output
|
||||
printf("format", $variables); # formatted output of strings and variables
|
||||
sprintf("format", $variables); # return formatted string
|
||||
```
|
||||
|
||||
### User Input
|
||||
|
||||
```php
|
||||
$var = readline("prompt");
|
||||
|
||||
# if readline is not installed
|
||||
if (!function_exists('readline')) {
|
||||
function readline($prompt)
|
||||
{
|
||||
$fh = fopen('php://stdin', 'r');
|
||||
echo $prompt;
|
||||
$userInput = trim(fgets($fh));
|
||||
fclose($fh);
|
||||
|
||||
return $userInput;
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## Variables
|
||||
|
||||
```php
|
||||
$varibleName = value; # weakly typed
|
||||
echo gettype(&variable); # output type of variable
|
||||
|
||||
var_dump($var); # prints info of variable (bit dimension, type & value)
|
||||
```
|
||||
|
||||
### Integers
|
||||
|
||||
```php
|
||||
&max = PHP_INT_MAX; # max value for int type -> 9223372036854775807
|
||||
&min = PHP_INT_MIN; # min value for int type -> -9223372036854775808
|
||||
&bytes = PHP_INT_SIZE; # bytes for int type -> 8
|
||||
|
||||
&num = 255; # decimal
|
||||
&num = 0b11111111; # binary
|
||||
&num = 0377; # octal
|
||||
&num = 0xff; # hexadecimal
|
||||
```
|
||||
|
||||
### Double
|
||||
|
||||
```php
|
||||
$a = 1.234; // 1.234
|
||||
$b = 1.2e3; // 1200
|
||||
$c = 7E-10; // 0.0000000007
|
||||
```
|
||||
|
||||
### Mathematical Operators
|
||||
|
||||
| Operator | Operation |
|
||||
|----------|----------------|
|
||||
| `-` | Subtraction |
|
||||
| `*` | Multiplication |
|
||||
| `/` | Division |
|
||||
| `%` | Modulo |
|
||||
| `**` | Exponentiatio |
|
||||
| `var++` | Post Increment |
|
||||
| `++var` | Pre Increment |
|
||||
| `var--` | Post Decrement |
|
||||
| `--var` | Pre Decrement |
|
||||
|
||||
### Mathematical Functions
|
||||
|
||||
- `sqrt($x)`
|
||||
- `sin($x)`
|
||||
- `cos($x)`
|
||||
- `log($x)`
|
||||
- `round($x)`
|
||||
- `floor($x)`
|
||||
- `ceil($x)`
|
||||
|
||||
## Strings
|
||||
|
||||
A string is a sequrnce of ASCII characters. In PHP a string is an array of charachters.
|
||||
|
||||
### String Concatenation
|
||||
|
||||
```php
|
||||
$string1 . $string2; method 1
|
||||
$string1 .= $string2; # method 2
|
||||
```
|
||||
|
||||
### String Functions
|
||||
|
||||
```php
|
||||
strlen($string); # returns the string length
|
||||
strpos($string, 'substring'); # position of dubstring in string
|
||||
substr($string, start, len); # ectract substring of len from position start
|
||||
strtoupper($string); # transfrorm to uppercase
|
||||
strtolower($string); # transform to lowerchase
|
||||
|
||||
explode(delimiter, string); # return array of substrings
|
||||
|
||||
$var = sprintf("format", $variables) # construct and return a formatted string
|
||||
```
|
||||
|
||||
## Constants
|
||||
|
||||
```php
|
||||
define ('CONSTANT_NAME', 'value')
|
||||
```
|
||||
|
||||
### Magic Constants `__NAME__`
|
||||
|
||||
- `__FILE__`: sctipt path + scrit filename
|
||||
- `__DIR__`: file directory
|
||||
- `__LINE__`: current line number
|
||||
- `__FUNCTION__`: the function name, or {closure} for anonymous functions.
|
||||
- `__CLASS__`: name of the class
|
||||
- `__NAMESPACE__`: the name of the current namespace.
|
||||
|
||||
## Array
|
||||
|
||||
Heterogeneous sequence of values.
|
||||
|
||||
```php
|
||||
$array = (sequence_of_items); # array declaration and valorization
|
||||
$array = [sequence_of_items]; # array declaration and valorization
|
||||
|
||||
# index < 0 selecst items starting from the last
|
||||
$array[index]; # accest to the items
|
||||
$array[index] = value; # array valorization (can add items)
|
||||
|
||||
$array[] = value; # value appending
|
||||
```
|
||||
|
||||
### Multi Dimensional Array (Matrix)
|
||||
|
||||
Array of array. Can have arbitrary number of nested array
|
||||
|
||||
```php
|
||||
$matrix = [
|
||||
[1, 2, 3],
|
||||
[4, 5, 6],
|
||||
[7, 8, 9]
|
||||
];
|
||||
```
|
||||
|
||||
### Array Printing
|
||||
|
||||
Single instruction to print whole array is ``
|
||||
|
||||
```php
|
||||
$array = [1, 2, 3];
|
||||
print_r($array); # print all the array values
|
||||
```
|
||||
|
||||
### Array Functions
|
||||
|
||||
```php
|
||||
count($array); # returns number of items in the array
|
||||
array_sum($array) # sum of the array value
|
||||
sort($array); # quick sort
|
||||
in_array($item, $array); // check if item is in the array
|
||||
array_push($array, $item); // append item to the array
|
||||
unset($array[index]); # item (or variable) deletion
|
||||
|
||||
# array navigation
|
||||
current();
|
||||
key();
|
||||
next();
|
||||
prev();
|
||||
reset();
|
||||
end();
|
||||
|
||||
# sorting
|
||||
sort($array, $sort_flags="SORT_REGULAR");
|
||||
|
||||
array_values($array); # regenerates the array fixing the indexes
|
||||
|
||||
list($array1 [, $array2, ...]) = $data; # Python-like tuple unpacking
|
||||
```
|
||||
|
||||
### Associative Arrays
|
||||
|
||||
Associative arrays have a value as an index. Alternative names are *hash tables* or *dictionaries*.
|
||||
|
||||
```php
|
||||
$italianDay = [
|
||||
'Mon' => 'Lunedì',
|
||||
'Tue' => 'Martedì',
|
||||
'Wed' => 'Mercoledì',
|
||||
'Thu' => 'Giovedì',
|
||||
'Fri' => 'Venerdì',
|
||||
'Sat' => 'Sabato',
|
||||
'Sun' => 'Domenica'
|
||||
];
|
||||
|
||||
$italianDay["Mon"]; # evaluates to Lunedì
|
||||
```
|
||||
|
||||
## Conditional Instructions
|
||||
|
||||
### Conditional Opeartors
|
||||
|
||||
| Operator | Operation |
|
||||
|-------------|--------------------------|
|
||||
| $a `==` $b | value equality |
|
||||
| $a `===` $b | value & type equality |
|
||||
| $a `!=` $b | value inequality |
|
||||
| $a `<>` $b | value inequality |
|
||||
| $a `!==` $b | value or type inequality |
|
||||
| $a `<` $b | less than |
|
||||
| $a `>` $b | greater than |
|
||||
| $a `<=` $b | less or equal to |
|
||||
| $a `>=` $b | greater or equal to |
|
||||
| $a `<=>` $b | spaceship operator |
|
||||
|
||||
With `==` a string evaluates to `0`.
|
||||
|
||||
### Logical Operators
|
||||
|
||||
| Operator | Example | Result |
|
||||
|----------|-------------|------------------------------------------------------|
|
||||
| `and` | `$a and $b` | TRUE if both `$a` and `$b` are TRUE. |
|
||||
| `or` | `$a or $b` | TRUE if either `$a` or `$b` is TRUE. |
|
||||
| `xor` | `$a xor $b` | TRUE if either `$a` or `$b` is TRUE, but *not both*. |
|
||||
| `not` | `!$a` | TRUE if `$a` is *not* TRUE. |
|
||||
| `and` | `$a && $b` | TRUE if both `$a` and `$b` are TRUE. |
|
||||
| `or` | `$a || $b` | TRUE if either `$a` or `$b` is TRUE. |
|
||||
|
||||
### Ternary Operator
|
||||
|
||||
```php
|
||||
condition ? result_if_true : result_if_false;
|
||||
condition ?: result_if_false;
|
||||
```
|
||||
|
||||
### NULL Coalesce
|
||||
|
||||
```php
|
||||
$var1 = $var2 ?? value; # if variable == NULL assign value, othervise return value of $var2
|
||||
|
||||
# equivalent to
|
||||
$var1 = isset($var2) ? $var2 : value
|
||||
```
|
||||
|
||||
### Spaceship Operator
|
||||
|
||||
```php
|
||||
$a <=> $b;
|
||||
|
||||
# equivalen to
|
||||
if $a > $b
|
||||
return 1;
|
||||
if $a == $b
|
||||
return 0;
|
||||
if $a < $b
|
||||
return 0;
|
||||
```
|
||||
|
||||
### `If` - `Elseif` - `Else`
|
||||
|
||||
```php
|
||||
if (condition) {
|
||||
# code here
|
||||
} elseif (condition) {
|
||||
# code here
|
||||
} else {
|
||||
# code here
|
||||
}
|
||||
|
||||
if (condition) :
|
||||
# code here
|
||||
elseif (condition):
|
||||
# code here
|
||||
else:
|
||||
# code here
|
||||
endif;
|
||||
```
|
||||
|
||||
### Switch Case
|
||||
|
||||
```php
|
||||
# weak comparison
|
||||
switch ($var) {
|
||||
case value:
|
||||
# code here
|
||||
break;
|
||||
|
||||
default:
|
||||
# code here
|
||||
}
|
||||
|
||||
# strong comparison
|
||||
switch (true) {
|
||||
case $var === value:
|
||||
# code here
|
||||
break;
|
||||
|
||||
default:
|
||||
# code here
|
||||
}
|
||||
```
|
||||
|
||||
### Match Expression (PHP 8)
|
||||
|
||||
`match` can return values, doesn't require break statements, can combine conditions, uses strict type comparisons and doesn't do any type coercion.
|
||||
|
||||
```php
|
||||
$result = match($input) {
|
||||
0 => "hello",
|
||||
'1', '2', '3' => "world",
|
||||
};
|
||||
```
|
||||
|
||||
## Loops
|
||||
|
||||
### For, Foreach
|
||||
|
||||
```php
|
||||
for (init, condition, increment){
|
||||
# code here
|
||||
}
|
||||
|
||||
for (init, condition, increment):
|
||||
# code here
|
||||
endfor;
|
||||
|
||||
foreach($sequence as $item) {
|
||||
# code here
|
||||
}
|
||||
|
||||
foreach($sequence as $item):
|
||||
# code here
|
||||
endforeach;
|
||||
|
||||
# foreach on dicts
|
||||
foreach($sequence as $key => $value) {
|
||||
# code here
|
||||
}
|
||||
```
|
||||
|
||||
### While, Do-While
|
||||
|
||||
```php
|
||||
while (condition) {
|
||||
# code here
|
||||
}
|
||||
|
||||
while (condition):
|
||||
# code here
|
||||
endwhile;
|
||||
|
||||
do {
|
||||
# code here
|
||||
} while (condition);
|
||||
```
|
||||
|
||||
### Break, Continue, exit()
|
||||
|
||||
`break` stops the iteration.
|
||||
`continue` skips one cycle of the iteration.
|
||||
`exit()` terminates the execution of any PHP code.
|
||||
|
||||
## Functions
|
||||
|
||||
[Function Docstring](https://make.wordpress.org/core/handbook/best-practices/inline-documentation-standards/php/)
|
||||
|
||||
Parameters with default values are optional in the function call and must be the last ones in the function declaration. Return type is optional if type checking is diasbled.
|
||||
|
||||
```php
|
||||
declare(strict_types=1); # activates type checking
|
||||
|
||||
/**
|
||||
* Summary.
|
||||
*
|
||||
* Description.
|
||||
*
|
||||
* @since x.x.x
|
||||
*
|
||||
* @see Function/method/class relied on
|
||||
* @link URL
|
||||
* @global type $varname Description.
|
||||
* @global type $varname Description.
|
||||
*
|
||||
* @param type $var Description.
|
||||
* @param type $var Optional. Description. Default.
|
||||
* @return type Description.
|
||||
*/
|
||||
function functionName (type $parameter, $parameter = default_value): Type
|
||||
{
|
||||
# code here
|
||||
return <expression>;
|
||||
}
|
||||
```
|
||||
|
||||
### Void function
|
||||
|
||||
```php
|
||||
function functionName (type $parameter, $parameter = default_value): Void
|
||||
{
|
||||
# code here
|
||||
}
|
||||
```
|
||||
|
||||
### Passing a parameter by reference (`&$`)
|
||||
|
||||
```php
|
||||
function functionName (type &$parameter): Type
|
||||
{
|
||||
# code here
|
||||
return <expression>;
|
||||
}
|
||||
```
|
||||
|
||||
### Variable number of parameters, variadic operator (`...`)
|
||||
|
||||
```php
|
||||
function functionName (type $parameter, ...$args): Type
|
||||
function functionName (type $parameter, type ...$args): Type
|
||||
{
|
||||
# code here
|
||||
return <expression>;
|
||||
}
|
||||
```
|
||||
|
||||
### Nullable parameters
|
||||
|
||||
```php
|
||||
function functionName (?type $parameter): ?Type
|
||||
{
|
||||
# code here
|
||||
return <expression>;
|
||||
}
|
||||
```
|
||||
|
||||
## Anonymous Functions (Closure)
|
||||
|
||||
```php
|
||||
# declaration and assignement to variable
|
||||
$var = function (type $parameter) {
|
||||
# code here
|
||||
};
|
||||
|
||||
$var($arg);
|
||||
```
|
||||
|
||||
### Use Operator
|
||||
|
||||
```php
|
||||
# use imports a variable into the closure
|
||||
$foo = function (type $parameter) use ($average) {
|
||||
# code here
|
||||
}
|
||||
```
|
||||
|
||||
### Union Types (PHP 8)
|
||||
|
||||
**Union types** are a collection of two or more types which indicate that *either* one of those *can be used*.
|
||||
|
||||
```php
|
||||
public function foo(Foo|Bar $input): int|float;
|
||||
```
|
||||
|
||||
### Named Arguments (PHP 8)
|
||||
|
||||
Named arguments allow to pass in values to a function, by specifying the value name, to avoid taking their order into consideration.
|
||||
It's also possible to skip optional parameters.
|
||||
|
||||
```php
|
||||
function foo(string $a, string $b, ?string $c = null, ?string $d = null) { /* … */ }
|
||||
|
||||
foo(
|
||||
b: 'value b',
|
||||
a: 'value a',
|
||||
d: 'value d',
|
||||
);
|
||||
```
|
||||
|
||||
## Object Oriented Programming
|
||||
|
||||
### Scope & Visibility
|
||||
|
||||
`public` methods and attrivutes are visible to anyone (*default*).
|
||||
`private` methods and attrivutes are visible only inside the class in which are declared.
|
||||
`protected` methods and attributes are visible only to child classes.
|
||||
|
||||
`final` classes cannot be extended.
|
||||
|
||||
### Class Declaration & Instantiation
|
||||
|
||||
```php
|
||||
# case insensitive
|
||||
class ClassName
|
||||
{
|
||||
|
||||
const CONSTANT = value; # public by default
|
||||
|
||||
public $attribute; # null by default if not assigned
|
||||
public Type $attribute; # specifing the type is optional, it will be enforced if present
|
||||
|
||||
# class constructor
|
||||
public function __construct(value)
|
||||
{
|
||||
$this->attrivute = value
|
||||
}
|
||||
|
||||
public getAttribute(): Type
|
||||
{
|
||||
return $this->attribute;
|
||||
}
|
||||
|
||||
public function func(): Type
|
||||
{
|
||||
# code here
|
||||
}
|
||||
}
|
||||
|
||||
$object = new ClassName; # case insensitive (CLASSNAME, CLassName, classname)
|
||||
$object->attrivute = value;
|
||||
$object->func();
|
||||
$object::CONSTANT;
|
||||
|
||||
$var = $object; # copy by reference
|
||||
$var = clone $object # copy by value
|
||||
|
||||
$object instanceof ClassName // check typo of the object
|
||||
```
|
||||
|
||||
### Static classes, attributes & methods
|
||||
|
||||
Inside static methods it's impossible to use `$this`.
|
||||
A static variable is unique for the class and all instances.
|
||||
|
||||
```php
|
||||
class ClassName {
|
||||
|
||||
public static $var;
|
||||
|
||||
public static function func(){
|
||||
//code here
|
||||
}
|
||||
|
||||
public static function other_func(){
|
||||
//code here
|
||||
self::func();
|
||||
}
|
||||
}
|
||||
|
||||
ClassName::func(); // use static function
|
||||
|
||||
$obj = new ClassName();
|
||||
$obj::$var; // access to the static variable
|
||||
```
|
||||
|
||||
### [Dependency Injection](https://en.wikipedia.org/wiki/Dependency_injection)
|
||||
|
||||
Parameters of the dependency can be modified before passing the required class to the constructor.
|
||||
|
||||
```php
|
||||
class ClassName
|
||||
{
|
||||
private $dependency;
|
||||
|
||||
public function __construct(ClassName requiredClass)
|
||||
{
|
||||
$this->dependency = requiredClass; # necessary class is passed to the constructor
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Inheritance
|
||||
|
||||
If a class is defind `final` it can't be extended.
|
||||
If a function is declared `final` it can't be overridden.
|
||||
|
||||
```php
|
||||
class Child extends Parent
|
||||
{
|
||||
public __construct() {
|
||||
parent::__construct(); # call parent's method
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Abstract Class
|
||||
|
||||
Abstract calsses cannot be instantiated;
|
||||
|
||||
```php
|
||||
abstract class ClassName
|
||||
{
|
||||
# code here
|
||||
}
|
||||
```
|
||||
|
||||
### Interface
|
||||
|
||||
An interface is a "contract" that defines what methods the implementing classes **must** have and implement.
|
||||
|
||||
A class can implement multiple interfaces but there must be no methods in common between interface to avoid ambiguity.
|
||||
|
||||
```php
|
||||
interface InterfaceName {
|
||||
|
||||
// it is possible to define __construct
|
||||
|
||||
// function has no body; must be implements in the class that uses the interface
|
||||
public function functionName (parameters) : Type; // MUST be public
|
||||
}
|
||||
|
||||
|
||||
class ClassName implements InterfaceName {
|
||||
public function functionName(parameters) : Type {
|
||||
//implementation here
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### Traits
|
||||
|
||||
`Traits` allows the riutilization of code inside different classes without links of inheritance.
|
||||
It can be used to mitigate the problem of *multiple inheritance*, which is absent in PHP.
|
||||
|
||||
In case of functions name conflic it's possible to use `insteadof` to specify which function to use. It's also possible to use an *alias* to resolve the conflicts.
|
||||
|
||||
```php
|
||||
trait TraitName {
|
||||
// code here
|
||||
}
|
||||
|
||||
class ClassName {
|
||||
use TraitName, {TraitName::func() insteadof OtherTrait}, { func() as alias }; # can use multiple traits
|
||||
# code here
|
||||
}
|
||||
```
|
||||
|
||||
### Anonymous Classes
|
||||
|
||||
```php
|
||||
$obj = new ClassName;
|
||||
|
||||
$obj->method(new class implements Interface {
|
||||
public function InterfaceFunc() {
|
||||
// code here
|
||||
}
|
||||
});
|
||||
```
|
||||
|
||||
## Serialization & JSON
|
||||
|
||||
```php
|
||||
$serialized = serialize($obj); # serailization
|
||||
$obj = unserialize($serialized); # de-serialization
|
||||
|
||||
$var = json_decode(string $json, bool $associative); # Takes a JSON encoded string and converts it into a PHP variable.ù
|
||||
$json = json_encode($value); # Returns a string containing the JSON representation of the supplied value.
|
||||
```
|
||||
|
||||
## Files
|
||||
|
||||
### Read/Write on Files
|
||||
|
||||
```php
|
||||
file(filename); // return file lines in an array
|
||||
|
||||
// problematic with large files (allocates memory to read all file, can fill RAM)
|
||||
file_put_contents(filename, data); // write whole file
|
||||
file_get_contents(filename); // read whole file
|
||||
```
|
||||
|
||||
## Regular Expressions
|
||||
|
||||
```php
|
||||
preg_match('/PATTERN/', string $subject, array $matches); # returns 1 if the pattern matches given subject, 0 if it does not, or FALSE if an error occurred
|
||||
# $mathces[0] = whole matched string
|
||||
# $matches[i] = i-th group of the regex
|
||||
```
|
||||
|
||||
## Hashing
|
||||
|
||||
Supported hashing algrithms:
|
||||
|
||||
- `md2`, `md4`, `md5`
|
||||
- `sha1`, `sha224`, `sha256`, `sha384`, `sha512/224`, `sha512/256`, `sha512`
|
||||
- `sha3-224`, `sha3-256`, `sha3-384`, `sha3-512`
|
||||
- `ripemd128`, `ripemd160`, `ripemd256`, `ripemd320`
|
||||
- `whirlpool`
|
||||
- `tiger128,3`, `tiger160,3`, `tiger192,3`, `tiger128,4`, `tiger160,4`, `tiger192,4`
|
||||
- `snefru`, `snefru256`
|
||||
- `gost`, `gost-crypto`
|
||||
- `adler32`
|
||||
- `crc32`, `crc32b`, `crc32c`
|
||||
- `fnv132`, `fnv1a32`, `fnv164`, `fnv1a64`
|
||||
- `joaat`
|
||||
- `haval128,3`, `haval160,3`, `haval192,3`, `haval224,3`, `haval256,3`, `haval128,4`, `haval160,4`, `haval192,4`, `haval224,4`, `haval256,4`, `haval128,5`, `haval160,5`, `haval192,5`, `haval224,5`, `haval256,5`
|
||||
|
||||
```php
|
||||
hash($algorithm, $data);
|
||||
```
|
||||
|
||||
### Password Hashes
|
||||
|
||||
`password_hash()` is compatible with `crypt()`. Therefore, password hashes created by `crypt()` can be used with `password_hash()`.
|
||||
|
||||
Algorithms currently supported:
|
||||
|
||||
- **PASSWORD_DEFAULT** - Use the *bcrypt* algorithm (default as of PHP 5.5.0). Note that this constant is designed to change over time as new and stronger algorithms are added to PHP.
|
||||
- **PASSWORD_BCRYPT** - Use the **CRYPT_BLOWFISH** algorithm to create the hash. This will produce a standard `crypt()` compatible hash using the "$2y$" identifier. The result will always be a 60 character string, or FALSE on failure.
|
||||
- **PASSWORD_ARGON2I** - Use the **Argon2i** hashing algorithm to create the hash. This algorithm is only available if PHP has been compiled with Argon2 support.
|
||||
- **PASSWORD_ARGON2ID** - Use the **Argon2id** hashing algorithm to create the hash. This algorithm is only available if PHP has been compiled with Argon2 support.
|
||||
|
||||
**Supported options for PASSWORD_BCRYPT**:
|
||||
|
||||
- **salt** (string) - to manually provide a salt to use when hashing the password. Note that this will override and prevent a salt from being automatically generated.
|
||||
If omitted, a random salt will be generated by password_hash() for each password hashed. This is the intended mode of operation.
|
||||
**Warning**: The salt option has been deprecated as of PHP 7.0.0. It is now preferred to simply use the salt that is generated by default.
|
||||
|
||||
- **cost** (integer) - which denotes the algorithmic cost that should be used. Examples of these values can be found on the crypt() page.
|
||||
If omitted, a default value of 10 will be used. This is a good baseline cost, but you may want to consider increasing it depending on your hardware.
|
||||
|
||||
**Supported options for PASSWORD_ARGON2I and PASSWORD_ARGON2ID**:
|
||||
|
||||
- **memory_cost** (integer) - Maximum memory (in kibibytes) that may be used to compute the Argon2 hash. Defaults to PASSWORD_ARGON2_DEFAULT_MEMORY_COST.
|
||||
- **time_cost** (integer) - Maximum amount of time it may take to compute the Argon2 hash. Defaults to PASSWORD_ARGON2_DEFAULT_TIME_COST.
|
||||
- **threads** (integer) - Number of threads to use for computing the Argon2 hash. Defaults to PASSWORD_ARGON2_DEFAULT_THREADS.
|
||||
|
||||
```php
|
||||
password_hash($passwrd, $algorithm); # create a new password hash using a strong one-way hashing algorithm.
|
||||
password_verify($password, $hash); # Verifies that a password matches a hash
|
||||
```
|
||||
|
||||
## Errors
|
||||
|
||||
Types of PHP errors:
|
||||
|
||||
- **Fatal Error**: stop the execution of the program.
|
||||
- **Warning**: generataed at runtime, does not stop the execution (non-blocking).
|
||||
- **Notice**: informative errors or messages, non-blocking.
|
||||
|
||||
```php
|
||||
$a = new StdClass()
|
||||
$a->foo(); // PHP Fatal Error: foo() does not exist
|
||||
```
|
||||
|
||||
```php
|
||||
$a = 0;
|
||||
echo 1/$a; // PHP Warning: Division by zero
|
||||
```
|
||||
|
||||
```php
|
||||
echo $a; // PHP Notice: $a undefined
|
||||
```
|
||||
|
||||
### Error Reporting
|
||||
|
||||
[PHP Error Constants](https://www.php.net/manual/en/errorfunc.constants.php)
|
||||
|
||||
Its possible to configure PHP to signal only some type of errors. Errors below a certain levels are ignored.
|
||||
|
||||
```php
|
||||
error_reporting(E_<type>); // set error report threshold (for log file)
|
||||
// does not disable PARSER ERROR
|
||||
|
||||
ini_set("display_errors", 0); // don't display any errors on stderr
|
||||
ini_set("error_log", "path\\error.log"); // set log file
|
||||
```
|
||||
|
||||
### Triggering Errors
|
||||
|
||||
```php
|
||||
// generate E_USER_ errors
|
||||
trigger_error("message"); // defaul type: E_USER_NOTICE
|
||||
trigger_error("message", E_USER_<Type>);
|
||||
|
||||
trigger_error("Deprecated Function", E_USER_DEPRECATED);
|
||||
```
|
||||
|
||||
### [Writing in the Log File](https://www.php.net/manual/en/function.error-log.php)
|
||||
|
||||
It's possible to use log files unrelated to the php log file.
|
||||
|
||||
```php
|
||||
error_log("message", 3, "path\\log.log"); // write log message to a specified file
|
||||
|
||||
//example
|
||||
error_log(sprintf("[%s] Error: _", date("Y-m-d h:i:s")), 3, "path\\log.log")
|
||||
```
|
||||
|
||||
## Exception Handling
|
||||
|
||||
PHP offers the possibility to handle errors with the *exception model*.
|
||||
|
||||
```php
|
||||
try {
|
||||
// dangerous code
|
||||
|
||||
} catch(ExcpetionType1 | ExceptionType2 $e) {
|
||||
printf("Errore: %s", $e->getMessage());
|
||||
} catch(Excpetion $e) {
|
||||
// handle or report exception
|
||||
}
|
||||
|
||||
throw new ExceptionType("message"); // trow an exception
|
||||
```
|
||||
|
||||
All exceptions in PHP implement the interface `Throwable`.
|
||||
|
||||
```php
|
||||
Interface Throwable {
|
||||
abstract public string getMessage ( void )
|
||||
abstract public int getCode ( void )
|
||||
abstract public string getFile ( void )
|
||||
abstract public int getLine ( void )
|
||||
abstract public array getTrace ( void )
|
||||
abstract public string getTraceAsString ( void )
|
||||
abstract public Throwable getPrevious ( void )
|
||||
abstract public string __toString ( void )
|
||||
}
|
||||
```
|
||||
|
||||
### Custom Exceptions
|
||||
|
||||
```php
|
||||
/**
|
||||
* Define a custom exception class
|
||||
*/
|
||||
class CustomException extends Exception
|
||||
{
|
||||
// Redefine the exception so message isn't optional
|
||||
public function __construct($message, $code = 0, Exception $previous = null) {
|
||||
// some code
|
||||
|
||||
// make sure everything is assigned properly
|
||||
parent::__construct($message, $code, $previous);
|
||||
}
|
||||
|
||||
// custom string representation of object
|
||||
public function __toString() {
|
||||
return __CLASS__ . ": [{$this->code}]: {$this->message}\n";
|
||||
}
|
||||
|
||||
public function customFunction() {
|
||||
echo "A custom function for this type of exception\n";
|
||||
}
|
||||
}
|
||||
```
|
97
PHP/Simple_MVC/REST API.md
Normal file
97
PHP/Simple_MVC/REST API.md
Normal file
|
@ -0,0 +1,97 @@
|
|||
# REST API with Simple-MVC
|
||||
|
||||
## Routing (Example)
|
||||
|
||||
```php
|
||||
// config/route.php
|
||||
return [
|
||||
[ 'GET', '/api/user[/{id}]', Controller\User::class ],
|
||||
[ 'POST', '/api/user', Controller\User::class ],
|
||||
[ 'PATCH', '/api/user/{id}', Controller\User::class ],
|
||||
[ 'DELETE', '/api/user/{id}', Controller\User::class ]
|
||||
];
|
||||
```
|
||||
|
||||
## Controller (Example)
|
||||
|
||||
```php
|
||||
public class UserController implements ControllerInterface
|
||||
{
|
||||
public function __construct(UserModel $user)
|
||||
{
|
||||
$this->userModel = $user;
|
||||
|
||||
// Set the Content-type for all the HTTP methods
|
||||
header('Content-type: application/json');
|
||||
}
|
||||
|
||||
// method dispatcher
|
||||
public function execute(ServerRequestInterface $request)
|
||||
{
|
||||
$method = strtolower($request->getMethod());
|
||||
if (!method_exists($this, $method)) {
|
||||
http_response_code(405); // method not exists
|
||||
return;
|
||||
}
|
||||
$this->$method($request);
|
||||
}
|
||||
|
||||
public function get(ServerRequestInterface $request)
|
||||
{
|
||||
$id = $request->getAttribute('id');
|
||||
try {
|
||||
$result = empty($id)
|
||||
? $this->userModel->getAllUsers()
|
||||
: $this->userModel->getUser($id);
|
||||
} catch (UserNotFoundException $e) {
|
||||
http_response_code(404); // user not found
|
||||
$result = ['error' => $e->getMessage()];
|
||||
}
|
||||
echo json_encode($result);
|
||||
}
|
||||
|
||||
public function post(ServerRequestInterface $request)
|
||||
{
|
||||
$data = json_decode($request->getBody()->getContents(), true);
|
||||
try {
|
||||
$result = $this->userModel->addUser($data);
|
||||
} catch (InvalidAttributeException $e) {
|
||||
http_response_code(400); // bad request
|
||||
$result = ['error' => $e->getMessage()];
|
||||
} catch (UserAlreadyExistsException $e) {
|
||||
http_response_code(409); // conflict, the user is not present
|
||||
$result = ['error' => $e->getMessage()];
|
||||
}
|
||||
echo json_encode($result);
|
||||
}
|
||||
|
||||
public function patch(ServerRequestInterface $request)
|
||||
{
|
||||
$id = $request->getAttribute('id');
|
||||
$data = json_decode($request->getBody()->getContents(), true);
|
||||
try {
|
||||
$result = $this->userModel->updateUser($data, $id);
|
||||
} catch (InvalidAttributeException $e) {
|
||||
http_response_code(400); // bad request
|
||||
$result = ['error' => $e->getMessage()];
|
||||
} catch (UserNotFoundException $e) {
|
||||
http_response_code(404); // user not found
|
||||
$result = ['error' => $e->getMessage()];
|
||||
}
|
||||
echo json_encode($result);
|
||||
}
|
||||
|
||||
public function delete(ServerRequestInterface $request)
|
||||
{
|
||||
$id = $request->getAttribute('id');
|
||||
try {
|
||||
$this->userModel->deleteUser($id);
|
||||
$result = ['result' => 'success'];
|
||||
} catch (UserNotFoundException $e) {
|
||||
http_response_code(404); // user not found
|
||||
$result = ['error' => $e->getMessage()];
|
||||
}
|
||||
echo json_encode($result);
|
||||
}
|
||||
}
|
||||
```
|
144
PHP/Simple_MVC/Simple_MVC.md
Normal file
144
PHP/Simple_MVC/Simple_MVC.md
Normal file
|
@ -0,0 +1,144 @@
|
|||
# [SimpleMVC](https://github.com/ezimuel/simplemvc) Mini-Framework
|
||||
|
||||
Mini framework MVC per scopi didattici basato su Dependency Injection ([PHP-DI][php-di]), Routing ([FastRoute][fastroute]), PSR-7 ([nyholm/psr7][psr7]) e Templates ([Plates][plates])
|
||||
|
||||
[php-di]: https://php-di.org/
|
||||
[fastroute]: https://github.com/nikic/FastRoute
|
||||
[psr7]:https://github.com/Nyholm/psr7
|
||||
[plates]: https://platesphp.com/
|
||||
|
||||
## Installation
|
||||
|
||||
```ps1
|
||||
composer create-project ezimuel/simple-mvc
|
||||
```
|
||||
|
||||
## Structure
|
||||
|
||||
```txt
|
||||
|- config
|
||||
| |- container.php --> DI Container Config (PHP-DI)
|
||||
| |- route.php --> routing
|
||||
|- public
|
||||
| |- img
|
||||
| |- index.php --> app entrypoint
|
||||
|- src
|
||||
| |- Model
|
||||
| |- View --> Plates views
|
||||
| |- Controller --> ControllerInterface.php
|
||||
|- test
|
||||
| |- Model
|
||||
| |- Controller
|
||||
```
|
||||
|
||||
### `indedx.php`
|
||||
|
||||
```php
|
||||
<?php
|
||||
declare(strict_types=1);
|
||||
|
||||
chdir(dirname(__DIR__));
|
||||
require 'vendor/autoload.php';
|
||||
|
||||
use DI\ContainerBuilder;
|
||||
use FastRoute\Dispatcher;
|
||||
use FastRoute\RouteCollector;
|
||||
use Nyholm\Psr7\Factory\Psr17Factory;
|
||||
use Nyholm\Psr7Server\ServerRequestCreator;
|
||||
use SimpleMVC\Controller\Error404;
|
||||
use SimpleMVC\Controller\Error405;
|
||||
|
||||
$builder = new ContainerBuilder();
|
||||
$builder->addDefinitions('config/container.php');
|
||||
$container = $builder->build();
|
||||
|
||||
// Routing
|
||||
$dispatcher = FastRoute\simpleDispatcher(function(RouteCollector $r) {
|
||||
$routes = require 'config/route.php';
|
||||
foreach ($routes as $route) {
|
||||
$r->addRoute($route[0], $route[1], $route[2]);
|
||||
}
|
||||
});
|
||||
|
||||
// Build the PSR-7 server request
|
||||
$psr17Factory = new Psr17Factory();
|
||||
$creator = new ServerRequestCreator(
|
||||
$psr17Factory, // ServerRequestFactory
|
||||
$psr17Factory, // UriFactory
|
||||
$psr17Factory, // UploadedFileFactory
|
||||
$psr17Factory // StreamFactory
|
||||
);
|
||||
$request = $creator->fromGlobals();
|
||||
|
||||
// Dispatch
|
||||
$routeInfo = $dispatcher->dispatch(
|
||||
$request->getMethod(),
|
||||
$request->getUri()->getPath()
|
||||
);
|
||||
switch ($routeInfo[0]) {
|
||||
case Dispatcher::NOT_FOUND:
|
||||
$controllerName = Error404::class;
|
||||
break;
|
||||
case Dispatcher::METHOD_NOT_ALLOWED:
|
||||
$controllerName = Error405::class;
|
||||
break;
|
||||
case Dispatcher::FOUND:
|
||||
$controllerName = $routeInfo[1];
|
||||
if (isset($routeInfo[2])) {
|
||||
foreach ($routeInfo[2] as $name => $value) {
|
||||
$request = $request->withAttribute($name, $value);
|
||||
}
|
||||
}
|
||||
break;
|
||||
}
|
||||
$controller = $container->get($controllerName);
|
||||
$controller->execute($request);
|
||||
```
|
||||
|
||||
### `route.php`
|
||||
|
||||
```php
|
||||
<?php
|
||||
use SimpleMVC\Controller;
|
||||
|
||||
return [
|
||||
[ 'GET', '/', Controller\Home::class ],
|
||||
[ 'GET', '/hello[/{name}]', Controller\Hello::class ],
|
||||
[ "HTTP Verb", "/route[/optional]", Controller\EndpointController::class ]
|
||||
];
|
||||
```
|
||||
|
||||
### `container.php`
|
||||
|
||||
```php
|
||||
<?php
|
||||
use League\Plates\Engine;
|
||||
use Psr\Container\ContainerInterface;
|
||||
|
||||
return [
|
||||
'view_path' => 'src/View',
|
||||
Engine::class => function(ContainerInterface $c) {
|
||||
return new Engine($c->get('view_path'));
|
||||
}
|
||||
|
||||
// PHP-DI configs
|
||||
];
|
||||
```
|
||||
|
||||
### `ControllerInterface.php`
|
||||
|
||||
Each controller *must* implement this interface.
|
||||
|
||||
```php
|
||||
<?php
|
||||
declare(strict_types=1);
|
||||
|
||||
namespace SimpleMVC\Controller;
|
||||
|
||||
use Psr\Http\Message\ServerRequestInterface;
|
||||
|
||||
interface ControllerInterface
|
||||
{
|
||||
public function execute(ServerRequestInterface $request);
|
||||
}
|
||||
```
|
42
PowerShell/Powershell Commands.md
Normal file
42
PowerShell/Powershell Commands.md
Normal file
|
@ -0,0 +1,42 @@
|
|||
# Powershell Commands
|
||||
|
||||
```ps1
|
||||
Get-Location # Gets information about the current working location or a location stack
|
||||
Set-Location -Path <path> # change current working directory to specified path (DEFAULTs to ~)
|
||||
Get-ChildItem -Path <path> # Gets the items and child items in one or more specified locations.
|
||||
Get-Content -Path <file> # Gets the content of the item at the specified location
|
||||
|
||||
Write-Output # Send specified objects to the next command in the pipeline. If the command is the last in the pipeline, the objects are displayed in the console
|
||||
Write-Host # Writes customized output to a host.
|
||||
Clear-Host # clear shell output
|
||||
|
||||
New-Item -ItemType File -Path filename.ext # create empty file
|
||||
New-Item -Path folder_name -Type Folder # create a folder
|
||||
New-Item -ItemType SymbolicLink -Path .\link -Target .\Notice.txt # create a symlink
|
||||
|
||||
Move-Item -Path <source> -Destination <target> # move and/or rename files and folders
|
||||
Copy-Item -Path <source> -Destination <target> # copy (and rename) files and folders
|
||||
|
||||
Test-Path "path" -PathType Container # check if the exising path exists and is a folder
|
||||
Test-Path "path" -PathType Leaf # check if the exising path exists and is a file
|
||||
|
||||
# start, list , kill processes
|
||||
Start-Process -FilePath <file> # open a file with the default process/program
|
||||
Get-Process # Gets the processes that are running on the local computer
|
||||
Stop-Process [-Id] <System.Int32[]> [-Force] [-Confirm] # Stops one or more running processes
|
||||
|
||||
# network
|
||||
Get-NetIPConfiguration # Gets IP network configuration
|
||||
Test-NetConnection <ip> # Sends ICMP echo request packets, or pings, to one or more computers
|
||||
|
||||
# compressing into archive
|
||||
Compress-Archive -LiteralPath <PathToFiles> -DestinationPath <PathToDestination> # destination can be a folder or a .zip file
|
||||
Compress-Archive -Path <PathToFiles> -Update -DestinationPath <PathToDestination> # update existing archive
|
||||
|
||||
# extraction from archive
|
||||
Expand-Archive -LiteralPath <PathToZipFile> -DestinationPath <PathToDestination>
|
||||
Expand-Archive -LiteralPath <PathToZipFile> # extract archive in folder named after the archive in the root location
|
||||
|
||||
# filtering stdout/stder
|
||||
Select-String -Path <source> -Pattern <pattern> # Finds text in strings and files
|
||||
```
|
445
PowerShell/Powershell Scripting.md
Normal file
445
PowerShell/Powershell Scripting.md
Normal file
|
@ -0,0 +1,445 @@
|
|||
# PowerShell Cheat Sheet
|
||||
|
||||
Cmdlets are formed by a verb-noun pair. Cmdlets are case-insensitive.
|
||||
|
||||
**It's all .NET**
|
||||
A PS string is in fact a .NET System.String
|
||||
All .NET methods and properties are thus available
|
||||
|
||||
Note that .NET functions MUST be called with parentheses while PS functions CANNOT be called with parentheses.
|
||||
If you do call a cmdlet/PS function with parentheses, it is the same as passing a single parameter list.
|
||||
|
||||
## Screen Output
|
||||
|
||||
```ps1
|
||||
Write-Host "message"
|
||||
```
|
||||
|
||||
## User Input
|
||||
|
||||
```ps1
|
||||
# Reading a value from input:
|
||||
$variable = Read-Host "prompt"
|
||||
```
|
||||
|
||||
## Variables
|
||||
|
||||
```ps1
|
||||
# Declaration
|
||||
[type]$var = value
|
||||
$var = value -as [type]
|
||||
|
||||
[int]$a = 5
|
||||
$b = 6 -as [double] # ?
|
||||
|
||||
# Here-string (multiline string)
|
||||
@"
|
||||
Here-string
|
||||
$a + $b = ($a + $b)
|
||||
"@
|
||||
|
||||
@'
|
||||
Literal Here-string
|
||||
'@
|
||||
|
||||
# Swapping
|
||||
$a, $b = $b, $a
|
||||
|
||||
# Interpolation
|
||||
Write-Host "text $variable" # single quotes will not interpolate
|
||||
Write-Host (<expresion>)
|
||||
```
|
||||
|
||||
### Built-in Variables
|
||||
|
||||
```ps1
|
||||
$True, $False # boolean
|
||||
$null # empty value
|
||||
$? # last program return value
|
||||
$LastExitCode # Exit code of last run Windows-based program
|
||||
$$ # The last token in the last line received by the session
|
||||
$^ # The first token
|
||||
$PID # Script's PID
|
||||
$PSScriptRoot # Full path of current script directory
|
||||
$MyInvocation.MyCommand.Path # Full path of current script
|
||||
$Pwd # Full path of current directory
|
||||
$PSBoundParameters # Bound arguments in a function, script or code block
|
||||
$Args # Unbound arguments
|
||||
|
||||
. .\otherScriptName.ps1 # Inline another file (dot operator)
|
||||
```
|
||||
|
||||
### Lists & Dictionaries
|
||||
|
||||
```ps1
|
||||
$List = @(5, "ice", 3.14, $True) # Explicit syntax
|
||||
$List = 2, "ice", 3.14, $True # Implicit syntax
|
||||
$List = (1..10) # Inclusive range
|
||||
$List = @() # Empty List
|
||||
|
||||
$String = $List -join 'separator'
|
||||
$List = $String -split 'separator'
|
||||
|
||||
# List comprhensions
|
||||
$List = sequence | Where-Object {$_ command} # $_ is current object
|
||||
$Dict = @{"a" = "apple"; "b" = "ball"} # Dict definition
|
||||
$Dict["a"] = "acron" # Item update
|
||||
|
||||
# Loop through keys
|
||||
foreach ($k in $Dict.keys) {
|
||||
# Code here
|
||||
}
|
||||
```
|
||||
|
||||
## Flow Control
|
||||
|
||||
```ps1
|
||||
if (condition) {
|
||||
# Code here
|
||||
} elseif (condition) {
|
||||
# Code here
|
||||
} else {
|
||||
# Code here
|
||||
}
|
||||
```
|
||||
|
||||
### Switch
|
||||
|
||||
`Switch` has the following parameters:
|
||||
|
||||
- **Wildcard**: Indicates that the condition is a wildcard string. If the match clause is not a string, the parameter is ignored. The comparison is case-insensitive.
|
||||
- **Exact**: Indicates that the match clause, if it is a string, must match exactly. If the match clause is not a string, this parameter is ignored. The comparison is case-insensitive.
|
||||
- **CaseSensitive**: Performs a case-sensitive match. If the match clause is not a string, this parameter is ignored.
|
||||
- **File**: Takes input from a file rather than a value statement. If multiple File parameters are included, only the last one is used. Each line of the file is read and evaluated by the Switch statement. The comparison is case-insensitive.
|
||||
- **Regex**: Performs regular expression matching of the value to the condition. If the match clause is not a string, this parameter is ignored. The comparison is case-insensitive. The `$matches` automatic variable is available for use within the matching statement block.
|
||||
|
||||
```ps1
|
||||
switch(variable) {
|
||||
20 { "Exactly 20"; break }
|
||||
{ $_ -eq 42 } { "The answer equals 42"; break }
|
||||
{ $_ -like 's*' } { "Case insensitive"; break }
|
||||
{ $_ -clike 's*'} { "clike, ceq, cne for case sensitive"; break }
|
||||
{ $_ -notmatch '^.*$'} { "Regex matching. cnotmatch, cnotlike, ..."; break }
|
||||
{ $list -contains 'x'} { "if a list contains an item"; break }
|
||||
default { "Others" }
|
||||
}
|
||||
|
||||
# syntax
|
||||
switch [-regex|-wildcard|-exact][-casesensitive] (<value>)
|
||||
{
|
||||
"string"|number|variable|{ expression } { statementlist }
|
||||
default { statementlist }
|
||||
}
|
||||
|
||||
# or
|
||||
|
||||
switch [-regex|-wildcard|-exact][-casesensitive] -file filename
|
||||
{
|
||||
"string"|number|variable|{ expression } { statementlist }
|
||||
default { statementlist }
|
||||
}
|
||||
```
|
||||
|
||||
### Loops
|
||||
|
||||
```ps1
|
||||
# The classic for
|
||||
for(setup; condition; iterator) {
|
||||
# Code here
|
||||
}
|
||||
|
||||
range | % { command }
|
||||
|
||||
foreach (item in iterable) {
|
||||
# Code Here
|
||||
}
|
||||
|
||||
while (condition) {
|
||||
# Code heer
|
||||
}
|
||||
|
||||
do {
|
||||
# Code here
|
||||
} until (condition)
|
||||
|
||||
do {
|
||||
# Code here
|
||||
} while (condition)
|
||||
```
|
||||
|
||||
### Operators
|
||||
|
||||
```ps1
|
||||
# Conditionals
|
||||
$a -eq $b # is equal to
|
||||
$a -ne $b # in not equal to
|
||||
$a -gt $b # greater than
|
||||
$a -ge $b # greater than or equal to
|
||||
$a -lt $b # less than
|
||||
$a -le $b # less than or equal to
|
||||
|
||||
# Logical
|
||||
$true -And $False
|
||||
$True -Or $False
|
||||
-Not $True
|
||||
```
|
||||
|
||||
### Exception Handling
|
||||
|
||||
```ps1
|
||||
try {} catch {} finally {}
|
||||
try {} catch [System.NullReferenceException] {
|
||||
echo $_.Exception | Format-List -Force
|
||||
}
|
||||
```
|
||||
|
||||
## [Functions](https://docs.microsoft.com/en-us/powershell/module/microsoft.powershell.core/about/about_functions?view=powershell-7)
|
||||
|
||||
```ps1
|
||||
function func() {}
|
||||
|
||||
# function with named parameters
|
||||
function func ([type]$param=default_value, ...) { }
|
||||
function func {
|
||||
param([type]$param=default_value, ...)
|
||||
|
||||
# statements
|
||||
}
|
||||
|
||||
# function call
|
||||
func argument
|
||||
func -param value
|
||||
|
||||
# switch parameters
|
||||
function func ([switch]$param, ...) { }
|
||||
|
||||
func # param is $false
|
||||
func -param # param is $true
|
||||
```
|
||||
|
||||
If the function defines a `Begin`, `Process` or `End` block, all the code **must reside inside** those blocks. No code will be recognized outside the blocks if any of the blocks are defined.
|
||||
|
||||
If the function has a `Process` keyword, each object in `$input` is removed from `$input` and assigned to `$_`.
|
||||
|
||||
```ps1
|
||||
function [<scope:>]<name> [([type]$parameter1[,[type]$parameter2])]
|
||||
{
|
||||
param([type]$parameter1 [,[type]$parameter2]) # other way to specify named parameters
|
||||
dynamicparam {<statement list>}
|
||||
|
||||
# processing pipelines
|
||||
begin {<statement list>} # runned once, at start of pipeline
|
||||
process {<statement list>} # runned for each item in the pipeline
|
||||
end {<statement list>} # runned once, at end of pipeline
|
||||
}
|
||||
```
|
||||
|
||||
Optionally, it's possible to provide a brief help string that describes the default value of the parameter, by adding the `PSDefaultValue` attribute to the description of the parameter, and specifying the `Help` property of `PSDefaultValue`.
|
||||
|
||||
```ps1
|
||||
function Func {
|
||||
param (
|
||||
[PSDefaultValue(Help = defValue)]
|
||||
$Arg = 100
|
||||
)
|
||||
}
|
||||
```
|
||||
|
||||
## Script Arguments
|
||||
|
||||
### Parsing Script Arguments
|
||||
|
||||
```ps1
|
||||
$args # array of passed arguments
|
||||
$args[$index] # access to the argumnets
|
||||
$args.count # number of arguments
|
||||
```
|
||||
|
||||
### Script Named Arguments
|
||||
|
||||
In `scripts.ps1`:
|
||||
|
||||
```ps1
|
||||
param($param1, $param2, ...) # basic usage
|
||||
param($param1, $param2=defvalue, ...) # with dafault values
|
||||
param([Type] $param1, $param2, ...) # specify a type
|
||||
param([Parameter(Mandatory)]$param1, $param2, ...) # setting a parameter as necessary
|
||||
|
||||
param([switch]$flag=$false, ...) # custom flags
|
||||
```
|
||||
|
||||
In PowerShell:
|
||||
|
||||
```ps1
|
||||
.\script.ps1 arg1 arg2 # order of arguments will determine which data goes in which parameter
|
||||
|
||||
.\script.ps1 -param2 arg2 -param1 arg1 # custom order
|
||||
```
|
||||
|
||||
### Filters
|
||||
|
||||
A filter is a type of function that runs on each object in the pipeline. A filter resembles a function with all its statements in a `Process` block.
|
||||
|
||||
```ps1
|
||||
filter [<scope:>]<name> {<statement list>}
|
||||
```
|
||||
|
||||
## PowerShell Comment-based Help
|
||||
|
||||
The syntax for comment-based help is as follows:
|
||||
|
||||
```ps1
|
||||
# .<help keyword>
|
||||
# <help content>
|
||||
```
|
||||
|
||||
or
|
||||
|
||||
```ps1
|
||||
<#
|
||||
.<help keyword>
|
||||
<help content>
|
||||
#>
|
||||
```
|
||||
|
||||
Comment-based help is written as a series of comments. You can type a comment symbol `#` before each line of comments, or you can use the `<#` and `#>` symbols to create a comment block. All the lines within the comment block are interpreted as comments.
|
||||
|
||||
All of the lines in a comment-based help topic must be contiguous. If a comment-based help topic follows a comment that is not part of the help topic, there must be at least one blank line between the last non-help comment line and the beginning of the comment-based help.
|
||||
|
||||
Keywords define each section of comment-based help. Each comment-based help keyword is preceded by a dot `.`. The keywords can appear in any order. The keyword names are not case-sensitive.
|
||||
|
||||
### .SYNOPSIS
|
||||
|
||||
A brief description of the function or script. This keyword can be used only once in each topic.
|
||||
|
||||
### .DESCRIPTION
|
||||
|
||||
A detailed description of the function or script. This keyword can be used only once in each topic.
|
||||
|
||||
### .PARAMETER
|
||||
|
||||
The description of a parameter. Add a `.PARAMETER` keyword for each parameter in the function or script syntax.
|
||||
|
||||
Type the parameter name on the same line as the `.PARAMETER` keyword. Type the parameter description on the lines following the `.PARAMETER` keyword. Windows PowerShell interprets all text between the `.PARAMETER` line and the next keyword or the end of the comment block as part of the parameter description. The description can include paragraph breaks.
|
||||
|
||||
```ps1
|
||||
.PARAMETER <Parameter-Name>
|
||||
```
|
||||
|
||||
The Parameter keywords can appear in any order in the comment block, but the function or script syntax determines the order in which the parameters (and their descriptions) appear in help topic. To change the order, change the syntax.
|
||||
|
||||
You can also specify a parameter description by placing a comment in the function or script syntax immediately before the parameter variable name. For this to work, you must also have a comment block with at least one keyword.
|
||||
|
||||
If you use both a syntax comment and a `.PARAMETER` keyword, the description associated with the `.PARAMETER` keyword is used, and the syntax comment is ignored.
|
||||
|
||||
```ps1
|
||||
<#
|
||||
.SYNOPSIS
|
||||
Short description here
|
||||
#>
|
||||
function Verb-Noun {
|
||||
[CmdletBinding()]
|
||||
param (
|
||||
# This is the same as .Parameter
|
||||
[string]$Computername
|
||||
)
|
||||
# Verb the Noun on the computer
|
||||
}
|
||||
```
|
||||
|
||||
### .EXAMPLE
|
||||
|
||||
A sample command that uses the function or script, optionally followed by sample output and a description. Repeat this keyword for each example.
|
||||
|
||||
### .INPUTS
|
||||
|
||||
The .NET types of objects that can be piped to the function or script. You can also include a description of the input objects.
|
||||
|
||||
### .OUTPUTS
|
||||
|
||||
The .NET type of the objects that the cmdlet returns. You can also include a description of the returned objects.
|
||||
|
||||
### .NOTES
|
||||
|
||||
Additional information about the function or script.
|
||||
|
||||
### .LINK
|
||||
|
||||
The name of a related topic. The value appears on the line below the `.LINK` keyword and must be preceded by a comment symbol `#` or included in the comment block.
|
||||
|
||||
Repeat the `.LINK` keyword for each related topic.
|
||||
|
||||
This content appears in the Related Links section of the help topic.
|
||||
|
||||
The `.Link` keyword content can also include a Uniform Resource Identifier (URI) to an online version of the same help topic. The online version opens when you use the **Online** parameter of `Get-Help`. The URI must begin with "http" or "https".
|
||||
|
||||
### .COMPONENT
|
||||
|
||||
The name of the technology or feature that the function or script uses, or to which it is related. The **Component** parameter of `Get-Help` uses this value to filter the search results returned by `Get-Help`.
|
||||
|
||||
### .ROLE
|
||||
|
||||
The name of the user role for the help topic. The **Role** parameter of `Get-Help` uses this value to filter the search results returned by `Get-Help`.
|
||||
|
||||
### .FUNCTIONALITY
|
||||
|
||||
The keywords that describe the intended use of the function. The **Functionality** parameter of `Get-Help` uses this value to filter the search results returned by `Get-Help`.
|
||||
|
||||
### .FORWARDHELPTARGETNAME
|
||||
|
||||
Redirects to the help topic for the specified command. You can redirect users to any help topic, including help topics for a function, script, cmdlet, or provider.
|
||||
|
||||
```ps1
|
||||
# .FORWARDHELPTARGETNAME <Command-Name>
|
||||
```
|
||||
|
||||
### .FORWARDHELPCATEGORY
|
||||
|
||||
Specifies the help category of the item in `.ForwardHelpTargetName`. Valid values are `Alias`, `Cmdlet`, `HelpFile`, `Function`, `Provider`, `General`, `FAQ`, `Glossary`, `ScriptCommand`, `ExternalScript`, `Filter`, or `All`. Use this keyword to avoid conflicts when there are commands with the same name.
|
||||
|
||||
```ps1
|
||||
# .FORWARDHELPCATEGORY <Category>
|
||||
```
|
||||
|
||||
### .REMOTEHELPRUNSPACE
|
||||
|
||||
Specifies a session that contains the help topic. Enter a variable that contains a **PSSession** object. This keyword is used by the [Export-PSSession](https://docs.microsoft.com/en-us/powershell/module/microsoft.powershell.utility/export-pssession?view=powershell-7)
|
||||
cmdlet to find the help topics for the exported commands.
|
||||
|
||||
```ps1
|
||||
# .REMOTEHELPRUNSPACE <PSSession-variable>
|
||||
```
|
||||
|
||||
### .EXTERNALHELP
|
||||
|
||||
Specifies an XML-based help file for the script or function.
|
||||
|
||||
```ps1
|
||||
# .EXTERNALHELP <XML Help File>
|
||||
|
||||
```
|
||||
|
||||
The `.ExternalHelp` keyword is required when a function or script is documented in XML files. Without this keyword, `Get-Help` cannot find the XML-based help file for the function or script.
|
||||
|
||||
The `.ExternalHelp` keyword takes precedence over other comment-based help keywords. If `.ExternalHelp` is present, `Get-Help` does not display comment-based help, even if it cannot find a help topic that matches the value of the `.ExternalHelp` keyword.
|
||||
|
||||
If the function is exported by a module, set the value of the `.ExternalHelp` keyword to a filename without a path. `Get-Help` looks for the specified file name in a language-specific subdirectory of the module directory. There are no requirements for the name of the XML-based help file for a function, but a best practice is to use the following format:
|
||||
|
||||
```ps1
|
||||
<ScriptModule.psm1>-help.xml
|
||||
```
|
||||
|
||||
If the function is not included in a module, include a path to the XML-based help file. If the value includes a path and the path contains UI-culture-specific subdirectories, `Get-Help` searches the subdirectories
|
||||
recursively for an XML file with the name of the script or function in accordance with the language fallback standards established for Windows, just as it does in a module directory.
|
||||
|
||||
For more information about the cmdlet help XML-based help file format, see [How to Write Cmdlet Help](https://go.microsoft.com/fwlink/?LinkID=123415) in the MSDN library.
|
||||
***
|
||||
|
||||
## Project Oriented Programming
|
||||
|
||||
### Classes
|
||||
|
||||
```ps1
|
||||
[class]::func() # use function from a static class
|
||||
[class]::attribute # access to static class attribute
|
||||
```
|
BIN
Python/Anaconda/Anaconda-Starter-Guide.pdf
Normal file
BIN
Python/Anaconda/Anaconda-Starter-Guide.pdf
Normal file
Binary file not shown.
BIN
Python/Anaconda/conda-cheatsheet.pdf
Normal file
BIN
Python/Anaconda/conda-cheatsheet.pdf
Normal file
Binary file not shown.
Some files were not shown because too many files have changed in this diff Show more
Loading…
Add table
Add a link
Reference in a new issue